The address-of operator (`&`) in C++ is used to obtain the memory address of a variable.
int main() {
int var = 10;
int* ptr = &var; // ptr now holds the address of var
return 0;
}
Understanding the Basics of Pointers
What is a Pointer?
In C++, a pointer is a variable that holds the memory address of another variable. This allows for dynamic data manipulation and efficient memory management. Pointers are fundamental in C++ programming as they provide powerful features such as dynamic memory allocation and the ability to pass variables by reference to functions.
Here’s a simple example of pointer declaration and initialization:
int var = 10;
int *ptr = &var; // ptr now holds the address of var
In this example, `var` is an integer variable, and `ptr` is a pointer that holds the address of `var` using the address of operator `&`.
The Concept of Memory Address
Every variable you declare in a program is stored in memory. Each memory location has a unique address that you can access via the address of operator. Understanding this concept is crucial as it lays the groundwork for working with pointers.
You can display the memory address of a variable like this:
std::cout << "Address of var: " << &var << std::endl;
This outputs the memory address where `var` is stored, demonstrating how variables reside in memory.
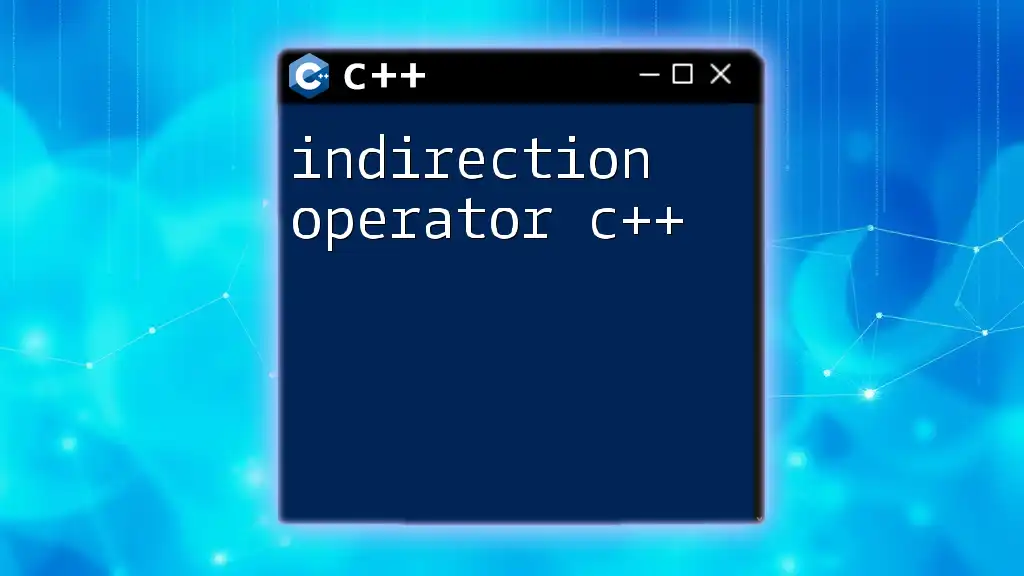
The Address of Operator (&)
Definition and Syntax
The address of operator (`&`) is used to retrieve the memory address of a variable. Its primary function in C++ is to return the address of the variable it's applied to, allowing programmers to manipulate memory directly.
Here's how you can use the address of operator in C++:
int value = 5;
int *address = &value; // Using & to get the address of value
In this snippet, `address` now points to the memory location of `value`.
How to Use the Address of Operator
Declaring Pointers
To declare a pointer, you need to use the address of operator. This is how you can declare a pointer and initialize it with the address of a variable:
int a = 10;
int *p = &a; // Assigning address of a to pointer p
Here, `p` now holds the address of `a`, and you can manipulate the value of `a` through this pointer.
Accessing Variable Value via Pointer
To access the value of a variable via a pointer, you must dereference the pointer using the asterisk operator (`*`). Here’s an example:
std::cout << "Value of a through pointer: " << *p << std::endl; // Dereferencing
This line will output the value of `a`. The `*` operator allows you to access the value at the memory address stored in `p`.
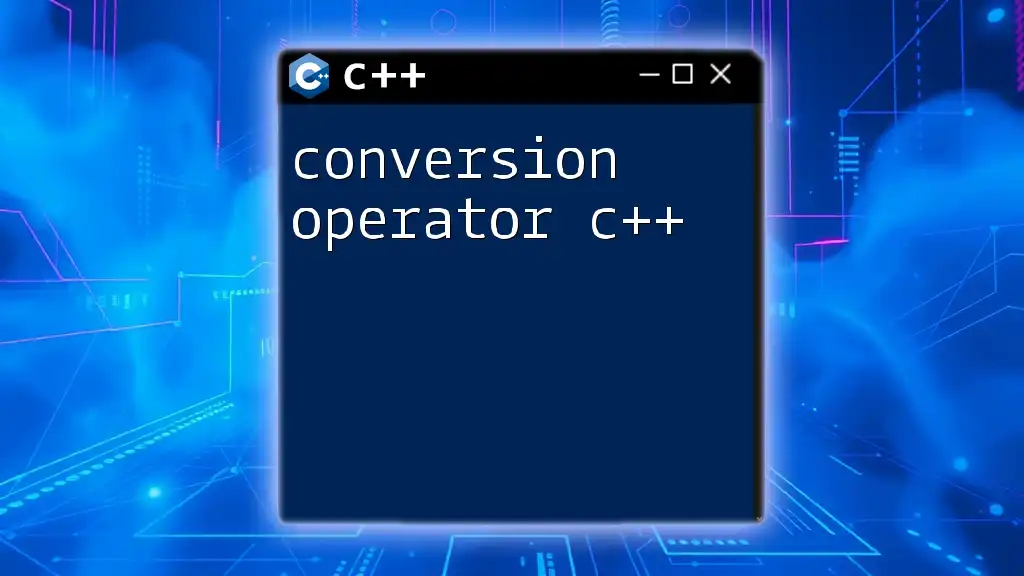
Practical Applications of Address of Operator
Dynamic Memory Allocation
One of the most essential applications of the address of operator is in dynamic memory allocation. Using `new` and `delete`, you can allocate and deallocate memory during runtime. Here's an example:
int *arr = new int[5]; // Allocate memory for an array of 5 integers
In this code, `arr` now points to a dynamically allocated block of memory sufficient to hold five integers. Remember to deallocate memory once it is no longer needed:
delete[] arr; // Free the allocated memory
Function Arguments via Pointers
Another common use case is passing variables to functions by reference using pointers. This enables the function to modify the original variable. Here’s how:
void increment(int *num) {
(*num)++; // Increment the value at the memory address pointed to by num
}
increment(&value); // Passing address of value
In this example, the function `increment` takes a pointer as an argument, allowing it to increment the value of `value` directly by calling it with `&value`.
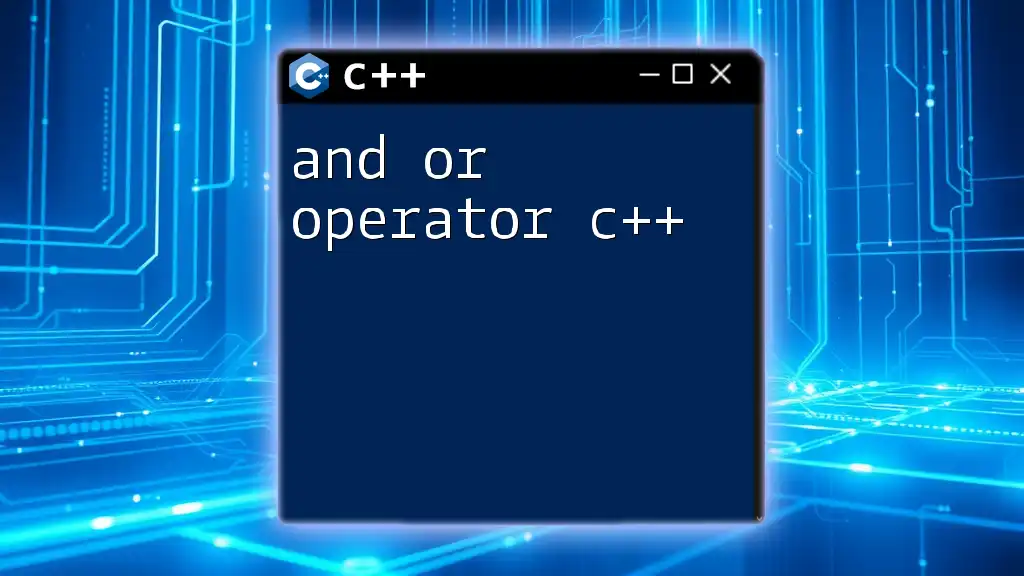
Common Mistakes and Misconceptions
Confusing `&` with Logical AND
A common mistake among beginners is confusing the address of operator `&` with the logical AND operator (`&&`). While both symbols look similar, their functions are entirely different. Here’s how they differ:
For logical AND:
if (a && b) {
std::cout << "Both are true." << std::endl;
}
For the address of operator:
int *ptr = &a; // Correct usage of address of operator
Understanding the distinction is vital to avoid syntax errors in your code.
Forgetting to Dereference a Pointer
A typical pitfall arises when you forget to dereference a pointer to access its value. Failing to do so can lead to confusion about what a pointer is referencing. Consider the following example:
std::cout << *ptr << std::endl; // Correct usage
std::cout << ptr << std::endl; // This will print the address, not the value
In the first line, the pointer is correctly dereferenced, displaying the value it points to. The second line, however, will show the address stored in `ptr`, leading to misconceptions.
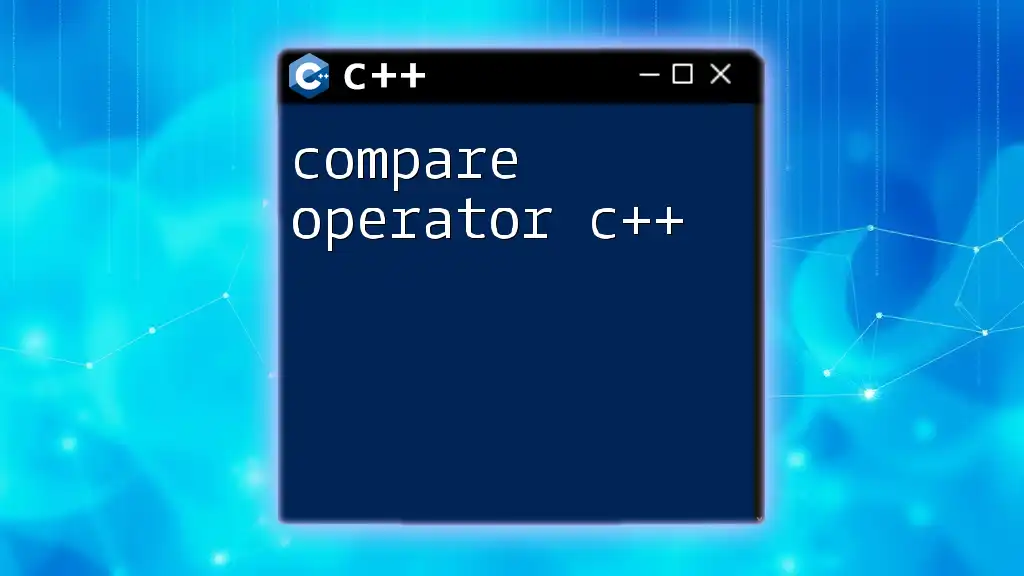
Conclusion
Understanding the address of operator in C++ is foundational for efficient memory management and effective programming. With pointers, you gain greater control over memory, allowing for dynamic resource usage and manipulation. Embrace the math behind addresses and pointers, and practice these concepts to become proficient in C++. The world of C++ programming opens up numerous possibilities when you master pointers. For further reading, consider diving into respected books and courses on C++ to deepen your comprehension.