In C++, you can display output to the console using the `std::cout` stream along with the insertion operator (`<<`).
Here's a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Basics of Output in C++
What is Output in Programming?
In programming, output refers to the data or information that is produced by the program. Output serves a crucial role, as it allows programmers and end-users to see the results of executed commands or functions. Whether displaying results for calculations, informing users of application status, or debugging, output is an indispensable facet of programming.
Understanding Standard Output
In C++, the standard output device is typically the console or terminal window. This is where texts and results from the program are displayed. C++ uses the `iostream` library, which provides functionalities for input and output operations. Before working with output in C++, you need to include the `iostream` header file to access these features.
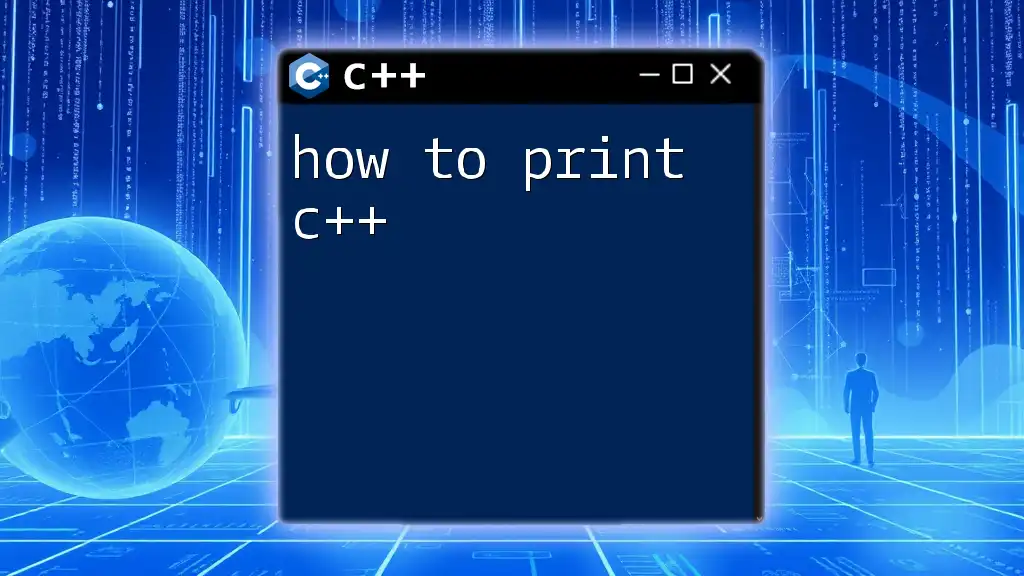
Using `cout` for Output
What is `cout`?
The `cout` (short for "character output") is an object of the `ostream` class used to output data to the standard output stream (usually the console). It is a fundamental component for anyone looking to print in C++. To use `cout`, you typically need to refer to the `std` namespace.
Syntax of `cout`
To perform output with `cout`, the syntax is straightforward. You utilize `<<` (the insertion operator) to direct the output to the console. Here’s a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `Hello, World!` is printed to the console. The `std::endl` manipulator is used to insert a new line after the output.
Combining Output with Operators
The ability to combine different types of data in a single output statement is incredibly powerful. With `cout`, you can seamlessly concatenate strings and other types of variables:
int age = 25;
std::cout << "I am " << age << " years old." << std::endl;
This prints: I am 25 years old. This illustrates the versatility of `cout`, allowing different data types to be included in the output.
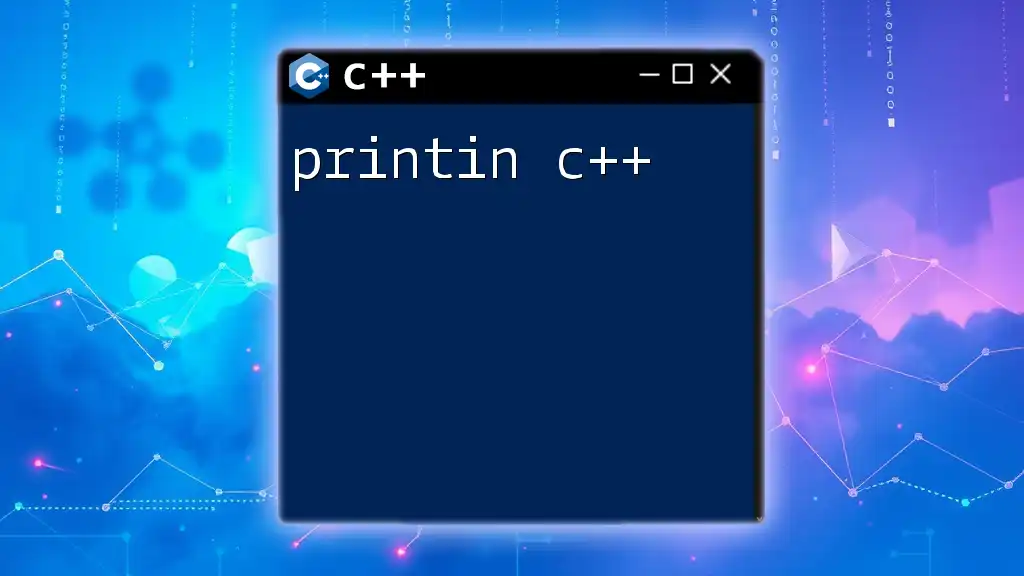
Formatting Output
Using Manipulators
Formatters, or manipulators, are key to presenting your output in a clean and professional manner. Commonly used manipulators include `std::endl`, `std::setw` (to set width), and `std::fixed` (to control floating-point format). For example:
#include <iomanip>
#include <iostream>
int main() {
std::cout << std::setw(10) << "Item" << std::setw(10) << "Price" << std::endl;
return 0;
}
In the code above, the `setw` manipulator sets the width of the output fields, ensuring that they are aligned nicely in columns.
Controlling Output Precision
When dealing with floating-point numbers, precision control is vital. C++ provides the manipulators `std::fixed` and `std::setprecision` to achieve this. Here’s how it can be done:
#include <iomanip>
#include <iostream>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl; // Outputs: 3.14
return 0;
}
In this example, `pi` is printed with two decimal places, showcasing control over formatting.
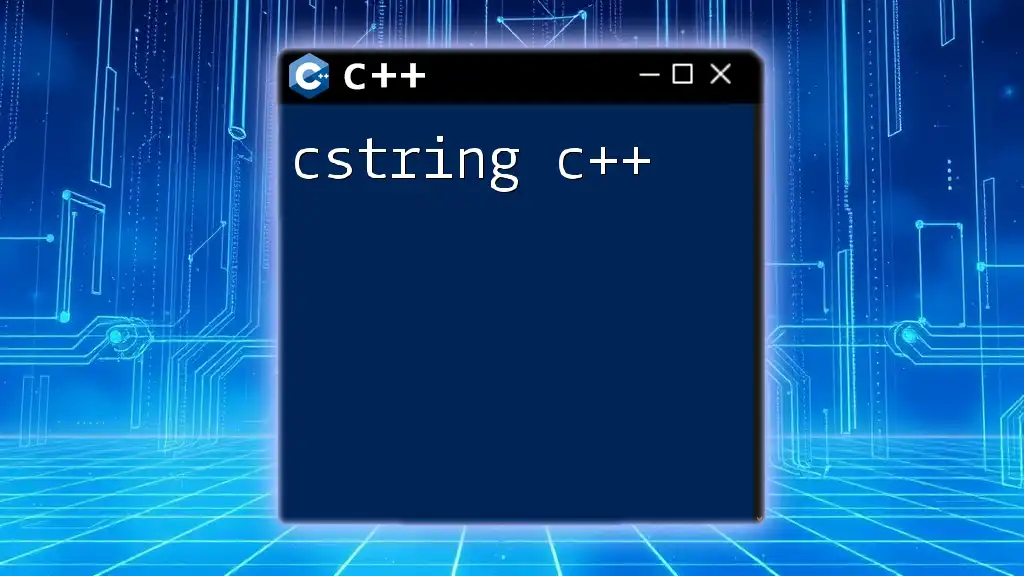
Advanced Output Techniques
Outputting Various Data Types
One of the remarkable features of `cout` is its adaptability in accepting various data types. This includes integers, characters, strings, and even more complex types like objects. Here’s an example featuring different data types:
int integerVar = 42;
char charVar = 'A';
std::cout << integerVar << " " << charVar << std::endl; // Outputs: 42 A
This showcases how easily C++ handles multiple data types using `cout`.
Handling Strings with `cout`
When it comes to string output, C++ offers different options, such as C-style strings (using `const char*`) and standard strings (using the `std::string` class). Both can be printed with `cout`. Consider the following:
#include <iostream>
#include <string>
int main() {
std::string message = "Hello, C++!";
std::cout << message << std::endl;
return 0;
}
This will print: Hello, C++! Using `std::string` makes string manipulation more accessible and safer in C++.
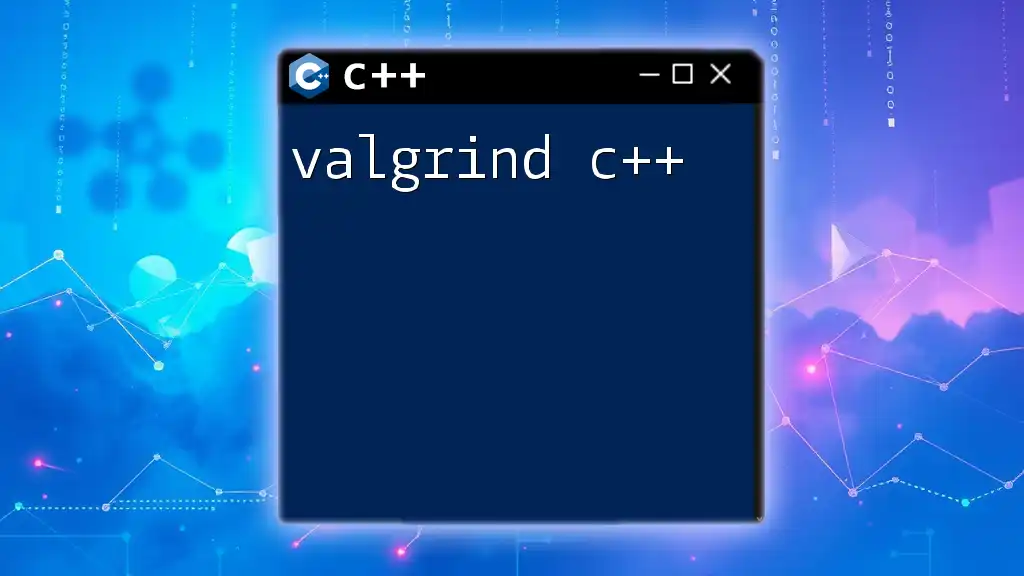
Error Handling in Output
Using `cerr` for Error Messages
In scenarios where error output is required, C++ provides `cerr` for this purpose. The `cerr` is unbuffered, making it suitable for immediate error messages:
std::cerr << "This is an error message." << std::endl;
This command sends an error message to the standard error stream, allowing for an instant alert to the user or developer.
Difference Between `cout`, `cerr`, and `clog`
It’s important to understand the nuances between `cout`, `cerr`, and `clog`. While `cout` sends output to the standard output stream, `cerr` is aimed at error messages, and it typically bypasses buffering, ensuring a timely display. Meanwhile, `clog` sends messages to the standard logging stream, but it is buffered. It can be used for logging information that may not require instant visibility.
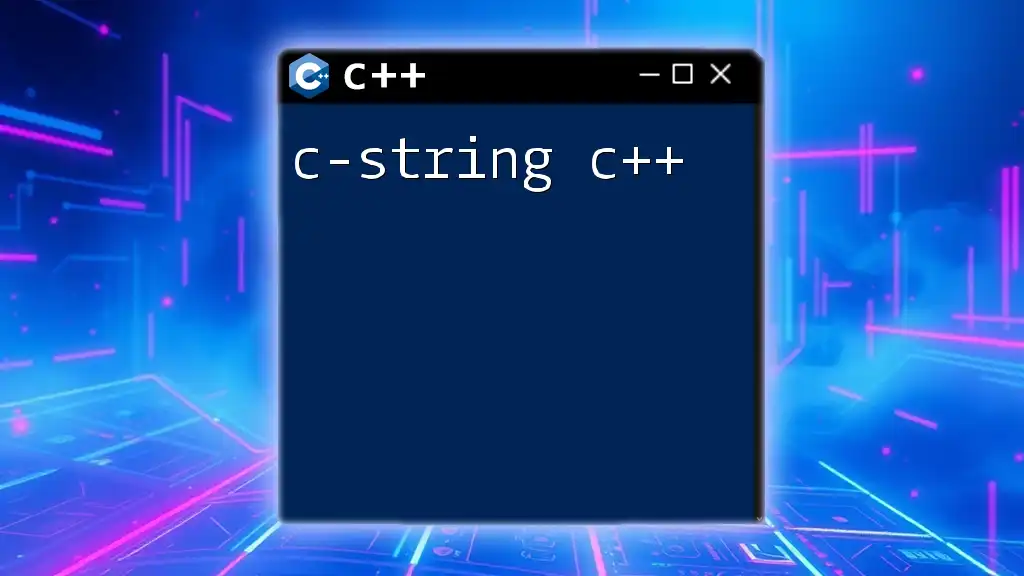
Creating Custom Output Functions
Why Create Custom Functions?
Writing custom output functions can enhance code reusability and clarity. This is particularly useful when you frequently print similar lines or formats throughout your program or when specific output actions need to be centralized.
Example of a Custom Output Function
Here’s an example of a simple custom output function that standardizes output:
#include <iostream>
#include <string>
void printMessage(const std::string& msg) {
std::cout << msg << std::endl;
}
int main() {
printMessage("Custom output message.");
return 0;
}
In this code, `printMessage` is a reusable function that takes a string and prints it. This encapsulation fosters cleaner code and easier maintenance.
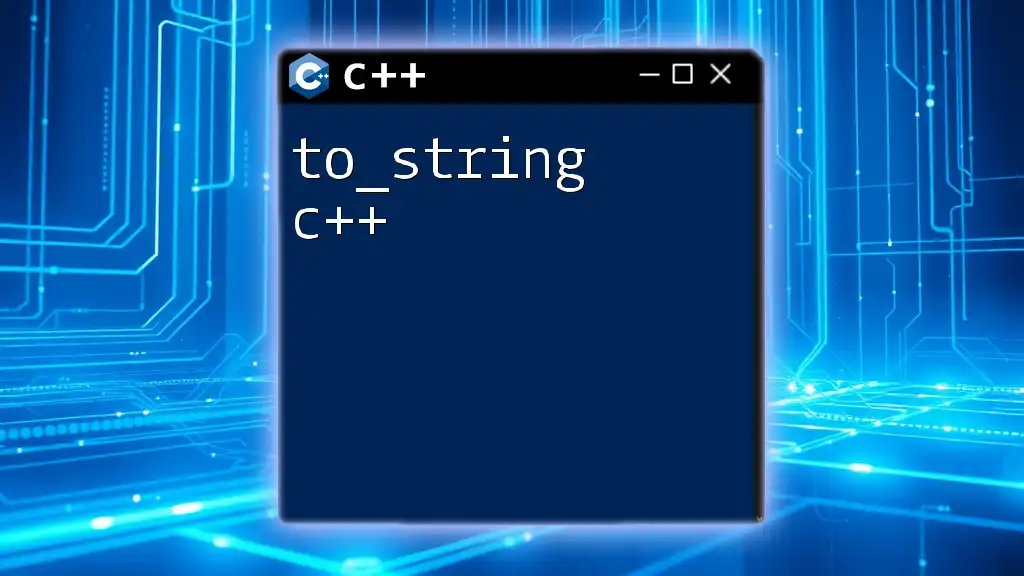
Conclusion
Understanding how to print in C++ is foundational for effective programming. From using `cout` to handle various data types to formatting outputs and creating custom output functions, mastering these techniques is essential for any C++ programmer. The methods discussed here will enhance your coding experience and allow you to create clearer, more professional outputs. Dive into practice, explore these examples, and expand your C++ skills with confidence!
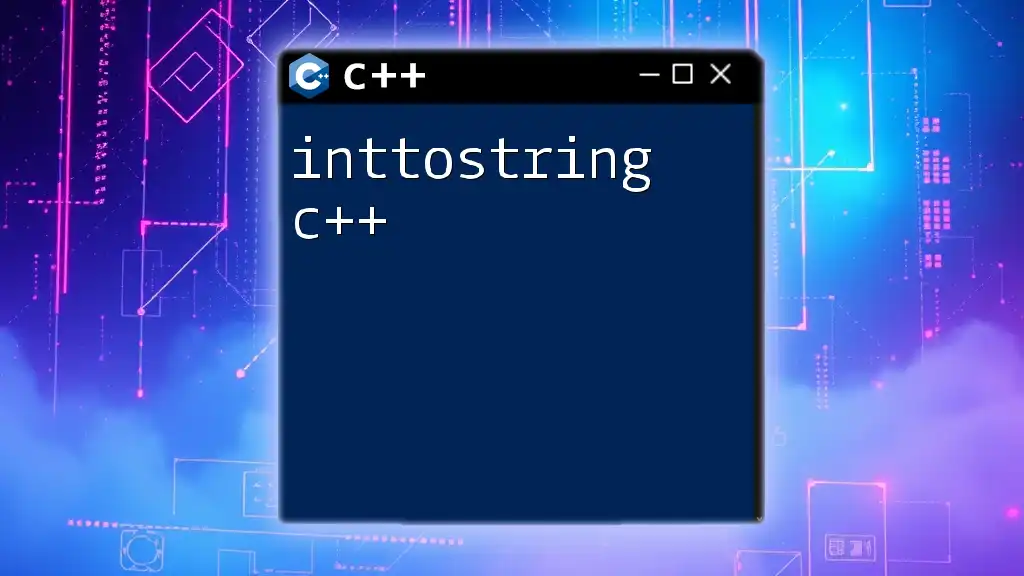
Additional Resources
For further exploration, consider diving into C++ documentation, online tutorials, and forums that delve deeper into advanced output techniques and best practices. Your journey in mastering C++ continues beyond just printing; it opens the doors to comprehensive programming skills.