`constexpr` in C++ is a keyword used to indicate that the value of a variable or the result of a function can be evaluated at compile time, enabling optimized performance and safer code.
constexpr int square(int x) {
return x * x;
}
constexpr int result = square(5); // result is evaluated at compile time
What is `constexpr`?
`constexpr` is a keyword in C++ that allows you to define functions and variables whose values can be computed at compile time. This capability enhances the performance of your programs by moving computations from runtime to compile time, resulting in faster execution. Historically introduced in C++11 and improved in subsequent standards, `constexpr` has become a fundamental feature for modern C++ programming.
C++ Standard Versions
- C++11: The original introduction of `constexpr` enabled simpler expressions and functions to be evaluated at compile time.
- C++14: Enhancements included the allowance for `constexpr` functions to contain more complex statements, making them more powerful while still being compiled at compile time.
- C++17: Further improvements allowed `if` and `for` statements within `constexpr` functions, greatly expanding functionality.
- C++20: Introduced even more capabilities, allowing mutable variables and lambdas to be `constexpr`, paving the way for more sophisticated compile-time computations.
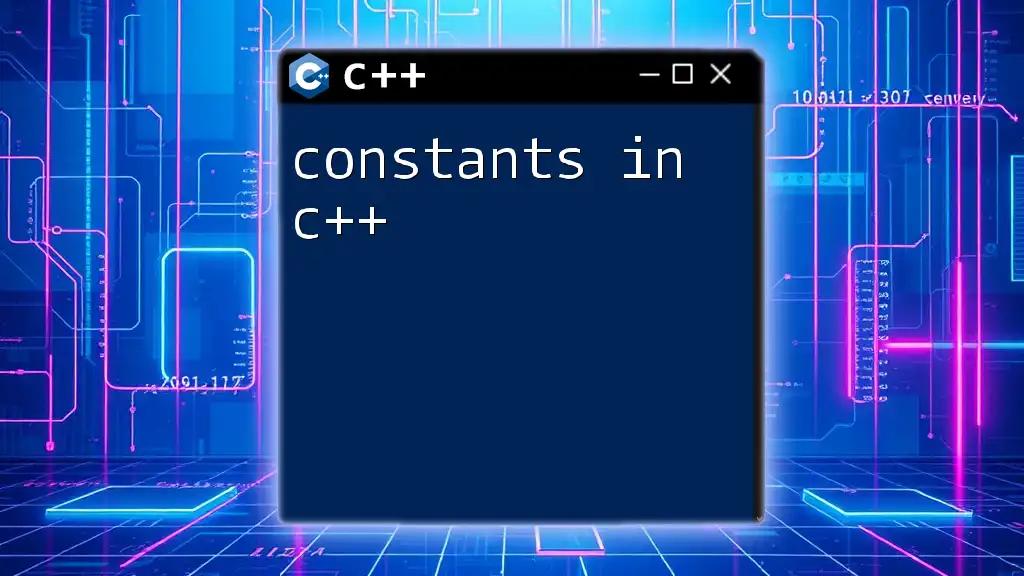
Benefits of Using `constexpr`
Using `constexpr in c++` opens up several advantages that optimize the performance and safety of your code.
Performance Optimization
The primary advantage of using `constexpr` is performance. Because values calculated within `constexpr` functions are evaluated at compile time, this reduces the runtime overhead. For example, using `constexpr` can speed up calculations typically performed in loops by pre-computing them.
Enhanced Code Clarity and Safety
Using `constexpr` can enhance code clarity. Functions declared as `constexpr` encourage the use of immutability, which can help prevent errors. Additionally, since these functions are checked at compile time, potential errors can be caught before they become runtime issues.
Improved Functionality
With `constexpr`, you can use functionality such as creating arrays or initializing data structures at compile time. This greatly simplifies certain tasks where constant data is often required.

Key Features of `constexpr`
Constant Expressions
A constant expression is an expression that can be evaluated at compile time. For example, a basic function can return a constant value multiplied by itself:
constexpr int square(int x) {
return x * x;
}
In this example, the `square` function can be evaluated during compilation if passed a constant argument.
Usage in Functions
Not all functions can be `constexpr`. For a function to be `constexpr`, it must comply with specific criteria—no dynamic memory allocation, no exceptions, and the body must consist solely of a return statement or a constexpr-compatible computation.
Consider this `constexpr` function for calculating the factorial of a number:
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
This recursive function adheres to the rules of `constexpr`, allowing it to compute values at compile time.
`constexpr` in Classes and Member Functions
Classes can also benefit from `constexpr`. Class members declared `constexpr` enable the use of initialization at compile time, leading to more efficient memory usage and instantiation. Check out the following example showing a `constexpr` constructor:
class Point {
public:
constexpr Point(double x, double y) : x_(x), y_(y) {}
constexpr double distance() const {
return sqrt(x_ * x_ + y_ * y_);
}
private:
double x_, y_;
};
In this example, the `Point` constructor is marked `constexpr`, allowing instances of `Point` to be created with compile-time arguments and facilitating efficient calculations.

Limitations of `constexpr`
While `constexpr` is powerful, it has its limitations. Certain operations and constructs are disallowed:
What You Cannot Do
Key restrictions include dynamic memory allocation and the use of try-catch blocks inside `constexpr` functions. Understanding these limitations is crucial for utilizing `constexpr` effectively.
`constexpr` in Recursive Functions
You can utilize recursion in `constexpr` functions, but it must be structured correctly. Recursive functions need a base case that references compile-time scenarios to prevent infinite recursion.
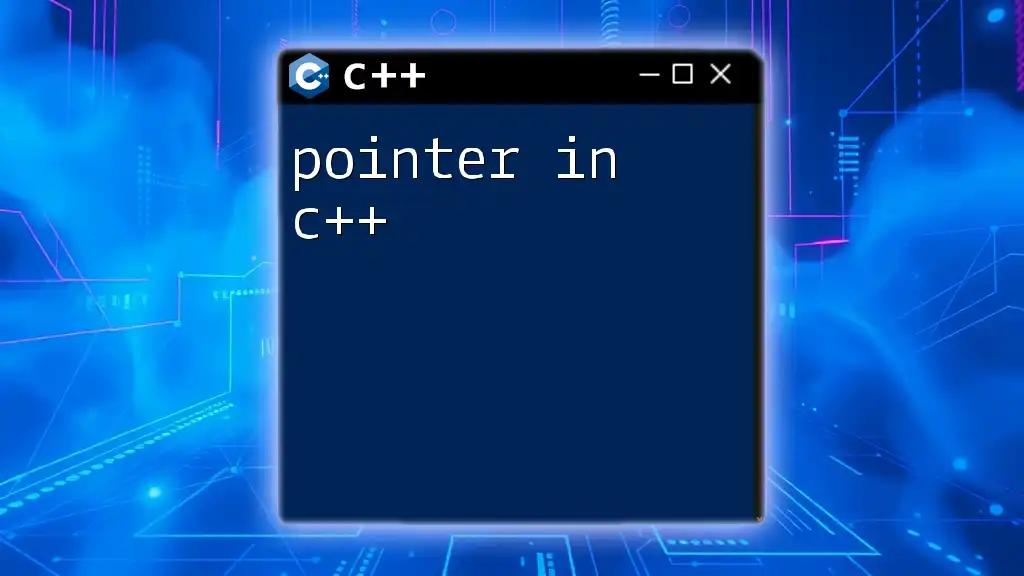
`constexpr` with C++ Standard Library
The C++ Standard Library has embraced `constexpr` through various functions. For instance, initializing containers with `constexpr` values can offer improved performance and enhanced readability.
Future of `constexpr`: Introductions in C++20 and Beyond
C++20 has brought forth exciting new capabilities. Mutable variables in `constexpr` functions allow the computation of values across various scopes, leading to increased versatility built directly into the language.

Best Practices for Using `constexpr`
When implementing `constexpr`, it is essential to consider the following guidelines for effective usage:
When to Use `constexpr`
Use `constexpr` when you know the values will remain constant and will benefit from compile-time evaluation. This includes mathematical functions and configuration constants, which simplify your code while boosting performance.
Common Pitfalls
Avoid common mistakes such as trying to modify mutable variables within `constexpr` functions or using unsupported constructs. Ensuring that your `constexpr` functions conform to the required standards is vital for their correct operation.
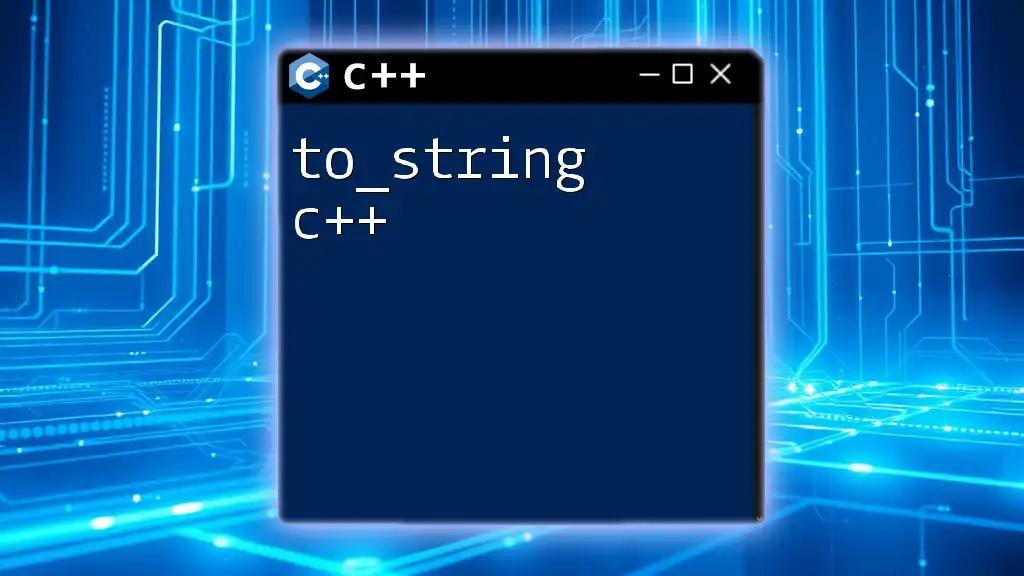
Conclusion
In summary, `constexpr in c++` is an indispensable feature that enhances both performance and code quality. By understanding its benefits, limitations, and appropriate usage, you can harness the full potential of compile-time calculations in your projects. As C++ continues to evolve, embracing and experimenting with `constexpr` will keep your skills at the forefront of modern programming.
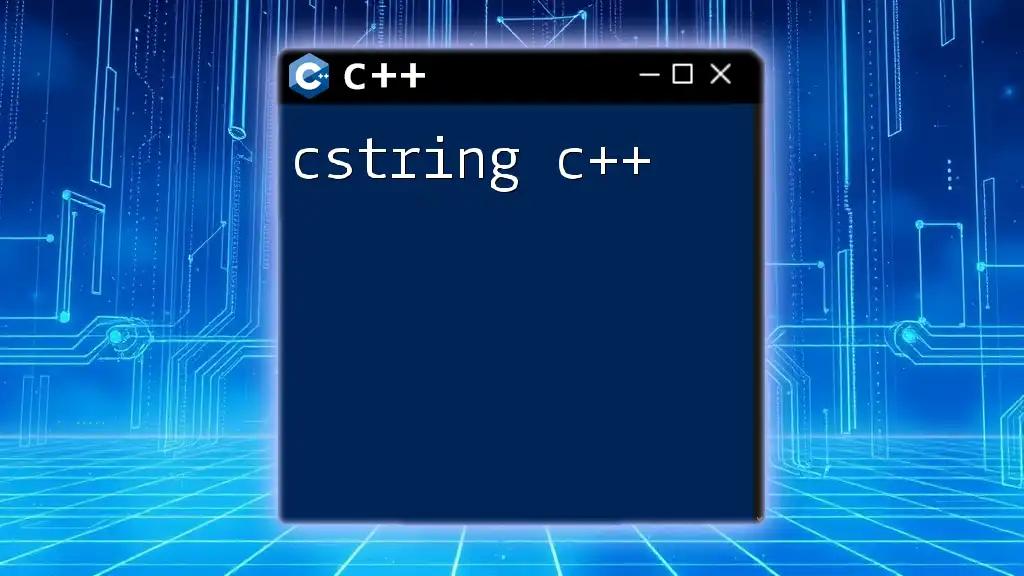
Additional Resources
To deepen your understanding of `constexpr`, consider exploring books, articles, and online resources dedicated to mastering C++. Getting hands-on experience through sample projects can solidify your grasp on `constexpr` applications.

Call to Action
Join a community of learners where you can exchange knowledge, participate in discussions, and enhance your C++ skills. Subscribe to newsletters or enroll in courses to keep updated with the latest advancements in C++.