In C++, the `exp` function from the `<cmath>` library calculates the exponential value of a given number, returning \( e \) raised to the power of that number. Here's a code snippet illustrating its usage:
#include <iostream>
#include <cmath>
int main() {
double number = 2.0;
double result = exp(number);
std::cout << "e^" << number << " = " << result << std::endl;
return 0;
}
Understanding the Exponential Function in C++
What is the Exponential Function?
The exponential function is a mathematical function denoted as \( e^x \), where \( e \) is the base of the natural logarithm, approximately equal to 2.71828. This function describes growth processes in various capacities, including population growth, compound interest, and radioactive decay. Its significance in mathematics stems from its unique properties:
- The derivative of \( e^x \) is itself, making it a fundamental function in calculus.
- It appears in numerous equations across fields such as physics, biology, and economics.
The Role of Exponential Functions in Programming
In programming, exponential functions are utilized for multiple purposes. They can help model real-world phenomena, solve equations, and implement algorithms that involve growth or decay rates. Understanding how to use the `exp` function effectively allows programmers to perform calculations that involve exponentiation without needing to implement complex algorithms from scratch.
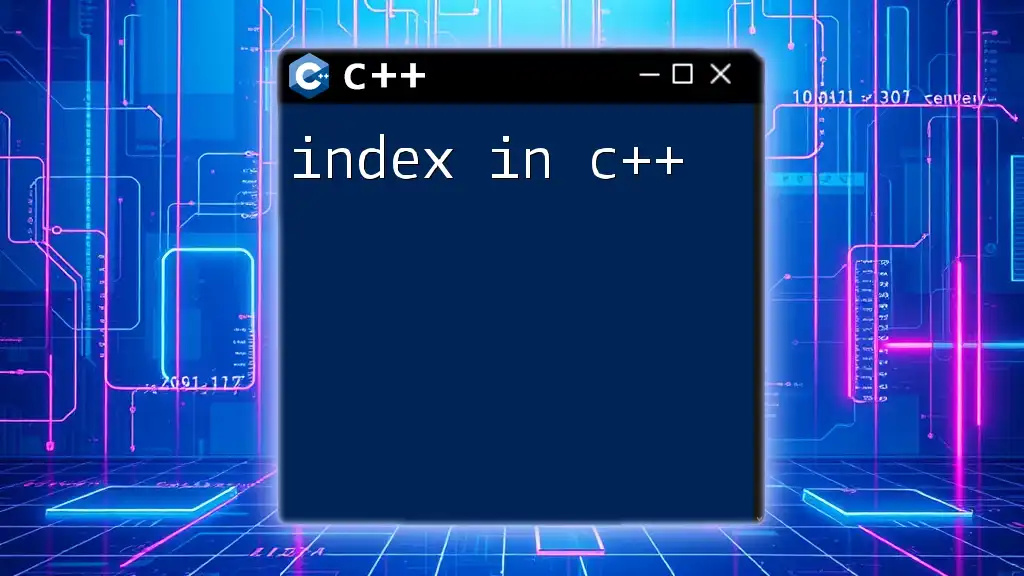
Introduction to the C++ exp Function
Overview of the C++ exp Function
The C++ `exp` function is an essential part of the C++ Standard Library, specifically included in the `<cmath>` header file. It calculates the value of \( e \) raised to the power of a specified number. Understanding this function allows you to perform rapid calculations involving exponential growth with ease.
Syntax of the C++ exp Function
The syntax for the `exp` function is simple and concise:
double exp(double x);
- Parameters:
- `x`: The exponent to which \( e \) is raised. It can be positive, negative, or zero.
- Return Type:
- This function returns a double representing \( e^x \).
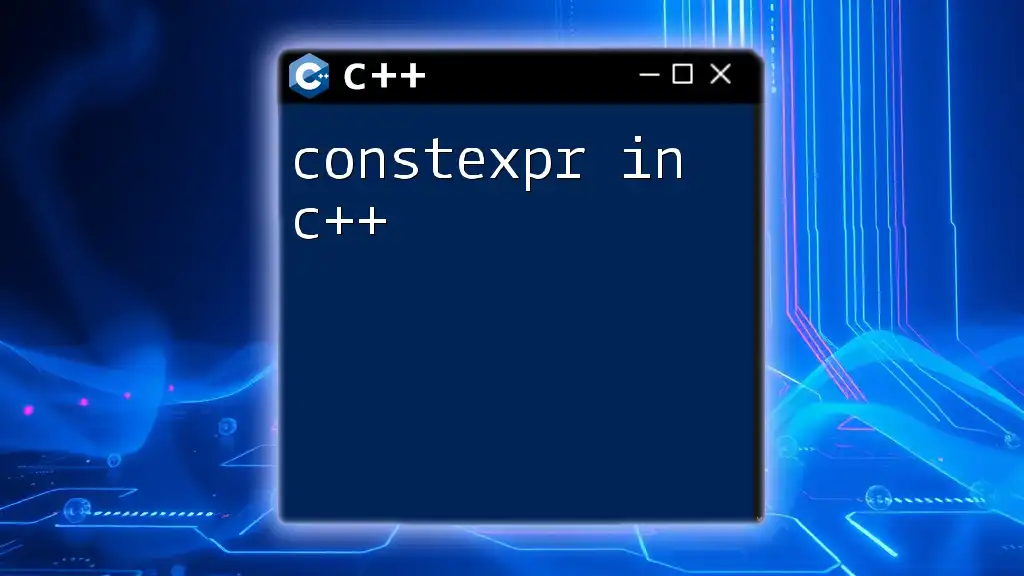
Using the C++ exp Function
How to Implement the exp Function
To demonstrate the usage of the `exp` function, consider the following basic example, where we calculate the exponential of a number.
#include <iostream>
#include <cmath>
int main() {
double value = 1.0;
double result = exp(value);
std::cout << "exp(" << value << ") = " << result << std::endl; // Output: exp(1) = 2.71828
return 0;
}
In this example, we include the necessary headers and declare a `value` of 1.0. The `exp` function is called, and the result is printed to the console. The output illustrates how the function calculates \( e^1 \) or \( e \) itself, which is approximately 2.71828.
Practical Applications of exp in C++
Exponential functions have vital applications across various fields, including finance and biology.
Graphing Exponential Growth
Graphing exponential functions can be powerful in visualizing growth behaviors. Implementing the `exp` function can aid in creating graphs to represent data.
Modeling Real-World Scenarios
Consider the application of compound interest, which can be illustrated through the use of the `exp` function as follows:
#include <iostream>
#include <cmath>
int main() {
double time = 5.0; // in years
double rate = 0.05; // 5% interest rate
double principal = 1000.0; // initial amount
double compoundInterest = principal * exp(rate * time);
std::cout << "Compound Interest after " << time << " years: " << compoundInterest << std::endl;
return 0;
}
In this situation, we calculate the compound interest earned on an initial investment using the formula \( A = P e^{rt} \). The `exp` function is used to compute \( e^{0.05 \times 5} \) which reflects the growth of the investment over time. The result showcases the potential returns of investing money at a given interest rate.
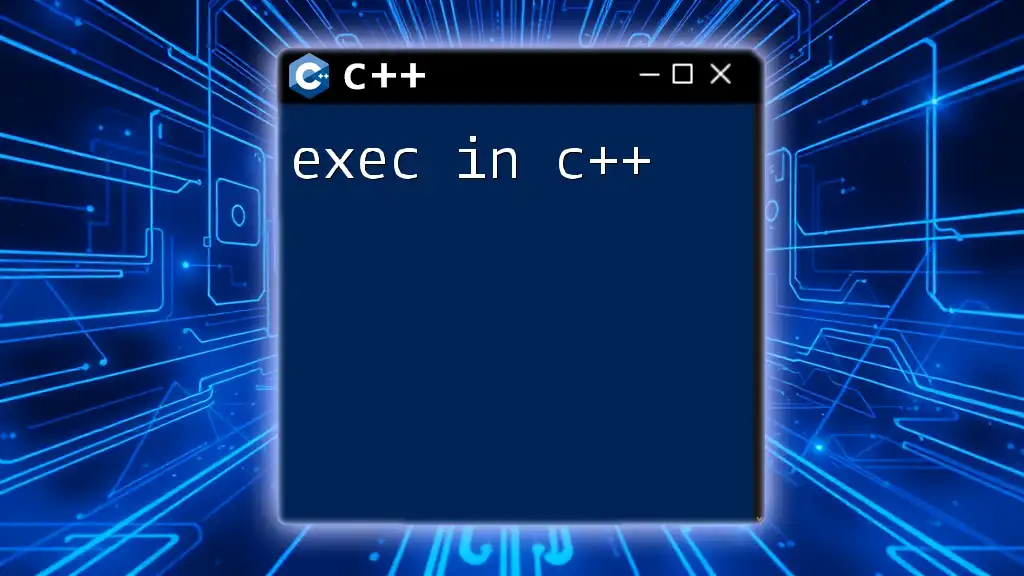
Detailed Explanation of the C++ Exp Function
How the C++ exp Function Handles Edge Cases
Understanding how the `exp` function behaves with different inputs is crucial.
- Negative Values: When passing a negative number, the `exp` function will provide results between 0 and 1. For example, `exp(-1)` equals approximately 0.36788.
- Zero: The value of `exp(0)` is always 1, reflecting the fundamental property of exponentiation.
- Large Values: Care should be taken when passing significantly large values, as the output can exceed the representable range of a double and lead to overflow errors.
Performance Considerations
The `exp` function is designed for efficiency. When used within performance-critical applications, it's invaluable to compare it against other exponentiation techniques. Typically, the `exp` function performs extremely well, but for extensive computations, consider profiling its performance within your specific use case.
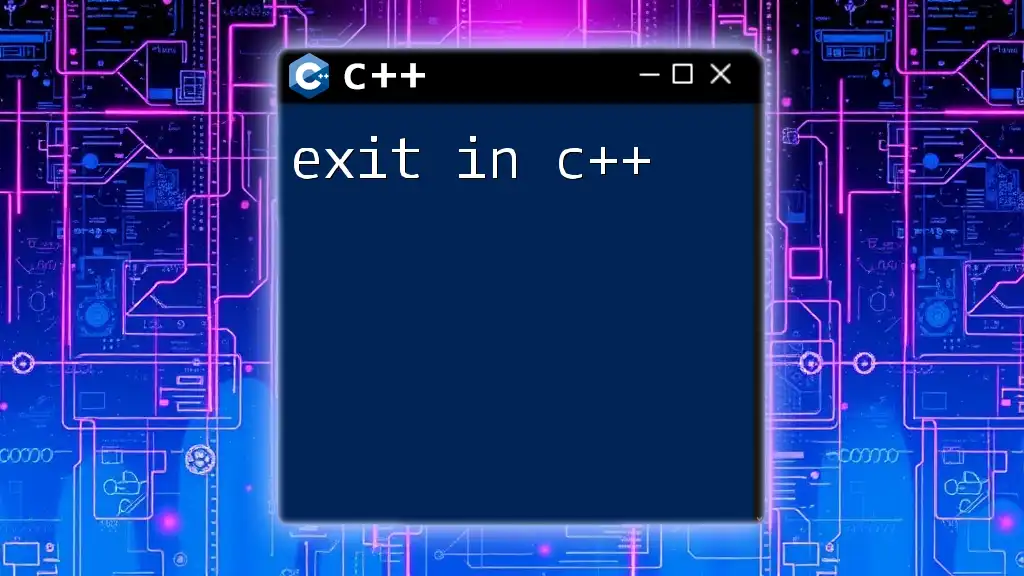
Troubleshooting Common Issues
Common Errors When Using the C++ exp Function
When using the `exp` function, errors can arise due to:
- Out-of-Range Errors: If you pass an excessively large value, it can lead to solutions that cannot be represented in the double data type.
- Incorrect Data Types: Ensuring the input is of type `double` is essential. Passing incorrect data types may result in compilation errors or incorrect behavior.
Debugging Tips and Techniques
When facing issues, utilizing logging methods can help trace the flow of data. In case of an error, consider implementing exception handling around the calls to the `exp` function to manage unexpected scenarios gracefully.
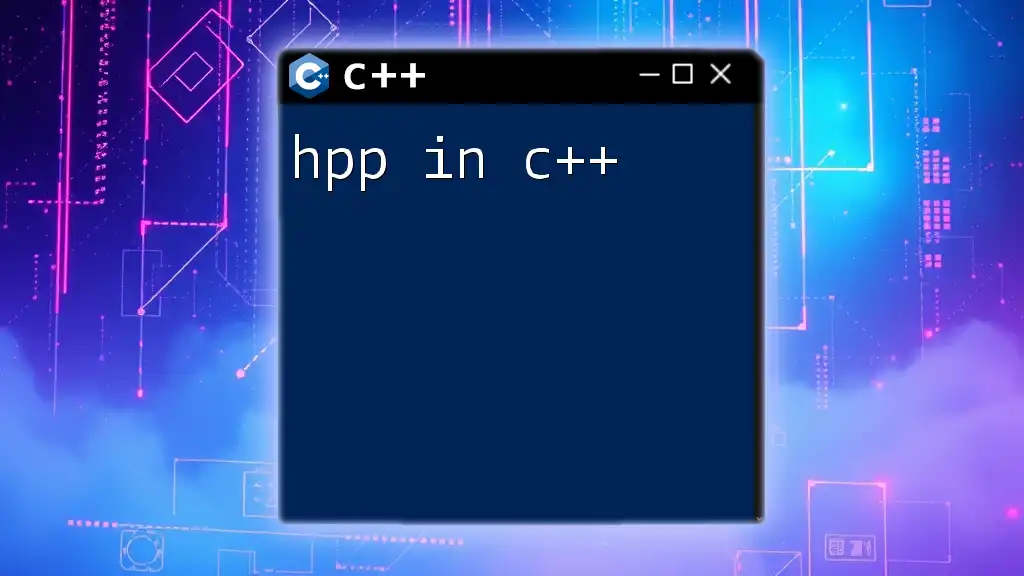
Conclusion
The Importance of Mastering the exp Function in C++
Mastering the `exp` function is crucial for anyone aiming to leverage mathematical concepts within programming, particularly in scientific or engineering contexts. It provides a concise way to handle calculations involving exponentiation while facilitating the modeling of real-world phenomena with simplicity.
Further Resources
Explore the C++ standard library documentation for more intricate details and advancements in mathematical functions. Engaging in practical exercises will further solidify your understanding.
Final Thoughts
As you continue your journey in learning C++ commands, remember that concise, practical implementations are key. Familiarizing yourself with functions such as `exp` will arm you with the capabilities to tackle complex programming tasks with greater efficiency and effectiveness.