In C++, trigonometric functions such as sine, cosine, and tangent can be utilized through the `<cmath>` library to perform calculations involving angles measured in radians.
Here's a code snippet demonstrating the use of these functions:
#include <iostream>
#include <cmath>
int main() {
double angle = 0.5; // angle in radians
std::cout << "Sine: " << sin(angle) << std::endl;
std::cout << "Cosine: " << cos(angle) << std::endl;
std::cout << "Tangent: " << tan(angle) << std::endl;
return 0;
}
Understanding Trigonometric Functions in C++
C++ trigonometric functions are essential mathematical tools used in various applications, from graphics programming to physics simulations. These functions are part of the C++ `<cmath>` library, which provides a rich set of mathematical operations, including the ability to perform calculations related to angles and trigonometric ratios.
Trigonometric functions operate primarily based on angles, and it is crucial to understand that they require angles to be specified in radians rather than degrees. The conversion between degrees and radians is essential for accurately using these functions. The formula is:
\[ \text{radians} = \text{degrees} \times \left(\frac{\pi}{180}\right) \]
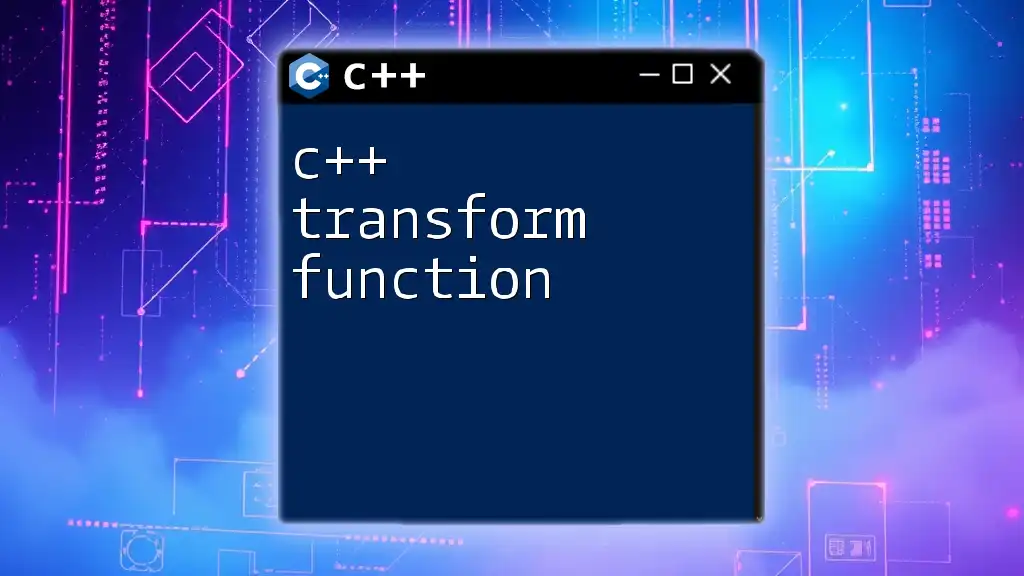
Basic Trigonometric Functions
Sine Function
The sine function, denoted as sin, computes the ratio of the opposite side to the hypotenuse in a right triangle. It is periodic with a period of \(2\pi\) radians.
Here's how you can use the `sin()` function in C++:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double angle = 30.0; // in degrees
double radians = angle * (M_PI / 180.0); // converting degrees to radians
cout << "Sine of " << angle << " degrees is: " << sin(radians) << endl;
return 0;
}
In this code, we convert 30 degrees to radians and calculate its sine, resulting in an output of 0.5. This demonstrates how the sine function can help determine the relationship between the angle and the lengths of the sides in right-angle scenarios.
Cosine Function
The cosine function, represented as cos, calculates the ratio of the adjacent side to the hypotenuse. Like sine, it is also periodic with a period of \(2\pi\) radians.
To use the `cos()` function in C++, follow this example:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double angle = 60.0; // in degrees
double radians = angle * (M_PI / 180.0); // converting degrees to radians
cout << "Cosine of " << angle << " degrees is: " << cos(radians) << endl;
return 0;
}
For an input of 60 degrees, the cosine function yields 0.5, which is critical for applications involving wave motions and alternating currents.
Tangent Function
The tangent function, denoted as tan, calculates the ratio of sine to cosine (opposite over adjacent). It has a period of \(\pi\) radians and can approach infinity, creating undefined points at certain angles.
Here’s a simple example of using the `tan()` function:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double angle = 45.0; // in degrees
double radians = angle * (M_PI / 180.0); // converting degrees to radians
cout << "Tangent of " << angle << " degrees is: " << tan(radians) << endl;
return 0;
}
For 45 degrees, the output will be 1, showcasing a unique scenario in right triangles where the opposite and adjacent sides are equal.
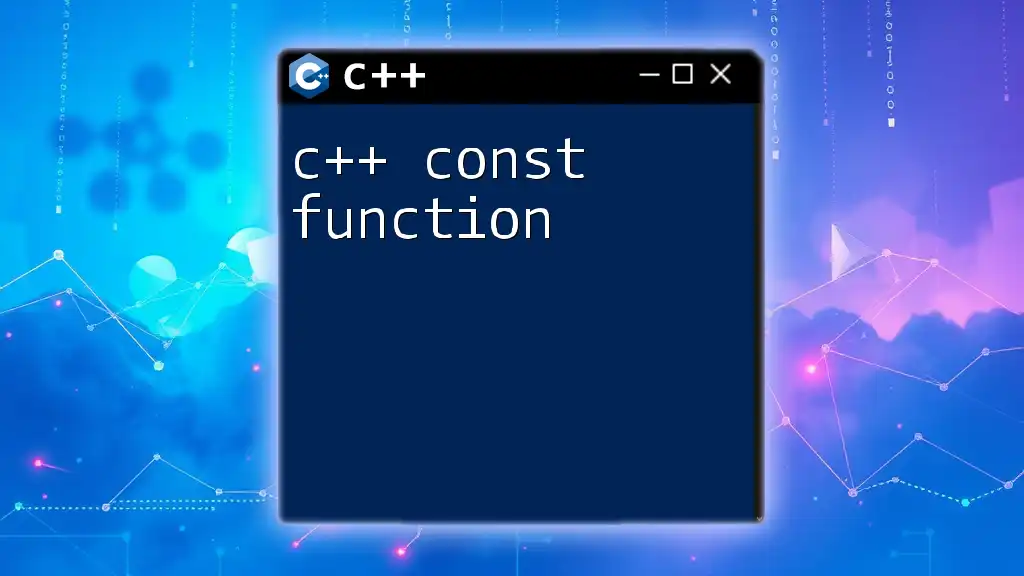
Inverse Trigonometric Functions
Inverse trigonometric functions help determine angles based on the values of trigonometric ratios. These include arcsine, arccosine, and arctangent.
Arcsine Function
The arcsine function, expressed as asin, returns the angle whose sine is a given value. The output is in radians and spans from \(-\frac{\pi}{2}\) to \(\frac{\pi}{2}\).
Utilizing the `asin()` function can be done as follows:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double value = 0.5; // the sine value
double result = asin(value); // result in radians
cout << "Arcsine of " << value << " gives: " << result * (180.0/M_PI) << " degrees" << endl;
return 0;
}
This outputs 30 degrees, indicating that 0.5 is the sine of 30 degrees.
Arccosine Function
The arccosine function, noted as acos, determines the angle whose cosine corresponds to a given value. It ranges from 0 to \(\pi\) radians.
Here's an example implementation:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double value = 0.5; // the cosine value
double result = acos(value); // result in radians
cout << "Arccosine of " << value << " gives: " << result * (180.0/M_PI) << " degrees" << endl;
return 0;
}
This computation results in an output of 60 degrees, illustrating how the cosine function is applied inversely.
Arctangent Function
The arctangent function, represented as atan, derives the angle from a given tangent ratio, and its output ranges from \(-\frac{\pi}{2}\) to \(\frac{\pi}{2}\).
You can calculate the arctangent as follows:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double value = 1.0; // the tangent value
double result = atan(value); // result in radians
cout << "Arctangent of " << value << " gives: " << result * (180.0/M_PI) << " degrees" << endl;
return 0;
}
For an input of 1.0, the output will be 45 degrees, which allows us to navigate through various scenarios and geometrical computations.
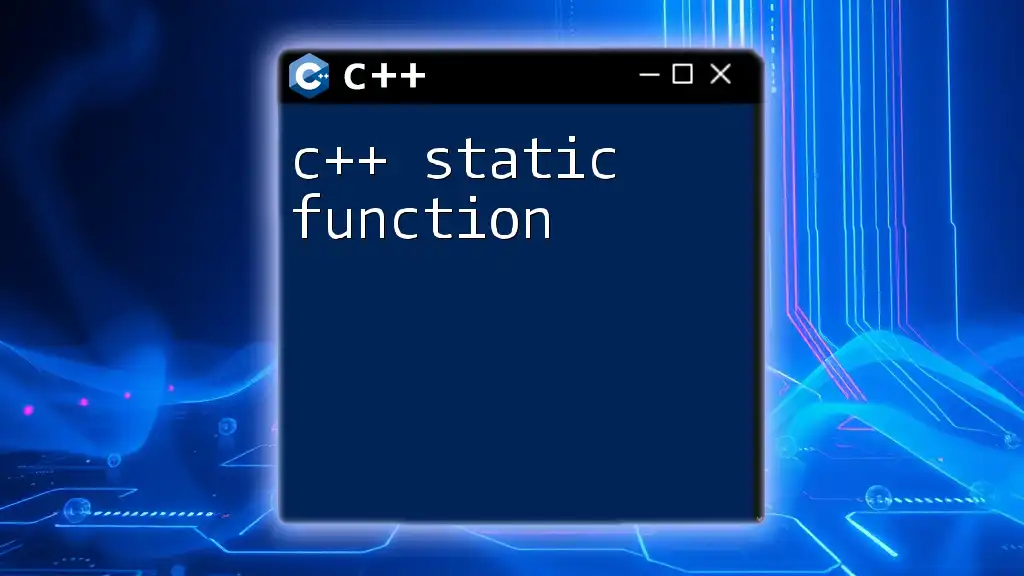
Advanced Trigonometric Functions
Hyperbolic Functions
In addition to basic trigonometric functions, C++ provides hyperbolic functions such as sinh, cosh, and tanh. These functions are helpful in advanced mathematics, particularly in calculus and physics.
Here's a usage example:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double angle = 1.0; // in radians
cout << "Hyperbolic sine: " << sinh(angle) << endl;
cout << "Hyperbolic cosine: " << cosh(angle) << endl;
cout << "Hyperbolic tangent: " << tanh(angle) << endl;
return 0;
}
This code provides insights into the exponential relationships in hyperbolic geometry, each calculation shedding light on the behavior of such functions.
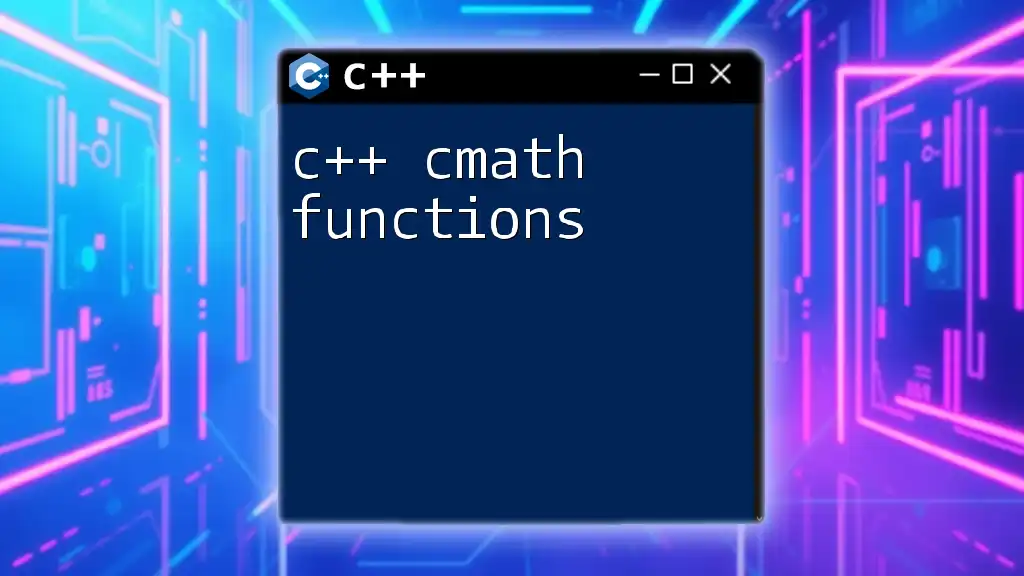
Practical Applications of Trigonometric Functions in C++
Graphics Programming
C++ trigonometric functions play a fundamental role in graphics programming, enabling developers to create realistic animations and graphical representations. For example, they can be used to facilitate rotation effects, where the position of an object is updated using sine and cosine functions for smooth transitions.
Physics Simulations
In physics simulations, trigonometric functions are vital for modeling periodic motion and oscillations, such as those seen in pendulums and waves. These calculations allow scientists and engineers to predict behavior accurately and design effective systems based on trigonometric relationships.
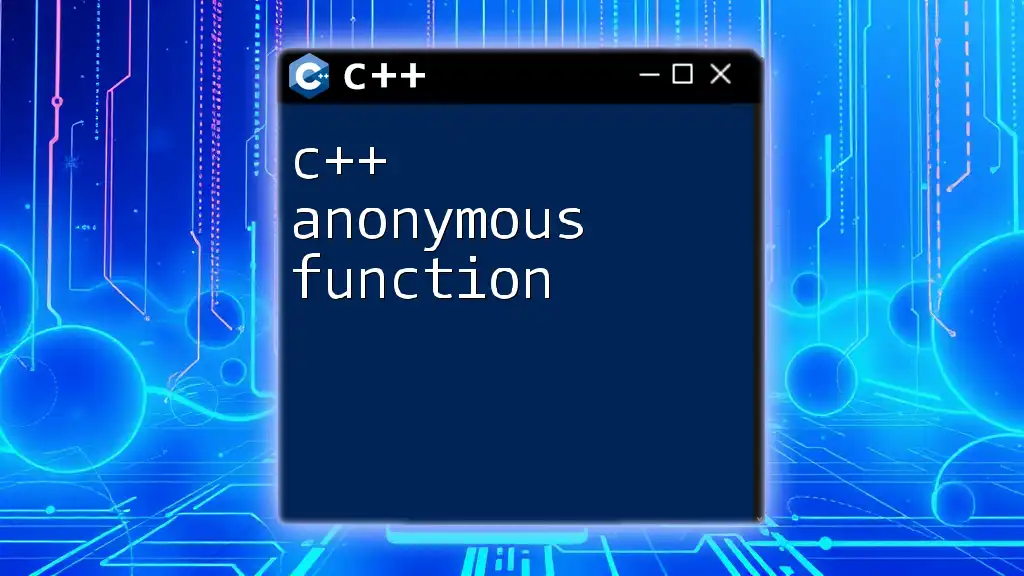
Conclusion
C++ trigonometric functions are invaluable tools capable of handling various mathematical challenges. With an understanding of their definitions, uses, and underlying mathematical principles, developers can leverage these functions in both simple and complex programming environments to enhance functionality and accuracy in their applications.
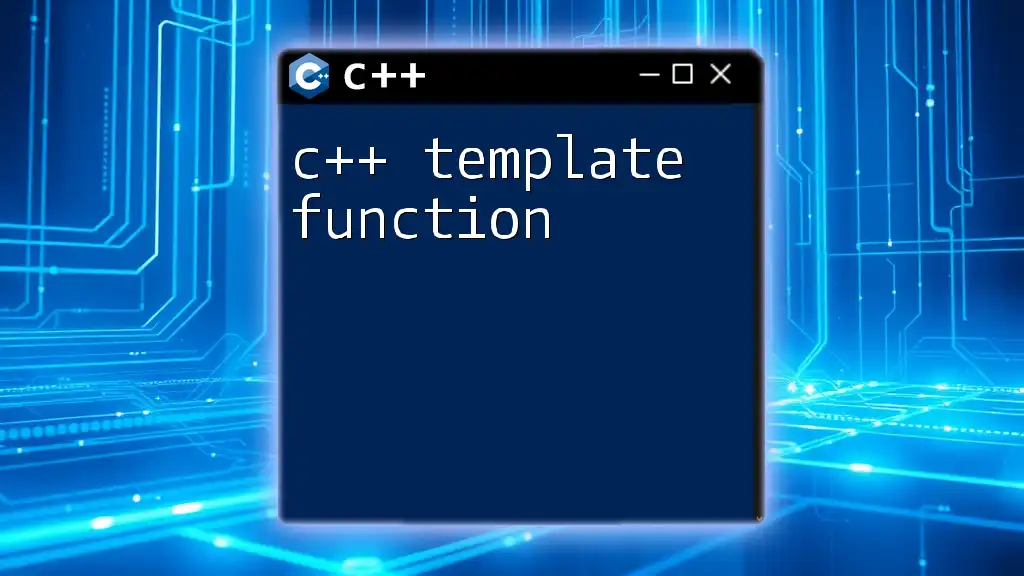
Additional Resources
For further exploration of C++ trigonometric functions, consider seeking out books on numerical analysis, online tutorials specific to C++ mathematical libraries, and forums where you can engage with other developers and share insights. The learning process is strengthened through practice and community, so dive in and start applying what you've learned!