In C++, parameter passing allows functions to receive arguments either by value or by reference, enabling them to manipulate data without altering the original variable when passed by value.
Here's a simple code snippet demonstrating both methods:
#include <iostream>
void passByValue(int num) { // Parameter passed by value
num = num * 2;
std::cout << "Inside passByValue: " << num << std::endl;
}
void passByReference(int &num) { // Parameter passed by reference
num = num * 2;
std::cout << "Inside passByReference: " << num << std::endl;
}
int main() {
int a = 5;
int b = 5;
passByValue(a); // a remains 5
std::cout << "After passByValue: " << a << std::endl;
passByReference(b); // b is now 10
std::cout << "After passByReference: " << b << std::endl;
return 0;
}
Understanding Parameters in C++
What are Function Parameters?
Function parameters are variables that allow data to be passed to functions. They act as placeholders for the values you pass (known as arguments) when you call a function. Understanding function parameters is pivotal because they determine how functions can interact with the data, allowing for flexibility and reusability.
Types of Parameters
Default Parameters
Default parameters allow functions to be called with fewer arguments than they are defined with. If an argument is not provided in the function call, the default value is used.
Example code snippet:
#include <iostream>
using namespace std;
void greet(string name = "Guest") {
cout << "Hello, " << name << "!" << endl;
}
int main() {
greet(); // Outputs: Hello, Guest!
greet("Alice"); // Outputs: Hello, Alice!
return 0;
}
In this example, if no name is provided, `"Guest"` serves as the default.
Constant Parameters
A constant parameter is defined using the `const` keyword, which prevents the function from modifying the value of the argument passed. This is crucial for maintaining the integrity of data, especially when using large data structures.
Example code snippet:
#include <iostream>
using namespace std;
void printArray(const int arr[], int size) {
for (int i = 0; i < size; ++i) {
cout << arr[i] << " ";
}
cout << endl;
}
int main() {
int myArray[] = {1, 2, 3, 4, 5};
printArray(myArray, 5);
return 0;
}
In the `printArray` function, `const` prevents any modifications to `arr`, thus protecting the original array.
Parameter Passing Methods
Pass by Value
In pass by value, a copy of the argument's value is made and passed to the function. Modifications to the parameter within the function do not affect the original argument.
Example code snippet:
#include <iostream>
using namespace std;
void modifyValue(int number) {
number += 5;
cout << "Inside function: " << number << endl; // Outputs modified value
}
int main() {
int num = 10;
modifyValue(num);
cout << "Outside function: " << num << endl; // Outputs original value
return 0;
}
Here, `num` remains unchanged outside the function.
Pass by Reference
When passing by reference, a reference to the actual variable is passed, allowing the function to modify the original argument’s value directly.
Example code snippet:
#include <iostream>
using namespace std;
void modifyValue(int &number) {
number += 5;
cout << "Inside function: " << number << endl; // Outputs modified value
}
int main() {
int num = 10;
modifyValue(num);
cout << "Outside function: " << num << endl; // Outputs modified value
return 0;
}
In this case, changes made to `number` inside the function reflect on `num`.
Pass by Pointer
Passing by pointer allows functions to manipulate the value of variables by utilizing their memory addresses. This method is particularly useful for dynamic memory management.
Example code snippet:
#include <iostream>
using namespace std;
void modifyValue(int *number) {
*number += 5; // Dereference to modify the value
cout << "Inside function: " << *number << endl; // Outputs modified value
}
int main() {
int num = 10;
modifyValue(&num);
cout << "Outside function: " << num << endl; // Outputs modified value
return 0;
}
Here, the function effectively alters the value of `num` by manipulating its memory address.
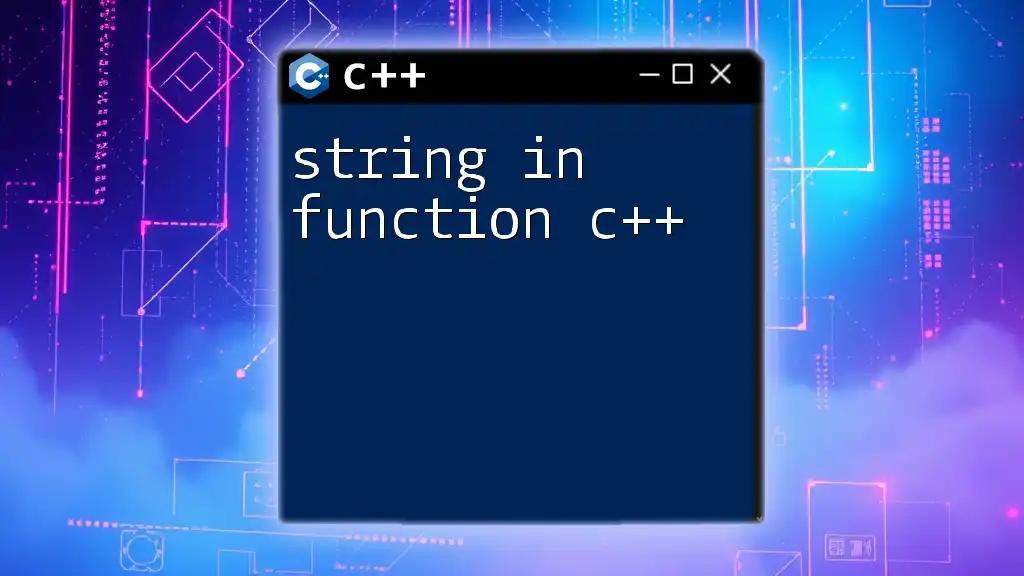
Choosing the Right Parameter Passing Method
When to Use Pass by Value
Pass by value is ideal when:
- The data being passed is small (like basic types).
- You want to ensure that the original variable remains unchanged. Performance-wise, pass by value can be less efficient when dealing with large data structures due to the overhead of copying.
When to Use Pass by Reference
Pass by reference is preferred when:
- You need to modify the original variable within the function.
- You are dealing with large objects, where copying could be expensive in terms of performance. This method minimizes overhead and ensures efficient use of memory.
When to Use Pass by Pointer
Pass by pointer comes in handy when:
- You need to manage dynamic memory or when working with arrays.
- You want to indicate the absence of values by passing `nullptr`. Be cautious with pointers, as they can lead to issues if not handled properly (like dangling pointers).

Comparing Parameter Passing Methods
Performance Implications
- Pass by value: Involves copying data, which can be costly for large objects. However, if function side effects aren't desired, it's safer.
- Pass by reference: Avoids the overhead of copying by passing a reference, enhancing performance.
- Pass by pointer: Similar to pass by reference in terms of performance, but adds complexity in the form of pointer management.
Code Readability
Choosing the right parameter passing method can greatly impact code clarity. Prefer pass by reference or pointer when you need to modify data; use pass by value when the original data should remain unchanged. Additionally, using `const` with pass by reference enhances readability and intent.

Examples of Parameter Passing in C++
Simple Function Example
Here’s a simple illustration of all three methods:
#include <iostream>
using namespace std;
void passByValue(int x) {
x += 10;
}
void passByReference(int &y) {
y += 10;
}
void passByPointer(int *z) {
*z += 10;
}
int main() {
int a = 5, b = 5, c = 5;
passByValue(a); // a remains 5
passByReference(b); // b becomes 15
passByPointer(&c); // c becomes 15
cout << "a: " << a << ", b: " << b << ", c: " << c << endl;
return 0;
}
Advanced Function Example Using Classes
Consider a more complex example where classes are involved:
#include <iostream>
using namespace std;
class Rectangle {
public:
int width, height;
Rectangle(int w, int h) : width(w), height(h) {}
};
void updateDimensions(Rectangle &rect) {
rect.width += 5;
rect.height += 5;
}
int main() {
Rectangle rect(10, 20);
cout << "Before: " << rect.width << "x" << rect.height << endl;
updateDimensions(rect);
cout << "After: " << rect.width << "x" << rect.height << endl;
return 0;
}
In this case, modifying `rect` within `updateDimensions` directly alters its attributes.
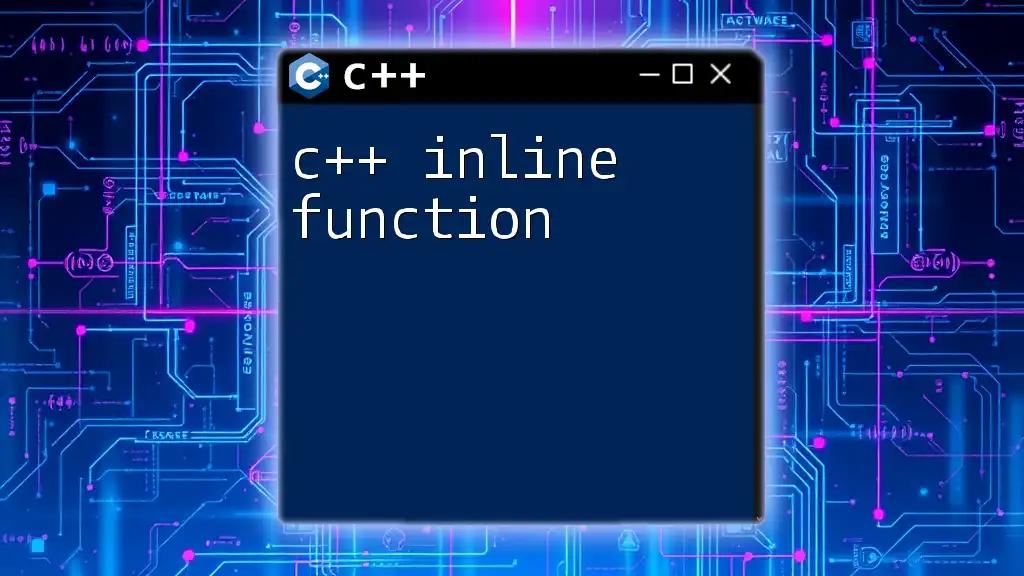
Common Mistakes in Parameter Passing
Forgetting Const Keyword
One common mistake in parameter passing is neglecting to use the `const` keyword for reference parameters. This can lead to unintended modifications and bugs. Always be cautious with references; use `const` when you intend to keep the argument immutable.
Example of potential mistake:
void incorrectFunction(int &number) {
number += 5; // This modifies the argument
}
Instead, write it as:
void correctFunction(const int &number) {
// Cannot modify number here
}
Misunderstanding References and Pointers
Many programmers confuse references with pointers. Here are key distinctions:
- References are automatically dereferenced, making them easier to use.
- Pointers must be explicitly dereferenced and can be `nullptr`, introducing potential risks.
Clarification with examples can prevent these misunderstandings.

Conclusion
Mastering parameter passing in C++ functions is essential for efficient coding. Knowing when and how to use pass by value, pass by reference, and pass by pointer can significantly impact your function's performance and behavior. Practice these concepts, and your grasp of C++ will become much stronger!

Call to Action
Start experimenting with different parameter passing methods in your own projects to gain a deeper understanding. For more advanced topics and extensive learning resources, explore our recommended materials and community forums.
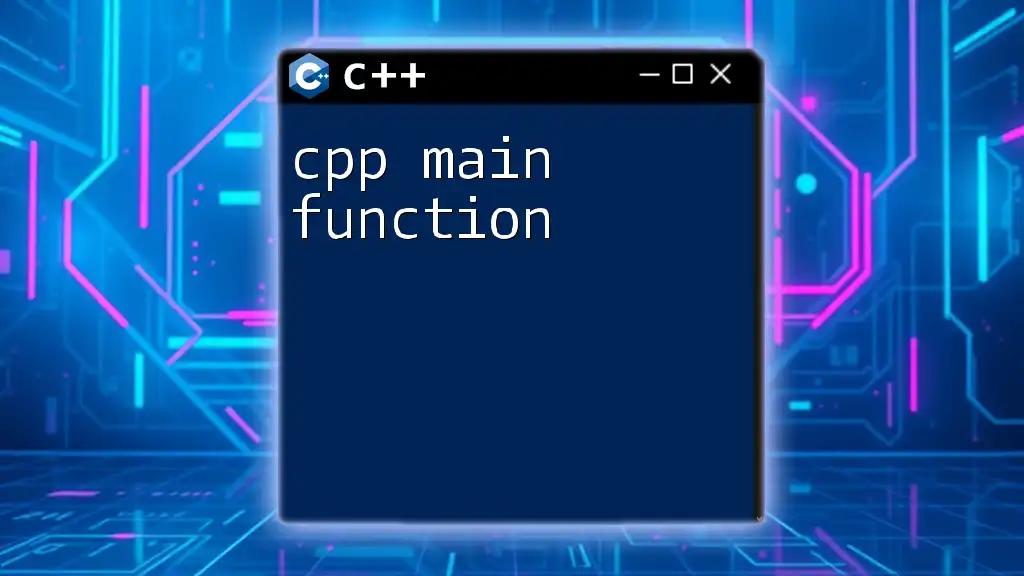
Additional Resources
Explore great C++ books, online courses, and community forums to further enhance your learning experience and connect with fellow programmers.