Arithmetic operators in C++ are symbols used to perform basic mathematical operations, including addition, subtraction, multiplication, division, and modulus.
#include <iostream>
int main() {
int a = 10, b = 3;
std::cout << "Addition: " << (a + b) << '\n';
std::cout << "Subtraction: " << (a - b) << '\n';
std::cout << "Multiplication: " << (a * b) << '\n';
std::cout << "Division: " << (a / b) << '\n';
std::cout << "Modulus: " << (a % b) << '\n';
return 0;
}
What are Arithmetic Operators?
Arithmetic operators are fundamental symbols used in programming to perform mathematical calculations. In C++, arithmetic operators are used to manipulate numerical data and execute mathematical operations such as addition, subtraction, multiplication, division, and modulus. Understanding these operators is crucial for writing effective C++ code, as they enable developers to create complex mathematical expressions with ease.
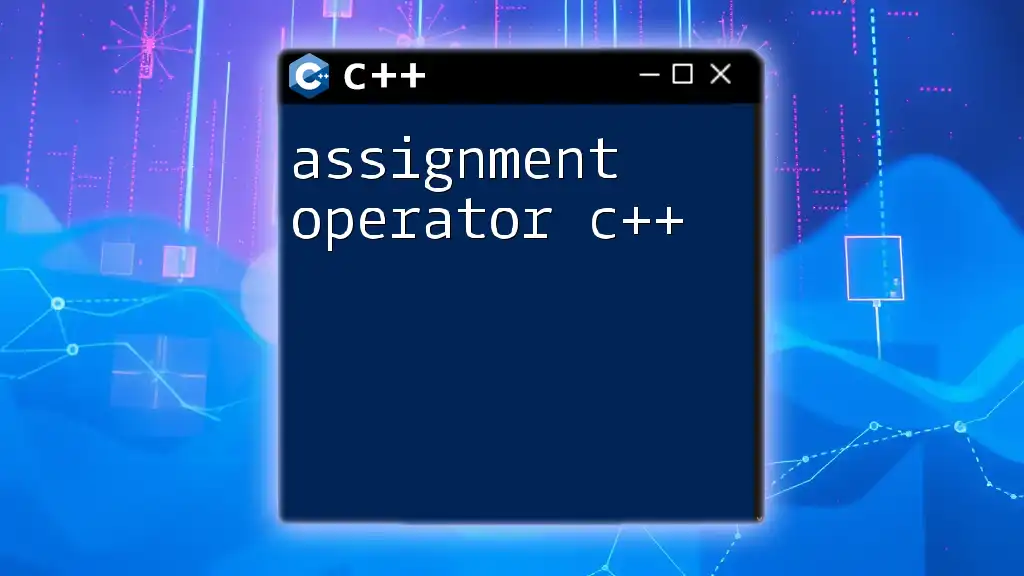
Types of C++ Arithmetic Operators
Addition Operator (+)
The addition operator is used to calculate the sum of two operands. It is one of the most frequently used arithmetic operations in C++ programming.
Syntax:
result = operand1 + operand2;
Example:
int a = 5;
int b = 10;
int sum = a + b; // sum is 15
Use Case: The addition operator is commonly used in scenarios where you need to combine quantities, calculate totals, or increase values.
Subtraction Operator (-)
The subtraction operator is used to find the difference between two operands. It allows you to decrease the value of one operand by the value of another.
Syntax:
result = operand1 - operand2;
Example:
int a = 15;
int b = 5;
int difference = a - b; // difference is 10
Use Case: This operator is particularly useful in financial calculations, where you might need to find out how much money is left after expenses.
Multiplication Operator (*)
The multiplication operator calculates the product of two operands, making it easy to perform scaling and repeated addition.
Syntax:
result = operand1 * operand2;
Example:
int a = 4;
int b = 5;
int product = a * b; // product is 20
Use Case: Useful in situations that require calculation of area, volume, or repeated operations like total price in a shopping cart.
Division Operator (/)
The division operator computes the quotient of two operands. It is important to note the behavior of integer and floating-point divisions in C++.
Syntax:
result = operand1 / operand2;
Example:
int a = 20;
int b = 4;
int quotient = a / b; // quotient is 5
Use Case: Division is frequently used in averaging calculations or distributing resources evenly.
Modulus Operator (%)
The modulus operator returns the remainder of a division operation. This operator is immensely valuable when you want to determine evenness or oddness or when dividing into groups.
Syntax:
result = operand1 % operand2;
Example:
int a = 14;
int b = 3;
int remainder = a % b; // remainder is 2
Use Case: It is commonly employed in scenarios such as determining if numbers are divisible or in looping structures that require periodic execution.
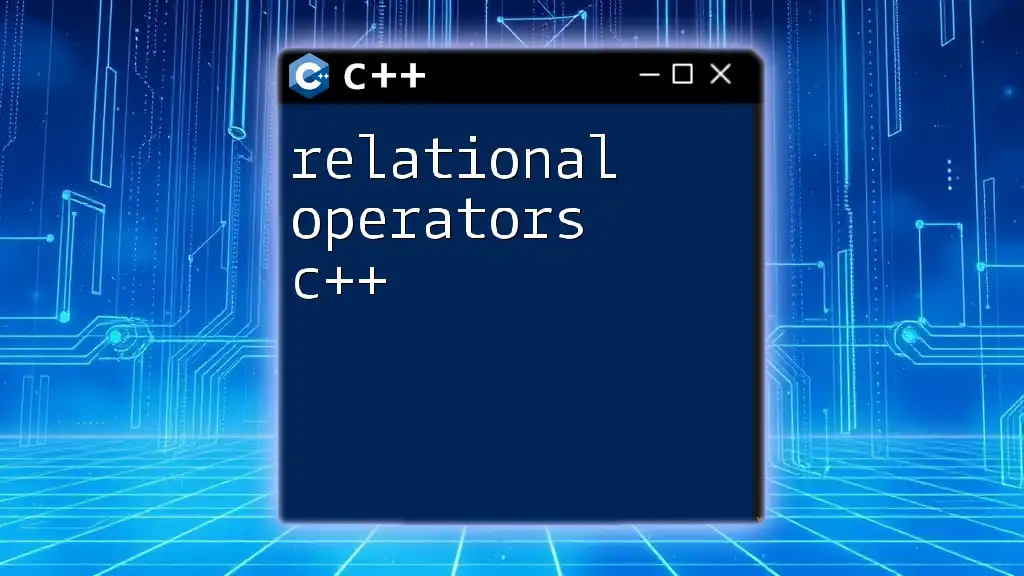
Precedence of C++ Arithmetic Operators
Operator precedence determines the order in which operators in an expression are evaluated. Understanding this concept is critical to ensure that calculations yield the expected results.
For instance, multiplication and division operators have higher precedence than addition and subtraction. This means they are evaluated first in expressions without parentheses.
Example:
int result = 10 + 20 * 3; // result will be 70, not 90 because of precedence
To avoid confusion, it's often advisable to use parentheses to make the order of operations explicit:
int result = (10 + 20) * 3; // result will be 90
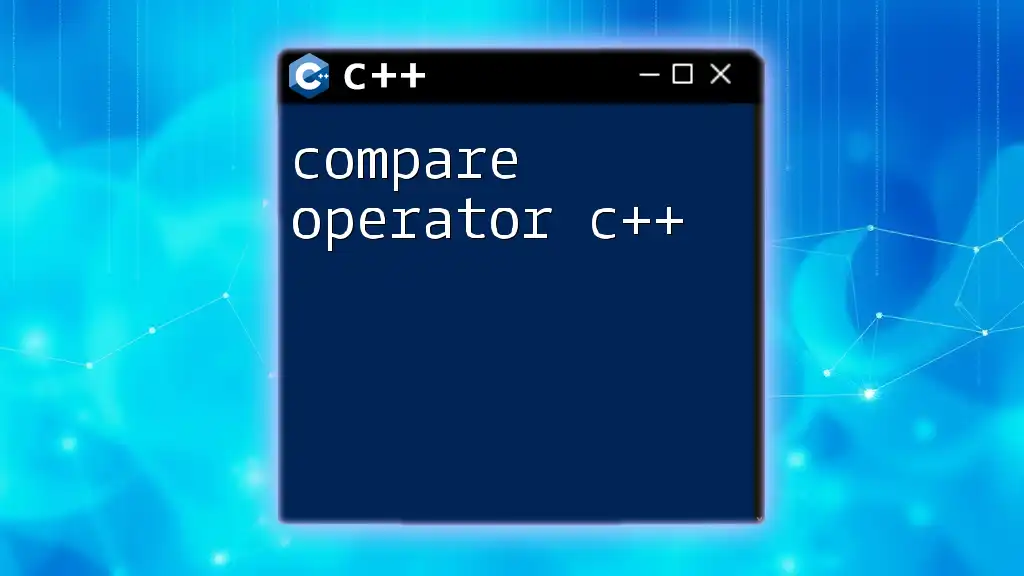
Combining Arithmetic Operators
Using multiple arithmetic operators within a single expression is commonplace in C++. However, it is essential to adhere to the operator precedence rules to ensure accuracy.
Example:
int a = 5;
int b = 2;
int c = 3;
int result = a + b * c; // result is 11 because multiplication takes precedence over addition
Best Practices: To aid clarity in your code, use parentheses when combining operators. This practice not only makes the code readable but also reduces errors in computations.
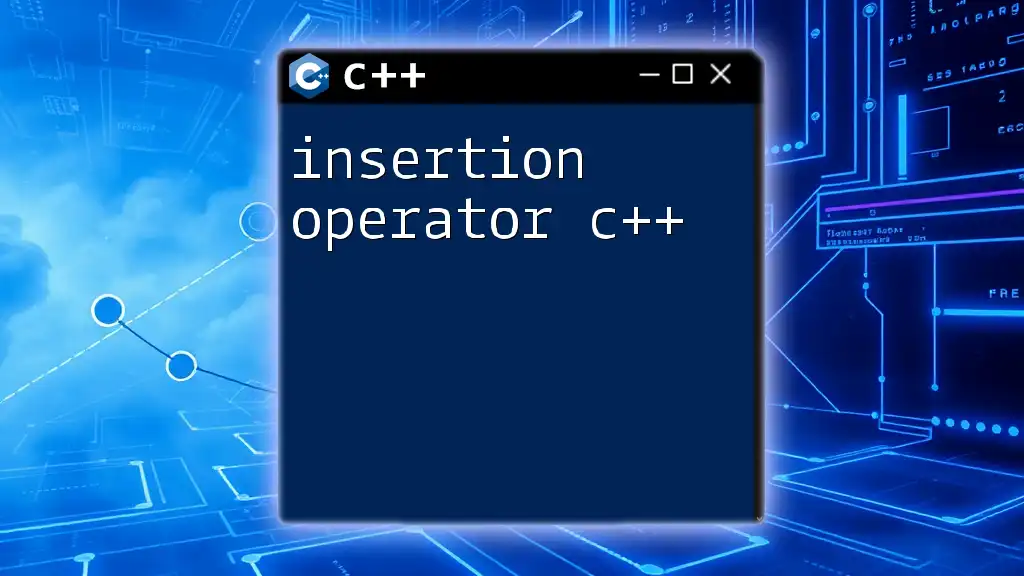
Common Errors with C++ Arithmetic Operators
While working with arithmetic operators, it is vital to be mindful of certain pitfalls that can lead to runtime errors or incorrect outputs:
- Division by Zero: Attempting to divide a number by zero will result in a runtime error. Always add checks to ensure the denominator is not zero before performing division.
Example:
int a = 10;
int b = 0;
// This will cause a runtime error
int divide = a / b;
- Integer Overflow: When the result of an arithmetic operation exceeds the maximum limit of the data type being used, it results in overflow. This can lead to unexpected behavior and should be managed effectively by using appropriate data types or checks.
Understanding these issues and addressing them in your code is essential to writing robust C++ programs.
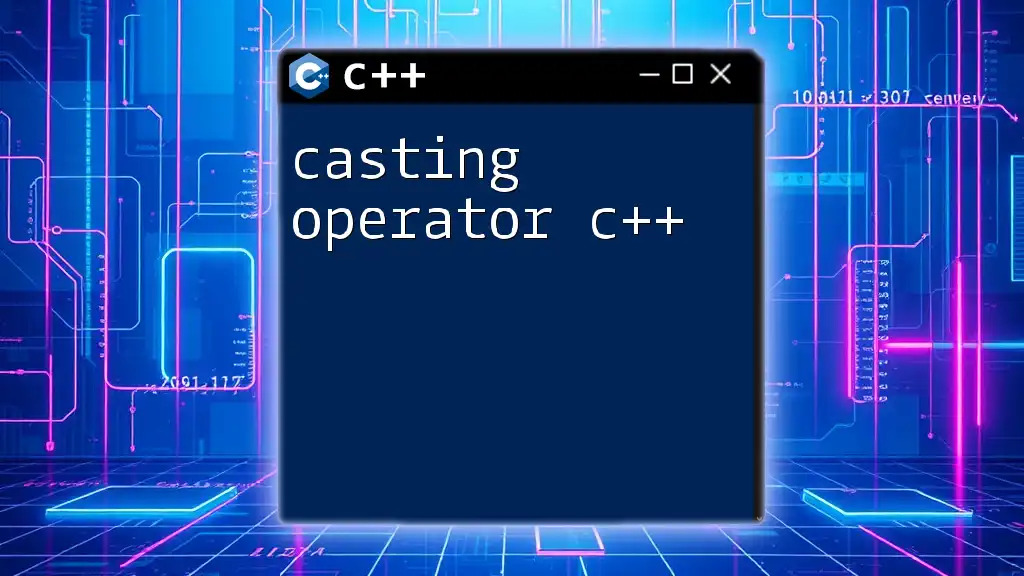
Conclusion
Mastering arithmetic operators in C++ is foundational for any programmer. These operators form the bedrock of mathematical operations and logic in your code. From simple calculations to more complex expressions, understanding how to use these operators allows for more efficient programming and better problem-solving skills. By practicing these concepts regularly, developers can become proficient in crafting effective and optimized C++ code.
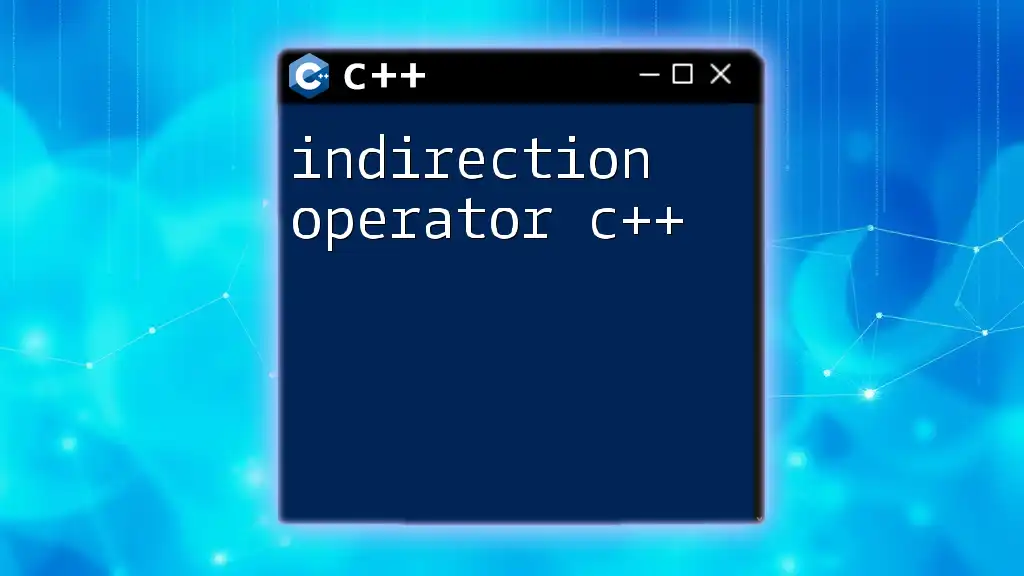
Additional Resources
For those eager to deepen their understanding of C++ arithmetic operations, consider exploring:
- Recommended books on C++ programming
- Online courses that provide interactive learning
- Coding practice platforms that focus on problem-solving with arithmetic operations
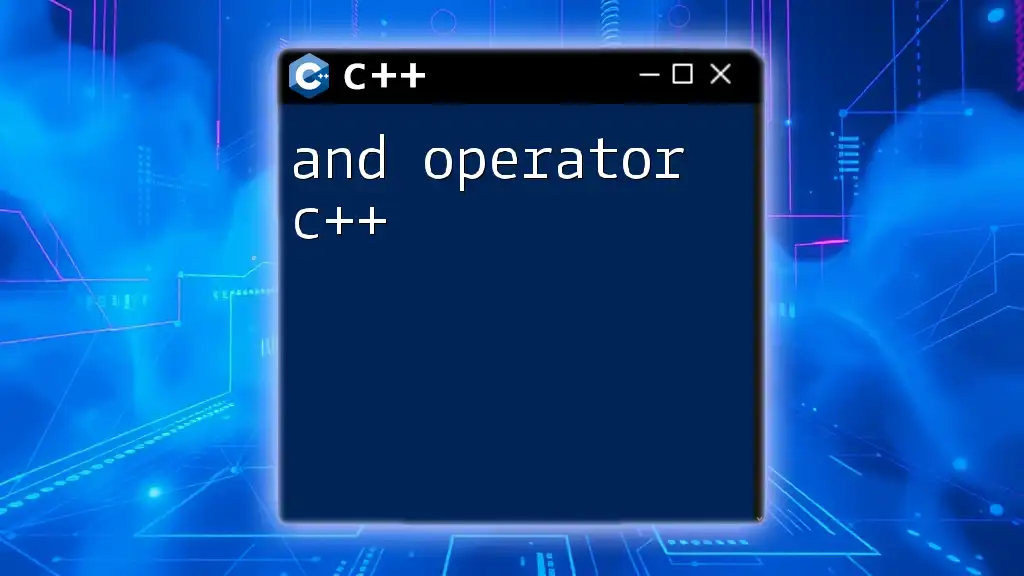
Frequently Asked Questions (FAQ)
What are the basic arithmetic operations in C++?
The basic arithmetic operations in C++ are addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
How do C++ arithmetic operators differ from other programming languages?
While the arithmetic operators themselves are similar across many programming languages, specific behaviors like integer division, overflow handling, and operator precedence may vary.
Can I use arithmetic operators on strings in C++?
No, arithmetic operators cannot be applied to strings directly. However, you may need to convert strings to numerical data types before performing arithmetic operations on their values.