In C++, arrays can be used within classes to store multiple values of the same type, allowing for organized data management and manipulation. Here's an example:
class NumberArray {
public:
int arr[5]; // Declare an array of integers with size 5
void setValue(int index, int value) {
if (index >= 0 && index < 5) {
arr[index] = value; // Set value at a specific index
}
}
int getValue(int index) {
if (index >= 0 && index < 5) {
return arr[index]; // Get value from a specific index
}
return -1; // Return -1 if index is out of bounds
}
};
Understanding Arrays and Classes
What is an Array in C++?
An array in C++ is defined as a collection of elements that share the same data type. This data structure is characterized by its fixed size and contiguous memory allocation, meaning that all elements are stored in sequential memory addresses, allowing for fast access to elements via indexing.
For example, if you have an array of integers, you can access each integer using its index: `myArray[0]`, `myArray[1]`, etc. Arrays are particularly useful for storing collections of similar types of data, such as a list of scores, names, or other related items.
What is a Class in C++?
A class in C++ can be thought of as a blueprint for creating objects. It encapsulates both data and functions that operate on that data, allowing for the modeling of real-world entities in a programming context. Classes provide key benefits like abstraction (hiding complex realities), encapsulation (restricting access to certain components), and code reusability.
A basic structure of a class consists of attributes (also known as data members) and methods (functions that belong to the class). An example class might look like this:
class Car {
public:
int speed;
void accelerate() {
speed += 10;
}
};
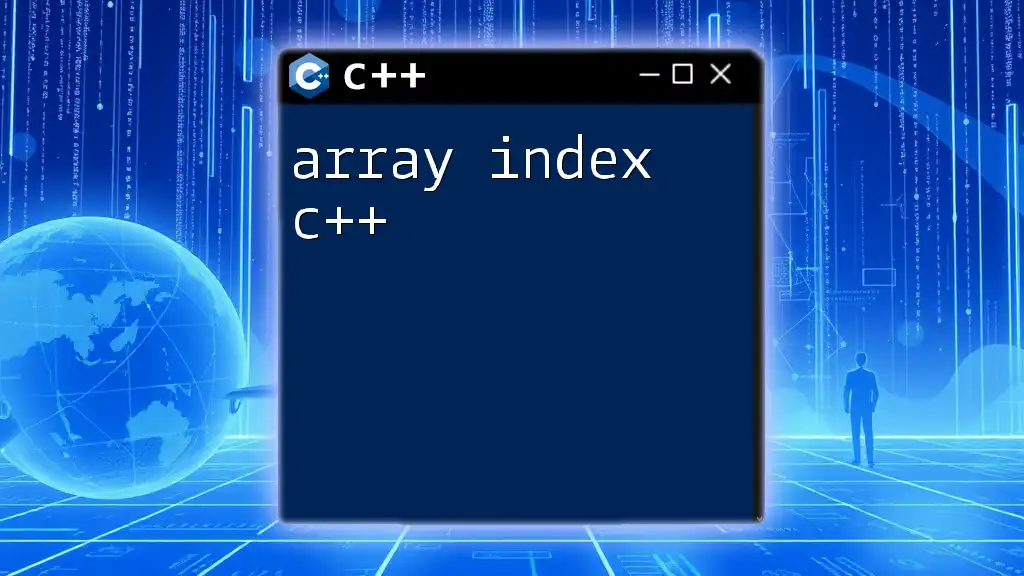
Integrating Arrays into Classes
Why Use an Array in a C++ Class?
Using an array within a C++ class allows for efficient data management, especially when dealing with multiple related pieces of data.
Encapsulating an array within a class enables methods to manipulate the array data directly, promoting a clean interface and enhancing the maintainability of your code. Additionally, arrays provide flexibility, allowing developers to define various data types according to the class design.
Declaring an Array in a C++ Class
To declare an array within a class, we set it as a private member to encourage encapsulation. Here's an example:
class MyArrayClass {
private:
int myArray[10]; // Array of integers
public:
void setElement(int index, int value) {
myArray[index] = value; // Setting array element
}
int getElement(int index) {
return myArray[index]; // Getting array element
}
};
In this example, `myArray` is a private member variable, accessible only through the public methods `setElement` and `getElement`. This structure demonstrates how we can manage array data while preserving the principles of encapsulation.
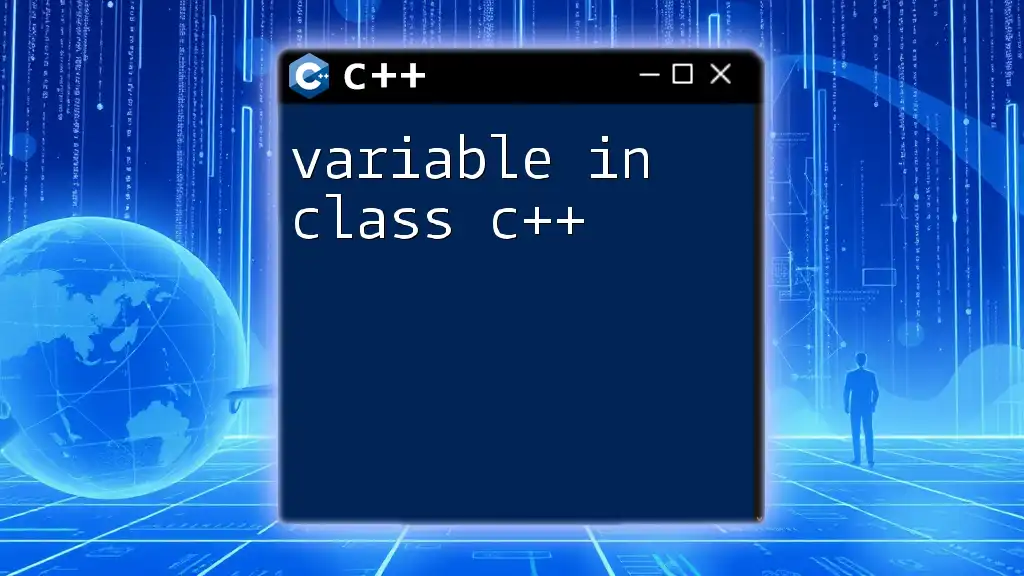
Creating a C++ Class with Array
Step-by-step Guide
Step 1: Defining the Class
When defining a class that incorporates an array, you need to identify the size and type of the array based on your requirements.
Step 2: Initializing the Array
A common method is to use dynamic memory allocation for array size flexibility. Here’s how you can do it:
class MyArray {
private:
int size;
int* array; // Dynamic array
public:
MyArray(int s) : size(s) {
array = new int[size]; // Dynamic allocation
}
In this snippet, we've declared a dynamic array, allowing us to set its size at runtime rather than compile time. This makes our class adaptable to various data needs.
Step 3: Implementing Constructor and Destructor
Proper object construction and destruction are critical for resource management. Implementing a constructor and destructor would look like this:
~MyArray() {
delete[] array; // Memory cleanup
}
This destructor ensures that we free up any dynamically allocated memory when an instance of `MyArray` goes out of scope, thereby preventing memory leaks—one of the most common issues in C++ programming.
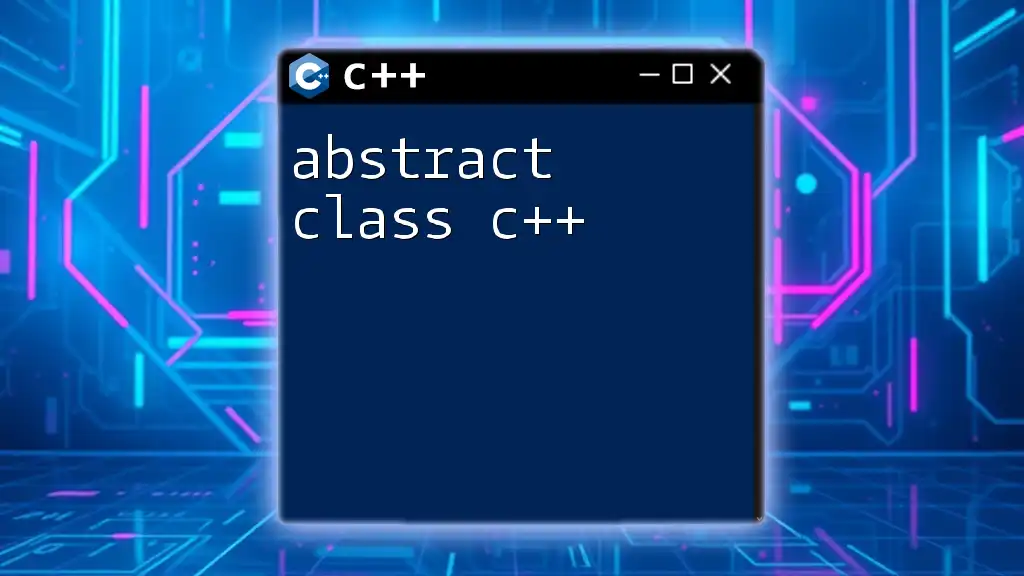
Using the Array in the Class
Accessing and Modifying Elements
An essential aspect of using arrays in classes is accessing and modifying their elements. Here’s an example method to set a value in the array:
void setElement(int index, int value) {
if (index >= 0 && index < size) {
array[index] = value; // Bound checking
}
}
Implementing bounds checking is crucial to ensure that we do not attempt to access or modify an index that does not exist, which can lead to undefined behavior.
Iterating Over the Array
Iterating through the array is another necessary operation, especially when you want to display its content or perform bulk operations. Here's a simple function to print all elements of the array:
void printArray() {
for (int i = 0; i < size; i++) {
std::cout << array[i] << " "; // Print each element
}
std::cout << std::endl;
}
This loop iterates over the elements and prints them out. Such iterations can be beneficial when you need to retrieve or manipulate all data stored in the array.
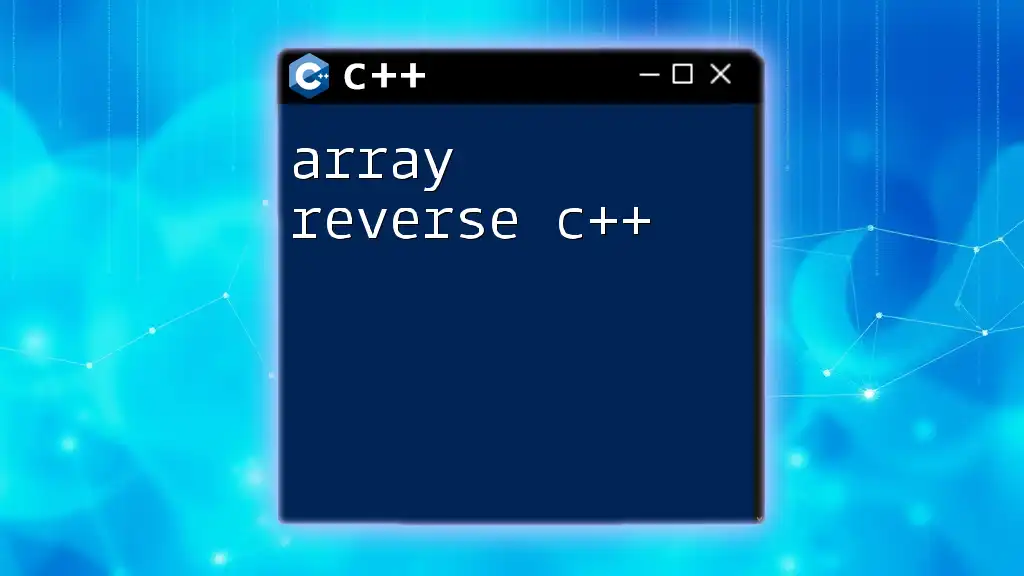
Real-world Applications
Example: Using Classes with Arrays in Practical Scenarios
Use Case 1: Inventory Management System
One practical application of combining arrays and classes is in an inventory management system. Below is a simplified class to manage the inventory:
class Inventory {
private:
std::string items[100]; // Array of item names
int itemCount;
public:
void addItem(std::string item) {
if (itemCount < 100) {
items[itemCount++] = item; // Add item to inventory
}
}
void viewItems() {
for (int i = 0; i < itemCount; i++) {
std::cout << items[i] << std::endl; // View all items
}
}
};
In this example, the `Inventory` class leverages an array to store item names, offering methods to add and view items. This clearly illustrates how arrays can enhance the organization and functionality of your program.
Example: Student Grades Management
Consider another scenario where you are managing student grades. Below is a simplistic implementation of such a class:
class Student {
private:
std::string name;
int grades[5]; // Array of grades
public:
void setGrades(int g1, int g2, int g3, int g4, int g5) {
grades[0] = g1; // ... and so forth
}
};
This class can be expanded to include methods for calculating averages or displaying grades, demonstrating how arrays can efficiently manage multiple related data points.
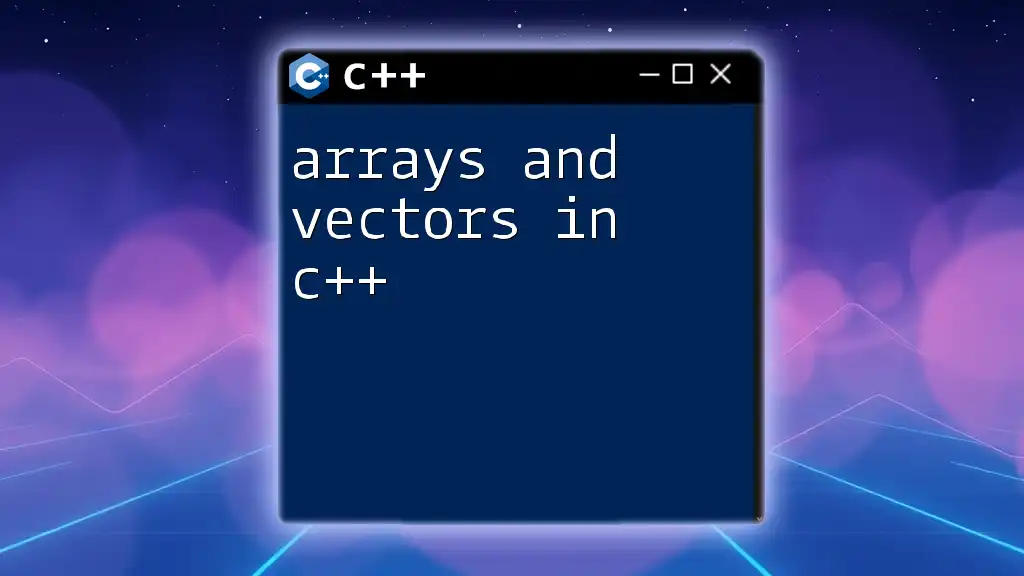
Conclusion
Recap of Arrays in C++ Class
Understanding arrays and classes in C++ is vital for writing effective and organized code. Utilizing arrays within classes not only streamlines data management but also provides a more structured approach to programming.
Further Learning Resources
To deepen your knowledge in C++, consider exploring recommended books and online courses that focus on advanced features, including data structures and object-oriented programming concepts.
Final Thoughts
Mastering both arrays and classes is a key step in your journey to becoming a proficient C++ developer. Delve into these concepts, practice, and you'll find they offer tremendous power in your programming toolkit.