In C++, an array index is a zero-based integer used to access individual elements in an array, allowing you to retrieve or modify values stored in that array.
Here's a simple example:
#include <iostream>
int main() {
int numbers[] = {10, 20, 30, 40, 50};
std::cout << "The first element is: " << numbers[0] << std::endl; // Accessing the first element
return 0;
}
Understanding Arrays in C++
What is an Array?
An array in C++ is a collection of variables that are stored in contiguous memory locations. It allows you to store multiple items of the same type under a single variable name, enabling easier data manipulation. For example, if you wanted to store the ages of ten students, instead of creating ten different variables, you could simply use an array.
Declaring a basic array involves specifying its data type and size, as shown in the following code snippet:
int ages[10]; // Declares an array of integers with 10 elements
Types of Arrays in C++
Arrays come in several types, which can cater to various programming needs.
One-Dimensional Arrays
A one-dimensional array is the simplest form, representing a linear sequence of elements. This type of array is used when you need to store a list of items.
You can declare a one-dimensional array and initialize it like this:
int numbers[5] = {1, 2, 3, 4, 5}; // Creates and initializes an array of integers
Multi-Dimensional Arrays
A multi-dimensional array allows the storage of data in a table-like structure. The most common is the two-dimensional array, often used to represent matrices.
For instance, here's how you could declare and initialize a 2D array:
int matrix[2][3] = {
{1, 2, 3},
{4, 5, 6}
}; // A 2x3 matrix
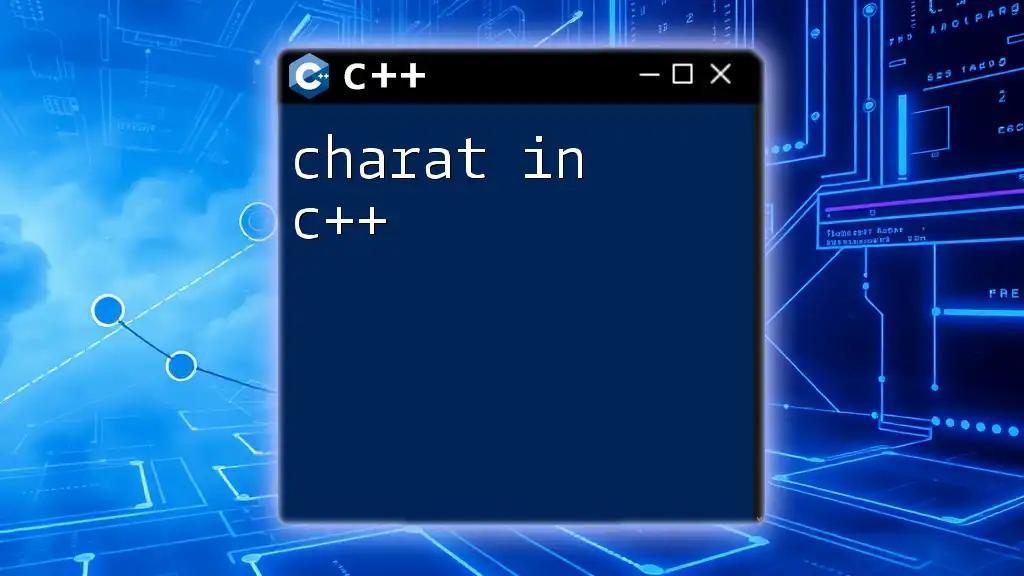
The Concept of Array Indexing in C++
What is Array Indexing?
Array indexing refers to the way we access elements within an array using their respective positions known as indices. C++ employs zero-based indexing, meaning that the first element of an array is accessed with index 0.
Understanding indexing is crucial for writing effective and efficient algorithms, as it allows direct access to specific elements.
Rules of Array Indexing
Each index in an array belongs to a valid range, starting from 0 up to `size - 1`. Accessing an index outside this range results in undefined behavior, potentially causing program crashes. Consider the following illustration:
int arr[3] = {10, 20, 30};
int value = arr[3]; // This will lead to undefined behavior
Negative indices are not valid in C++. If used, they lead to accessing unexpected portions of memory, which can lead to bugs or data corruption.
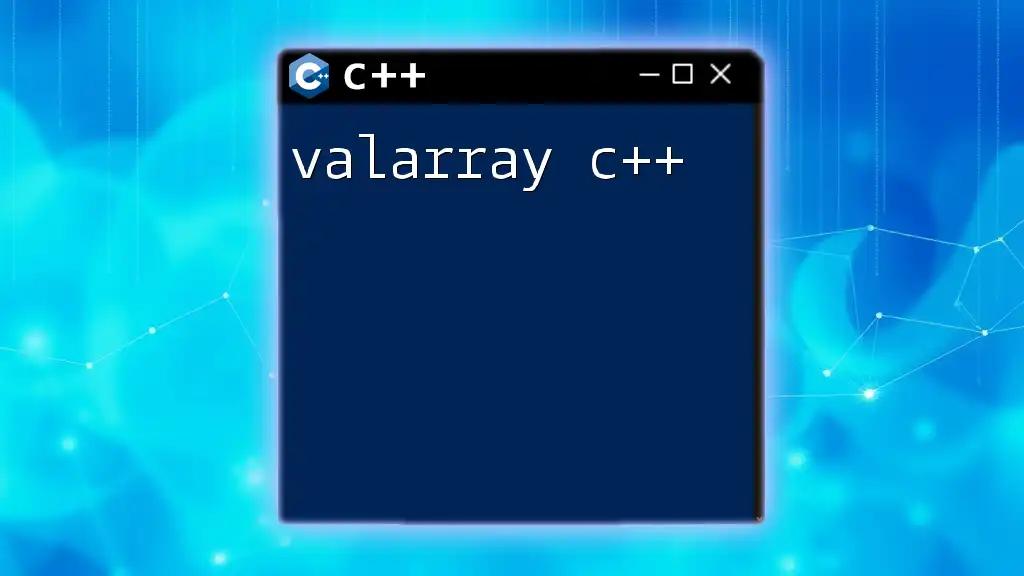
Accessing Array Elements
Reading Array Elements
Accessing elements in an array uses the syntax `array_name[index]`. Here’s how you can retrieve an array element:
int element = arr[1]; // Retrieves the second element (20) from the array
Modifying Array Elements
Changing the value of an array element is just as straightforward. Using the indexing syntax, you can set a new value:
arr[1] = 99; // Changes the second element from 20 to 99
Understanding the implications of array indexing is vital, as incorrect manipulations can lead to unexpected behavior or errors.
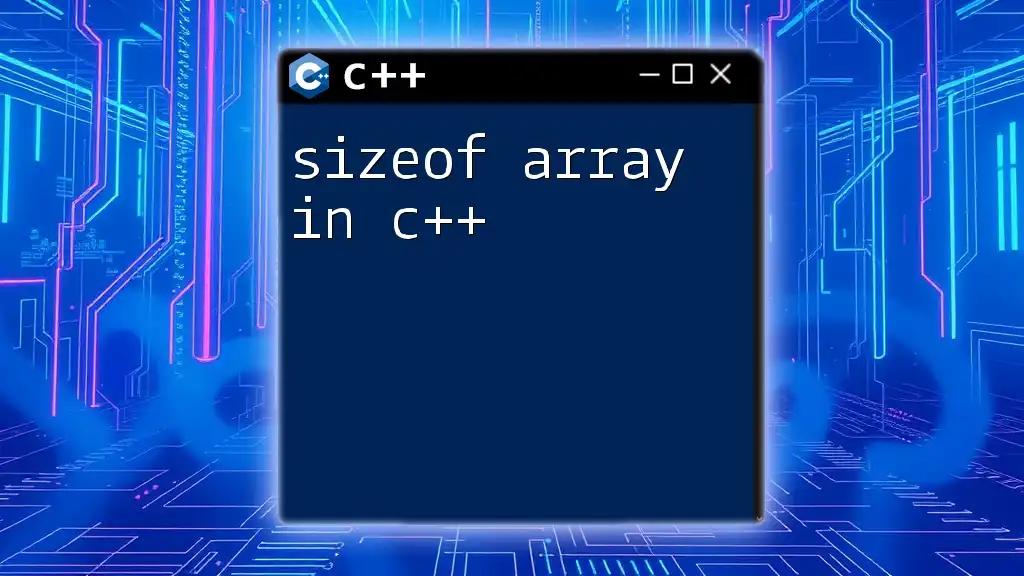
Array Indexing Techniques
Using Loops for Traversing Arrays
You can efficiently traverse arrays using loops. This is particularly useful for reading or modifying each element. Below is an example using a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << numbers[i] << " "; // Outputs each element of the array
}
Advanced Indexing Techniques
Pointers in C++ offer another powerful way to work with arrays. An array name can be treated as a pointer to its first element, thus allowing manipulation through pointer arithmetic. Here’s a snippet demonstrating this concept:
int* ptr = numbers; // Pointing to the first element
std::cout << *(ptr + 2); // Accesses the third element
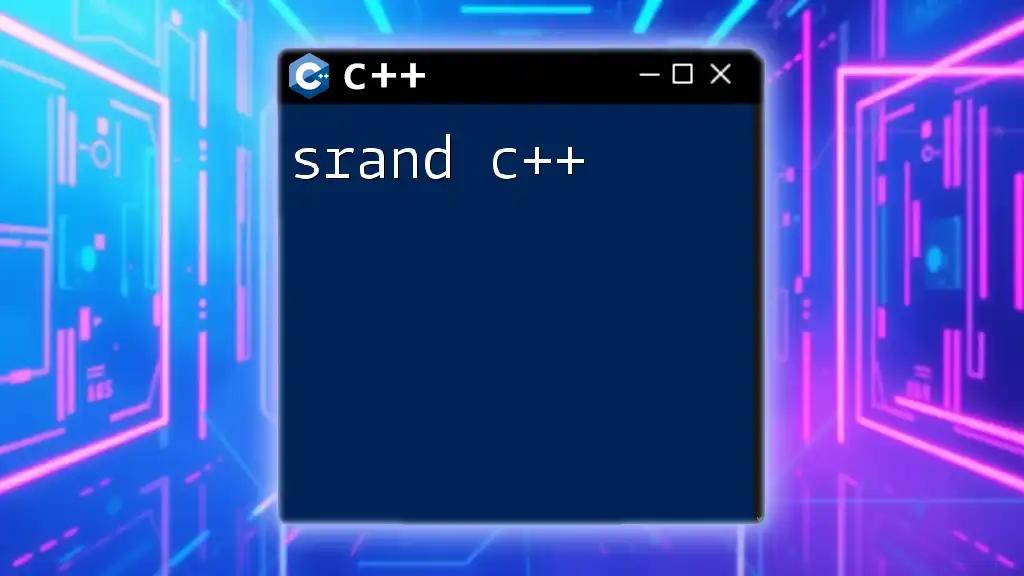
Common Use Cases for Array Indexing
Searching in Arrays
Searching algorithms, like linear search or binary search, utilize array indexing for locating desired elements. Here’s a simple linear search example:
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target) {
return i; // Returns the index where the target is found
}
}
return -1; // Target not found
}
Sorting Arrays
Sorting is essential for many algorithms and affects the efficiency of array indexing. Consider using a simple sorting algorithm, such as bubble sort:
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]); // Swap the elements
}
}
}
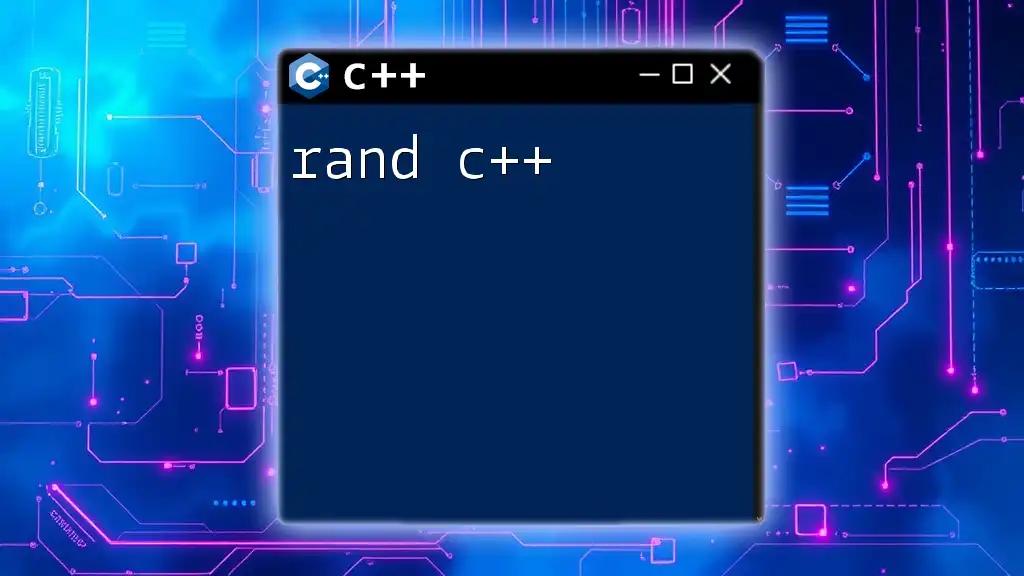
Best Practices for Array Indexing in C++
Avoiding Common Pitfalls
Mistakes like out-of-bounds access or using uninitialized arrays can lead to unpredictable behavior. Always ensure that indices are within the valid range and that the arrays are initialized before use.
Performance Considerations
Array indexing involves time complexity of O(1) for access operations. However, for more complex operations like searching or sorting, it may vary, with linear search being O(n) while binary search is O(log n), provided the array is sorted. Proper indexing and efficient algorithms can significantly enhance program performance.
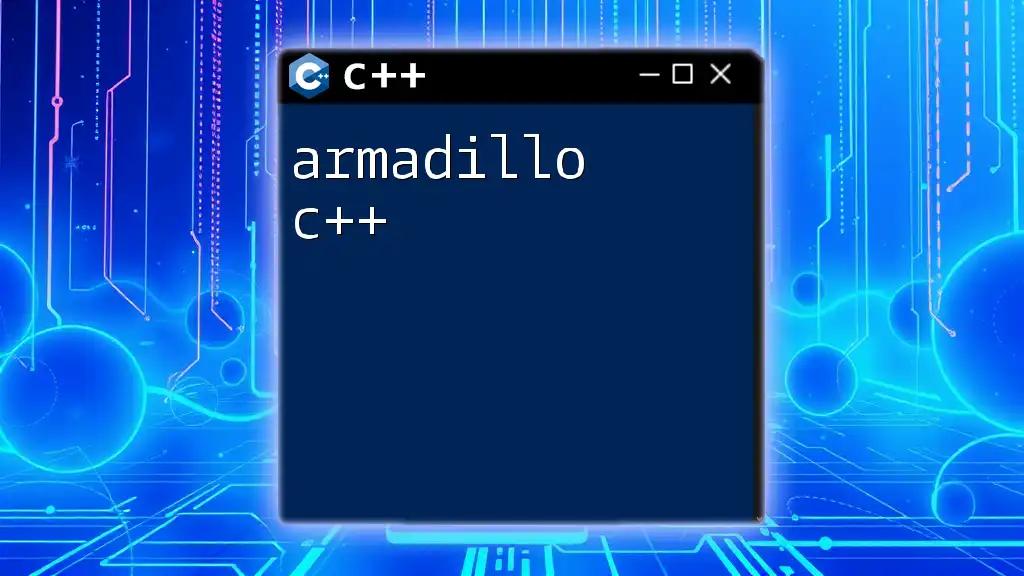
Conclusion
Understanding array index C++ and its implications is crucial for effective programming. With a grasp of array types, indexing rules, and practical techniques, you can write more efficient code. Remember, practicing these concepts through coding exercises will strengthen your skills and confidence in using arrays effectively.

Additional Resources
Consider exploring books, online courses, and coding platforms that dive deeper into C++ and array handling. These resources can provide more context and practical examples that reinforce your learning.
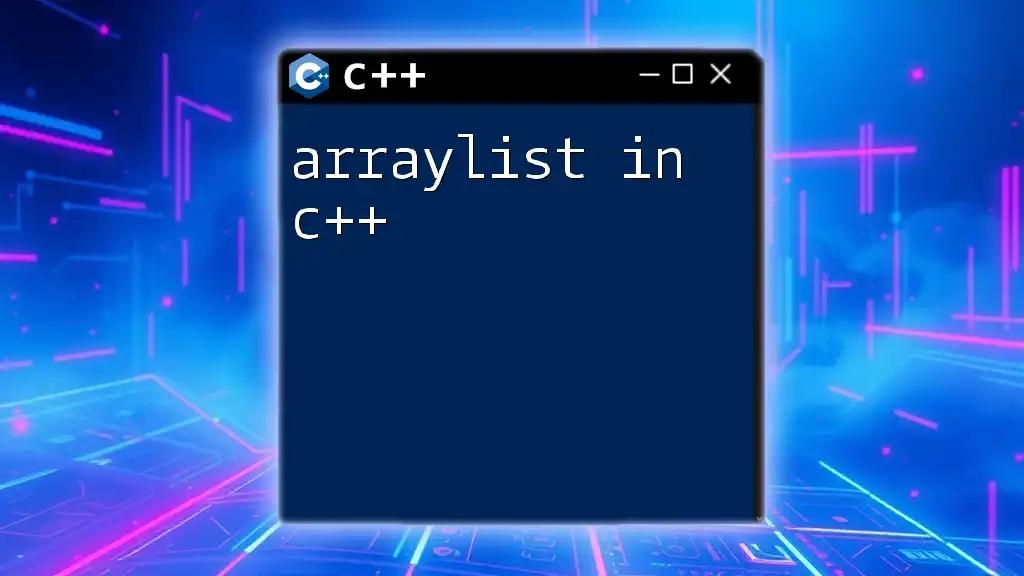
Call to Action
Don't hesitate to begin experimenting with array indexing in your C++ projects. Whether you are building a simple application or a complex system, mastering these concepts will be invaluable. Join our courses to delve deeper into C++ and enhance your programming skills!