Smart pointers in C++ are objects that manage the lifetime of dynamically allocated memory, ensuring proper resource deallocation and preventing memory leaks.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr = std::make_unique<int>(42);
std::cout << *ptr << std::endl; // Outputs: 42
return 0;
}
Understanding the Basics of Smart Pointers
What are Smart Pointers?
Smart pointers are essential constructs in C++ that encapsulate raw pointers to manage memory more effectively and safely. Unlike raw pointers, smart pointers automatically deallocate memory once they go out of scope or are no longer needed, thereby reducing the chances of memory leaks and undefined behavior.
Using smart pointers means having a more robust memory management strategy, enabling developers to focus on their application logic rather than the intricacies of manual memory management.
Types of Smart Pointers in C++
C++ provides several types of smart pointers, each serving different purposes. The three primary types are `std::unique_ptr`, `std::shared_ptr`, and `std::weak_ptr`.
Unique Pointer (std::unique_ptr)
The `std::unique_ptr` provides exclusive ownership of the pointed object. This means that only one unique pointer can point to a given memory resource at any time. If the unique pointer goes out of scope, its destructor is called, and thus it automatically frees the associated memory.
Key Characteristics:
- Ownership Semantics: A unique pointer cannot be copied. Ownership can only be transferred through move semantics.
- Memory Efficiency: Ideal for managing resources that do not require shared ownership.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> p1(new int(10));
std::cout << *p1 << std::endl; // Outputs: 10
// std::unique_ptr<int> p2 = p1; // Error: cannot copy
std::unique_ptr<int> p2 = std::move(p1); // Transfer ownership
std::cout << *p2 << std::endl; // Outputs: 10
return 0;
}
Shared Pointer (std::shared_ptr)
`std::shared_ptr` allows multiple pointers to share ownership of a single object. The underlying object is destroyed only when the last owner (shared pointer) is destroyed or reset. This feature is particularly useful in scenarios where multiple objects need to access the same resource.
How Shared Ownership Works:
- Each `std::shared_ptr` maintains a reference count that tracks how many shared pointers point to the same object.
#include <iostream>
#include <memory>
void show_count(std::shared_ptr<int> ptr) {
std::cout << "Reference count: " << ptr.use_count() << std::endl;
}
int main() {
std::shared_ptr<int> p1(new int(20));
show_count(p1); // Reference count: 1
std::shared_ptr<int> p2 = p1;
show_count(p1); // Reference count: 2
return 0;
}
Weak Pointer (std::weak_ptr)
A `std::weak_ptr` is a companion to `std::shared_ptr`. It allows access to an object that is managed by `std::shared_ptr` without increasing the reference count. This means that a weak pointer can be used to observe or check an object without affecting its lifetime, thereby helping to prevent circular references and memory leaks.
Use Cases:
- When you want to monitor an object without influencing its lifespan.
#include <iostream>
#include <memory>
class Node {
public:
int value;
std::shared_ptr<Node> next;
Node(int val) : value(val) {}
};
int main() {
std::shared_ptr<Node> n1 = std::make_shared<Node>(1);
std::weak_ptr<Node> n2 = n1; // Create weak_ptr from shared_ptr
if (auto sp = n2.lock()) { // Check if shared_ptr is valid
std::cout << sp->value << std::endl; // Outputs: 1
}
return 0;
}

Comparing Smart Pointers in C++
Smart Pointer vs. Raw Pointer
When comparing smart pointers to raw pointers, the distinctive advantage lies in automatic memory management. Smart pointers automatically release memory when they go out of scope, thereby minimizing the risk of memory leaks and dangling pointers, which are common pitfalls with raw pointers.
In contrast, raw pointers require manual deallocation, which increases the likelihood of human error and leads to unsafe programming.
When to Use Each Type:
- Raw pointers: Suitable for simple scenarios where ownership management isn't necessary.
- Smart pointers: Ideal for complex applications that require efficient and safe memory management.
Smart Pointers vs. Standard Containers
While smart pointers manage the lifetime of individual resources, standard containers (like `std::vector`, `std::list`, etc.) manage collections of resources. Understanding when to use smart pointers in conjunction with standard containers can lead to cleaner and more maintainable code.
For example, a `std::vector<std::unique_ptr<T>>` can manage a collection of dynamically allocated objects where each object has a single owner, while `std::vector<std::shared_ptr<T>>` can manage objects shared among multiple owners.

Best Practices with Smart Pointers
When to Use Smart Pointers
Choosing the right smart pointer is crucial for proper memory management. Here are some guidelines:
- Use `std::unique_ptr` when ownership is exclusive—when only one entity should manage the resource's lifetime.
- Use `std::shared_ptr` when multiple entities need access to the same resource, ensuring the resource's lifecycle is managed through reference counting.
- Use `std::weak_ptr` to break circular references in shared ownership scenarios.
Common Pitfalls to Avoid
Despite their advantages, smart pointers can introduce pitfalls if not used correctly:
- Overusing `shared_ptr` can lead to memory bloat due to reference counting overhead.
- Forgetting to manage cyclic dependencies can lead to memory leaks, even with smart pointers.
- Misusing `weak_ptr` can cause dangling references if not properly checked.

Performance Considerations
Efficiency of Smart Pointers
Smart pointers provide a safety net for memory management, but they come with performance trade-offs. Generally, the overhead associated with smart pointers is minimal, though shared pointers incur the additional cost of maintaining a reference count.
In performance-critical applications, it is essential to balance safety with speed. When performance is paramount, using raw pointers in controlled scenarios might be appropriate, but with the risk of manual memory management.
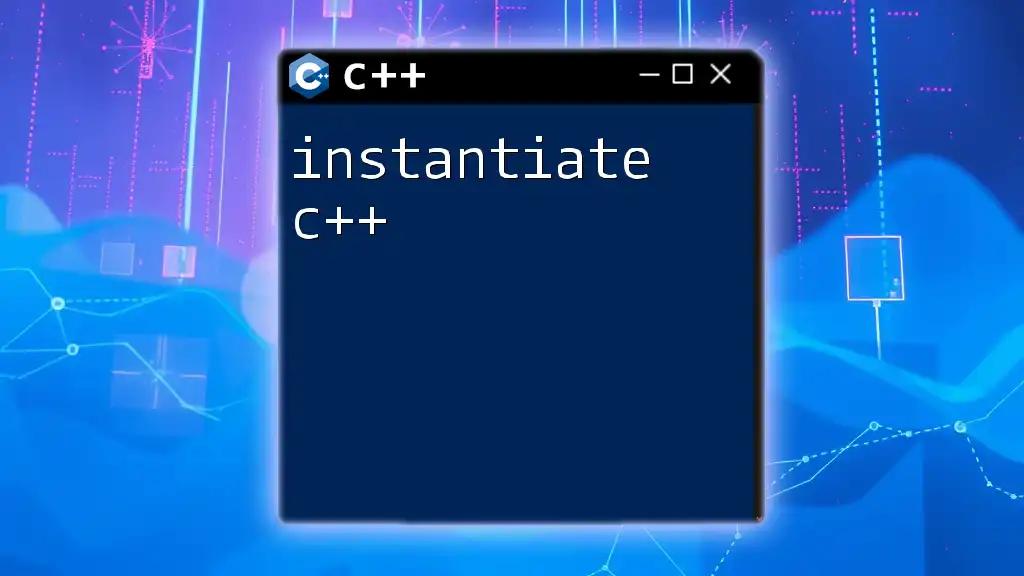
Conclusion
Smart pointers in C++ are pivotal for modern memory management, offering robust solutions to handling dynamic resources without the usual pitfalls associated with raw pointers. Adopting best practices around smart pointers not only enhances program safety but also results in cleaner and more maintainable code structures. By understanding when and how to use smart pointers, developers can leverage their full potential in programming.

Additional Resources
For further exploration of smart pointers in C++, consider looking into books specifically focused on modern C++ practices, as well as reputable online platforms that offer tutorials and courses dedicated to mastering smart memory management techniques.