In C++, the `map::insert` function allows you to add key-value pairs to a map, ensuring that the keys are unique.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap.insert(std::make_pair(1, "Apple")); // Inserting a key-value pair
myMap.insert({2, "Banana"}); // Another way to insert
return 0;
}
Understanding C++ Maps
Maps are a vital component of C++ programming, designed to store key-value pairs. Each key is unique, allowing efficient access to its corresponding value. The C++ Standard Library provides the `map` container, which organizes data that can be accessed via keys.
What is a C++ Map?
A map is an associative container that contains elements in the form of key-value pairs. It allows fast retrieval of values based on their keys, making it an excellent choice when you need to manage paired data.
Maps not only maintain the pairing of keys and values, but they also ensure that the keys are sorted. This unique feature allows you to perform various operations quickly, such as searching, inserting, and deleting elements.
Syntax of C++ Map
Declaring a map in C++ is straightforward. The general syntax looks like this:
std::map<KeyType, ValueType> myMap;
- KeyType is the data type of the keys.
- ValueType is the data type of the values.
For example:
std::map<std::string, int> fruitCount;
This declares a map where the keys are of type `std::string` (fruit names) and values are of type `int` (the quantity of each fruit).
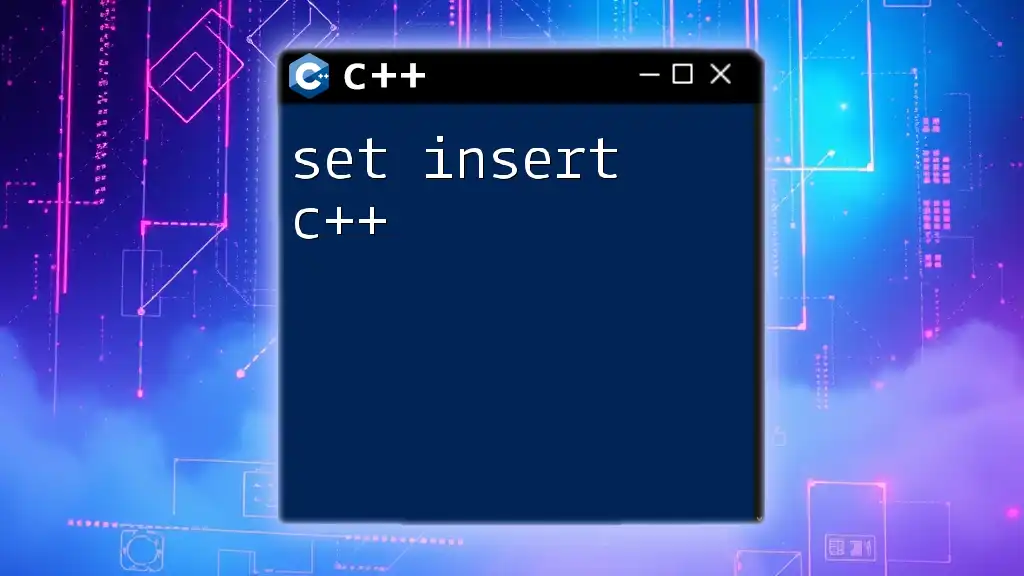
The `insert` Function in C++
What is `insert`?
The `insert` function is crucial for adding new key-value pairs to a map. Utilizing this function allows for the dynamic growth of the map as you add elements. It’s important to note that maps do not allow duplicate keys; if you attempt to insert a key that already exists, the insertion will not occur.
Syntax of `insert`
The basic syntax for using the `insert` function is as follows:
myMap.insert(std::make_pair(key, value));
This pairs a specified key with a value, effectively adding it to the map.
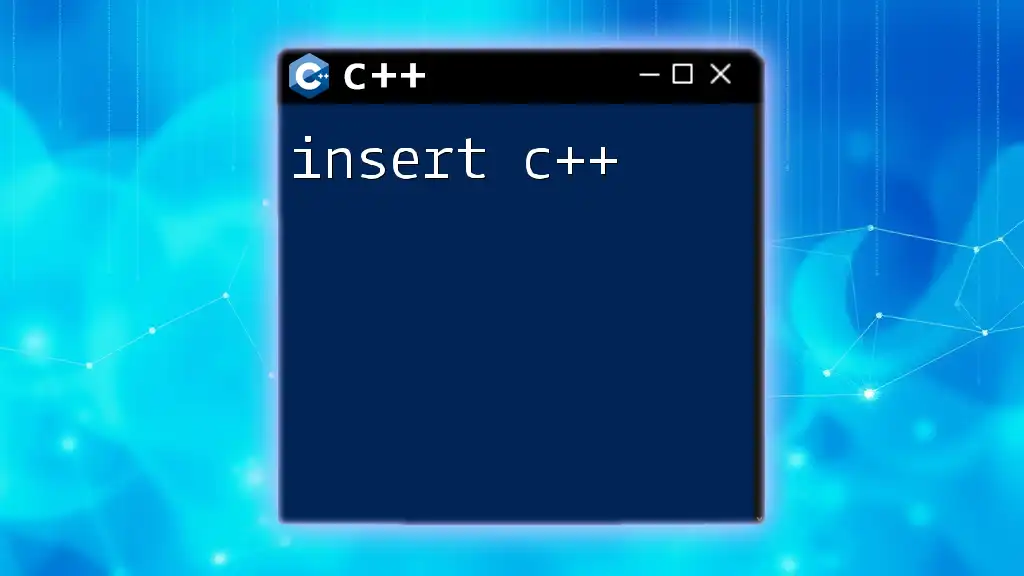
Different Ways to Insert Elements in a Map
C++ provides several methods for inserting elements into a map, each with its unique benefits.
Using `std::make_pair`
You can easily create and insert a key-value pair using `std::make_pair`. Here’s an example:
myMap.insert(std::make_pair("apple", 1));
Using `make_pair` is beneficial since it efficiently constructs a pair, making the syntax cleaner and less prone to errors.
Using `std::pair`
Another method for insertion utilizes `std::pair`, which can be slightly more verbose but offers flexibility in key and value types:
myMap.insert(std::pair<std::string, int>("banana", 2));
This explicitly specifies the types of the key and value, providing clarity in the code.
Using the `[]` Operator
For insertion, the `[]` operator can also be used, which automatically initializes the value for a given key if it doesn’t already exist. Here’s an example:
myMap["cherry"] = 3;
This method is quite convenient; however, it’s worth noting that if the key already exists, this operator will overwrite the current value.
Using `insert` with Initializer List
You can insert multiple key-value pairs simultaneously through an initializer list, which improves code readability:
myMap.insert({{"date", 4}, {"elderberry", 5}});
This concise syntax allows you to add several pairs in one go, enhancing efficiency.
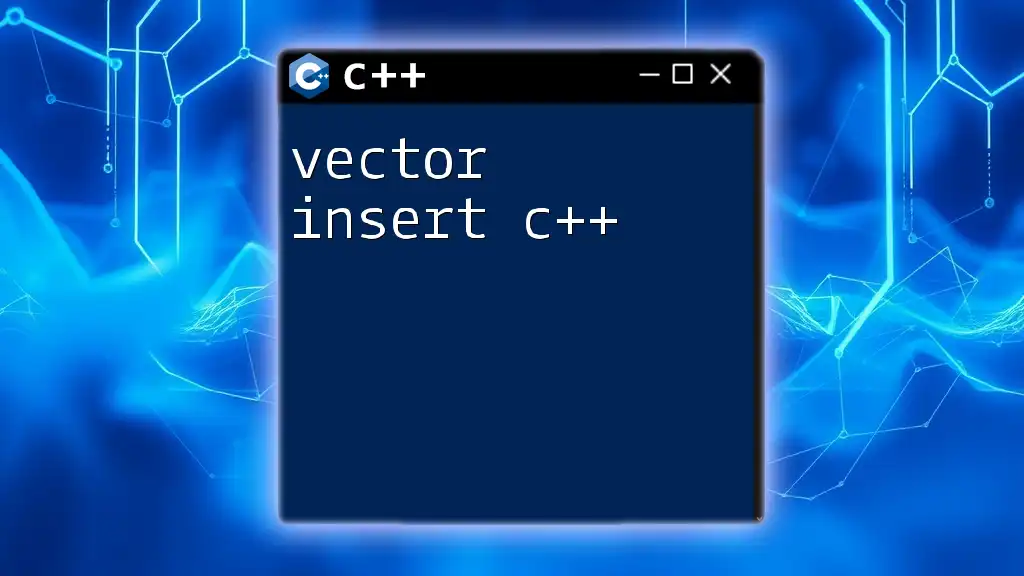
Handling Duplicate Keys
How Maps Handle Duplicates
An essential characteristic of maps is that they do not allow duplicate keys. If you attempt to insert a key that is already present, the map remains unchanged. Consider this example:
myMap.insert(std::make_pair("apple", 10)); // This will not insert because "apple" already exists.
This property is what helps maintain the integrity of the data structure.
Checking for Key Existence Before Insertion
To avoid attempting to insert a duplicate key, you can first check if the key exists using the `find` method:
if (myMap.find("apple") == myMap.end()) {
myMap.insert({"apple", 1});
}
By leveraging this approach, you can ensure that your insertions are valid and do not overwrite existing data.
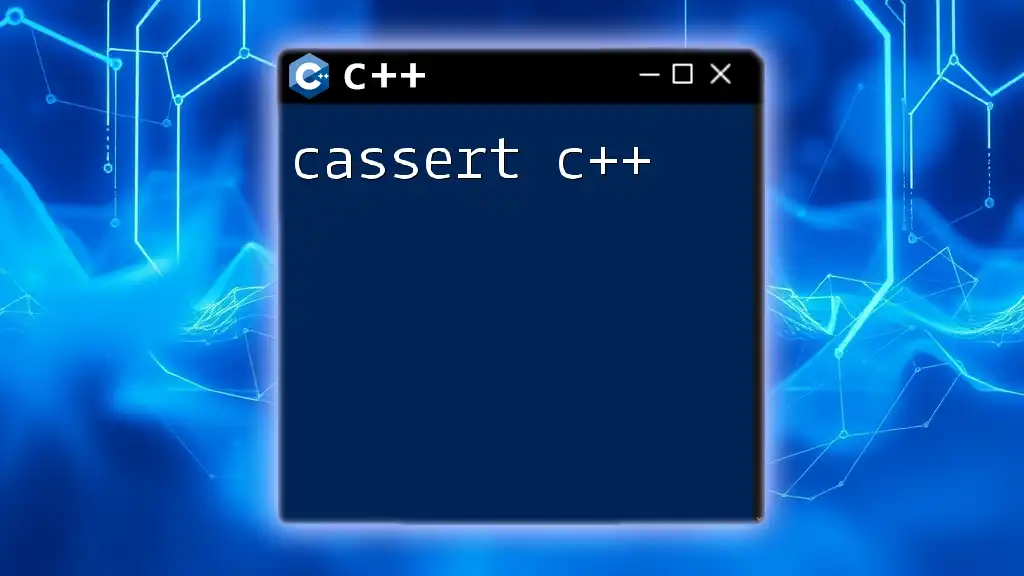
Common Errors and Best Practices
Avoiding Common Mistakes
One common mistake when using `insert` is assuming it updates values for existing keys. Remember that `insert` will not modify an existing pair; it simply will not insert if the key already exists. This often leads to unexpected results, so it’s crucial to double-check whether a key is present before inserting.
Best Practices for Using Maps
To optimize the efficiency of your C++ maps, consider the following best practices:
- Choose the Right Data Types: Select data types for keys and values that suit the application and minimize memory usage.
- Preallocate Space: If you know the number of elements to be inserted, consider using `reserve()` to preallocate memory. This can prevent multiple memory allocations as the map grows.
- Utilize Iterators: For performance-sensitive applications, use iterators to traverse a map efficiently.
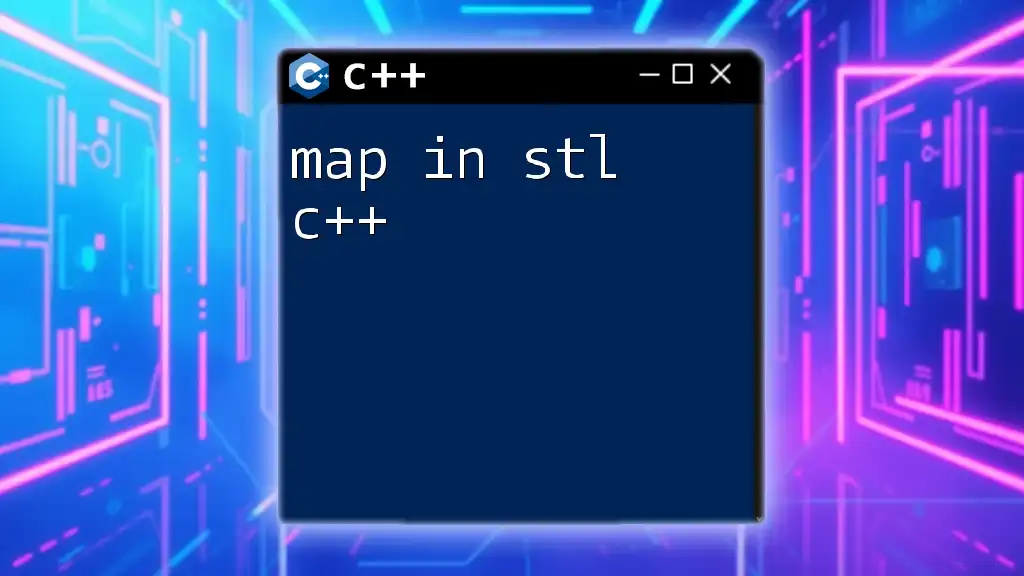
Conclusion
Inserting elements into a C++ map using the `insert` function is a fundamental skill for any programmer who wishes to effectively manage key-value data. By mastering various insertion techniques and understanding how to handle duplicates, you can leverage the full potential of C++ maps in your projects. Experiment with the examples provided and consider the best practices to enhance your programming efficiency and accuracy in working with maps.
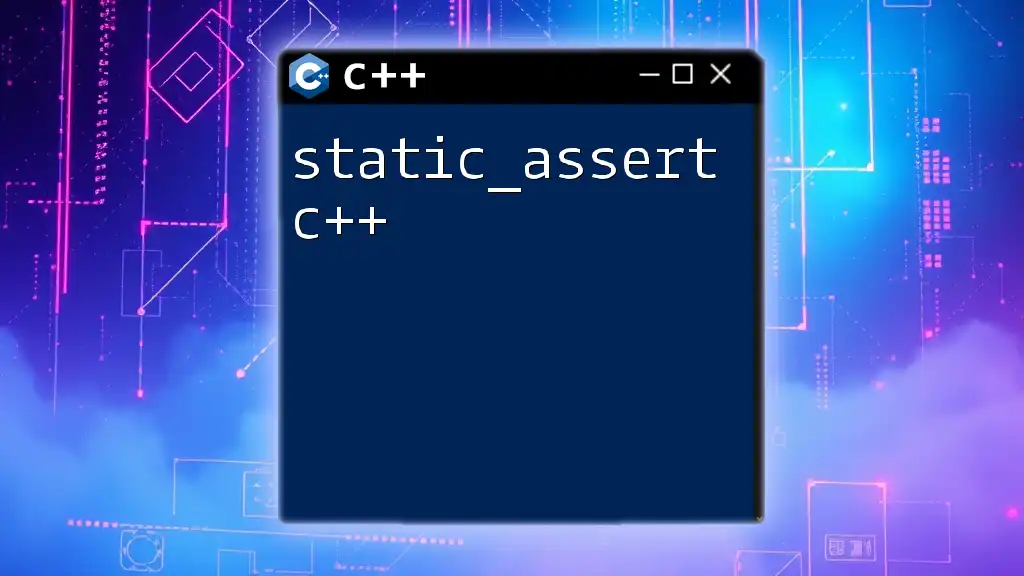
Additional Resources
For further reading, refer to the official C++ documentation regarding the `map` container and its associated functions. This will deepen your understanding and help you explore other advanced use cases.
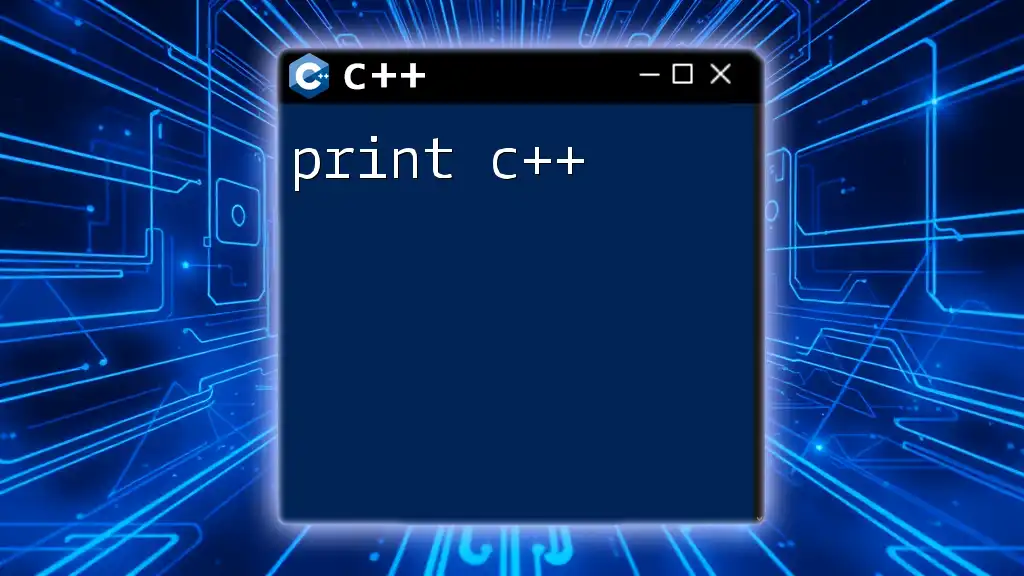
Code Snippet Collection
Here are some practical examples you can refer to for a quick recap:
-
Simple Inventory System:
std::map<std::string, int> inventory; inventory.insert(std::make_pair("sword", 10)); inventory["shield"] = 5;
-
Counting Occurrences of Characters in a String:
std::map<char, int> charCount; std::string text = "hello"; for (char c : text) { charCount[c]++; }
By using these examples, you can grasp the versatility of map insertions and their practical applications in real-world scenarios.