In C++, you can compare characters using relational operators such as `==`, `!=`, `<`, `>`, `<=`, and `>=`, which allow for straightforward evaluations of their ASCII values.
Here's a code snippet demonstrating character comparison:
#include <iostream>
int main() {
char a = 'A';
char b = 'B';
if (a < b) {
std::cout << a << " is less than " << b << std::endl;
} else if (a > b) {
std::cout << a << " is greater than " << b << std::endl;
} else {
std::cout << a << " is equal to " << b << std::endl;
}
return 0;
}
Understanding Characters in C++
What is a Character in C++?
In C++, the `char` type represents a single character. It's an integral data type that stores a character's value, which is typically mapped to an ASCII or Unicode value. The `char` data type occupies one byte (8 bits) of memory, making it efficient for representing single characters. Understanding this basic data type is crucial for effective programming, particularly when dealing with text processing.
Character Representation
Characters can be represented using the ASCII (American Standard Code for Information Interchange), which assigns numeric values to characters. For instance, 'A' has an ASCII value of 65, and 'a' is 97. It's important to note that there's a fundamental difference between characters and strings; a string is essentially an array of characters, often terminated by a null character (`'\0'`).
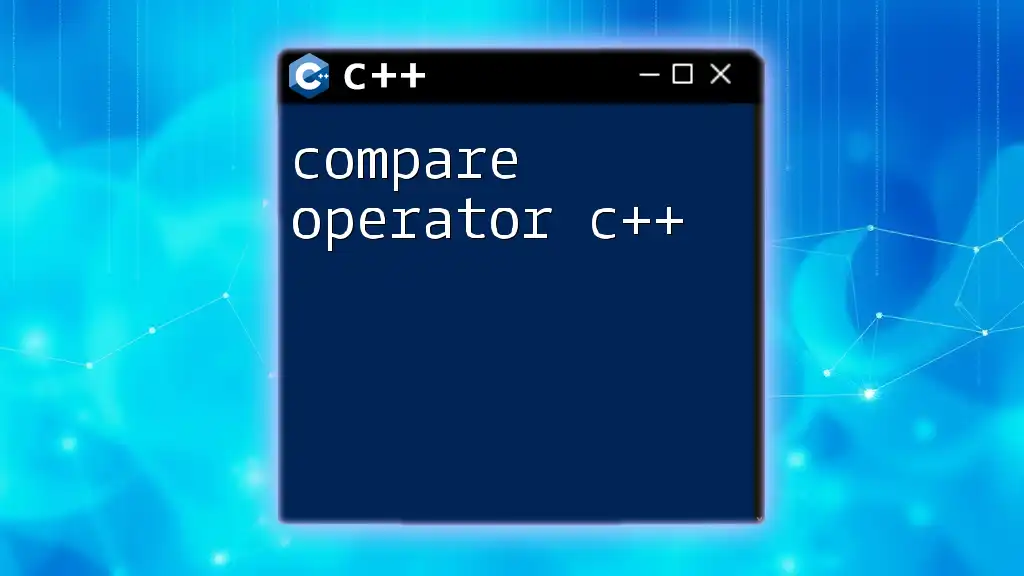
Basics of Comparing Characters in C++
Why Compare Characters?
Comparing characters is a critical part of many programming tasks. For instance, you might need to check user input, sort characters, or perform validations. By mastering character comparisons, you can enhance the functionality of your applications while ensuring the data integrity you're working with.
How to Perform Character Comparison in C++
C++ provides several operators for character comparison that allow you to compare characters effectively. The equality operator (`==`) checks if two characters are the same, while relational operators (`<`, `>`, `<=`, `>=`, `!=`) let you compare characters based on their ASCII values.
Code Snippet: Basic Character Comparison
Here’s a simple example demonstrating the basic characters comparison:
#include <iostream>
int main() {
char charA = 'A';
char charB = 'B';
if (charA == charB) {
std::cout << "Characters are equal." << std::endl;
} else {
std::cout << "Characters are not equal." << std::endl;
}
return 0;
}
In this example, we compare two characters using the equality operator. The output clearly indicates whether the characters are the same.
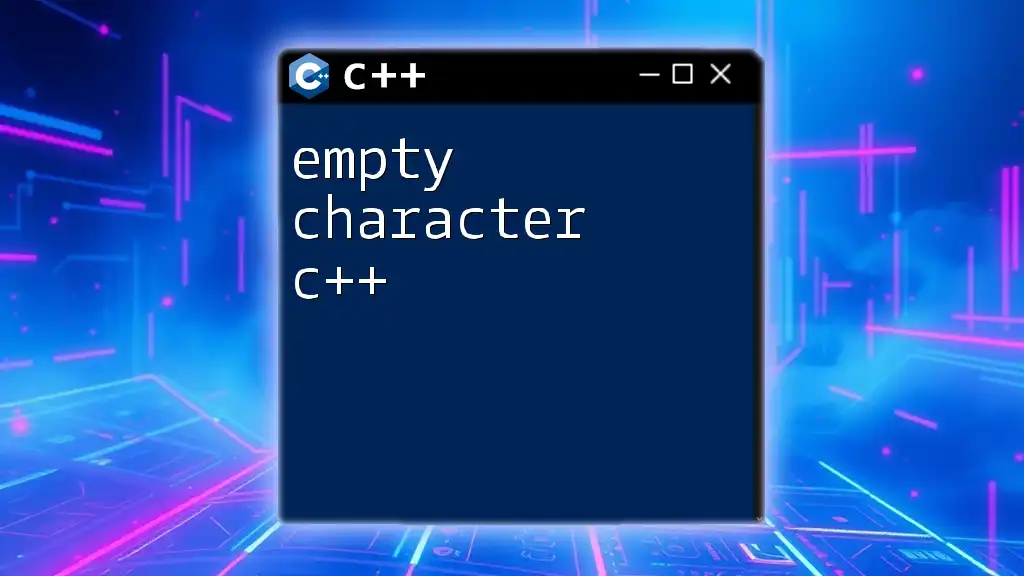
Advanced Character Comparison Techniques in C++
Comparing Characters Using Standard Library Functions
The C++ Standard Library offers functions for manipulating characters through the `<cctype>` header. Functions such as `toupper()` and `tolower()` can be particularly useful when you want to perform case-insensitive comparisons.
Code Snippet: Using Library Functions for Comparison
Here’s how to implement case-insensitive comparison:
#include <iostream>
#include <cctype>
int main() {
char charA = 'a';
char charB = 'A';
if (tolower(charA) == tolower(charB)) {
std::cout << "Characters are equal (case insensitive)." << std::endl;
} else {
std::cout << "Characters are not equal." << std::endl;
}
return 0;
}
This code snippet demonstrates the use of the `tolower()` function to normalize the case of both characters before comparison. This is crucial when ensuring that comparisons are not affected by character cases.
Comparing Characters with String Functions
In addition to comparing individual characters, you may often find yourself comparing characters within a string context. C++ provides powerful string manipulation functions that can assist in this regard.
Code Snippet: Comparing Characters in a String
Here’s an example of comparing a character with the first character of a string:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
char charA = 'H';
if (str[0] == charA) {
std::cout << "First character matches!" << std::endl;
} else {
std::cout << "No match found." << std::endl;
}
return 0;
}
In this example, we compare the first character of a given string with a specified character. By accessing the first element of the string using indexing, we can effectively compare the two.
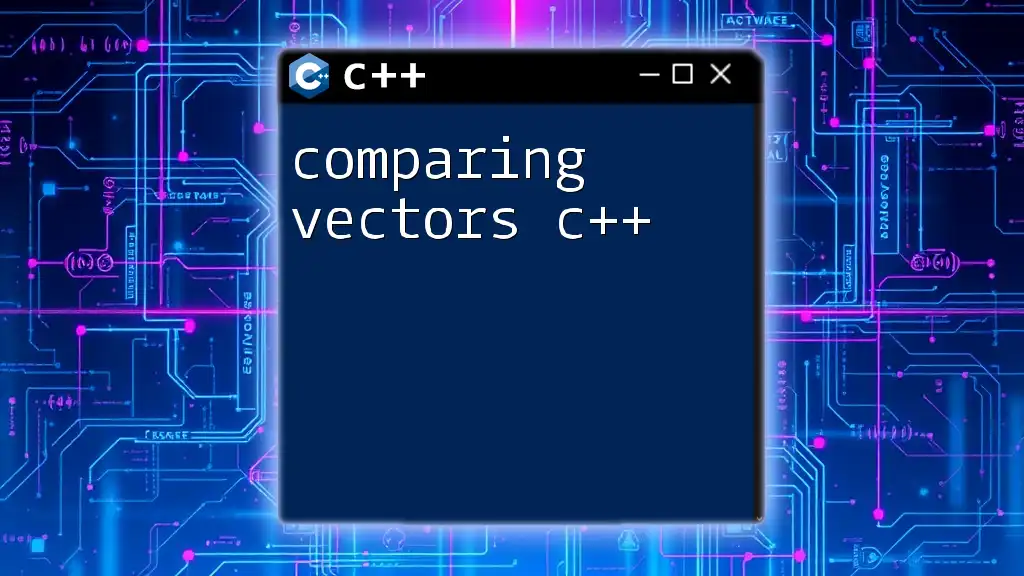
Tips and Best Practices for Comparing Characters in C++
Common Pitfalls in Character Comparison
When comparing characters, there are several commonly encountered pitfalls. One of the most prevalent is case sensitivity. It's essential to remain aware that 'A' and 'a' are treated as different characters—this can lead to confusing results if not handled properly.
Additionally, be mindful of character encoding issues. ASCII is usually the default, but if you're working with internationalization or specialized character sets, discrepancies may arise.
Optimizing Character Comparison
To optimize character comparison in your applications, consider which comparison method will best suit your needs. For instance, if you're frequently performing case-insensitive comparisons, it might make sense to use `tolower()` early on and standardize character cases throughout your codebase.
Furthermore, in scenarios involving large datasets, consider performance implications and select the most efficient approach for your specific use-case.
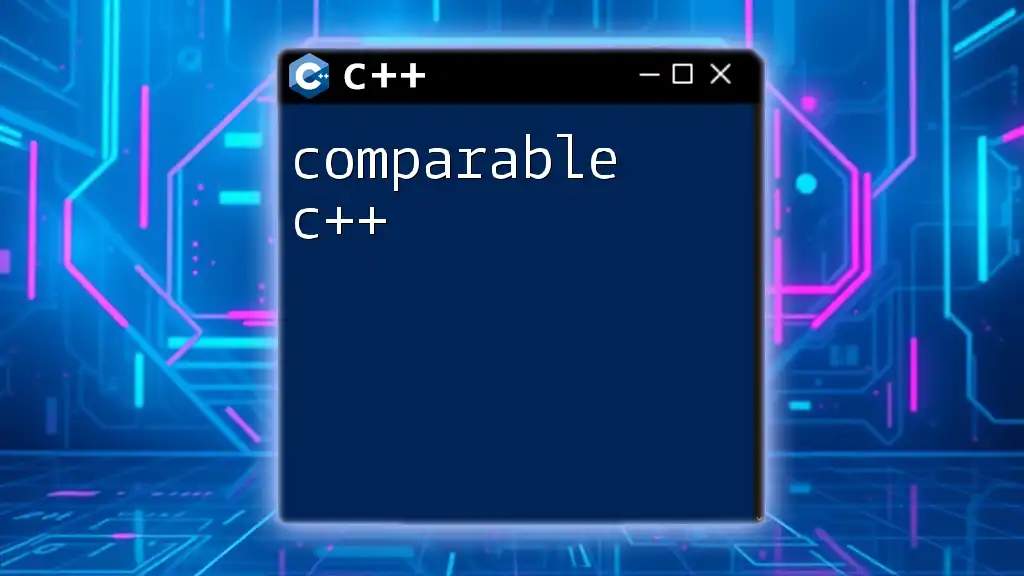
Conclusion
Mastering how to compare characters in C++ is a foundational skill that significantly enhances your programming abilities. By using various operators, library functions, and being mindful of common pitfalls, you will be better equipped to handle character comparison efficiently. It's crucial to practice these techniques so you can confidently implement character comparisons in your future coding projects.
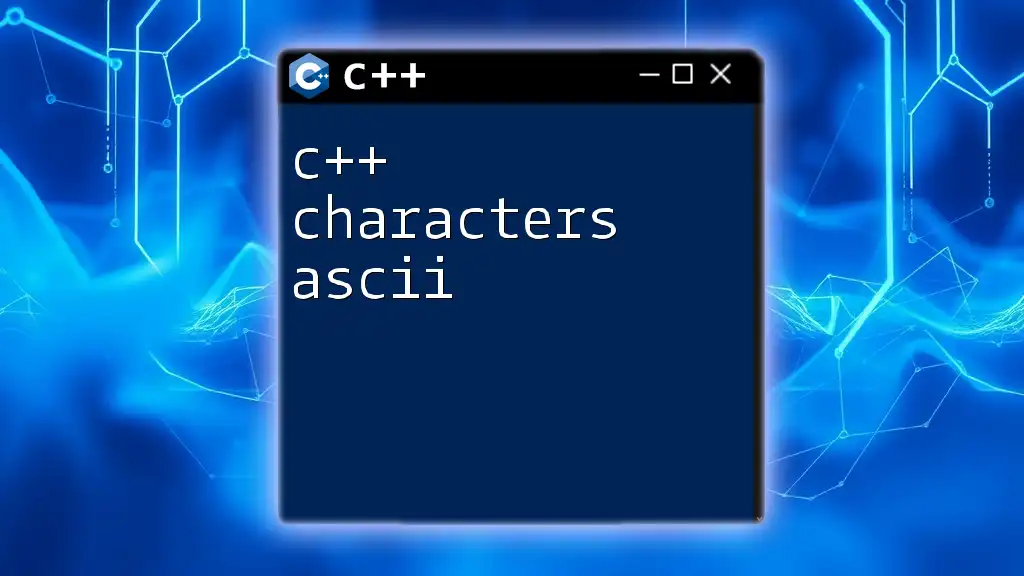
Additional Resources
For further exploration, consider looking into the official C++ documentation for in-depth explanations of character and string handling. There are numerous books and tutorials available that can deepen your understanding and provide additional practice opportunities.