In C++, variables are used to store data that can be changed during program execution, allowing developers to perform operations on that data efficiently.
Here's a simple example of declaring and initializing variables in C++:
int main() {
int age = 25; // Integer variable
double height = 5.9; // Double variable
char initial = 'A'; // Character variable
return 0;
}
What Are Variables in C++?
In C++, variables are fundamental constructs that serve as storage locations in memory, associated with a name. They allow programmers to store and manipulate data throughout the execution of a program. Understanding variables in C++ is crucial for writing efficient and readable code, enabling you to hold values that your program can reference and alter.
Why Use Variables?
Variables facilitate several key aspects of programming:
- Storing Data: They hold information that can be processed and manipulated.
- Manipulating Data: Variables allow programmers to change their values and perform calculations or comparisons.
- Enhancing Code Readability: Well-named variables make code easier to read and understand, helping others (or even your future self) grasp the logic without extensive comments.

C++ Define Variable: Syntax and Basic Concepts
C++ Declaring Variables
Declaring a variable involves specifying its type and its name. The basic syntax is straightforward:
type variableName;
For instance:
int age;
In the above example, `int` specifies that `age` can store integer values.
C++ Defining Variables
While declaring a variable tells the compiler to reserve space in memory, defining it assigns a value to that space. Understanding this distinction is vital. Here’s how you can both declare and define a variable:
int age = 30; // Declaration and definition
Here, `age` is declared as an integer and initialized with the value `30` in one step.
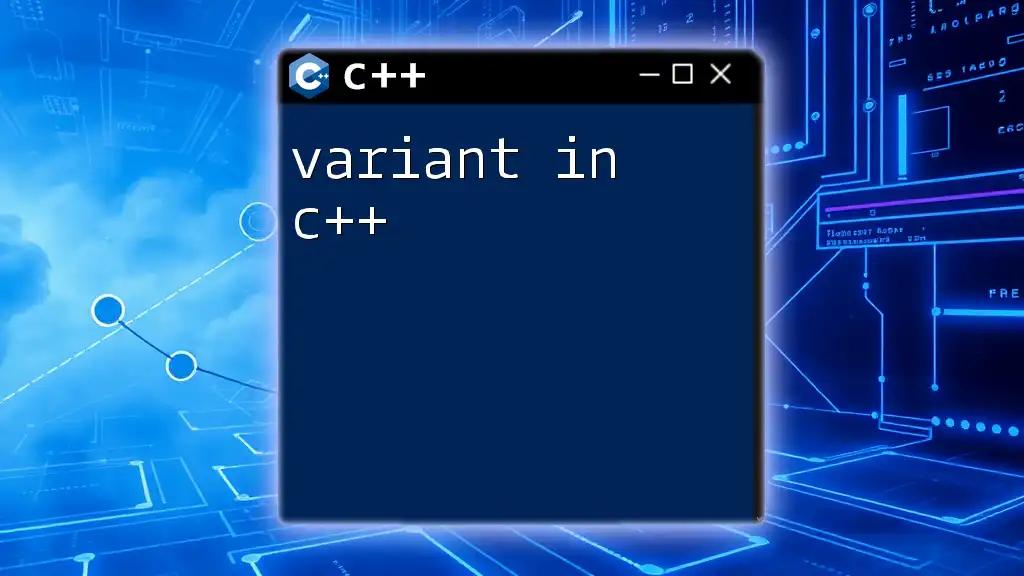
How to Define Variables in C++
Variable Types in C++
C++ supports various types of variables that cater to different requirements. Here are some of the most commonly used data types:
- Primitive Data Types:
- `int` (integer)
- `float` (floating point)
- `char` (character)
- `double` (double-precision floating point)
Example usage:
int number = 10;
float pi = 3.14;
char letter = 'A';
- Derived Data Types:
- Arrays, pointers, and functions also qualify as variable types, allowing for more complex data management.
Variable Initializers
When you assign a value directly at the time of variable declaration, it is termed initialization. This practice not only clarifies what value a variable holds but also helps prevent garbage values:
int score = 100; // Initialization at declaration
Proper initialization is a critical step in managing variables effectively.

C++ Variables Scope and Lifetime
Understanding Variable Scope
The scope of a variable refers to the region within the code where the variable is accessible. In C++, variables can be classified into global and local scopes:
- Local Variables: Defined within a function or block, these variables are only accessible within that context. For instance:
void function() {
int localVar = 5; // Local to the function
}
- Global Variables: Declared outside any function, these can be accessed from any function within the same file.
Variable Lifetime
The lifetime of a variable spans the duration of its existence in memory. Local variables are created when a block is entered and destroyed when it’s exited. In contrast, global variables exist throughout the program's execution. Understanding this can significantly impact memory management and resource optimization.

Variable Naming Conventions in C++
Rules for Naming Variables
Choosing appropriate variable names is essential for code readability. Here are the rules you must follow:
- Variable names cannot begin with a digit.
- They must not include spaces or special characters (except for underscores).
- Case sensitivity matters (e.g., `myVariable` and `MyVariable` are different).
Best Practices For Naming Variables
To improve code clarity, adhere to these best practices:
- Use camelCase or snake_case conventions to differentiate names (e.g., `myVariable` or `my_variable`).
- Ensure names convey their purpose, such as `totalScore` or `userAge`, rather than vague terms like `x`.

Common Errors When Working with Variables in C++
Even seasoned programmers can make mistakes when managing variables. Here are some common pitfalls:
- Starting Variable Names with Digits:
int 1stNumber; // Incorrect: Starts with a digit
- Using Uninitialized Variables: Accessing a variable without initialization can lead to unpredictable behavior.
int uninitializedVar; // Contains a garbage value if used
To avoid these mistakes, always initialize your variables, and follow naming conventions.

Conclusion
Understanding variables in C++ is a foundational step for any programmer. They are essential for storing, manipulating, and managing data effectively within programs. By mastering how to declare, define, and appropriately name variables, you enhance both your coding skills and the readability of your code.
Feel free to dive deeper into the methods for working with variables, and remember that practice is your best teacher in grasping these concepts! Happy coding!