In C++, the newline character can be represented using `'\n'`, which is commonly used to move the cursor to the next line in the output.
#include <iostream>
int main() {
std::cout << "Hello, World!" << '\n' << "Welcome to C++ commands." << std::endl;
return 0;
}
Understanding the Concept of Newline Character
The newline character in C++ is a vital component in text processing and output formatting. It serves as a marker to indicate the end of a line and the beginning of a new one in text files and console output. In the C++ language, the newline character is represented by `'\n'`, which holds an ASCII value of 10.
Using newline characters effectively can greatly enhance the readability of your output, making it easier for users to understand information presented in multiple lines.
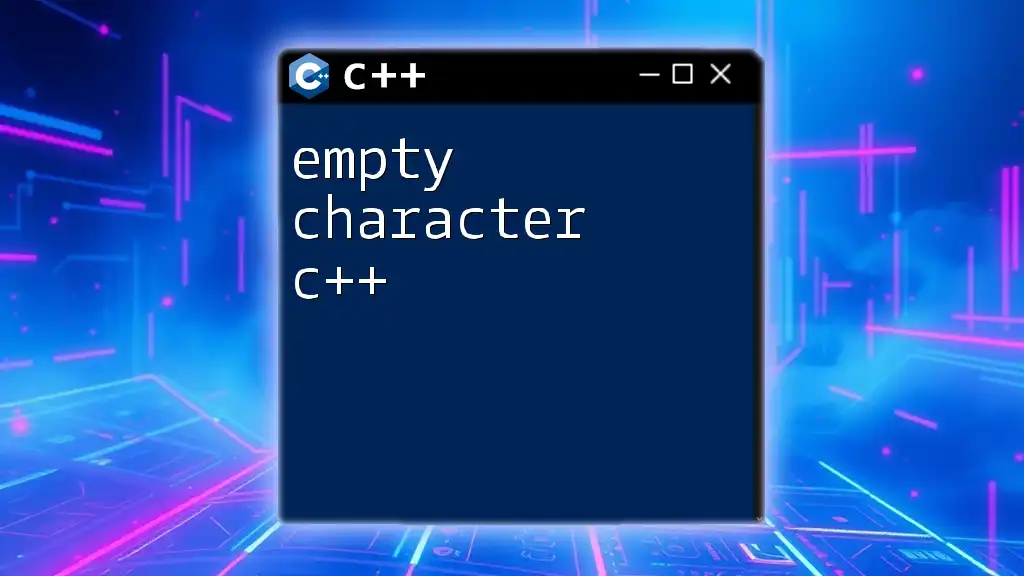
New Line in C++: Basic Usage
What is a New Line in C++?
A new line in C++ represents the end of one line of text and the start of another. While it may seem simple, understanding how to use new lines can significantly impact the way data is displayed.
In C++, two common methods for creating new lines in output are through the use of `std::endl` and the newline character `'\n'`.
The C++ endl Command
The `std::endl` command is a manipulator primarily used to insert a newline character into the output stream and flush the buffer. Flushing the buffer can be useful in some scenarios, such as when you need to ensure that output is displayed immediately.
Example demonstrating the usage of `std::endl`:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl; // This moves the cursor to the next line
return 0;
}
In this example, the output will display "Hello World!" followed by moving the cursor to the next line, thanks to `std::endl`.
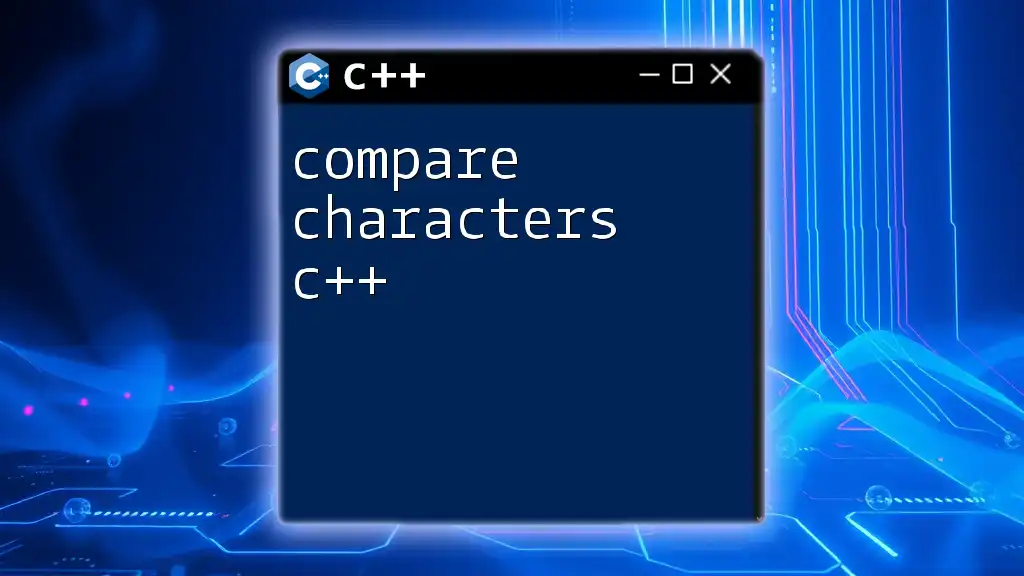
The C++ New Line Character
What is the New Line Character in C++?
The newline character in C++ is coded as `'\n'`. Unlike `std::endl`, using `'\n'` does not flush the output buffer; it merely adds a line break. This subtle but significant difference can affect performance in situations where output is generated rapidly.
Example of Using New Line Character
When you want to create multiple lines of output without the additional overhead of flushing the stream, you can use `'\n'`. Here’s a demonstration:
#include <iostream>
using namespace std;
int main() {
cout << "Line 1\nLine 2\nLine 3"; // Outputs three lines
return 0;
}
The output from this code will be:
Line 1
Line 2
Line 3
Each `\n` instructs the console to move to the next line, making the output clearer and more organized.
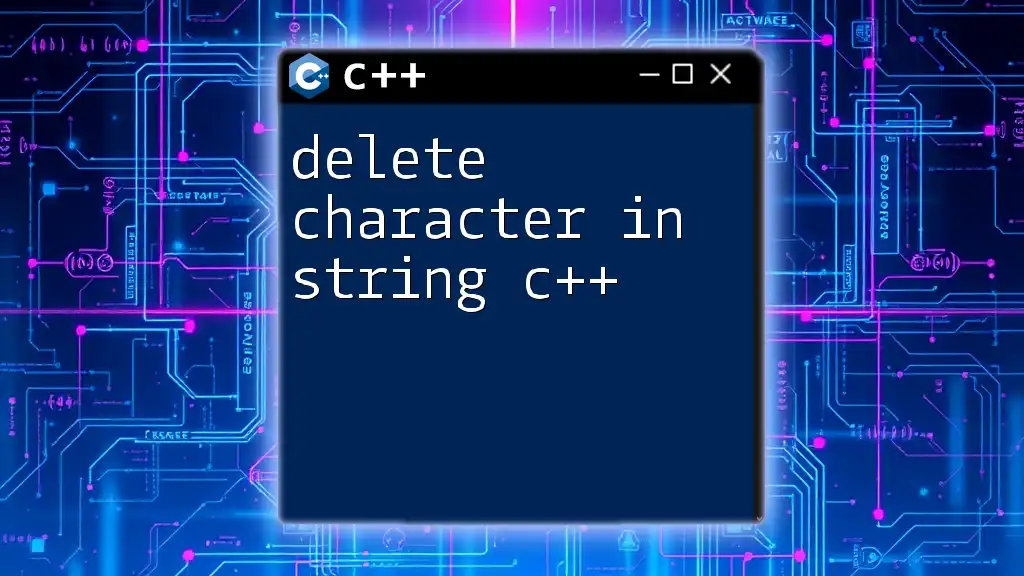
Differences Between New Line in C++ and Other Languages
Comparing Syntax: New Line in C++ vs. Java/Python
While C++ uses `'\n'` and `std::endl`, other programming languages implement newline characters in slightly different ways. For instance:
- Java uses `System.out.println()` to print on a new line and `"\n"` within strings.
- Python uses the `print()` function with automatic new line placement or `"\n"` within strings.
Benefits of Using New Line in C++
Using new line characters in C++ enhances both clarity and performance. A well-structured output is easier to read, and it prevents confusion that could arise from crowded lines. Moreover, understanding the differences between `'\n'` and `std::endl` allows developers to optimize output operations and use resources more effectively.
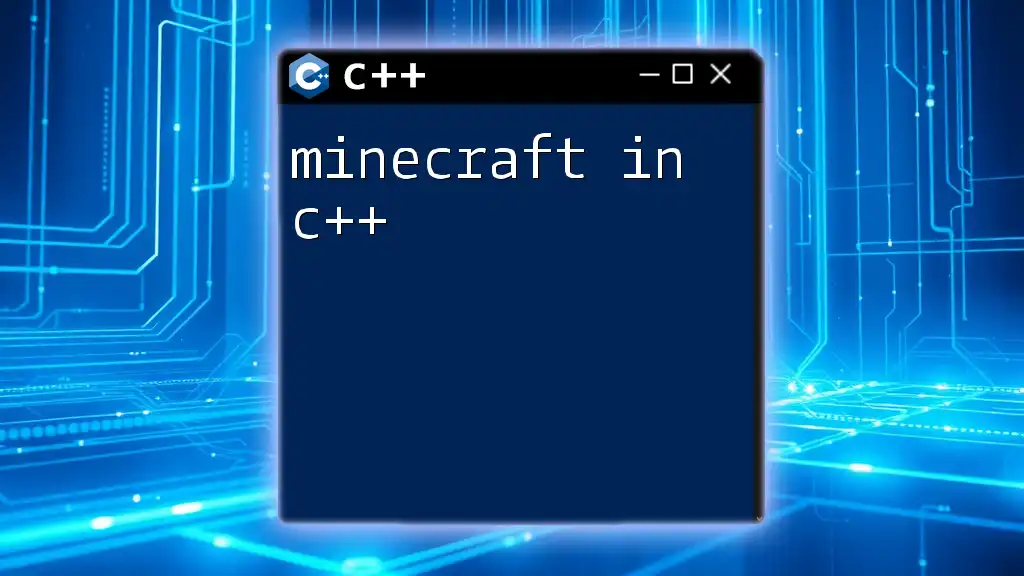
Advanced Usage of C++ New Line Character
Combining New Lines with Other Output
Newline characters can also be combined with other output for formatting purposes. Here’s how you can create spaces between outputs using multiple newline characters:
#include <iostream>
using namespace std;
int main() {
cout << "Header\n\nThis is with two new lines.\nEnd." << endl;
return 0;
}
This will output:
Header
This is with two new lines.
End.
In this example, two `\n` are included between "Header" and "This is with two new lines." This technique is useful when formatting output to improve readability.
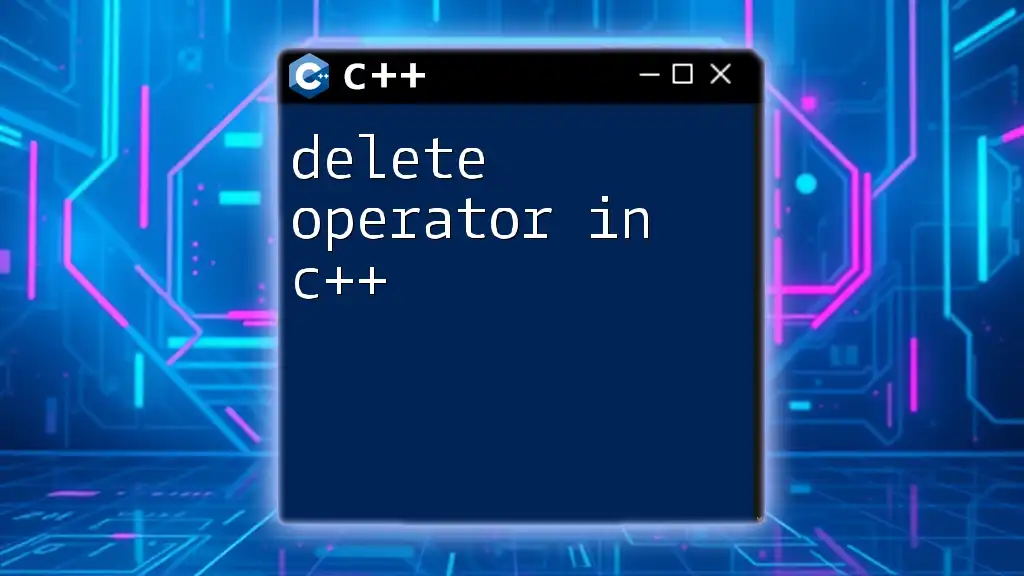
Common Mistakes with New Line Characters
One common error is misunderstanding the use of `endl` and the newline character `'\n'`. Some programmers may mistakenly think they are interchangeable, but they work differently. The following snippet demonstrates a common mistake:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl; // correct
cout << "Hello World!" \n; // incorrect
return 0;
}
In the incorrect example, the attempt to use `'\n'` without proper string concatenation will lead to a compilation error. Always ensure your commands are syntactically correct.
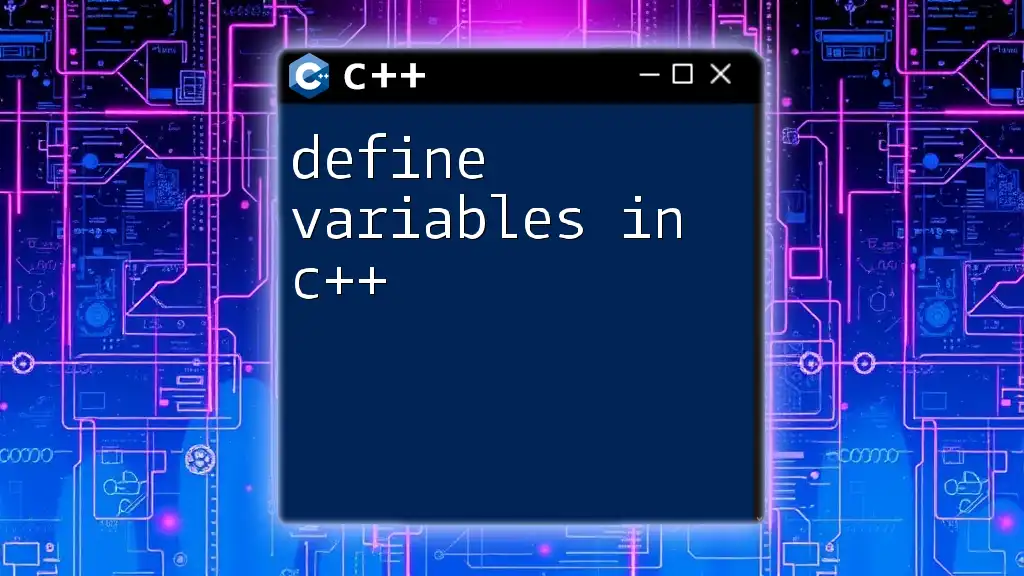
Best Practices for Using New Line in C++
To maximize efficiency and readability when using newline characters in C++, consider the following best practices:
-
Prefer `'\n'` for performance: If flushing the buffer is not critical, use `'\n'` to avoid potential performance hits from flushing each line.
-
Maintain consistency: Use either `'\n'` or `std::endl` throughout your program to ensure uniformity and predictability in your output.
-
Comment on complex structures: If you're creating intricate console outputs, include comments to clarify your logic for future reference.
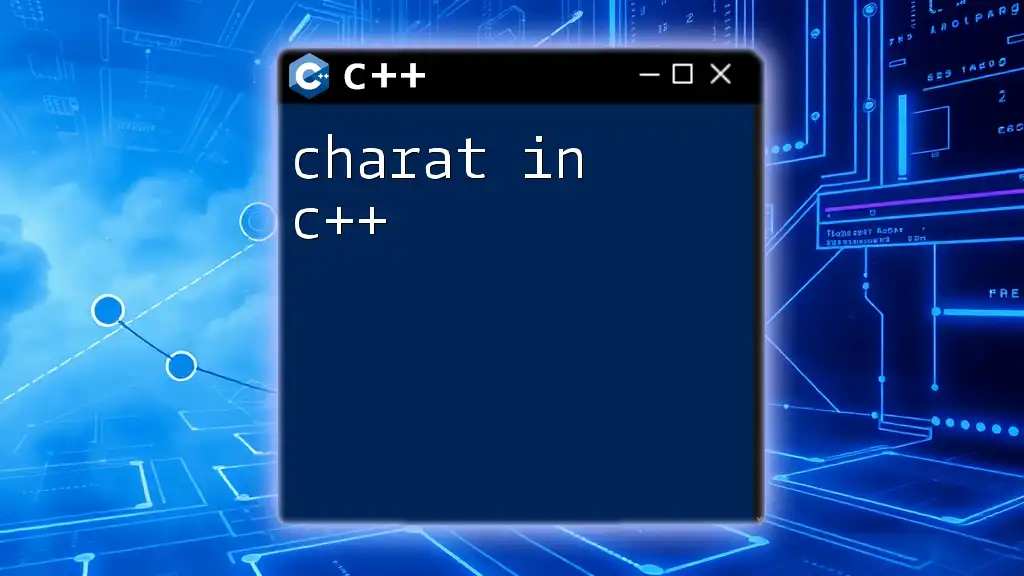
Conclusion
The newline character in C++ plays a crucial role in structuring output effectively. By mastering its application, you can create clear, concise, and easily understandable console outputs. Practice using newline characters in various applications will also aid your overall C++ programming skills.
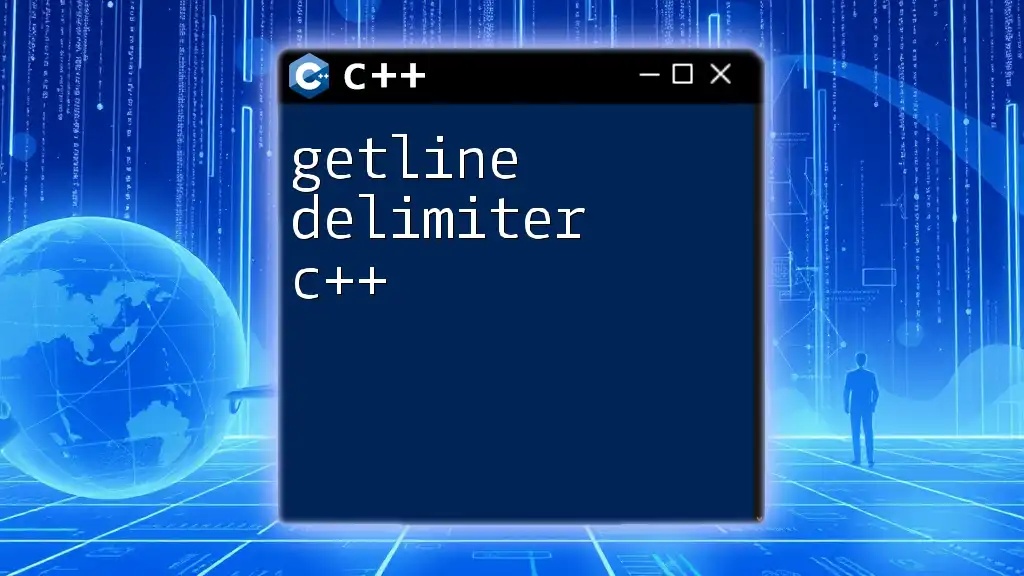
Additional Resources
For further learning, the following resources can be extremely beneficial:
- C++ Standard Library documentation for in-depth coverage of output manipulation.
- Recommended books on C++ programming and output formatting techniques.
- Online community forums for discussions and clarifications on specific queries related to C++.
Call to Action
If you found this guide helpful, subscribe for more quick tips in C++, or consider enrolling in our courses to further enhance your programming skills!