The compare operator in C++ is used to compare two values, returning true if the first value is less than, equal to, or greater than the second value, facilitating decisions in control flow.
Here's a code snippet demonstrating the use of the compare operator:
#include <iostream>
int main() {
int a = 5;
int b = 10;
if (a < b) {
std::cout << "a is less than b" << std::endl;
} else if (a > b) {
std::cout << "a is greater than b" << std::endl;
} else {
std::cout << "a is equal to b" << std::endl;
}
return 0;
}
What Are Compare Operators?
Compare operators are fundamental elements in C++ that allow you to evaluate relationships between values, helping determine the flow of a program. In essence, these operators facilitate comparisons between data types—such as integers, floats, and characters—enabling developers to establish conditional logic.
Understanding how to use compare operators effectively is crucial for writing functional, efficient, and logical C++ programs.
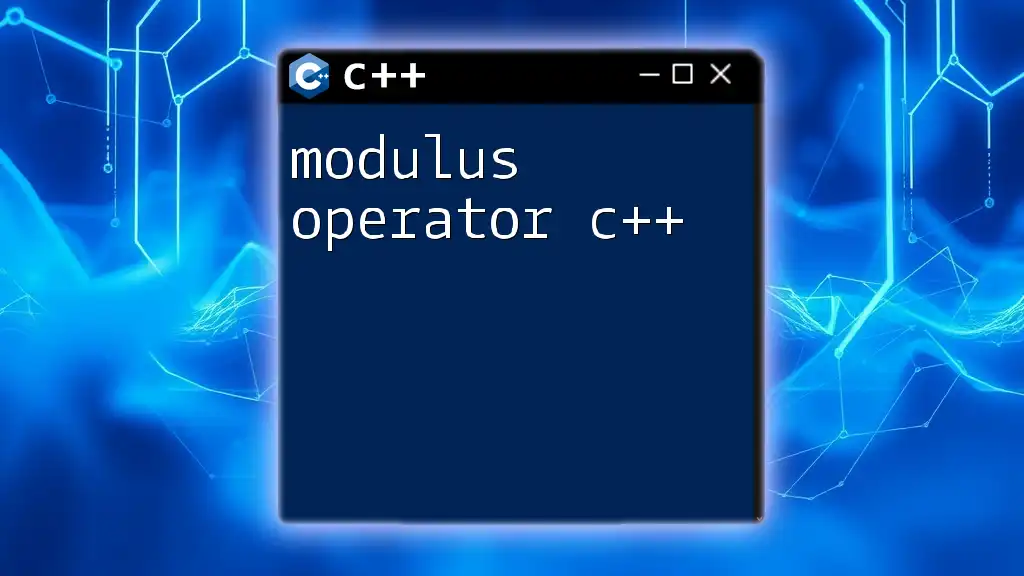
The Role of Compare Operators in Conditional Statements
Compare operators play a vital role in control flow, often used within conditional statements such as `if`, `else if`, and `while` loops. For instance, they help ascertain whether certain conditions are met or to execute different parts of the code accordingly. Using compare operators enhances code readability and clarity, as they directly reflect the logic intended by the developer.
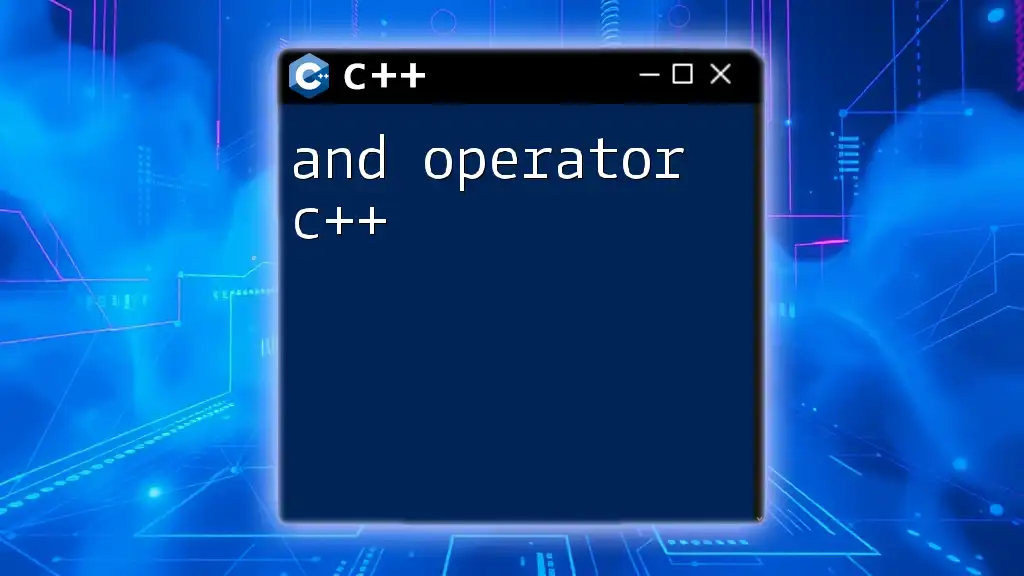
Types of Compare Operators in C++
Basic Comparison Operators
Equal to (`==`)
This operator checks whether two values are identical. The syntax is straightforward and quite intuitive:
if (a == b) {
// Code when a is equal to b
}
With `==`, you are asserting that the values of `a` and `b` are the same. A common pitfall arises when comparing different data types, leading to potential implicit conversions that yield unexpected results.
Not Equal to (`!=`)
In contrast, the `!=` operator assesses whether two values differ. The usage is similar:
if (a != b) {
// Code when a is not equal to b
}
This operator becomes particularly useful in loops or validations, ensuring that a condition is met unless explicitly differentiated.
Relational Comparison Operators
Greater Than (`>`)
The greater than operator evaluates whether one value surpasses another. When implemented, it can result in control flow that depends on size relationships:
if (a > b) {
// Code when a is greater than b
}
This operator is commonly utilized in algorithms requiring comparisons of sorted elements or acquiring maximum values.
Less Than (`<`)
Conversely, the less than operator determines if a value is smaller than another:
if (a < b) {
// Code when a is less than b
}
The `less than` operator is crucial when iterating over ranges or implementing conditional logic based on ordered data.
Greater Than or Equal To (`>=`)
The `>=` operator is a combination of greater than and equals. This means that your condition can pass if both scenarios are true:
if (a >= b) {
// Code when a is greater than or equal to b
}
Utilizing this operator is especially handy in scenarios where boundary conditions need to be considered.
Less Than or Equal To (`<=`)
The less than or equal to operator works similarly:
if (a <= b) {
// Code when a is less than or equal to b
}
This operator can simplify comparisons by condensing conditional statements that would otherwise require an `if-else` structure.
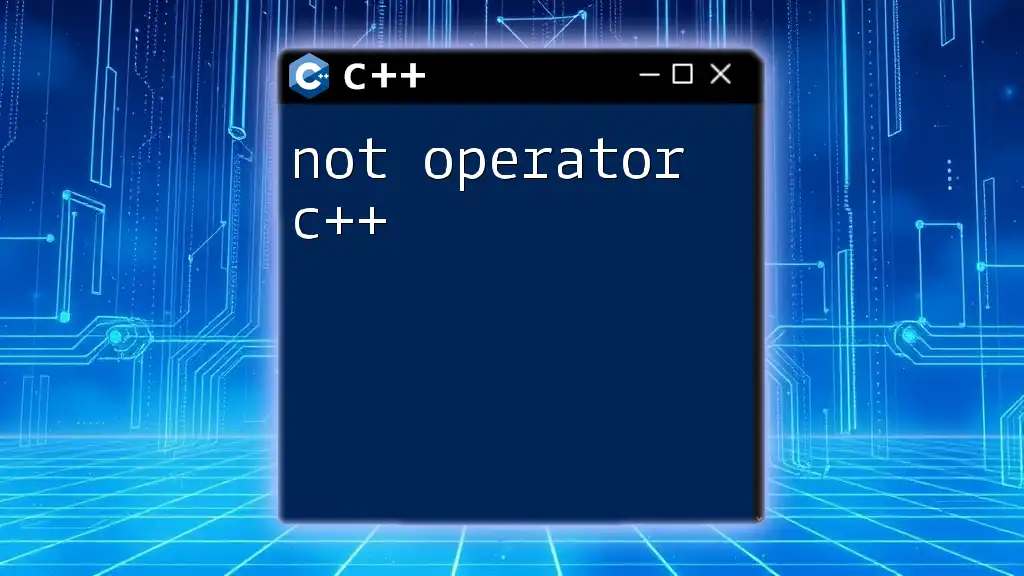
Combining Comparison Operators
Logical Operators with Comparison
C++ allows you to combine multiple comparison conditions, providing a robust framework for decision-making:
Using `&&` (AND)
The logical AND operator allows you to check whether multiple conditions hold true simultaneously:
if (a > b && a < c) {
// Code when a is between b and c
}
This is often employed in range-checking scenarios or validating two separate conditions before proceeding.
Using `||` (OR)
Alternatively, the logical OR operator checks if at least one of the conditions is satisfied:
if (a < b || a > c) {
// Code when a is out of bounds of b and c
}
Utilizing `||` can streamline condition checks, especially in validating user inputs or multiple critical thresholds.
Nested Compare Operators
When conditions become complicated, nesting can prove useful and necessary. Nested comparisons allow you to introduce additional checks within existing conditions:
if (a > b) {
if (a > c) {
// Code when a is the greatest
}
}
While powerful, ensure that nested statements remain readable. Overly complex nesting can confuse both the developer and future maintainers.
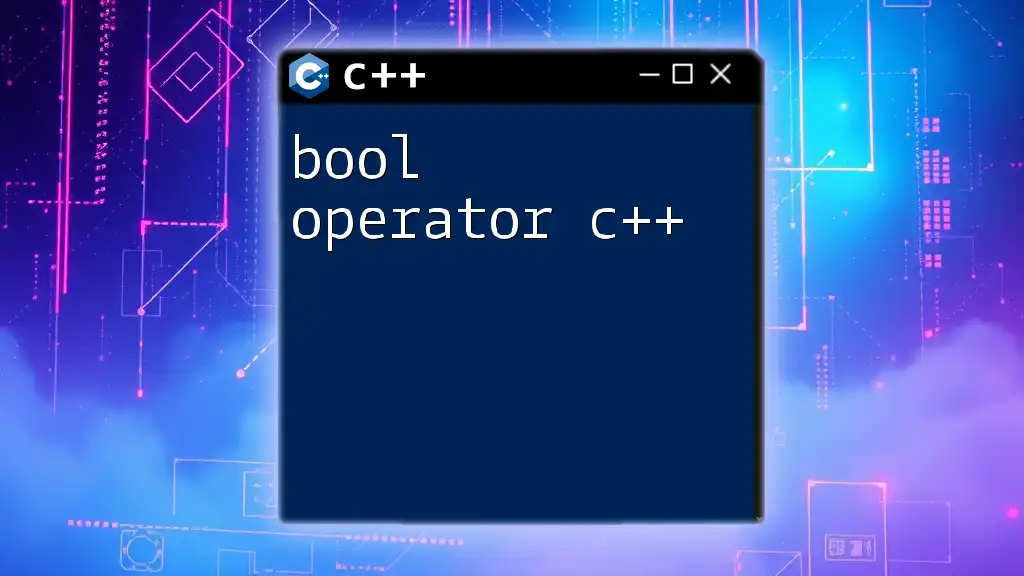
Common Mistakes with Compare Operators
Comparison of Different Data Types
One critical aspect to consider is the compatibility of data types. In C++, `int`, `float`, and `double` have different representation ranges and may yield unexpected results when compared directly.
int a = 5;
double b = 5.0;
if (a == b) {
// Evaluates to true, but understand why
}
While `a` and `b` may seem identical in value, type differences could lead to misleading behavior in complex statements.
Floating Point Comparisons
Comparing floating-point numbers can lead you to unexpected results due to precision errors. They should often not be directly compared for equality:
float a = 0.1f, b = 0.2f;
if (a + b == 0.3f) {
// Potential pitfalls
}
Instead, consider using an epsilon value approach to determine if two floats are "close enough" to being equal.
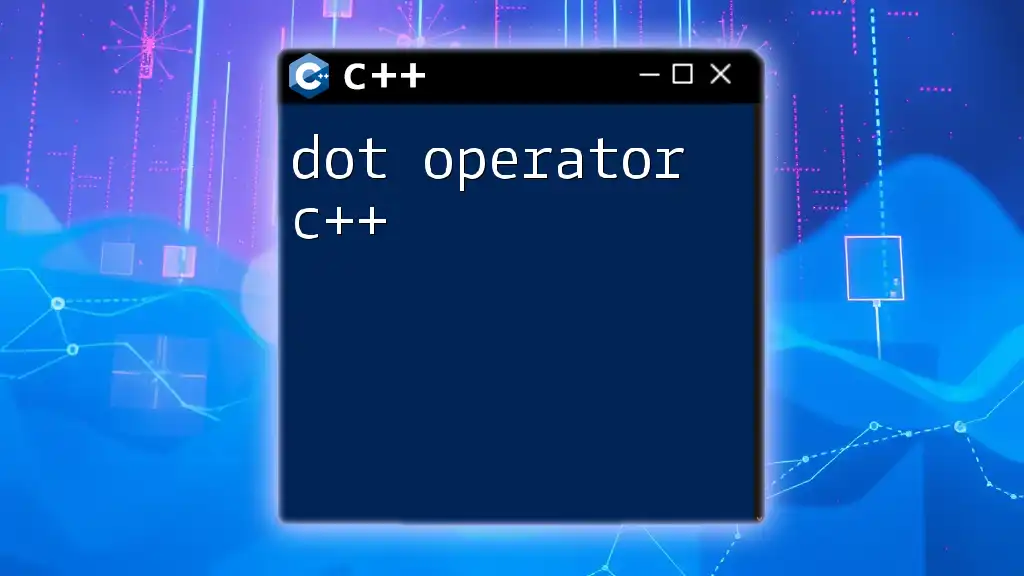
Best Practices for Using Compare Operators
Readability and Clarity
Prioritize readability in your comparisons. Using parentheses can clarify the order of evaluations and enhance the maintainability of your code:
if ((a > b) && (c < d)) {
// Code block demonstrating clear conditions
}
Clear comparisons contribute to a better understanding of your logic at a glance, especially in collaborative environments.
Optimizing Performance with Comparison
Another advanced practice involves optimizing performance by evaluating conditions that might short-circuit:
For instance, unnecessary calculations may be avoided if the first comparison fails, conserving resources and time.
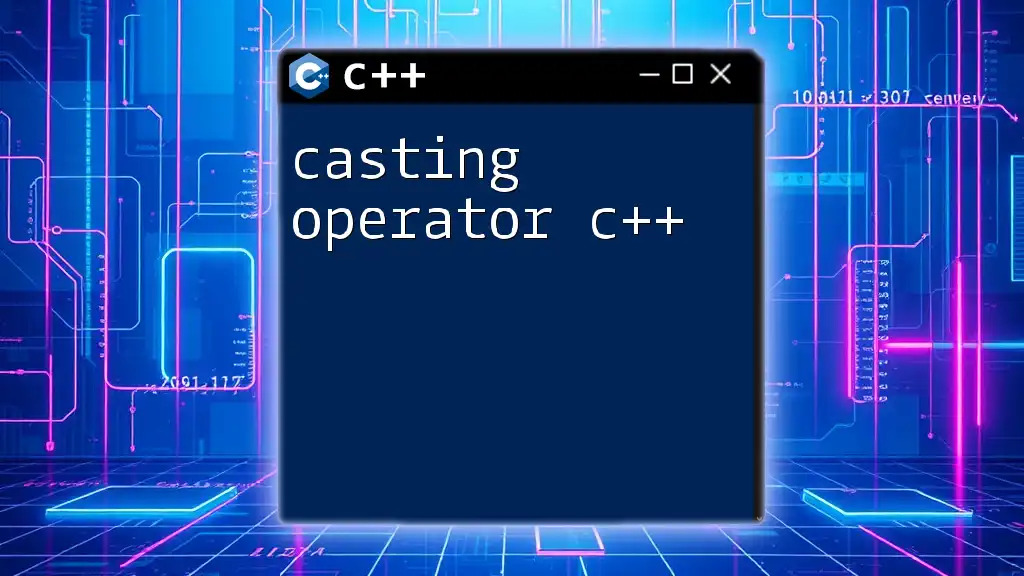
Conclusion
Understanding the compare operator in C++ is essential for any budding programmer. These operators are invaluable for data manipulation and control flow within your applications. Mastering their use enhances your programming skills and contributes to creating robust, efficient code. As you continue your journey in C++, incorporate these comparison techniques into your code and witness significant improvements in decision-making capabilities. For further learning, explore recommended books and online resources to deepen your understanding of C++.