In C++, character constants are always enclosed in single quotes, allowing the representation of individual characters.
char myChar = 'A';
What are Character Constants?
Character constants are fundamental building blocks in C++. They represent single characters that can be assigned to variables of the `char` data type. Unlike strings, which can consist of multiple characters and are enclosed in double quotes, character constants encapsulate a single character and are always enclosed in single quotes.
For example, when we declare a character constant like this:
char c = 'A'; // 'A' is a character constant
In this line, `'A'` is a character constant. It's crucial to understand that using single quotes signifies that we are working with a single character, as opposed to a more extensive text string.
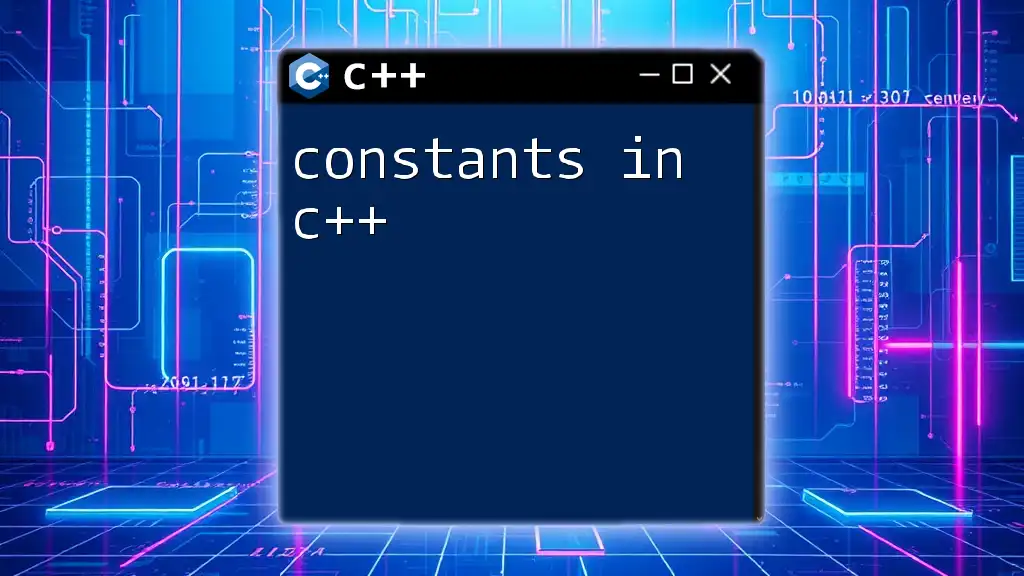
Syntax of Character Constants
The syntax for character constants is straightforward. They are specified using single quotes and can include any valid character. Characters can be letters, digits, punctuation marks, or special characters.
Here's an example:
char letter = 'B'; // Valid character constant
char digit = '3'; // Valid character constant
char specialChar = '@'; // Valid character constant
One key point to remember is that while character constants are simple, they must always be enclosed in single quotes. Not doing so will lead to compilation errors because C++ won't recognize them correctly.
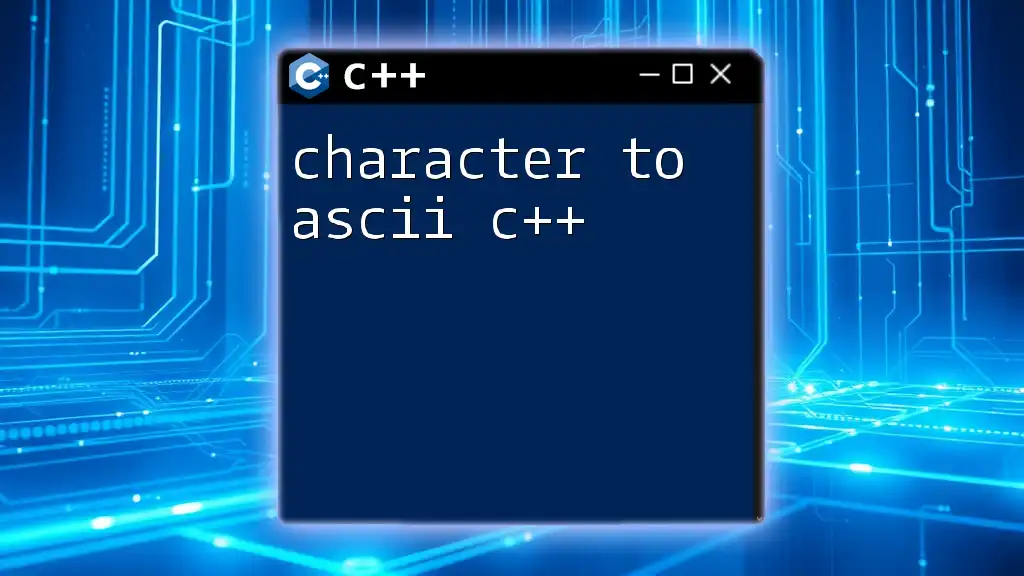
Why Are Character Constants Enclosed in Single Quotes?
The use of single quotes for character constants goes back to the design of the C++ language. Single quotes indicate that the enclosed value is a single character, which is critical for differentiating between different data types.
In comparison, when you use double quotes, you are defining a string literal—a sequence of characters treated as a single entity:
char str[] = "Hello"; // "Hello" is a string literal and NOT a character constant
It’s important to note that using double quotes for character constants is a common mistake. The compiler will throw an error, insisting that you use single quotes instead.
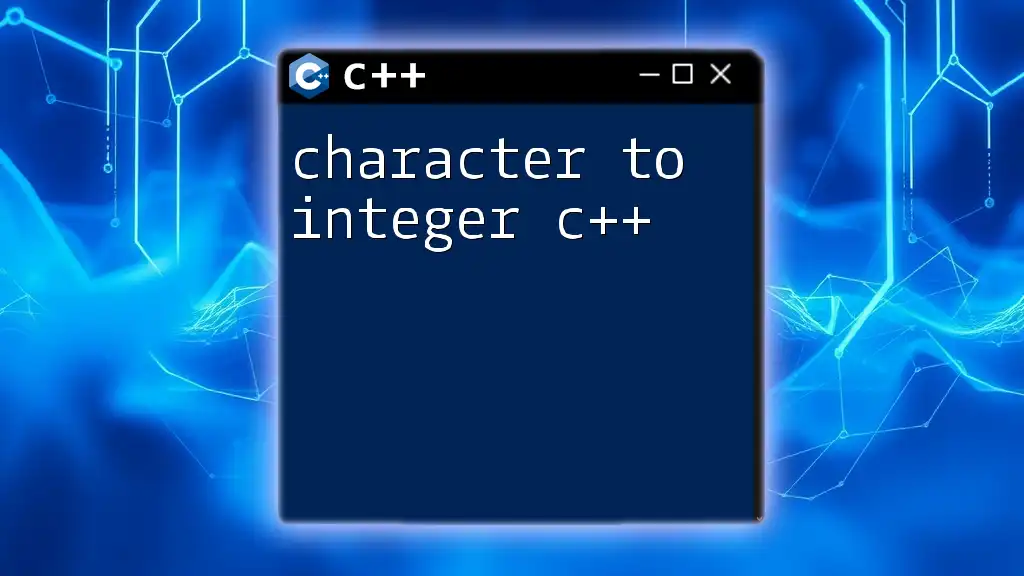
Types of Character Constants
Regular Character Constants
Regular character constants are the most basic form of character representation in C++. They consist of a single character and can be assigned like any other variable. Here’s an example:
char x = 'x'; // Regular character constant
Escape Sequences
An escape sequence is a special way to represent certain characters that cannot be easily typed on the keyboard. They allow you to include characters like new lines or tabs within a character constant.
Here’s a list of some common escape sequences:
- `\'` for a single quote
- `\"` for a double quote
- `\\` for a backslash
- `\n` for a new line
- `\t` for a tab
For example, to represent a new line in your code, you would write:
char newline = '\n'; // New line is represented using escape sequence
Using escape sequences can significantly enhance the readability of your code, allowing for special character inclusion where necessary.
Wide Character Constants
Wide character constants are used to handle a larger variety of characters than the standard `char` type. In C++, wide characters are defined using the `wchar_t` type, which is suitable for representing characters from languages that require more extensive character sets.
Usage of wide character constants is marked by the prefix `L`, as shown here:
wchar_t w = L'성'; // Korean character as a wide character constant
The distinction between `char` and `wchar_t` is important, especially in applications requiring internationalization or when working with a broader range of Unicode characters.
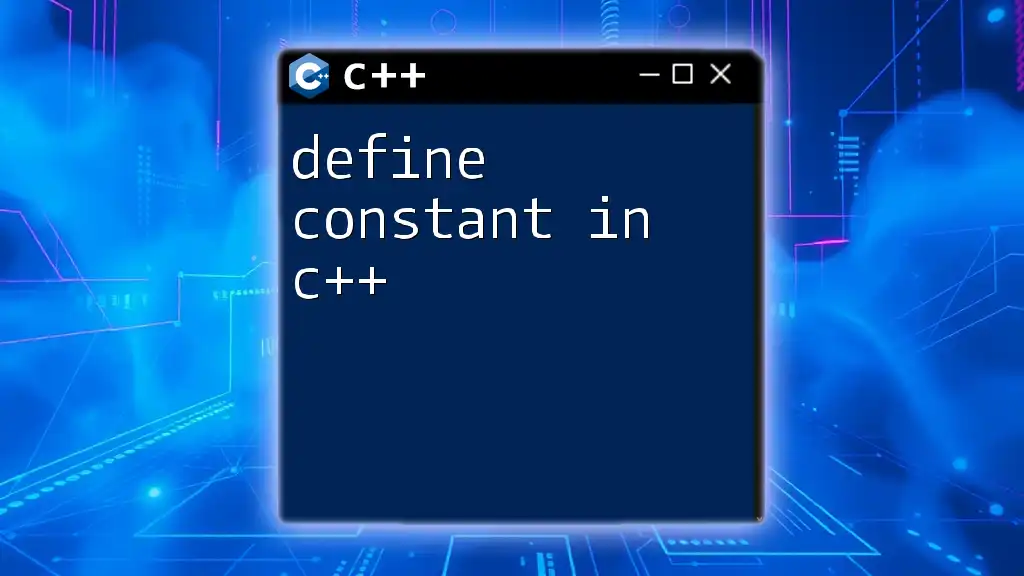
Common Mistakes with Character Constants
One of the most frequent mistakes beginners make is misusing double quotes instead of single quotes. For instance, if you mistakenly write:
char wrong = "A"; // Compilation error
This will lead to a compilation error because the compiler expects a character constant but sees a string literal instead.
Another common mistake is forgetting to escape special characters. For instance:
char misused = 'A\n'; // Incorrect: using escape in character constant
In this case, `'\n'` cannot be used inside a single-quoted character constant, as it denotes a single character only.
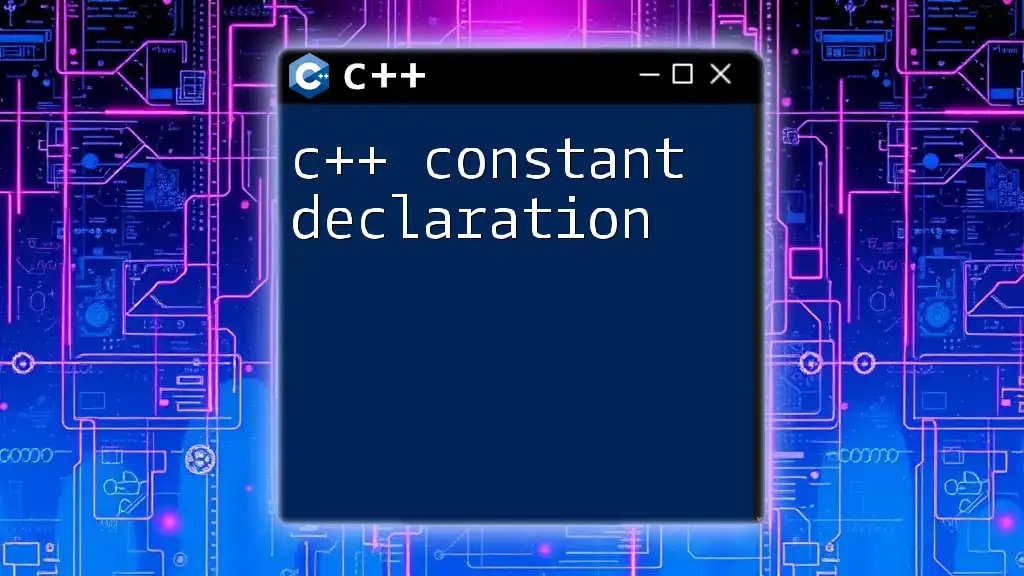
Best Practices for Using Character Constants
When you work with character constants, adhering to best practices can significantly improve your code’s clarity and maintainability:
- Always use single quotes for your character constants.
- Use escape sequences carefully to maintain readability without cluttering your code.
- Ensure that your code is not overly reliant on special characters.
By following these simple guidelines, your C++ code will be more intuitive and easier to read.
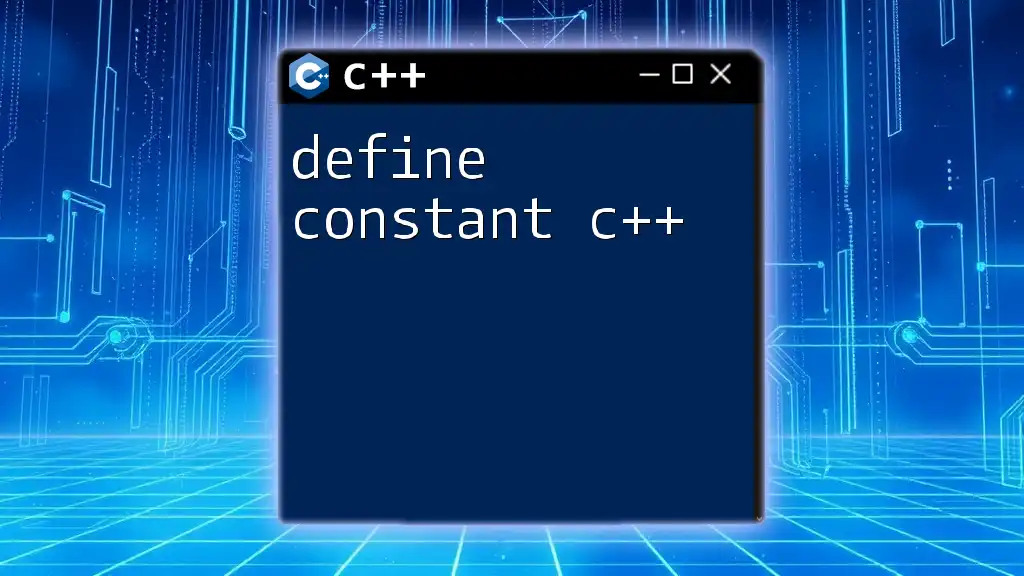
Real-World Applications
Character constants find their place in numerous real-world applications, from control statements to comparison operations. Here’s an example of how you might use a character constant in a conditional statement:
if (inputChar == 'y') {
// Do something specific if user input is 'y'
}
Here, `inputChar` is compared directly to the character constant `'y'`, showing how character constants can guide program flow based on user input.
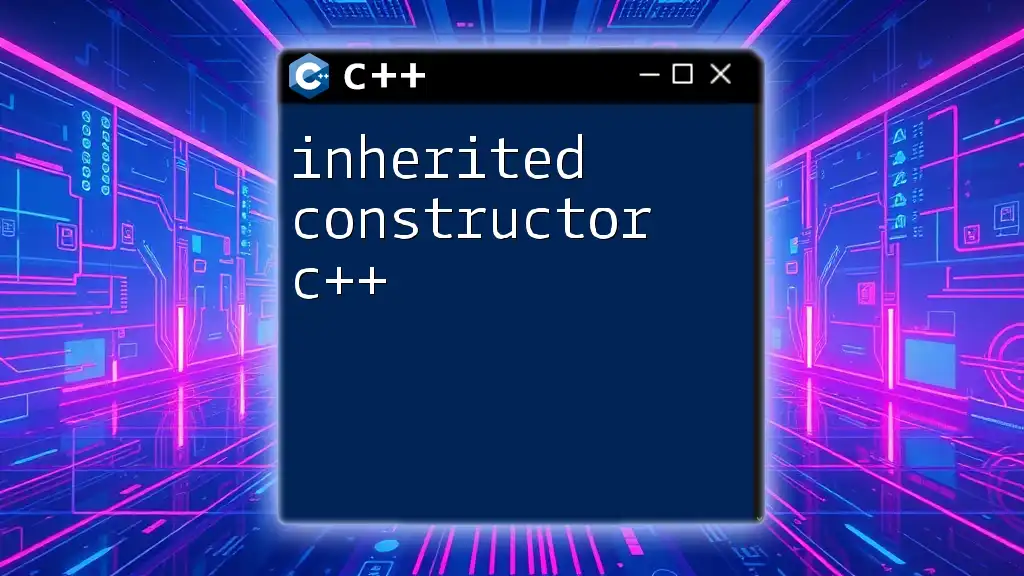
Conclusion
In conclusion, understanding that character constants in C++ are always enclosed in single quotes is fundamental for correctly writing and interpreting code. These constants are crucial for representing single characters and play a significant role in control flows and textual data manipulation. By practicing with these character constants and following the discussed guidelines, you will be better prepared for your journey in mastering C++.