In C++, a variable in a class is a member variable defined within the class, which can hold data pertaining to the objects created from that class. Here's an example:
class MyClass {
public:
int myVariable; // This is a member variable
};
Understanding Variables in Classes
What Are Class Variables?
In C++, class variables, often referred to as member variables, are attributes that define the state or properties of an object. There are two primary types: instance variables and static variables. Understanding the distinction between these two is critical for effective class design.
Scope of Class Variables:
Class variables exist within the context of the class itself. Unlike local variables, which only exist within a function or block, class variables persist for the lifetime of an object. Instance variables are recreated whenever a new object of the class is instantiated, while static variables are shared among all instances of a class.
Types of Variables in a Class
Instance Variables
Instance variables are the attributes unique to each object created from the class. They hold values that define the individual characteristics of the objects.
Code Example:
class Car {
public:
std::string make;
std::string model;
int year;
Car(std::string mke, std::string mdl, int yr) {
make = mke;
model = mdl;
year = yr;
}
};
In this code snippet, the `Car` class has three instance variables: `make`, `model`, and `year`. Each object of the `Car` class can have different values for these attributes, allowing for the representation of different car models.
Static Variables
Static variables differ from instance variables in that they are shared among all instances of a class. They maintain a single value that is shared across all objects.
Code Example:
class Student {
public:
static int studentCount; // static variable
Student() {
studentCount++;
}
};
int Student::studentCount = 0; // initializing static variable
Here, `studentCount` is a static variable that keeps track of the number of `Student` objects created. Regardless of how many `Student` instances exist, there is only one `studentCount` variable that accumulates the count.
Const Variables
Const variables are used to define values that should not change throughout the lifetime of an object. They are crucial for maintaining values that do not require modification.
Code Example:
class Circle {
public:
const double PI = 3.14159; // constant variable
};
In this example, `PI` is a constant variable. Once initialized, it cannot be altered, ensuring that its value remains consistent, which is vital in calculations involving circles.

Access Modifiers and Their Impact on Variables
Public, Private, and Protected Access
Public Variables: These can be accessed from anywhere in the program, making them readily available but potentially compromising encapsulation.
Private Variables: These can only be accessed within the class itself. This encapsulation is a fundamental concept of object-oriented programming, allowing the internal state of the object to be protected from outside interference and misuse.
Code Example:
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
balance += amount;
}
};
The `balance` variable is private, which means it cannot be directly accessed outside the class. Only the `deposit` function can modify it, ensuring controlled access.
Protected Variables: Similar to private variables but are accessible within the class and by derived classes. This is useful in inheritance scenarios.
Code Example:
class Base {
protected:
int value;
};
class Derived : public Base {
public:
void setValue(int v) {
value = v; // Accessing protected variable
}
};
In this case, `value` is protected in the `Base` class, allowing derived classes like `Derived` to access it while preventing access from outside.

Initialization of Variables in a Class
Default Constructors
Understanding constructors is essential, as they initialize class variables at the time of object creation, providing a foundational setup for each new object instance.
Code Example:
class Book {
public:
std::string title;
double price;
Book() {
title = "Unknown";
price = 0.0;
}
};
In the `Book` class, the default constructor initializes `title` and `price` to default values when a `Book` object is created. This is important for ensuring that each object starts with valid data.
Parameterized Constructors
These constructors allow the user to specify initial values for class variables upon instantiation, offering flexibility.
Code Example:
class Laptop {
public:
std::string brand;
double price;
Laptop(std::string br, double pr) {
brand = br;
price = pr;
}
};
Here, the `Laptop` class uses a parameterized constructor, allowing the user to set the `brand` and `price` values when a `Laptop` object is created, providing meaningful state right from initialization.

Member Functions and Variable Interaction
Getters and Setters
Getters and setters are member functions allowing controlled access to private variables. They are fundamental for encapsulation, providing a way to modify and retrieve values without exposing internal data directly.
Code Example:
class Employee {
private:
std::string name;
public:
void setName(std::string n) {
name = n;
}
std::string getName() {
return name;
}
};
In the `Employee` class, `setName` is a setter method that enables modification of the private `name` variable, while `getName` is a getter method used to retrieve its value.
Friend Functions
Friend functions enhance flexibility by allowing specific functions to access private and protected variables of a class. This is particularly useful for operator overloading or when two classes need to work closely together.
Code Example:
class Box {
private:
int length;
public:
Box(int l) : length(l) {}
friend void displayLength(Box b);
};
void displayLength(Box b) {
std::cout << "Length: " << b.length; // Accessing private variable
}
The `displayLength` function is declared as a friend of the `Box` class, granting it access to the private `length` variable, enabling the function to perform operations that would otherwise be restricted.

Conclusion
Variables in a class form the backbone of object-oriented programming in C++. They reflect the properties and behaviors of the objects created and dictate how those objects interact with the rest of the program. From instance and static variables to access modifiers and constructors, understanding how these elements work together enhances your ability to design robust and maintainable code.
As you continue your journey in learning C++, consider diving deeper into advanced topics related to variable manipulation and class design. Each concept you grasp allows for more sophisticated programming techniques, making you a more proficient C++ developer.
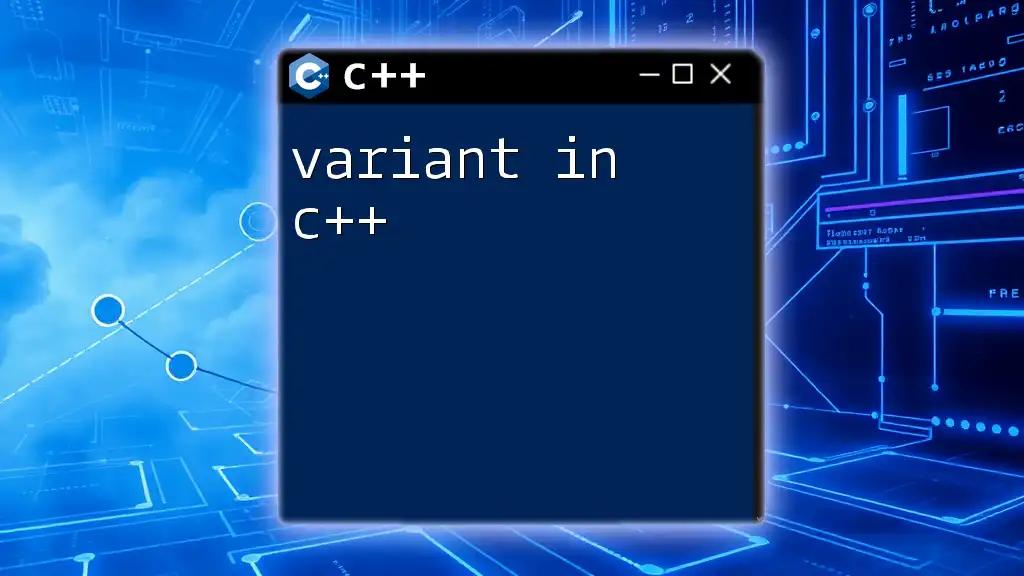
References
- Look for reputable C++ programming books and online resources for further reading and practice.

Call to Action
What are your experiences with variables in classes? Do you have any questions or topics you'd like to explore? Engage with us and share your thoughts!