The indirection operator (`*`) in C++ is used to access the value at a specific memory address that a pointer is pointing to.
Here's a simple example using the indirection operator in C++:
#include <iostream>
int main() {
int value = 42;
int* ptr = &value; // pointer to the variable 'value'
std::cout << "The value is: " << *ptr << std::endl; // using indirection operator to access the value
return 0;
}
What is the Indirection Operator?
Definition
In C++, the indirection operator, denoted by an asterisk (`*`), is a crucial part of working with pointers. A pointer is a variable that stores the memory address of another variable. The indirection operator allows us to access or manipulate the value stored at that memory address, effectively "dereferencing" the pointer.
Syntax
The syntax for the indirection operator is straightforward. You can declare a pointer using `*`, and then use the same symbol to dereference it:
int* ptr; // Declaration of a pointer to an integer
int value = 10; // A regular integer variable
ptr = &value; // Assigning the address of value to ptr
int dereferencedValue = *ptr; // Dereferencing the pointer to get the value
Here, `*ptr` allows us to access the value that `ptr` points to, which is `10`.
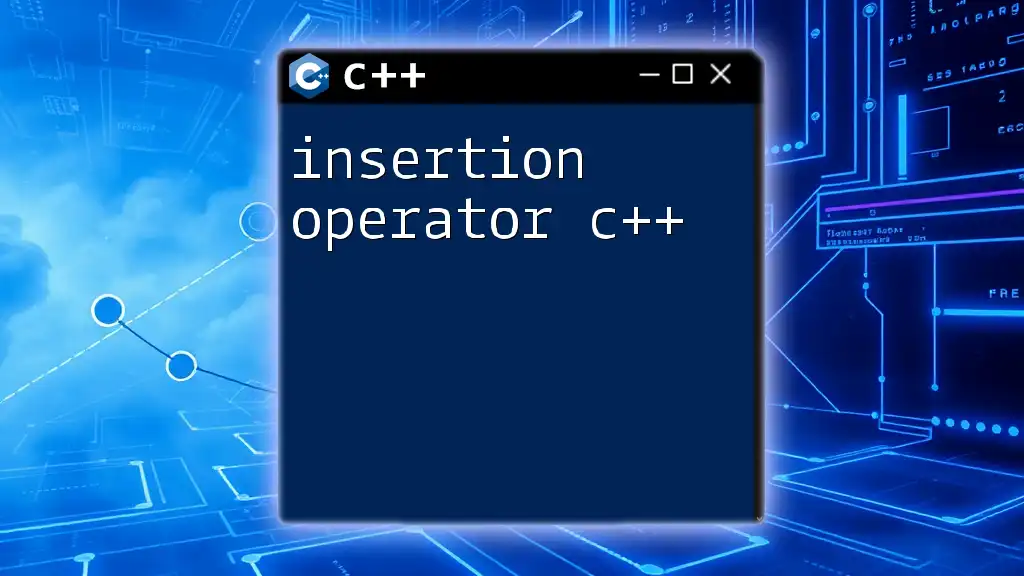
How the Indirection Operator Works
Pointers and Memory Addressing
Understanding pointers is essential for effective C++ programming. A pointer points to the memory address of another variable, allowing for low-level memory manipulation and efficient management of dynamic data structures. When you declare a pointer, it's vital to recognize that it doesn't store the value but rather the address of the variable it references.
Dereferencing with Indirection Operator
When you use the indirection operator, you dereference a pointer, fetching the value stored at its associated address. This capability is essential for various operations in C++, and it facilitates dynamic memory allocation, among other use cases.
Here’s a simple example demonstrating dereferencing:
int num = 42; // Declare an integer
int* numPtr = # // Create a pointer pointing to num
int retrievedNum = *numPtr; // Dereference numPtr to get the value of num
In this case, `retrievedNum` will hold the value `42` after dereferencing.
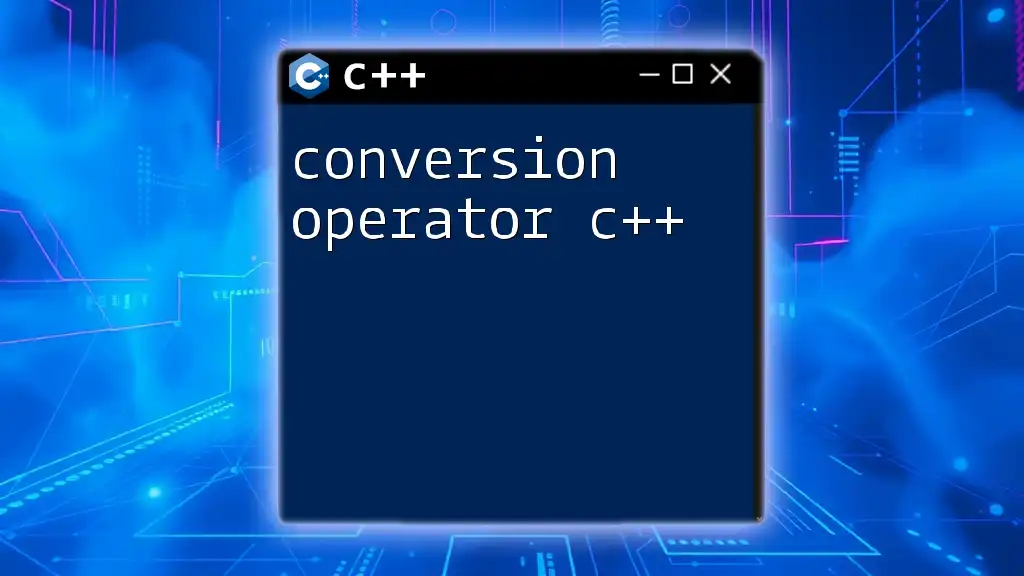
Practical Examples of Using the Indirection Operator
Basic Usage
Let's see some basic usage of the indirection operator in action.
int a = 5; // Declare an integer variable
int* aPtr = &a; // Create a pointer to a
*aPtr = 10; // Use the indirection operator to modify the value of a
// Now, a will be equal to 10.
In this snippet, we’ve changed the value of `a` by dereferencing `aPtr` and assigning a new value. This illustrates how powerful the indirection operator can be for manipulating variables.
Manipulating Values
The indirection operator can also play a significant role in modifying variable values via pointers.
void updateValue(int* ptr) {
*ptr = 20; // Modify value at the address pointed by ptr
}
int b = 15;
updateValue(&b); // Pass the address of b to our function
// Now, b will be equal to 20.
In this example, we’ve defined a function that takes an integer pointer and updates its value. This demonstrates how we can use pointers to pass variables to functions by reference.
Array and Indirection
Arrays and pointers in C++ are closely related, and the indirection operator can be utilized to access array elements directly.
int arr[] = {1, 2, 3, 4, 5};
int* arrPtr = arr; // Points to the first element of arr
int firstElement = *arrPtr; // Dereferences to get the first element, which is 1
In this case, `arrPtr` points to the first element of the array, and when we dereference it with the indirection operator, we retrieve the first element.
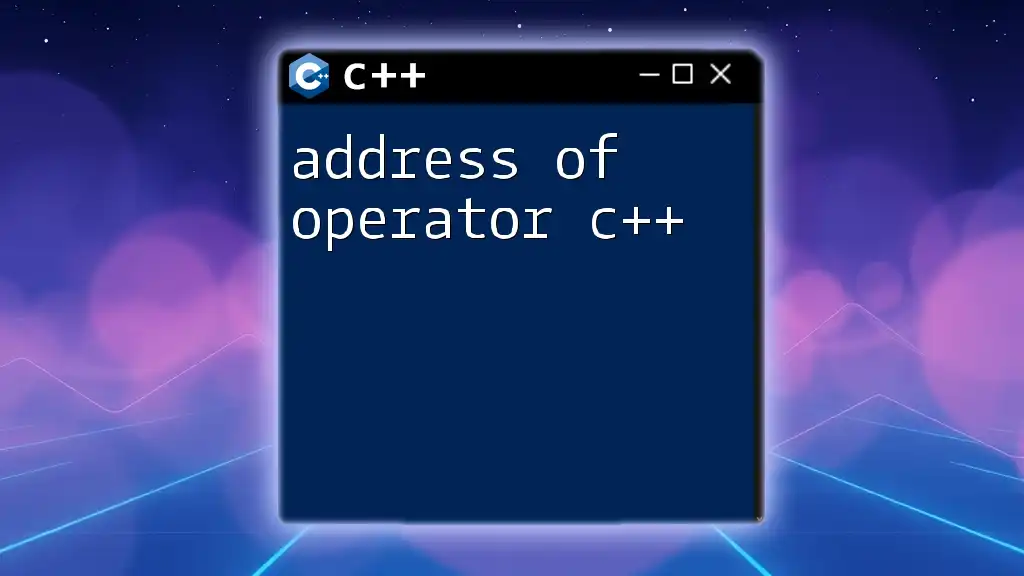
Common Mistakes When Using the Indirection Operator
Dereferencing Null Pointers
One of the most critical mistakes in C++ programming involves dereferencing null pointers. Failing to check whether a pointer is initialized can lead to undefined behavior and program crashes.
int* nullPtr = nullptr;
int value = *nullPtr; // Dereferencing a null pointer!
This example will likely lead to a runtime error. Always ensure that pointers are valid before dereferencing them.
Confusion Between Operators
C++ has several pointer-related operators that can confuse newcomers:
- The indirection operator (`*`) is used to dereference pointers.
- The address-of operator (`&`) gives the memory address of a variable.
int c = 30;
int* cPtr = &c; // Using & to get the address of c
int valueC = *cPtr; // Using * to get the value of c
In this snippet, `&c` fetches the address of `c`, while `*cPtr` allows access to the value at that address.
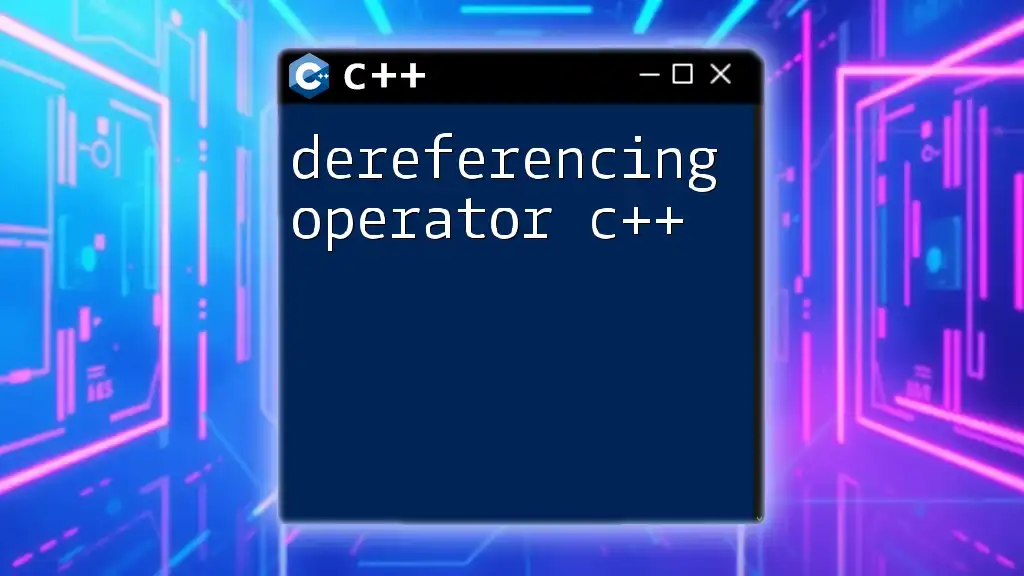
Best Practices When Using the Indirection Operator
Safe Pointer Practices
Using pointers does come with risks, but several best practices can help:
-
Initialize Pointers: Always initialize pointers to avoid undefined behavior. Prefer using `nullptr` for null pointers rather than leaving them uninitialized.
-
Use Smart Pointers: Consider using smart pointers (`std::unique_ptr`, `std::shared_ptr`) from the C++11 standard library when applicable. They manage memory automatically, reducing memory leaks and improving safety.
Comments and Documentation
When using pointers and the indirection operator, clear documentation and comments in your code are vital. Explain what each pointer does and why it’s necessary. Clear code promotes understandability, making your work more accessible to others (or even your future self!).
// Pointer to an integer
int* numberPointer = new int; // Dynamically allocate memory
*numberPointer = 50; // Set the value of the allocated integer
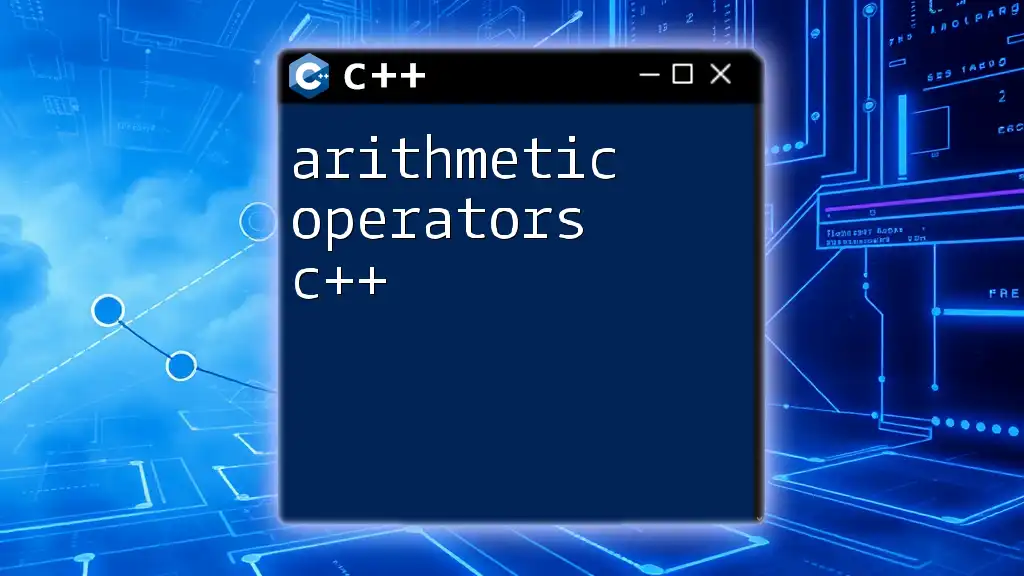
Conclusion
The indirection operator in C++ is a powerful feature that allows for direct manipulation of memory and variables through pointers. Understanding pointers, how to dereference them, and recognizing common pitfalls are all crucial for effective use of this operator. By following best practices and maintaining clear documentation, you can leverage the full power of C++ while minimizing risks.
Feel free to explore more about pointers and the `indirection operator c++`, practice these concepts, and enhance your C++ skills!