An abstract class in C++ is a class that cannot be instantiated on its own and is designed to be a base class for other classes, typically containing at least one pure virtual function.
Here’s a simple example:
#include <iostream>
class AbstractClass {
public:
virtual void show() = 0; // Pure virtual function
};
class ConcreteClass : public AbstractClass {
public:
void show() override {
std::cout << "Concrete class implementation." << std::endl;
}
};
int main() {
// AbstractClass obj; // This would cause an error
ConcreteClass obj;
obj.show();
return 0;
}
Understanding the Concept of Abstract Classes
What is an Abstract Class?
An abstract class is a class that cannot be instantiated directly. It serves as a blueprint for other classes. Abstract classes are designed to be inherited by child classes, which must provide implementations for any pure virtual functions (also known as abstract methods).
Characteristics of Abstract Classes
- Cannot create instances: You cannot instantiate an abstract class on its own; it requires a derived class for instantiation.
- Contains pure virtual functions: At least one function must be declared as pure virtual.
- Supports polymorphism: Abstract classes facilitate polymorphism—the ability for different classes to be treated as instances of the same class through a common interface.
C++ Abstract Base Class
In C++, an abstract base class is a specialized type of abstract class that implements an interface. It usually provides a protocol for derived classes that must be followed.
Differences between Abstract Classes and Regular Classes
Regular classes can be instantiated and contain implementations for all their member functions, while abstract classes contain one or more pure virtual functions, making them abstract and non-instantiable.
Key features of a C++ Abstract Base Class
- Must include at least one pure virtual function.
- Signals a contract that derived classes must fulfill.
- Encourages adherence to a defined structure for derived classes.
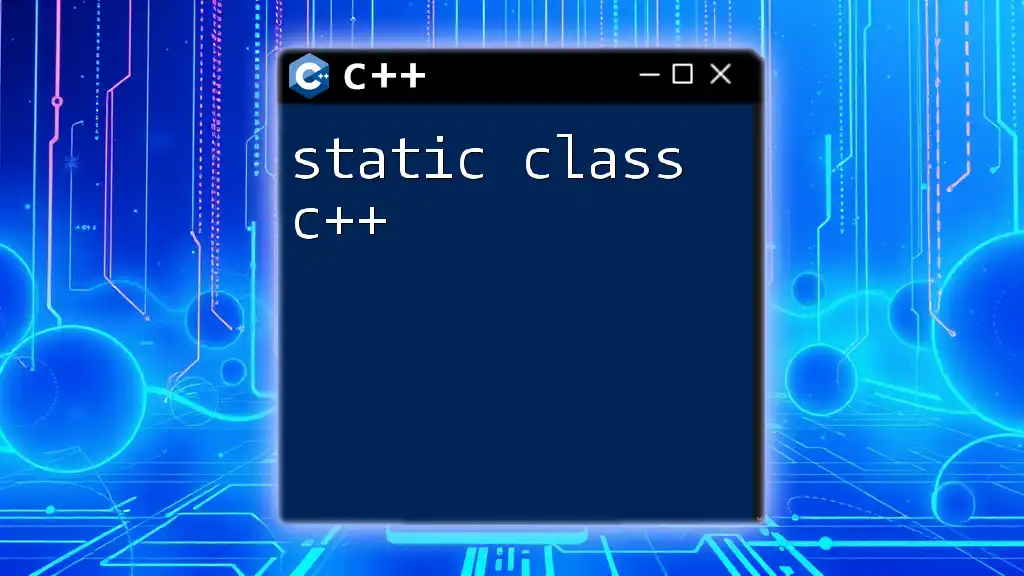
Syntax and Structure of Abstract Classes in C++
Declaring an Abstract Class
To declare an abstract class, you define a class with at least one pure virtual function. A pure virtual function is declared by appending `= 0` to the function prototype.
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
In the above example, `Shape` is an abstract class with one pure virtual function called `draw`. Since `draw` is not implemented in `Shape`, any derived class must provide an implementation.
Implementing Abstract Classes
Derived classes must implement all pure virtual functions to be instantiated. If a derived class does not implement all inherited pure virtual functions, it will also be considered abstract.
class Circle : public Shape {
public:
void draw() override {
// Implementation for Circle
std::cout << "Drawing a Circle" << std::endl;
}
};
class Square : public Shape {
public:
void draw() override {
// Implementation for Square
std::cout << "Drawing a Square" << std::endl;
}
};
In this code snippet, `Circle` and `Square` both implement the `draw` function, providing their specific implementations. These classes can now be instantiated.
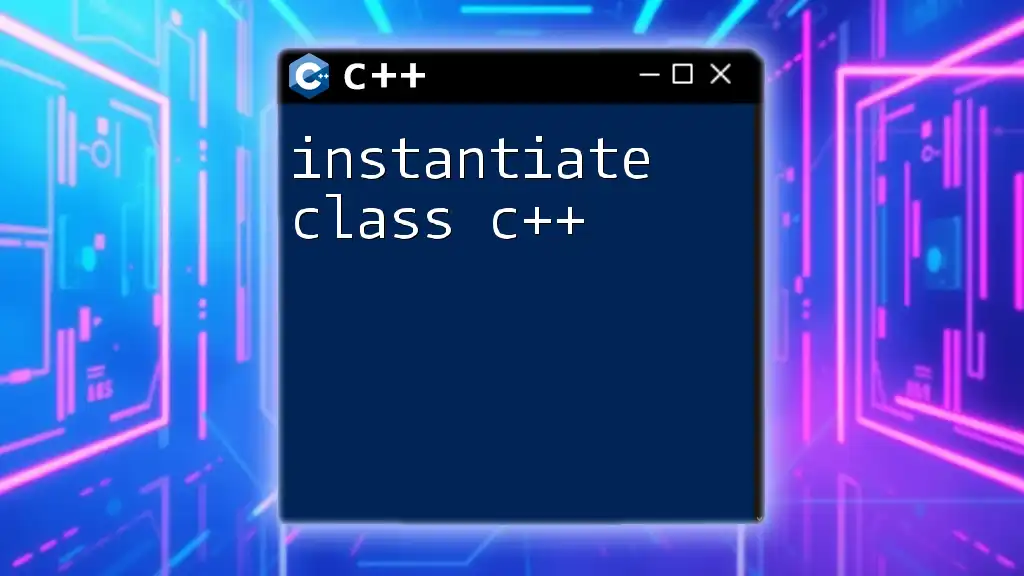
The Role of Virtual Functions
What are Virtual Functions?
Virtual functions are member functions declared within a base class that you expect to override in derived classes. Declaring a member function as virtual allows for dynamic dispatch, enabling the correct function to be called for an object, regardless of the type of reference or pointer used for the function call.
Pure Virtual Functions Explained
A pure virtual function is a virtual function that has no implementation in the base class. It forces any derived class to implement the function. You use this feature to establish a common interface while delegating the implementation details to derived classes.
class Vehicle {
public:
virtual void start() = 0; // Pure virtual function
};
In the `Vehicle` class, the `start` function is pure virtual, signaling that any derived class must provide its own implementation.
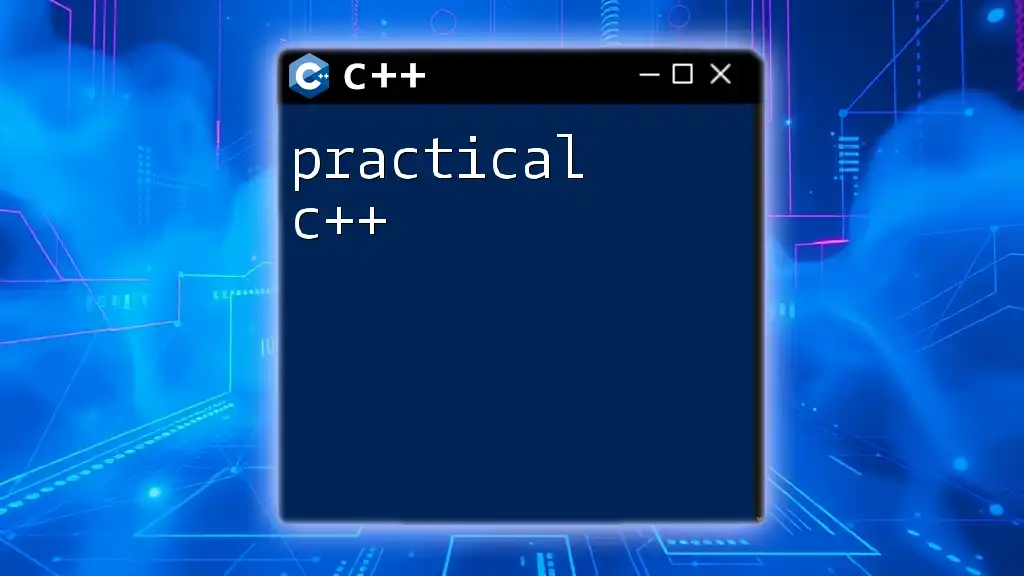
Advantages of Using Abstract Classes in C++
Enhancing Code Reusability
Abstract classes promote code reusability by allowing developers to define common interfaces and behavior that can be inherited and specialized by derived classes. This design pattern minimizes redundancy and organizes code more effectively.
For example, by creating an abstract class for different types of shapes, we can ensure all derived shape classes share core properties and methods while allowing each shape to maintain its unique behavior.
Facilitating Polymorphism
Polymorphism allows different classes to be treated as instances of the same class through a common interface. Abstract classes play a critical role in achieving polymorphism because they define function declarations that derived classes must implement.
void startVehicle(Vehicle *v) {
v->start(); // Polymorphic call to start
}
In this example, `startVehicle` can accept any derived class of `Vehicle`, allowing for flexible code that can handle multiple types of vehicles without modifying its internals.
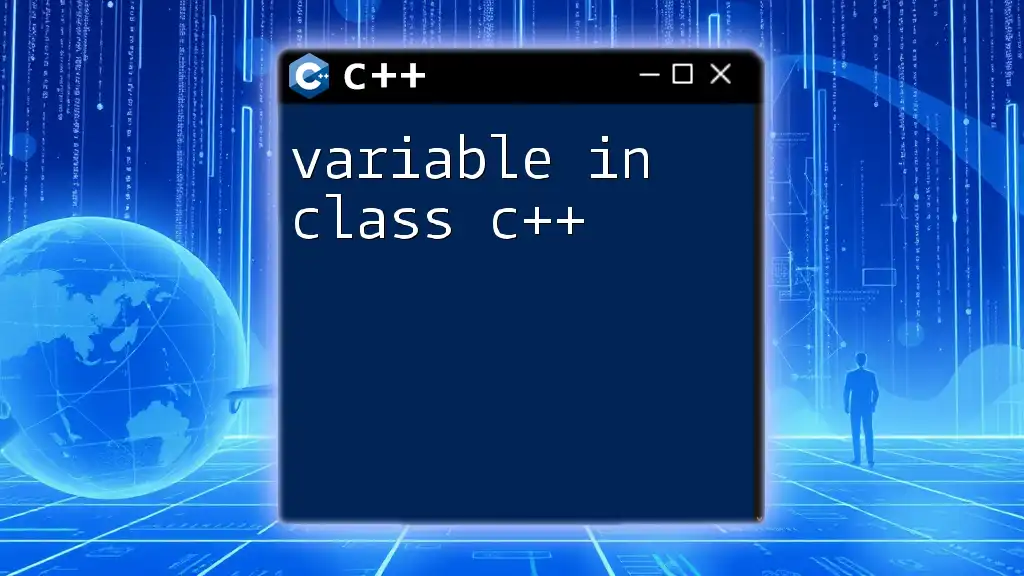
Common Use Cases for Abstract Classes
Implementing Interfaces
Abstract classes can serve as interfaces for application components. By declaring an interface via an abstract class, you can define a set of methods that implementing classes must provide, ensuring consistent use throughout the application.
Building Frameworks
Abstract classes are essential for software frameworks, where they define the structure and behavior expected from user-defined classes. For instance, in a game development framework, an abstract class could define basic properties and methods for all game entities, while specific entities (e.g., players, enemies) would implement these methods accordingly.
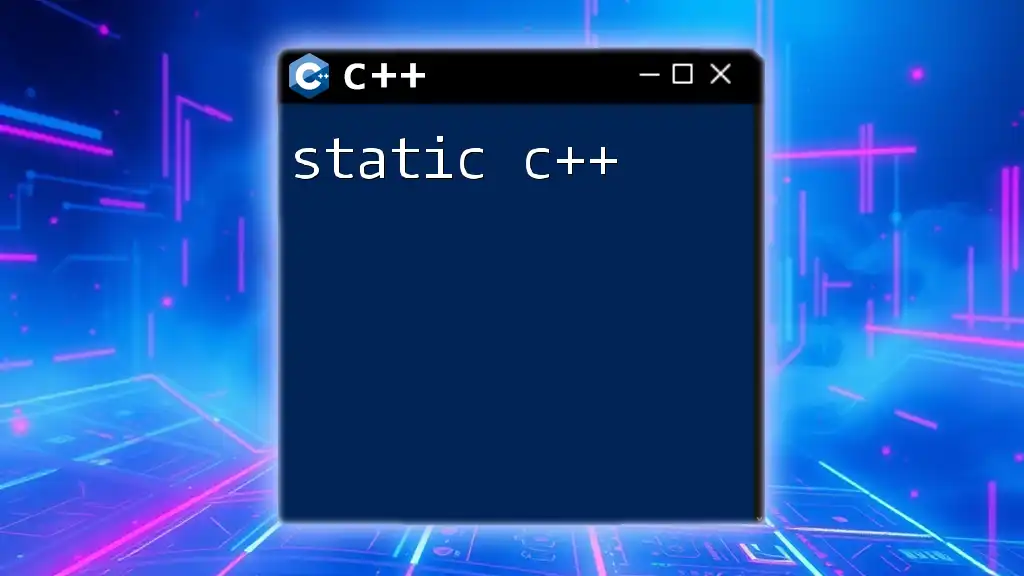
Best Practices for Using Abstract Classes in C++
Proper Naming Conventions
Using clear and descriptive names for your abstract classes and their methods is crucial. This practice not only enhances readability but also helps other developers understand the intended use and functionality at a glance.
Efficient Design Patterns
Familiarize yourself with design patterns such as the Factory pattern and Strategy pattern, which commonly utilize abstract classes. These patterns enhance code organization, enable separation of concerns, and facilitate extensibility and maintainability.
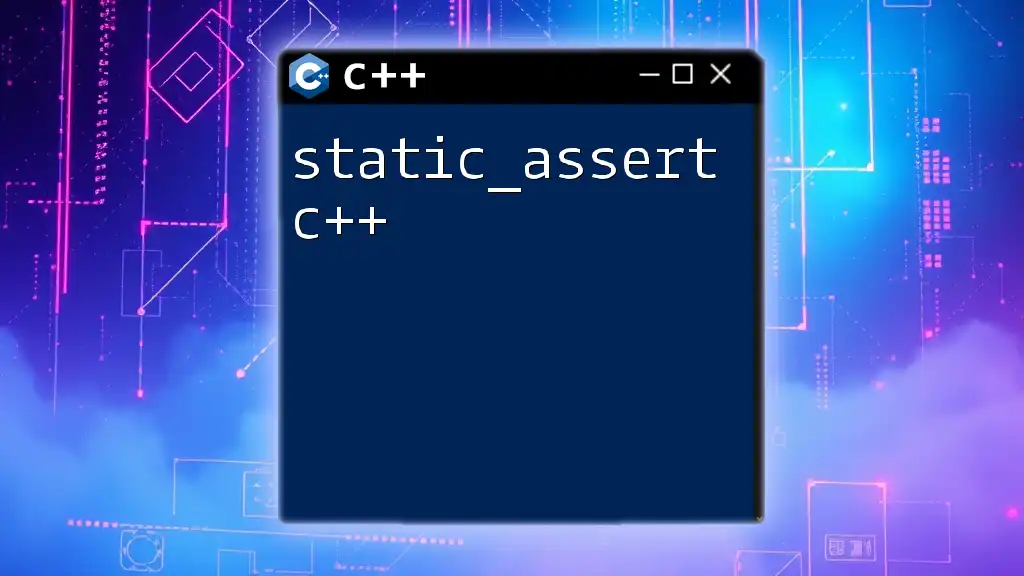
Conclusion
Abstract classes in C++ are powerful tools that enhance code structure, promote reusability, and facilitate polymorphism. Understanding and effectively implementing abstract classes can significantly improve your programming skills and lead to cleaner, more maintainable code. Embrace this important concept, and explore its applications in your projects for enhanced software design.
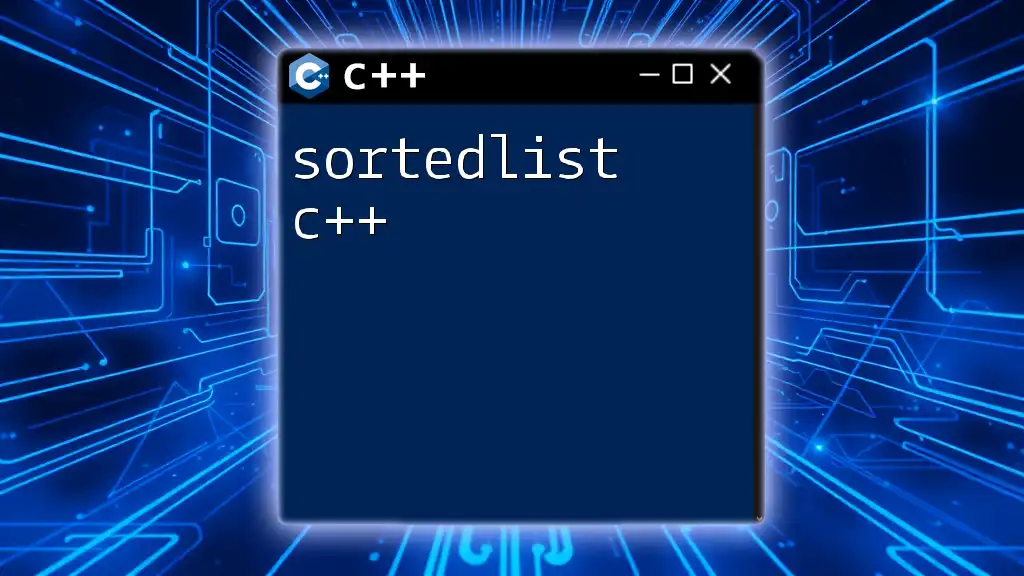
Additional Resources
For further learning, consider exploring recommended books, online courses, and documentation focused on abstract classes and advanced C++ programming techniques. Stay curious and keep coding!