Constants in C++ are fixed values that cannot be altered during program execution, ensuring that certain key variables remain unchanged, enhancing code stability and clarity.
Here is a code snippet demonstrating the use of constants:
#include <iostream>
using namespace std;
int main() {
const int MAX_USERS = 100; // Constant declaration
cout << "The maximum number of users is: " << MAX_USERS << endl;
return 0;
}
Understanding Constants in C++
Constants in C++ Definition
Constants in C++ are essentially immutable values that cannot be altered during the execution of a program. They serve as fixed points of reference that help improve the clarity of code by eliminating the need for magic numbers or hardcoded values throughout your application.
In essence, while variables can be changed as the program runs, constants maintain the same value throughout its lifespan. This fundamental difference aids in making your code more predictable and easier to maintain.
Why Use Constants?
Using constants in your code offers a myriad of advantages:
-
Enhances Code Readability: When you define a constant with a meaningful name, it allows anyone reading your code to infer what that value represents without needing extensive comments.
-
Avoids Magic Numbers: Magic numbers are literal values that appear in your code without context. If a number is defined as a constant, it becomes clear what its purpose is, significantly reducing confusion.
-
Improves Maintainability: If a constant value needs to be changed, only one line of code requires alteration, rather than hunting through the entire program. This not only reduces the likelihood of introducing errors, but also makes updates quicker and less painful.
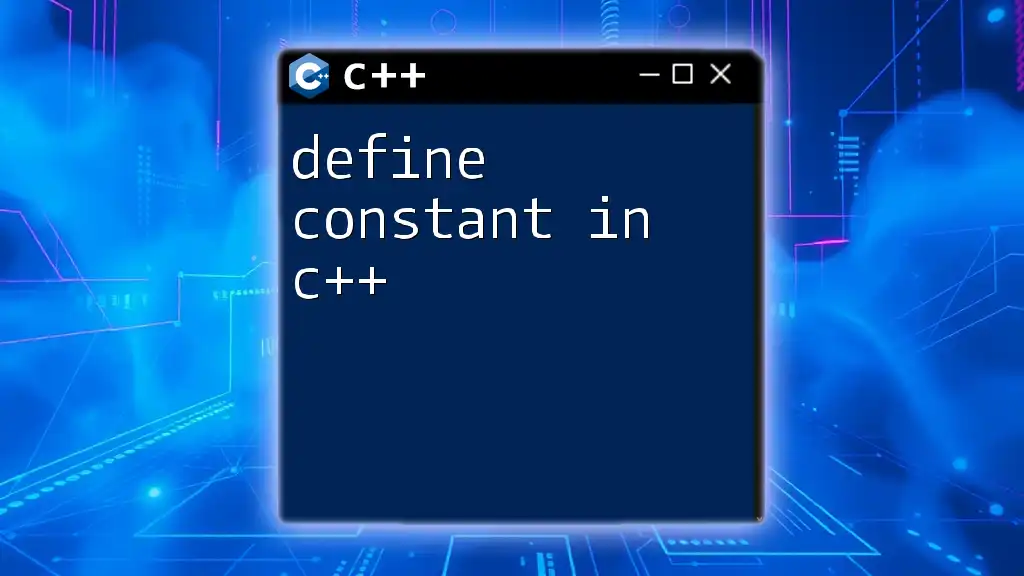
Types of Constants in C++
Literal Constants
Literal constants represent fixed values that do not change. They can be of various types, such as:
- Integer Constants: e.g., `100`, `-5`
- Floating-Point Constants: e.g., `3.14`, `-0.001`
- Character Constants: e.g., `'a'`, `'Z'`
- String Constants: e.g., `"Hello, World!"`
By using these constants, you can ensure that your values are correctly interpreted by the compiler without any ambiguity.
Symbolic Constants
Symbolic constants refer to values that are defined using keywords or preprocessor directives, providing better context. For example, instead of using `3.14` directly in your code, you might define it as a symbolic constant like this:
const double pi = 3.14159;
This approach improves readability and maintainability. If you ever need to update the value of `pi`, you only need to change it in one place.
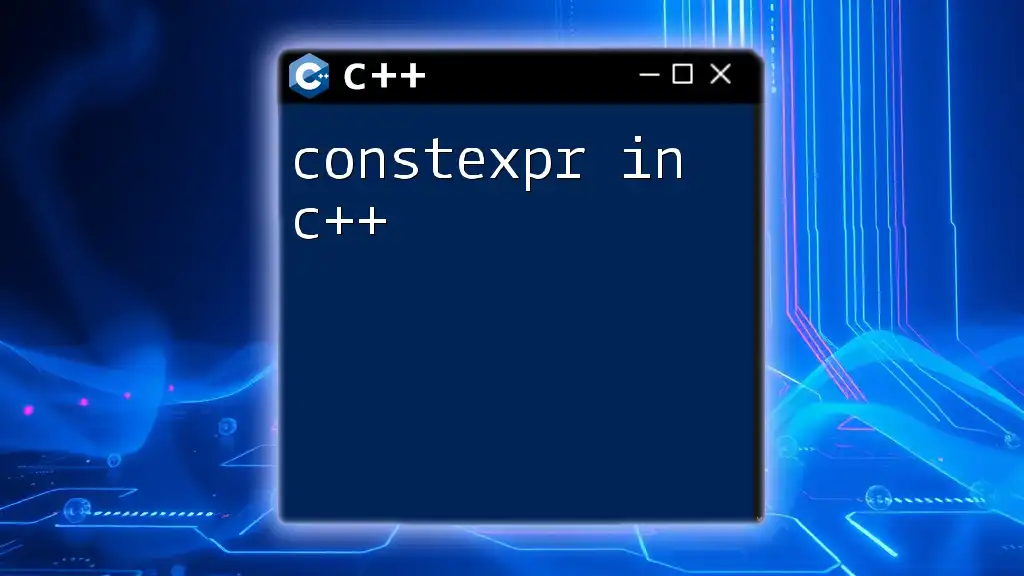
Declaring a Constant in C++
Using `const`
The `const` keyword is a common way to define constants in C++. Here’s how it works:
const int maxItems = 100;
In the example above, `maxItems` is defined as a constant integer. Once assigned a value, `maxItems` cannot be modified throughout the program.
Using `constexpr`
The `constexpr` keyword allows you to define compile-time constants. It enforces that the value is compiled at the time of compilation rather than at runtime. This can optimize performance. For example:
constexpr double pi = 3.14159;
Key Difference: While `const` allows you to define variables that may need to be initialized at runtime, `constexpr` ensures that the variable is efficiently available at compile time.
Using `#define`
The `#define` directive is a preprocessor command to create symbolic constants. Here’s an example:
#define MAX_BUFFER_SIZE 512
While using `#define` can be handy, it's essential to recognize its downsides. Preprocessor directives don’t respect scope and can lead to unexpected errors. Furthermore, because `#define` has no type, type checking doesn’t occur, which can introduce bugs.
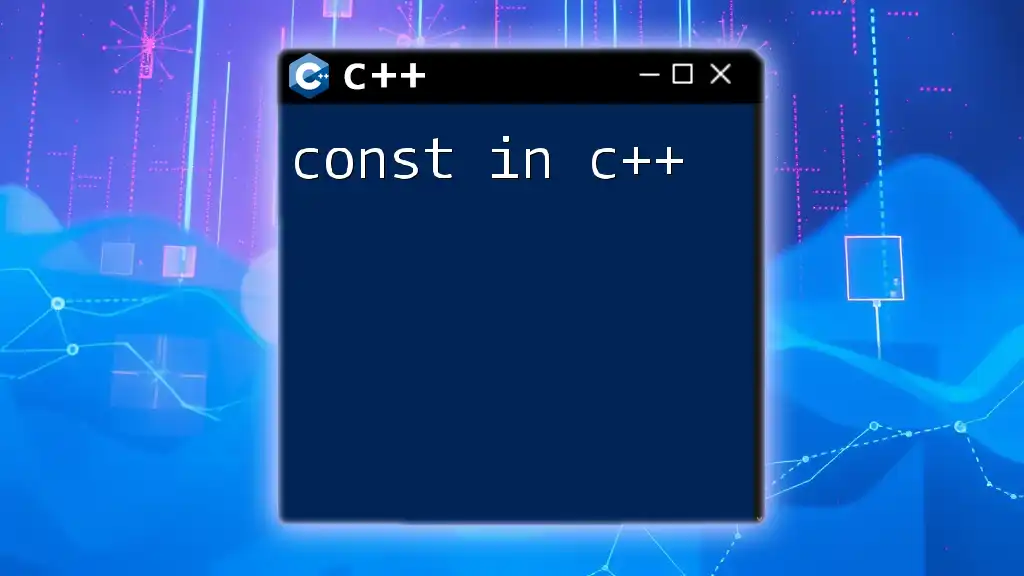
Best Practices for Using Constants
Choosing Appropriate Naming Conventions
To enhance the clarity of your code, it's essential to employ consistent naming conventions for constants. Generally, they are defined in uppercase letters with underscores separating words, such as `MAX_CONNECTIONS`. This practice clearly distinguishes constants from variables, improving readability.
Utilizing Constants in Functions
Constants can also be incredibly useful as function parameters. For example:
void processArray(const int arr[], const size_t size);
By using `const` in function parameters, you signify that the passed array should not be modified within the function, ensuring that the data remains intact.
Avoiding Common Pitfalls
While constants bring numerous benefits, they should not be overused. Ensure that you only define constants where they genuinely improve readability or maintainability. Excessive frivolous constants can clutter your code and lead to confusion.
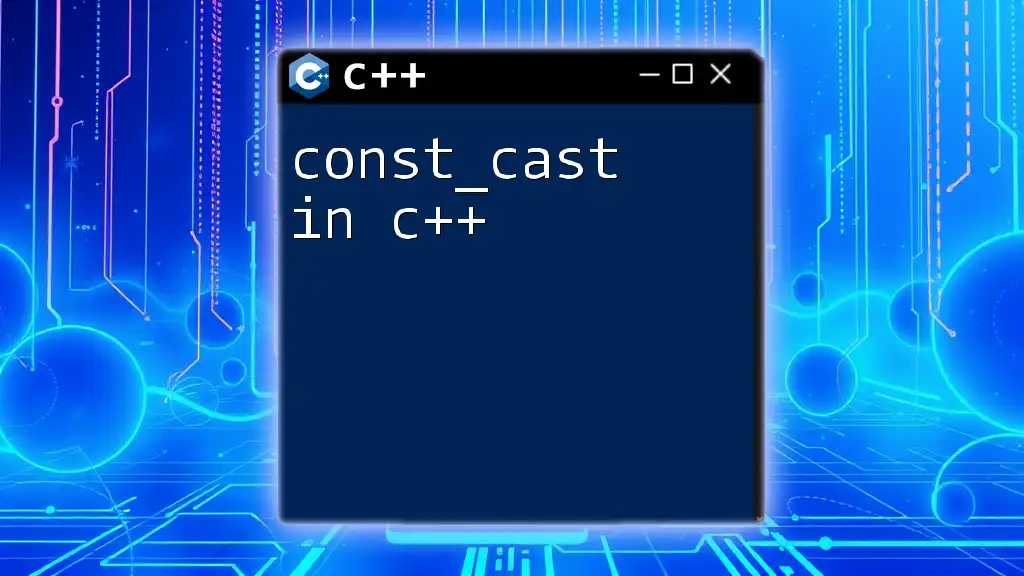
Real-world Applications of Constants in C++
Constants play a crucial role in developing robust software applications. For instance, in game development, constants are often used to define attributes like maximum levels, character statistics, or difficulty settings. Utilizing constants in games not only improves organization but also ensures that specific parameters remain unchanged throughout the gameplay.
In financial applications, constants are vital for maintaining fixed interest rates, tax percentages, and other financial parameters that should remain identical across various calculations.
By defining these parameters as constants, developers can swiftly modify the values as regulations or conditions change without combing through extensive codebases.
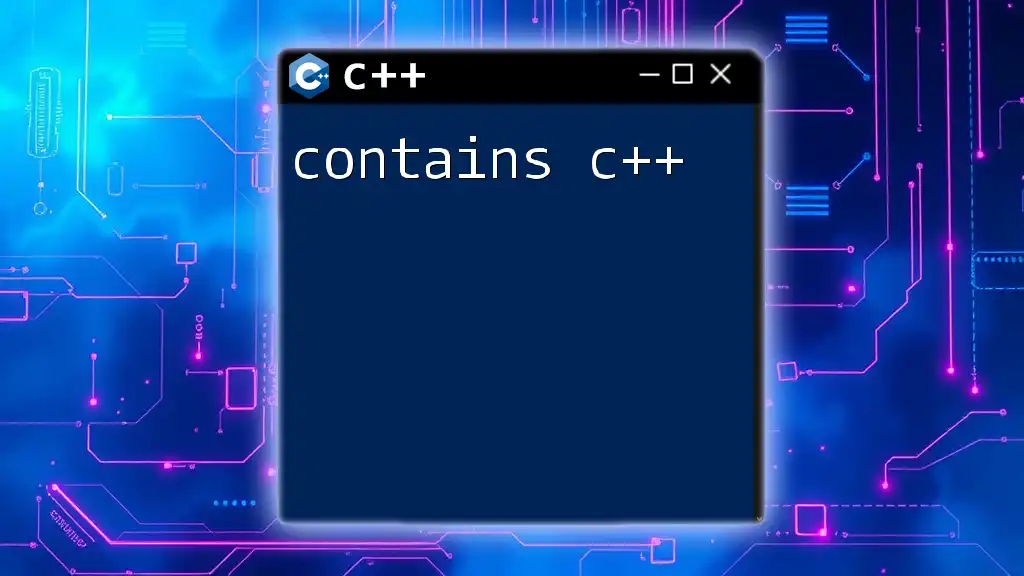
Conclusion
Understanding and utilizing constants in C++ is essential for writing clean and maintainable code. By adopting best practices, such as appropriate naming conventions and careful placement of constants, you'll enhance the readability and reliability of your applications. Furthermore, constants form a foundation for efficient programming, allowing for potential optimizations that improve the overall performance of your code.
By leveraging the power of constants in C++, you empower yourself to create better software that is both flexible and easy to manage. Take the time to integrate constants into your coding practice, and experience the positive impact on your programming journey.