In C++, arrays are fixed-size collections of elements of the same type, while strings are used to represent sequences of characters, allowing easy manipulation of text; here is an example that demonstrates both concepts:
#include <iostream>
#include <string>
int main() {
// Array of integers
int numbers[5] = {1, 2, 3, 4, 5};
// String
std::string greeting = "Hello, World!";
// Output array and string
for(int i = 0; i < 5; i++) {
std::cout << numbers[i] << " "; // Output array elements
}
std::cout << std::endl << greeting; // Output string
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of items stored at contiguous memory locations. They are primarily used for storing multiple values of the same type, enabling easier management of data. Arrays have several key characteristics: they are of a fixed size once declared, and they hold homogeneous elements, meaning all items must be of the same data type.
Declaring and Initializing Arrays
In C++, arrays can be declared using the following syntax:
data_type array_name[array_size];
For example, to declare an integer array of size 5, you can write:
int numbers[5];
You can also initialize the array at the time of declaration:
int numbers[5] = {1, 2, 3, 4, 5};
This assigns the values 1 through 5 to the array `numbers`.
Accessing Array Elements
Array elements are accessed using their indices, starting from `0`. For instance, to access the third element in the `numbers` array, you can use:
int thirdElement = numbers[2]; // Accessing the third element
You can also modify an array element easily. Here’s how to change the third element to `10`:
numbers[2] = 10; // Change the third element to 10
Multidimensional Arrays
C++ allows the creation of multidimensional arrays, most commonly 2D arrays, which can be thought of as an array of arrays. You declare and initialize a 2D array like this:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
To access elements in a 2D array, you provide two indices. Here’s an example of accessing the element in the first row and second column:
int value = matrix[0][1]; // Accessing first row, second column (value: 2)
Common Operations on Arrays
When working with arrays, basic operations are fundamental. Traversing through arrays is usually done using loops. Here’s a simple example that prints the elements of the `numbers` array:
for(int i = 0; i < 5; i++) {
std::cout << numbers[i] << " ";
}
This will output: `1 2 10 4 5`.
You can find the length of an array in C++ using the `sizeof` operator:
size_t length = sizeof(numbers) / sizeof(numbers[0]);
This calculates the number of elements in the array by dividing the total size of the array by the size of a single element.
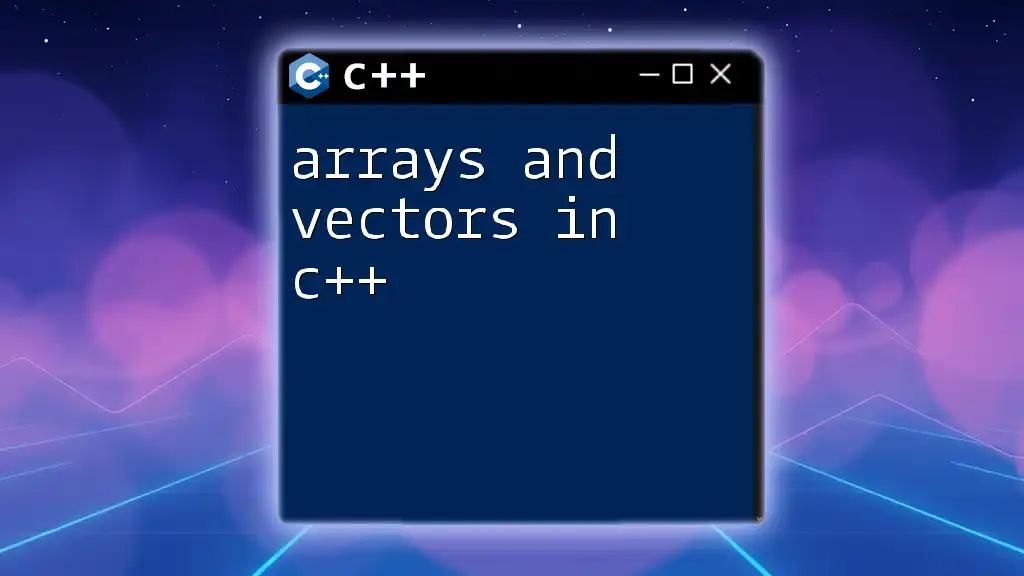
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters. There are two main ways to handle strings: using C-style strings or the C++ `string` class. C-style strings are arrays of characters terminated by a null character (`'\0'`), while the `string` class provides a more intuitive and safer approach with rich functionalities.
Declaring and Initializing Strings
For C-style strings, you might declare them as follows:
char greeting[6] = "Hello";
To use the C++ `string` class, you can do this:
std::string welcome = "Hello, World!";
Accessing String Characters
You can easily access individual characters within a string using indices. For example, to get the first character of a C++ string:
char firstChar = welcome[0]; // 'H'
String Operations
The C++ string class offers various operations to manipulate strings:
-
Concatenation can be done using the `+` operator to concatenate two strings:
std::string name = "Alice"; std::string fullGreeting = welcome + " " + name; // "Hello, World! Alice"
-
To find the length of a string, use the `length()` method:
size_t length = welcome.length(); // Returns the length of "Hello, World!"
-
To extract a substring, use the `substr()` method:
std::string sub = welcome.substr(0, 5); // Returns: "Hello"
Comparing Strings
String comparison in C++ can be done using the `==` operator:
if (name == "Alice") {
// Do something
}
The C++ string class handles comparisons safely, making it much easier than comparing C-style strings that require functions like `strcmp`.
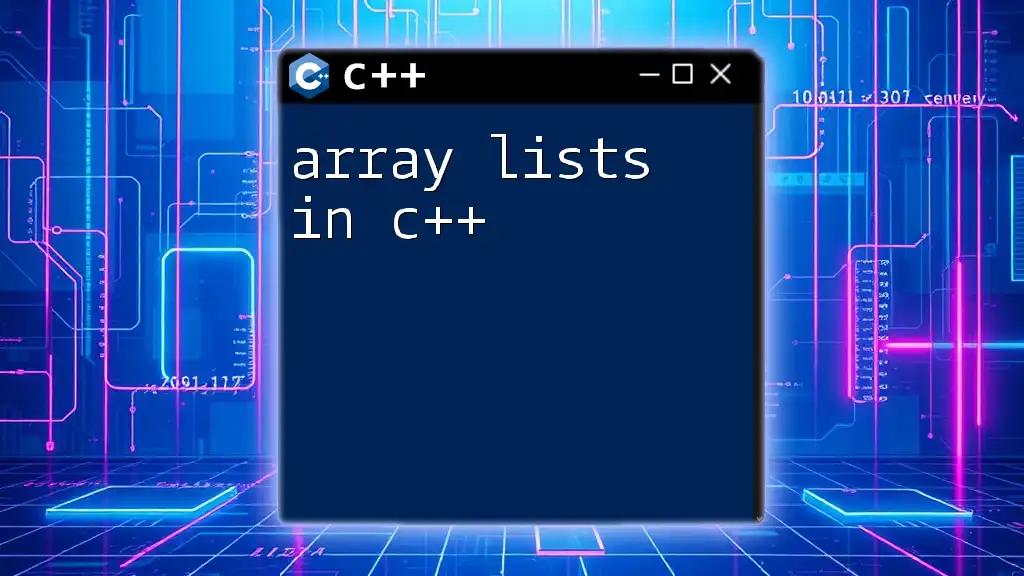
Combining Arrays and Strings
Arrays of Strings
When you need to store multiple strings, you can create an array of strings, declared like this:
std::string names[] = {"Alice", "Bob", "Charlie"};
This line initializes an array named `names` containing three strings.
Operations with Arrays of Strings
You can loop through an array of strings just like you do with simple arrays. Here's an example that displays each name:
for(int i = 0; i < 3; i++) {
std::cout << names[i] << std::endl; // Prints each name on a new line.
}

Best Practices in Using Arrays and Strings
Choosing Between Arrays and Vectors
In many situations, especially when dealing with dynamically sized collections, consider using `std::vector`. Vectors are part of the C++ STL and offer several advantages, including dynamic sizing and efficient memory management, which reduces the risk of memory overflow.
Memory Management
Understanding memory management is crucial when working with arrays. Arrays can be allocated on the stack, which is limited in size, or on the heap, where memory can be dynamically allocated. For dynamic arrays, use:
int* dynamicArray = new int[size]; // Dynamically allocate memory
When finished, don’t forget to free the memory:
delete[] dynamicArray; // Deallocate memory
String Handling Safety
When using C-style strings, avoid buffer overflow by ensuring that there is always room for the terminating null character (`'\0'`). The C++ `string` class provides automatic memory management, making it a safer and easier alternative for handling strings.
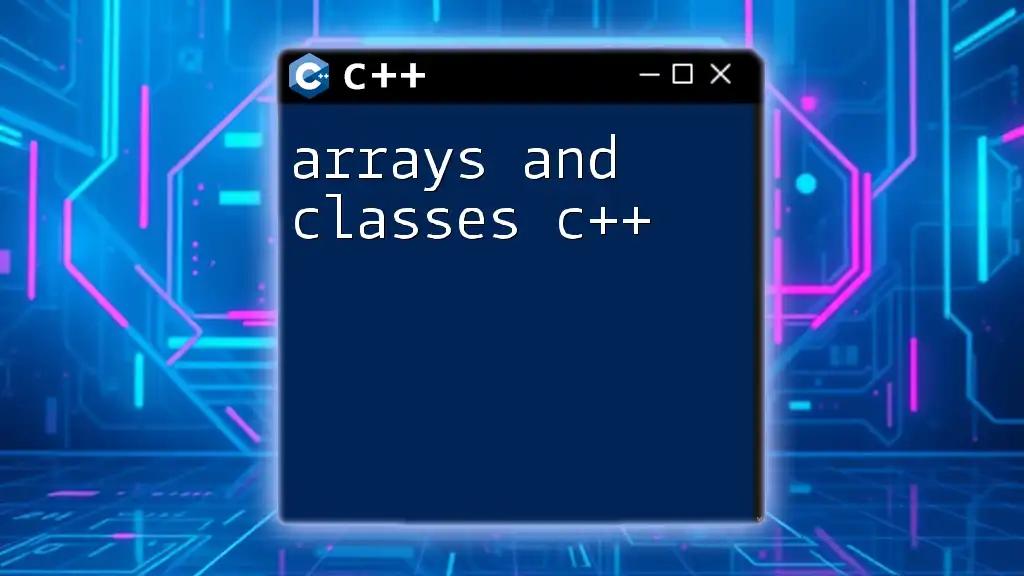
Conclusion
The mastery of arrays and strings in C++ is vital for programming effectively in this language. Understanding how to work with both allows for efficient data storage and manipulation, which will enhance your coding projects. Practice through exercises and small projects involving arrays and strings to solidify your understanding and improve your skills.
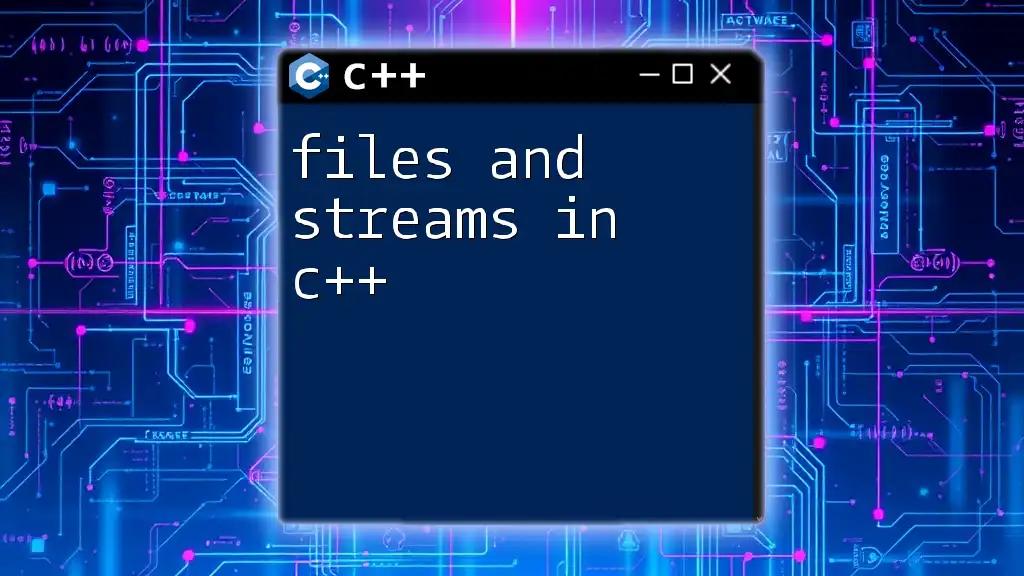
Additional Resources
To further enhance your knowledge, consider exploring additional resources available online, including C++ documentation, tutorials, and coding platforms where you can engage in real-time coding practice. Make use of these resources to deepen your understanding of arrays, strings, and other crucial elements of C++.