Arrays and vectors in C++ are both used to store collections of data, but arrays have a fixed size while vectors are dynamic and can grow or shrink in size as needed.
Here's a simple example demonstrating both:
#include <iostream>
#include <vector>
int main() {
// Array example
int myArray[5] = {1, 2, 3, 4, 5};
// Vector example
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.push_back(6); // Dynamically adds an element
// Displaying elements
for(int i : myArray) std::cout << i << " "; // Output: 1 2 3 4 5
std::cout << std::endl;
for(int i : myVector) std::cout << i << " "; // Output: 1 2 3 4 5 6
return 0;
}
Understanding Arrays in C++
Definition of Arrays
In C++, an array is a collection of elements of the same data type, stored under a single name. The size of an array must be defined at compile time, meaning you cannot change its size during the program's execution.
The syntax for declaring an array looks like this:
int arr[5]; // This declares an array of integers with size 5
Characteristics of Arrays
- Fixed Size: The size of an array must be specified upon initialization, making it unchangeable throughout its lifetime. This rigidity can be a limitation in situations where the volume of data isn't known beforehand.
- Homogeneous Data Types: All elements within an array are of the same type, whether integers, floats, or other data types. This feature allows for organized and efficient data handling but restricts data diversity within a single array.
- Storage in Contiguous Memory: Arrays allocate memory in a single block, which leads to quick access to each element based on its index. This property significantly enhances performance when reading data.
Accessing Array Elements
You access array elements using their index, which starts from 0. For instance, to modify and retrieve the first element of an array:
arr[0] = 5; // Assigning a value
int val = arr[0]; // Accessing the value
This code assigns the value `5` to the first element of `arr` and then retrieves that value.
Limitations of Arrays
While arrays are excellent for storing fixed-size collections, they have some distinct disadvantages:
- Fixed Size Issues: If you need to increase or decrease the number of elements, you must create a new array and copy the data, which can be inefficient.
- Lack of Built-in Range Checking: Accessing an array out of its defined bounds may lead to undefined behavior, making error handling complex and potentially leading to security vulnerabilities.
- Complexity in Adding/Removing Elements: Adding or removing elements can be cumbersome since elements may require shifting.
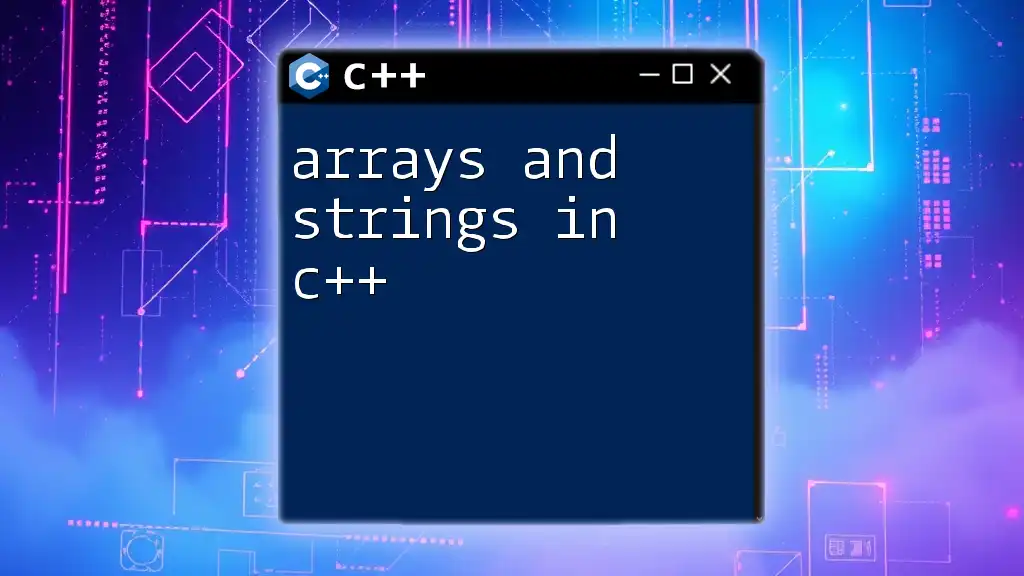
Understanding Vectors in C++
Definition of Vectors
A vector in C++ is part of the Standard Template Library (STL) and represents a dynamic array that can grow and shrink automatically. Vectors are flexible and allow for easy management of collections of data.
The syntax for declaring a vector looks like this:
#include <vector>
std::vector<int> vec; // This declares a vector of integers
Characteristics of Vectors
- Dynamic Size: Unlike arrays, vectors can dynamically allocate or release memory as elements are added or removed. This feature is particularly useful when the total number of items isn't known in advance.
- Homogeneous Data Types: Similar to arrays, vectors also store elements of a single data type. This ensures consistency and integrity of data.
- Memory Management: Vectors manage their own memory. When the capacity is exceeded, vectors may automatically allocate a larger memory block and copy existing elements to this new location.
Accessing Vector Elements
You can access and manipulate vector elements using the same indexing method as arrays. Here’s a simple example:
vec.push_back(10); // Adding an element to the vector
int val = vec[0]; // Accessing the first element
`push_back` adds `10` to the end of the vector, and we retrieve the first value just like with an array.
Advantages of Vectors Over Arrays
Vectors offer several advantages:
- Automatic Memory Management: You do not have to worry about memory allocation or deallocation, as vectors handle that automatically.
- Built-in Functions: Vectors come with numerous built-in functions for operations, such as adding (`push_back`), removing (`pop_back`), and resizing. For example:
vec.push_back(20); // Adds 20 to the end of the vector
vec.pop_back(); // Removes the last element
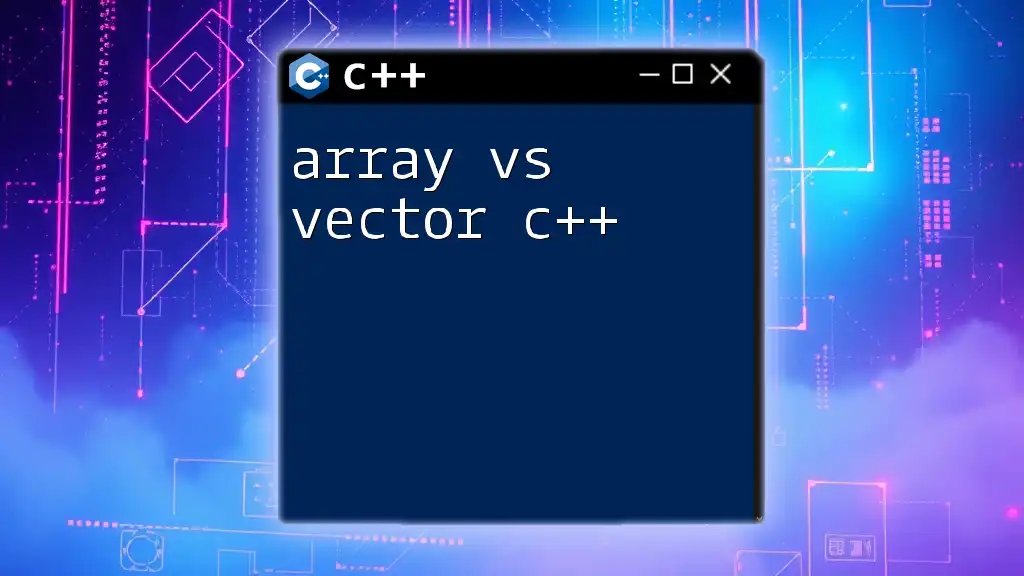
C++ Arrays vs Vectors
Performance Comparison
When considering arrays and vectors in C++, understanding performance is crucial:
- Arrays provide rapid access time given their contiguous memory layout. This makes them favorable in performance-critical applications where size is constant.
- Vectors, while more flexible, may involve a minor overhead due to their dynamic nature. However, this overhead is often negligible compared to the benefits gained through automatic memory management.
Code Examples
To illustrate the performance characteristics, consider the following scenarios:
// Array Example
int arr[1000000]; // Allocating a large array directly
// Vector Example
std::vector<int> vec(1000000); // Initializing with size but can easily resize
While both can handle large data sets, vectors afford greater flexibility without the need for manual memory management.
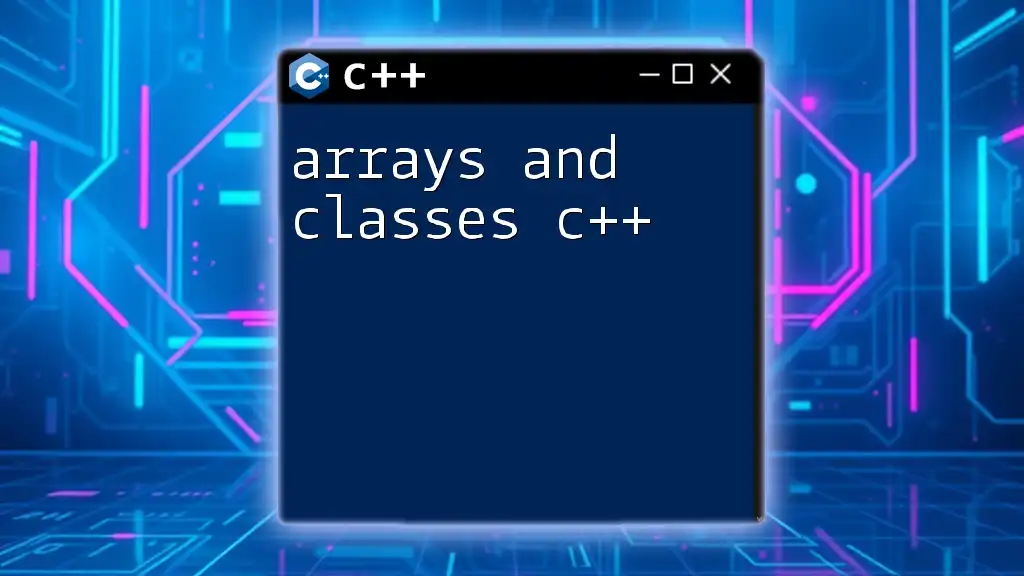
Use Cases of Arrays and Vectors
When to Use Arrays
Arrays are best suited for scenarios where:
- The size of data is known and constant, such as in embedded systems.
- Maximum speed and memory efficiency are essential, and the flexibility of size is unnecessary.
When to Use Vectors
Vectors are ideal for:
- Situations where the size of data can fluctuate, such as user-driven inputs or variable datasets.
- Programs that benefit from the convenience of automatic memory handling and easier resizing.
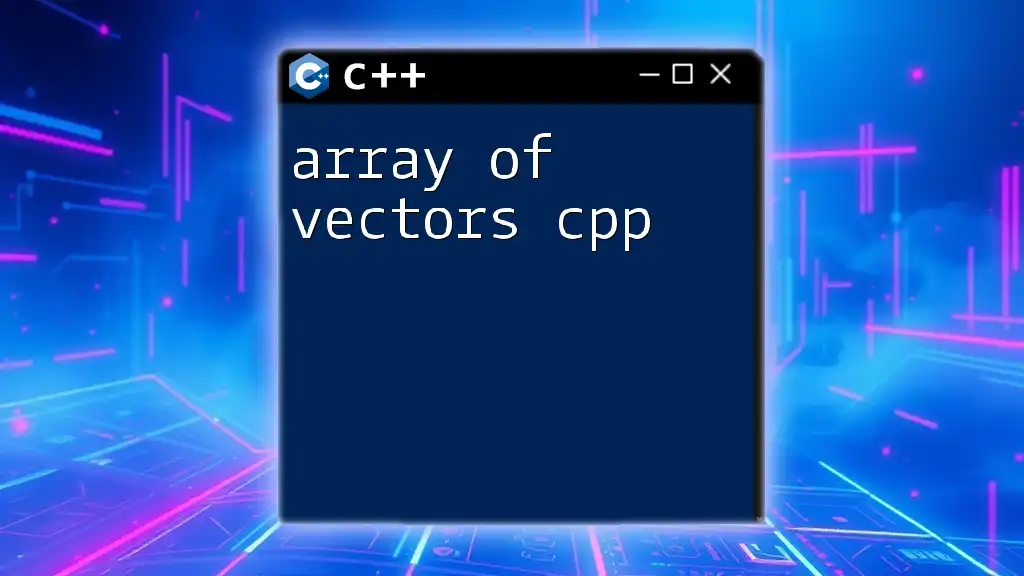
Common Operations on Arrays and Vectors
Iterating Through Arrays and Vectors
A fundamental operation when working with both arrays and vectors is iteration:
// Iterating through an array
for(int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
// Iterating through a vector
for(const auto& val : vec) {
std::cout << val << " ";
}
Searching in Arrays and Vectors
Searching techniques can differ:
- Linear Search for both arrays and vectors, where you traverse each element to find the desired value.
- For sorted data, Binary Search applies more efficiently to vectors.
Sorting Arrays and Vectors
Sorting can utilize various techniques. C++ offers an easy way to sort vectors using built-in functions:
std::sort(vec.begin(), vec.end()); // Sorting the vector
This command leverages the STL to arrange elements in ascending order swiftly.
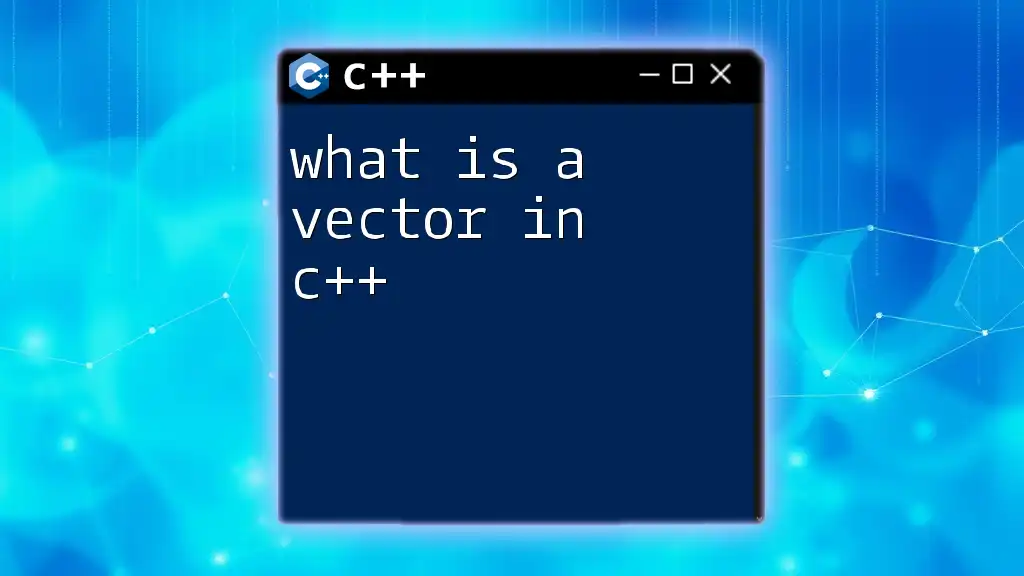
Conclusion
Understanding arrays and vectors in C++ is foundational to effective programming. While both serve to hold collections of data, knowing their characteristics, advantages, and use cases will enable you to choose the right structure based on your specific needs. By grasping these concepts, you are better equipped to write efficient and effective C++ code.
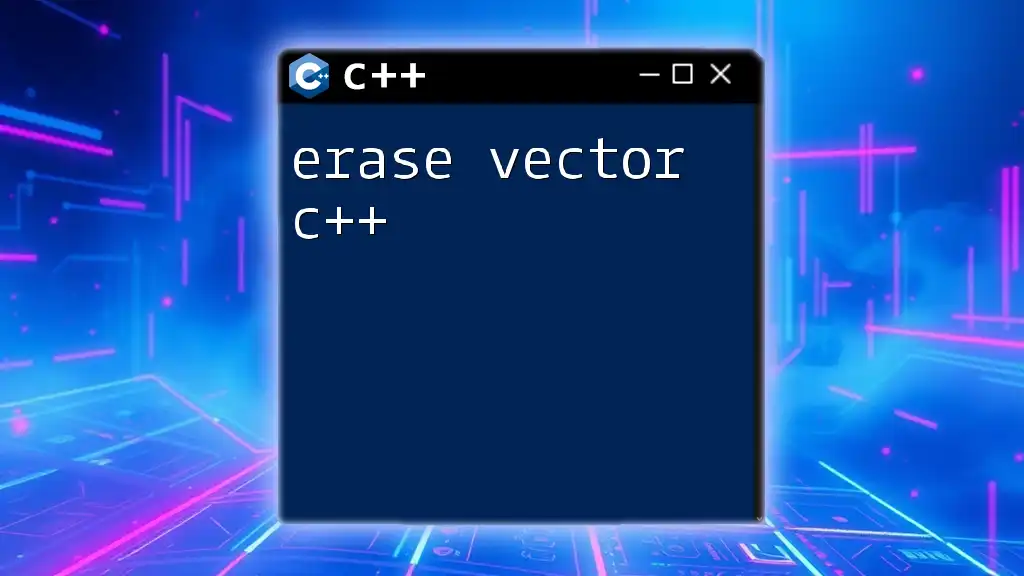
Call to Action
Dive into coding hands-on with these examples! Learn more about C++ programming by signing up for our tutorials and courses, where you'll master not just arrays and vectors, but the entire C++ language, preparing you for real-world applications.