In C++, variables can be categorized into several types, including fundamental types like int, float, char, and user-defined types such as structs and classes.
Here's a code snippet demonstrating different types of variables in C++:
#include <iostream>
using namespace std;
int main() {
// Fundamental types
int integerVar = 10; // Integer
float floatVar = 5.5f; // Floating-point
char charVar = 'A'; // Character
bool boolVar = true; // Boolean
// User-defined type
struct Person {
string name;
int age;
};
Person personVar = {"Alice", 30}; // Person struct
// Output
cout << "Integer: " << integerVar << endl;
cout << "Float: " << floatVar << endl;
cout << "Char: " << charVar << endl;
cout << "Bool: " << boolVar << endl;
cout << "Person Name: " << personVar.name << ", Age: " << personVar.age << endl;
return 0;
}
What is a Variable?
A variable in programming refers to a storage location identified by a name that can hold a value. In the context of C++, variables are fundamental building blocks that allow programmers to manipulate data. They can be thought of as containers that store information for use during program execution.
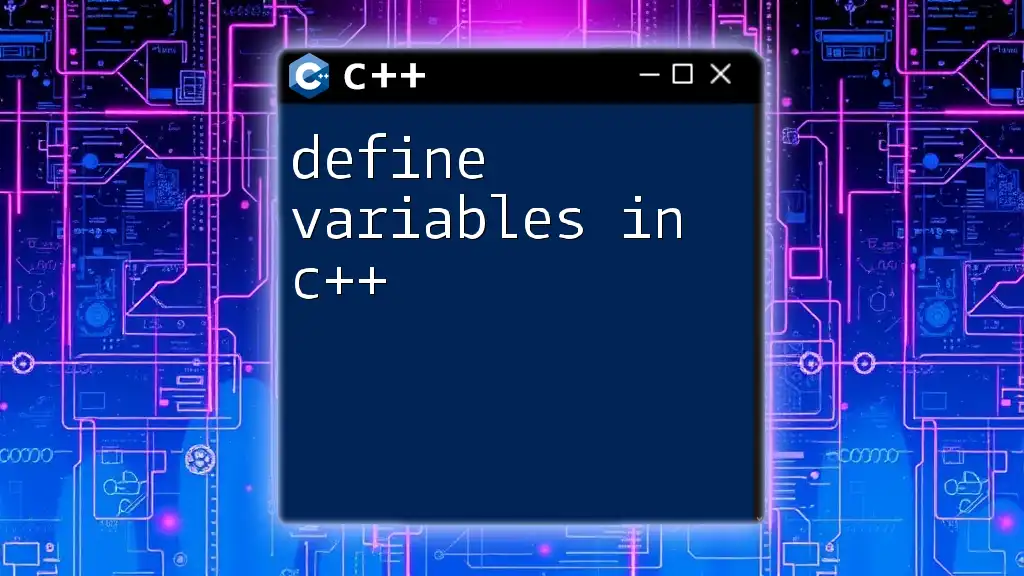
The Importance of Variable Types in C++
Why should you care about variable types? The variable type determines how much memory to allocate, what kind of values the variable can hold, and how operations can be performed on it. Understanding the different types of variables in C++ is crucial for efficient coding, enhancing performance, and ensuring type safety, thereby helping to prevent errors during development.
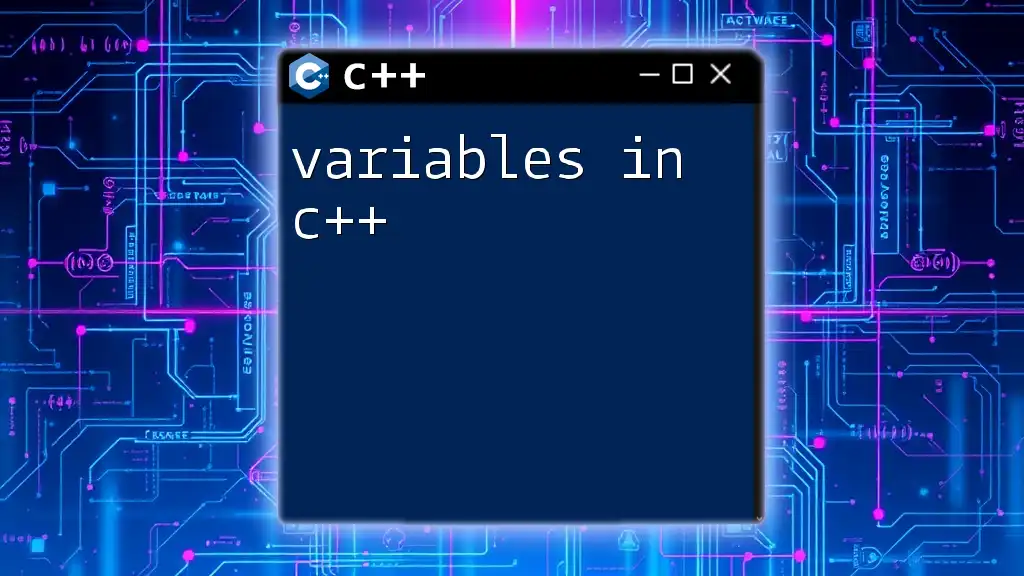
Basic Data Types in C++
Fundamental Types of C++ Variables
-
Integer Types In C++, integer types are used to store whole numbers. The common integer types include:
- `int`: A standard integer.
- `short`: A smaller integer type.
- `long`: A larger range of integer values.
- `unsigned`: A type that only holds positive values.
Here’s an example of how to use integer types:
int age = 30; unsigned int positiveNumber = 50;
-
Floating-Point Types Floating-point types are utilized for storing numbers that require decimal places. The primary floating-point types are:
- `float`: A single-precision floating-point number.
- `double`: A double-precision floating-point number for greater accuracy.
- `long double`: An extended precision type for handling very large or very small numbers.
Example usage:
float height = 5.9f; double precisionValue = 3.14159;
-
Character Type The character type in C++ is used to store single characters. Characters are represented using the `char` data type.
Example:
char initial = 'A';
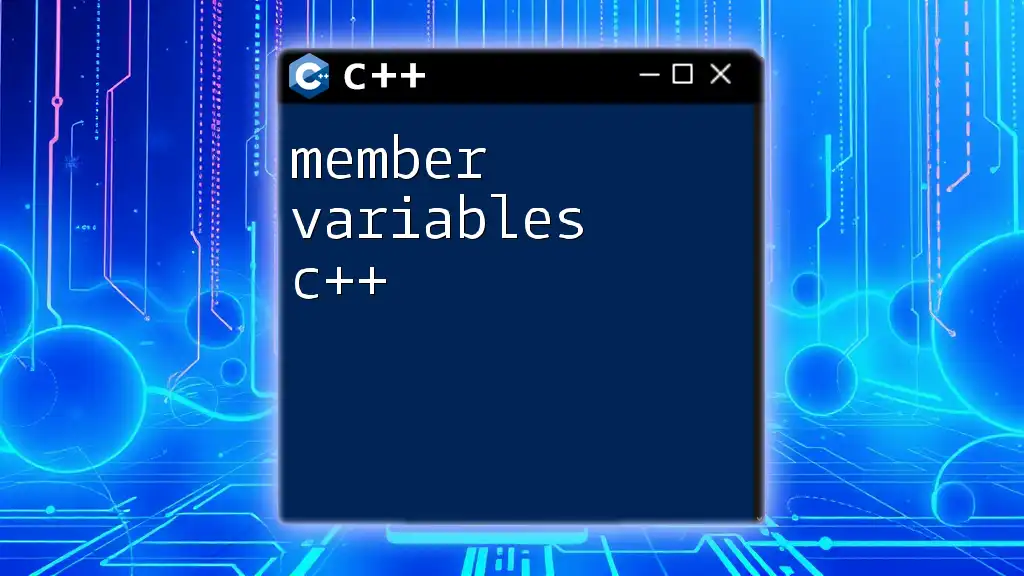
Derived Data Types
Understanding Kinds of Variables in C++
-
Arrays An array is a collection of elements of the same type, stored contiguously in memory. Arrays can be accessed using an index starting from zero.
Here’s how an array can be defined:
int numbers[5] = {1, 2, 3, 4, 5};
-
Pointers A pointer is a variable that stores the memory address of another variable. Pointers are powerful as they allow direct memory manipulation.
Example of a pointer:
int age = 30; int* p = &age; // p now holds the address of age
-
References A reference variable acts as an alias for another variable. Once a reference is initialized to a variable, you cannot change that reference to refer to another variable.
Code snippet showing reference:
int age = 30; int& ref = age; // ref now refers to the same memory location as age
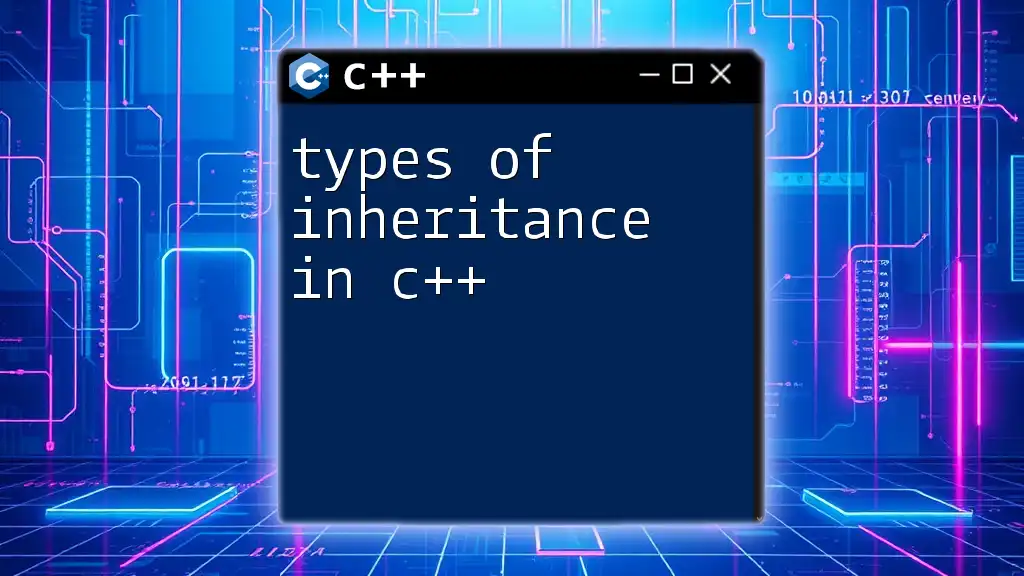
User-Defined Data Types
Exploring Custom Variable Types
-
Structures (`struct`) Structures allow you to group different data types together. This is helpful to represent a complex data type.
Example of a structure:
struct Person { string name; int age; };
-
Unions (`union`) A union can store different data types but only one at a time. This makes unions memory efficient since they only allocate enough memory to hold the largest member.
Union example:
union Data { int intValue; float floatValue; };
-
Enumerations (`enum`) Enumerations provide a way to define a variable that can hold a set of predefined constants. This improves code readability.
Example of an enumeration:
enum Color { Red, Green, Blue };
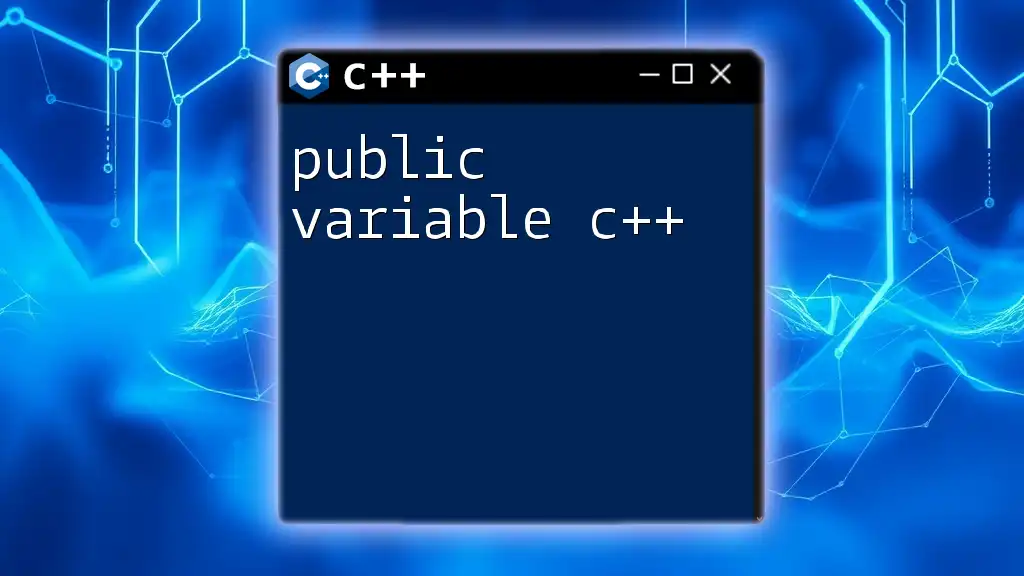
The Role of Type Casting
Type casting is the method of converting one data type to another. In C++, type casting can be either implicit (automatic conversion) or explicit (manual conversion).
An example of explicit type casting is:
double pi = 3.14;
int truncatedPi = (int)pi; // Explicit casting to convert double to int
Understanding type casting is important, as it can prevent potential data loss or errors during operations.
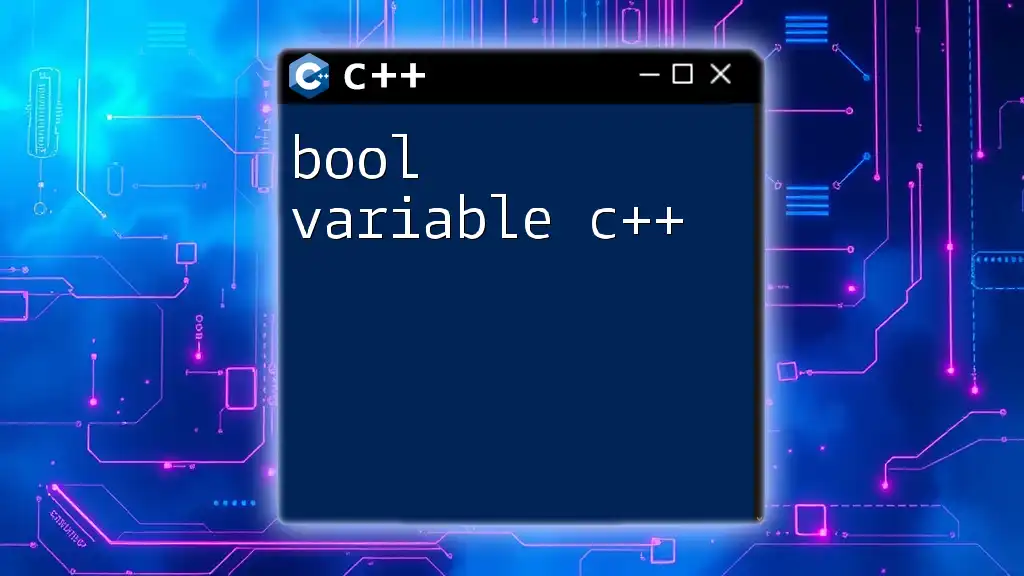
Best Practices for Using Variable Types
When dealing with variable types in C++, consider the following best practices:
-
Choosing Appropriate Types: Select the most suitable type based on the data range you wish to accommodate. For instance, if you only need to store positive values, prefer using `unsigned` types.
-
Descriptive Naming Conventions: Variable names should be clear and meaningful, describing the purpose of the variable. This improves code readability and maintainability.
-
Avoiding Type Mismatches: Be cautious of operations between different types that may cause unintended behavior or data loss. Using the right type and appropriate casting ensures that operations yield expected results.
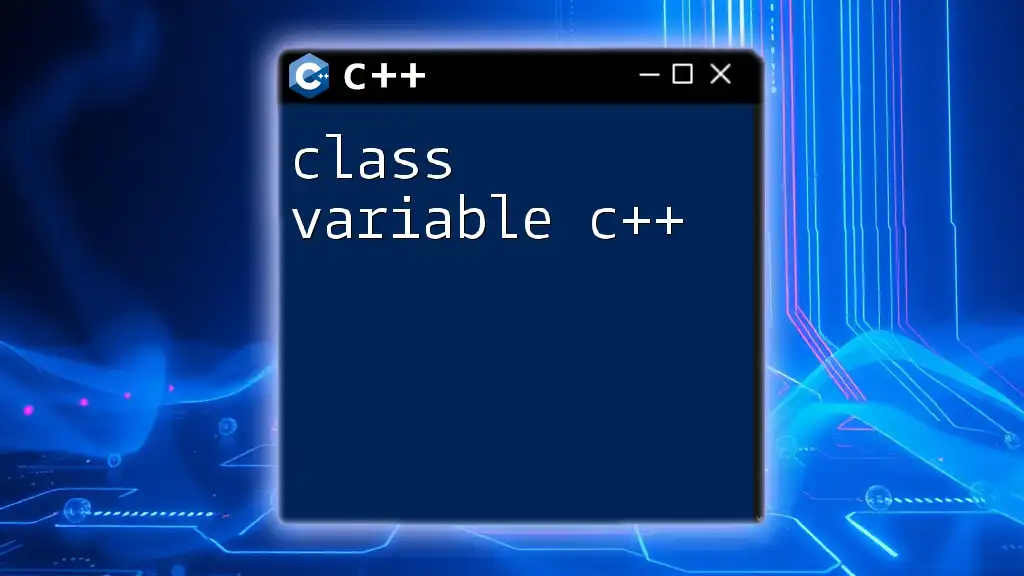
Conclusion
The understanding of types of variables in C++ opens a pathway to writing efficient, error-free code that performs well in various scenarios. By harnessing the power of various data types, programmers can write more effective and readable C++ code that is easier to manage and debug. Continue to explore C++ programming concepts and practice handling variables to build a solid foundation in your coding journey.