In C++, you define a variable by specifying its data type followed by the variable name, and you can optionally initialize it with a value.
int age = 25; // Defines an integer variable named 'age' and initializes it to 25
Understanding Variables in C++
What is a Variable?
A variable is essentially a storage location in a computer's memory that holds data that can change during program execution. In C++, variables allow programmers to manipulate data dynamically based on user input or program logic.
Importance of Variables in C++
Variables are fundamental in programming, especially in C++. They facilitate data storage, manipulation, and retrieval, making it possible to implement algorithms, manage input and output, and build complex applications efficiently. Understanding how to define variables in C++ is crucial for any programmer.
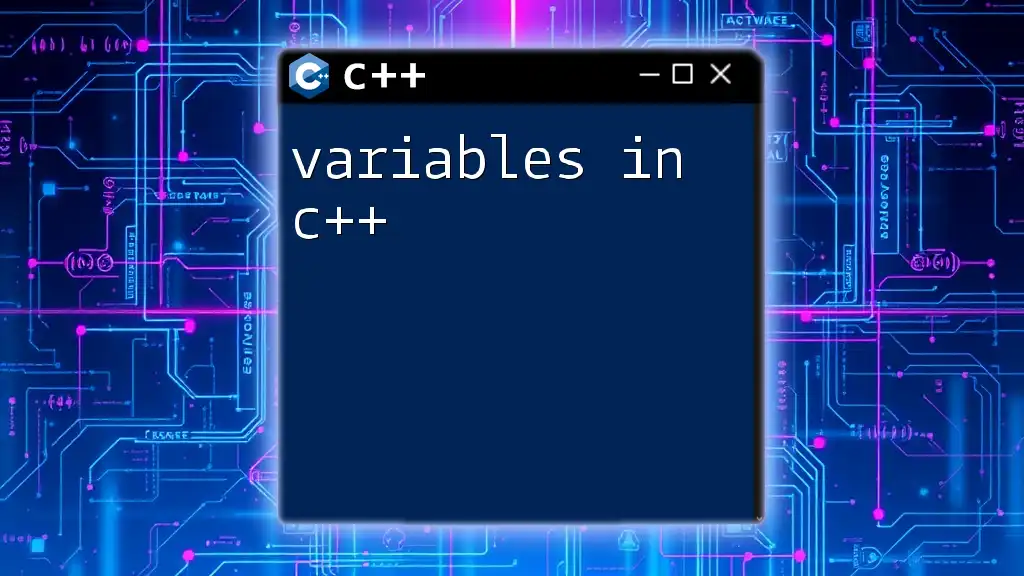
Understanding Data Types in C++
Basic Data Types
C++ supports several basic data types, each serving distinct purposes:
- `int`: Used for integer values.
- `float`: Represents single-precision floating-point numbers (decimals).
- `char`: Stores single characters.
- `double`: A data type for double-precision floating-point numbers.
Example:
int age = 25; // An integer variable to store age.
float salary = 50000.50; // A float variable for salary.
char initial = 'A'; // A char variable for initials.
double pi = 3.14159; // A double variable for representing pi.
Derived Data Types
Derived data types are built from fundamental types. This includes:
- Arrays: A collection of similar data types.
- Structures (`struct`): A user-defined data type that groups different data types together.
- Pointers: Variables that store the address of other variables.
User-Defined Data Types
C++ also allows you to create your own data types, such as:
- `struct`: Used to define a structure that can hold multiple types of related data.
- `enum`: Helps in defining variables that can be assigned to a set of predefined constants.
- `class`: Used in object-oriented programming to create complex data types with properties and methods.
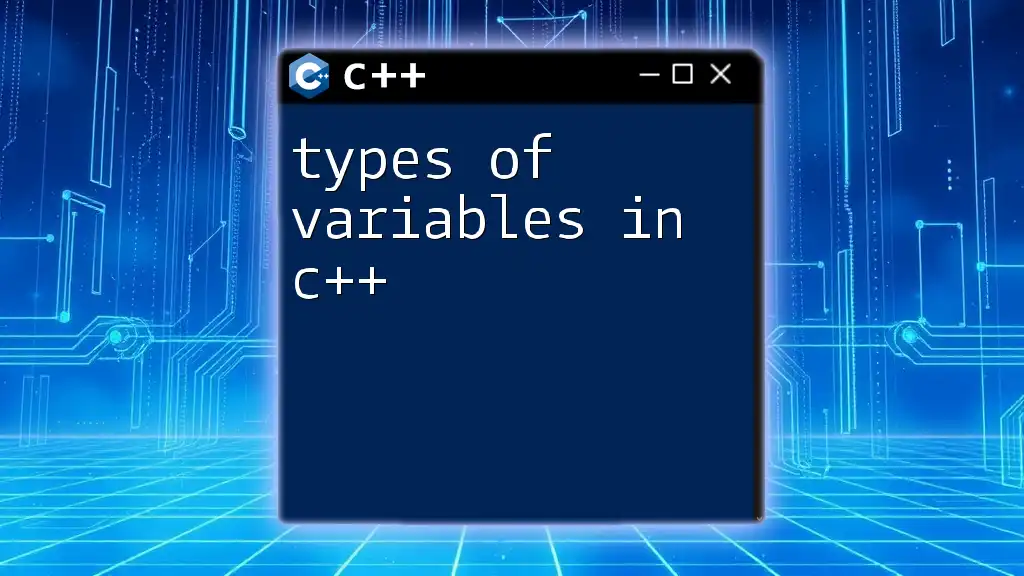
How to Define a Variable in C++
Syntax for Defining Variables
To define a variable in C++, you use a simple syntax:
data_type variable_name;
This statement declares a variable of `data_type` with the name `variable_name`.
Example:
int number; // Declares a variable named number of type int
Initializing Variables
Initialization involves assigning an initial value to a variable at the time of declaration. This is crucial because using uninitialized variables leads to unpredictable behavior.
Example:
int number = 10; // Declaration and initialization
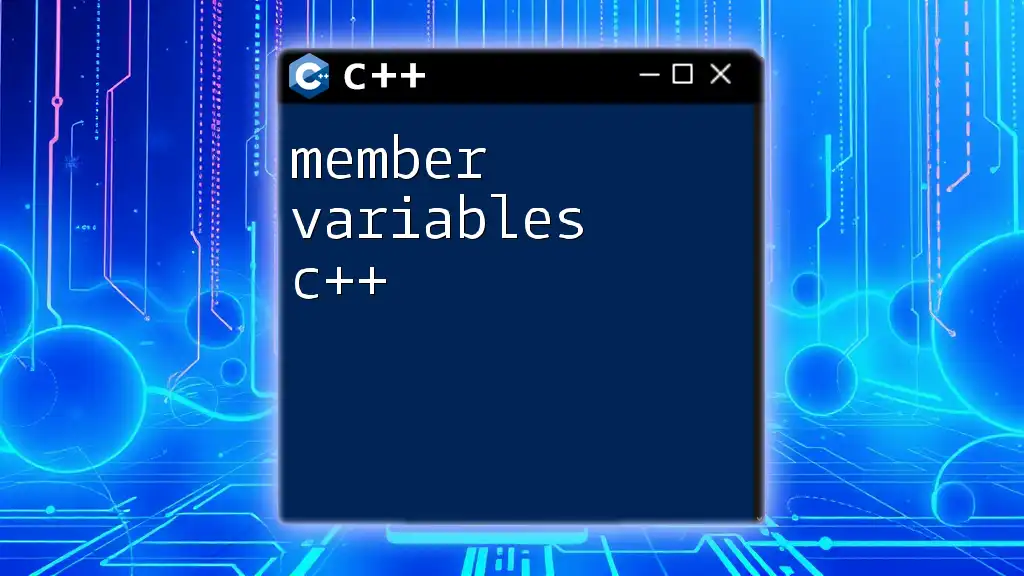
Different Ways to Declare Variables in C++
Single Declaration
You can declare a single variable by simply stating its type and name.
Multiple Declarations
C++ allows the declaration of multiple variables of the same type in a single line, enhancing code conciseness.
Example:
int a = 5, b = 10, c = 15; // Declares three int variables
Using the `auto` Keyword
The `auto` keyword lets C++ infer the data type of a variable automatically based on the initializer's value, streamlining variable declaration.
Example:
auto myNumber = 10; // Automatically deduces 'myNumber' as an int
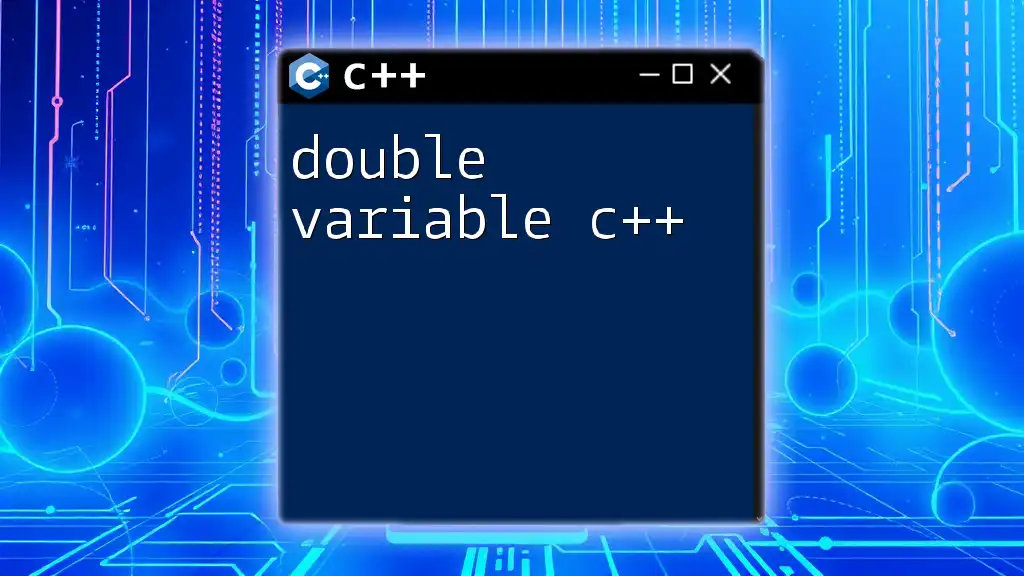
Kinds of Variables in C++
Local Variables
Local variables are defined within a function or block and can only be accessed within that scope.
Example:
void function() {
int localVar = 25; // Accessible only within this function
}
Global Variables
Global variables are defined outside of any function and can be accessed from any function in the program.
Example:
int globalVar = 100; // Accessible throughout the entire program
Static Variables
Static variables retain their values even after the scope in which they were declared has ended. This is particularly useful for maintaining state across function calls.
Example:
static int count = 0; // Preserves its value between function calls
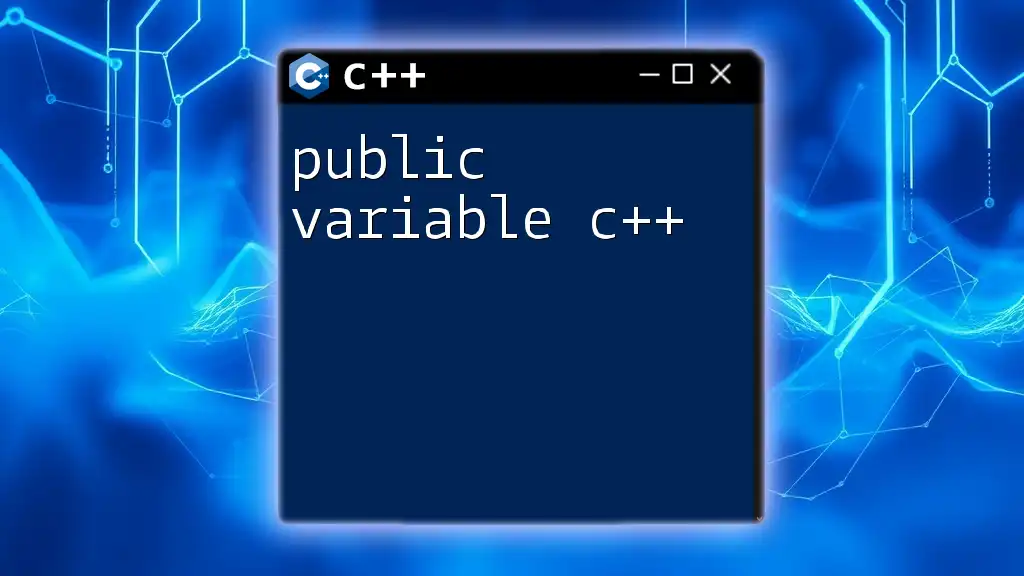
Best Practices for Defining Variables in C++
Meaningful Naming Conventions
Choose descriptive and meaningful names for variables, which enhances code readability and understanding. For instance, use `studentAge` instead of just `age`.
Scope Considerations
Always be mindful of the scope of your variables. Limiting the scope of variables to the smallest necessary block can reduce unintended side effects and improve maintainability.
Type Safety and Consistency
Use appropriate data types to maintain type safety. Mixing data types can lead to undesirable results or runtime errors. Being consistent with variable types enhances code reliability.
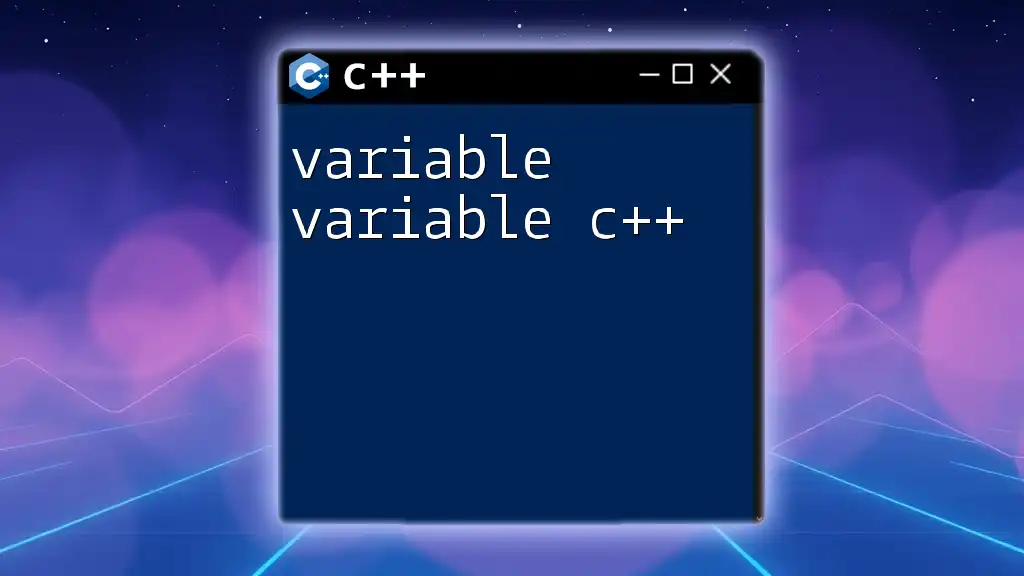
Common Errors and How to Avoid Them
Uninitialized Variables
Working with uninitialized variables can lead to unpredictable behavior, bugs, or crashes. Always ensure variables are initialized before use.
Example:
int x; // Uninitialized, may lead to undefined behavior
Conflicting Variable Names
Using the same variable name in different scopes can create confusion and lead to bugs. Ensure that variable names are unique in their respective scopes.
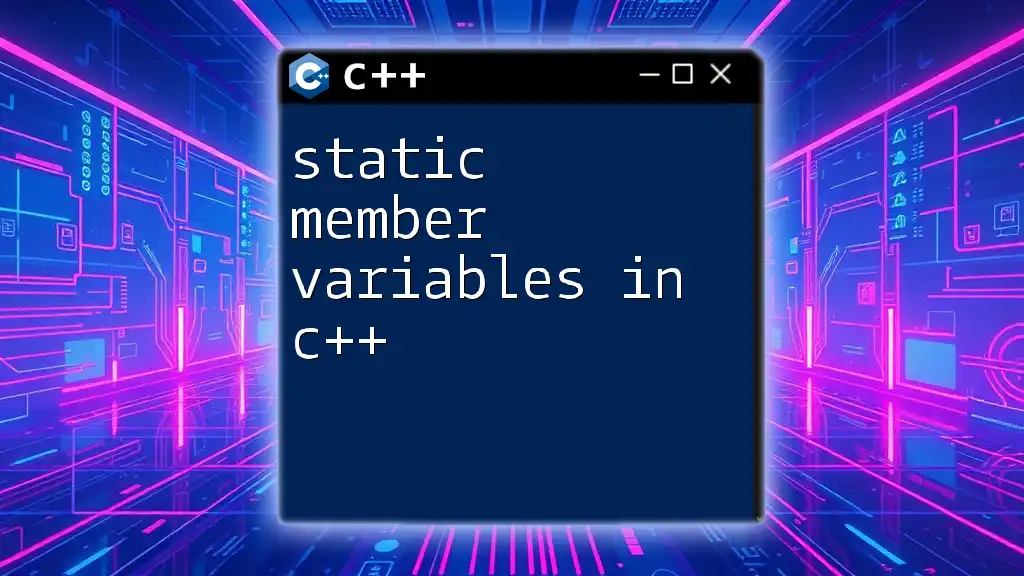
Conclusion
Understanding how to define variables in C++ is foundational to programming in this language. With clear definitions, appropriate data types, and consideration of scope and best practices, you can create efficient and readable code. Practice defining variables in C++ to reinforce your understanding and build your programming skills.