The C++ `find` function, part of the `<algorithm>` library, allows you to search for a specific element in a given range, returning an iterator to the first occurrence of the element or the end of the range if not found.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find(numbers.begin(), numbers.end(), 3);
if (it != numbers.end()) {
std::cout << "Found: " << *it << std::endl; // Output: Found: 3
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
Understanding the C++ Find Function
Definition of the Find Function
The C++ `find` function is a standard library function that helps in searching for a specific element within a range of elements. It's essential for developers working with various data structures, such as arrays, vectors, and lists. The `find` function simplifies the process of element search, ensuring that developers can locate an item efficiently without manually iterating through each element.
Where to Find the Function
The `find` function is included in the Standard Template Library (STL), specifically found within the `<algorithm>` header. By including this header at the top of your program, you gain access to a wide array of algorithms, including `find`.

Syntax of the Find Function
Basic Syntax
The syntax for the `find` function is straightforward:
iterator find(iterator first, iterator last, const T& value);
Parameters Explained
- first: This is the beginning of the range where the search will begin.
- last: This marks the end of the range, and the search will consider elements up to, but not including, this specified position.
- value: The specific value you are searching for within the designated range.
Return Value
The `find` function returns an iterator pointing to the first occurrence of the specified value. If the value is not found, the function returns the value of the `last` iterator, indicating that the end of the search range has been reached.
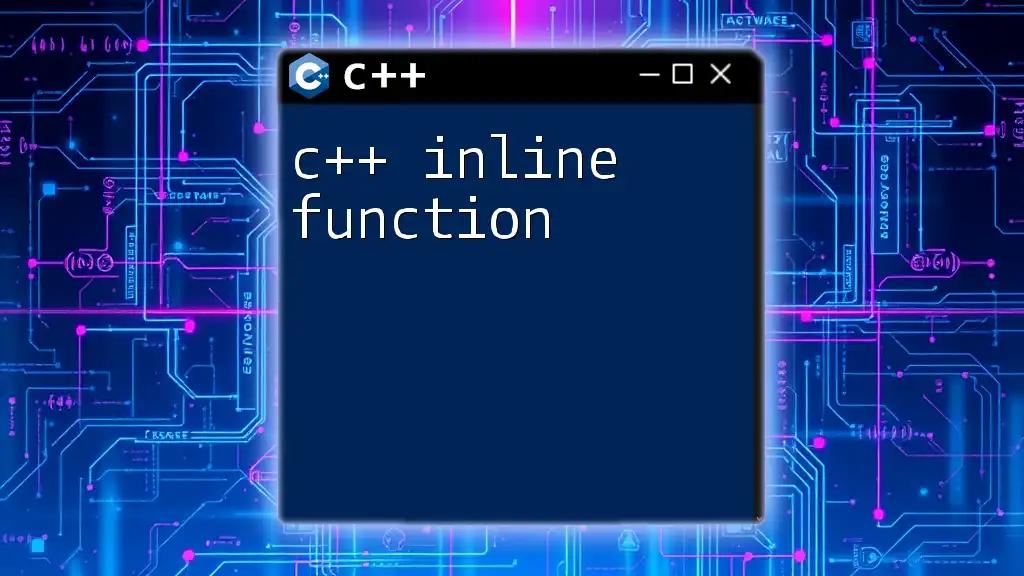
Using the Find Function in Practice
Finding Elements in a Vector
Vectors are commonly used because of their dynamic size and ease of access. Here’s a simple example to demonstrate how to utilize the `find` function with a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find(numbers.begin(), numbers.end(), 3);
if (it != numbers.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
In this code, a vector named `numbers` is created. The `find` function is used to search for the integer `3`. If found, it prints the value; otherwise, it informs that the value was not located.
Finding Elements in a List
Similar to vectors, lists can also be searched using the `find` function. Here's an example demonstrating this:
#include <iostream>
#include <list>
#include <algorithm>
int main() {
std::list<std::string> names = {"Alice", "Bob", "Charlie"};
auto it = std::find(names.begin(), names.end(), "Bob");
if (it != names.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
In this instance, the list `names` is searched for the string "Bob". The `find` function effectively locates the name, demonstrating its versatility in different STL containers.
Finding Elements in an Array
Arrays can also be efficiently searched using the `find` function. Here is how it works:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int* it = std::find(std::begin(arr), std::end(arr), 30);
if (it != std::end(arr)) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
This code leverages `std::begin` and `std::end` to define the search range of the array, allowing the `find` function to effectively locate the value `30`.
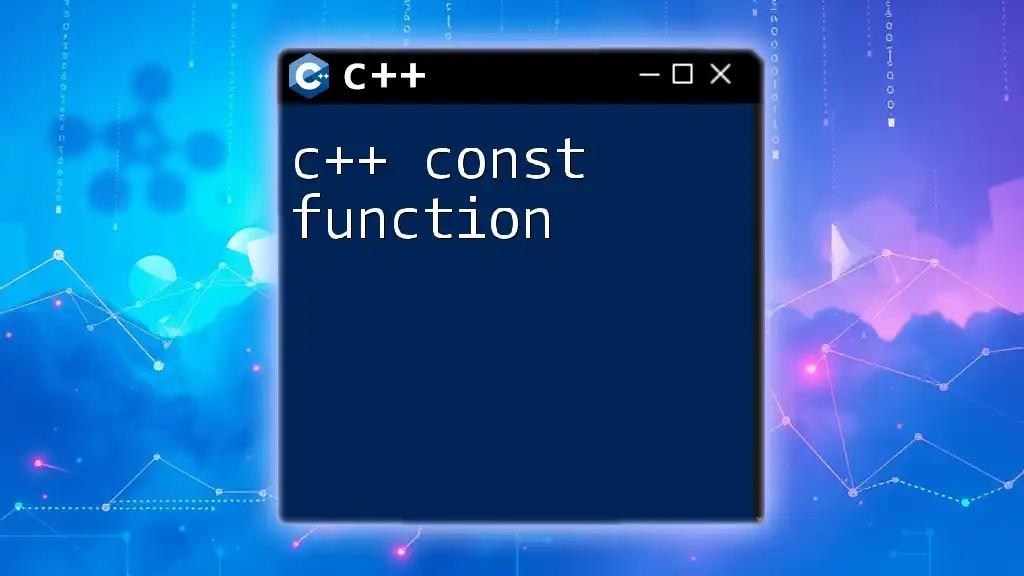
Advanced Use Cases of Find Function
Finding with Custom Data Types
The `find` function can be adapted for use with user-defined data types. This is particularly useful in real-world applications. Consider the following example:
#include <iostream>
#include <vector>
#include <algorithm>
struct Person {
std::string name;
int age;
};
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}};
auto it = std::find_if(people.begin(), people.end(),
[](const Person& p) { return p.name == "Alice"; });
if (it != people.end()) {
std::cout << "Found: " << it->name << " Age: " << it->age << std::endl;
}
return 0;
}
In this example, we define a `Person` struct with `name` and `age`. Using `std::find_if` alongside a lambda function allows sophisticated searches that check multiple attributes or conditions.
Performance Considerations
While the `find` function is a straightforward solution, it executes with O(n) time complexity. Therefore, it's essential to evaluate whether the use of `find` is the best solution for your immediate needs, especially in scenarios where fast element retrieval is necessary. For large datasets, consider using unordered maps or sets, which can provide average O(1) complexity for search operations.
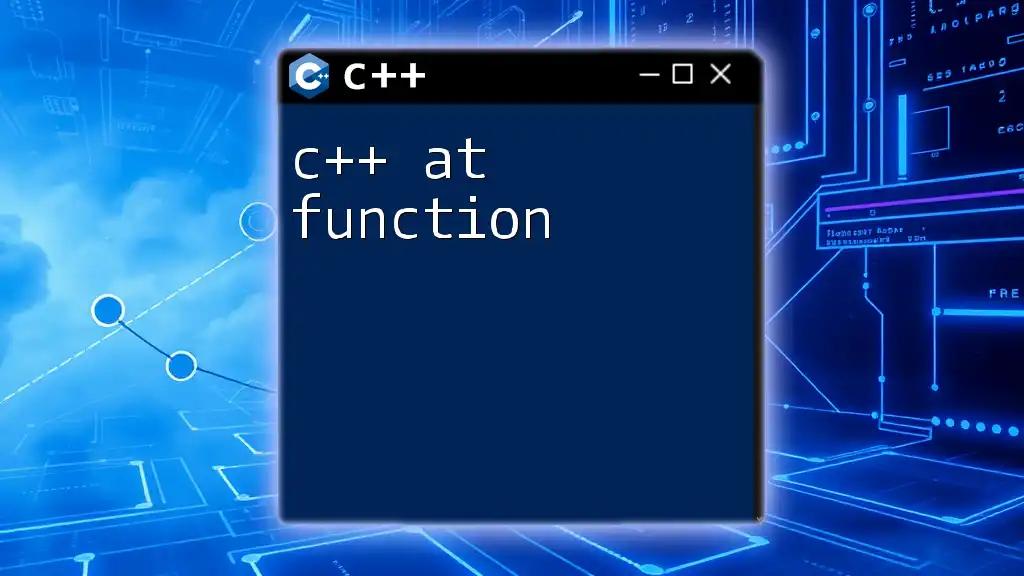
Common Pitfalls and Troubleshooting
Pitfalls of Using Find
Certain pitfalls may occur when using the `find` function:
- Failing to check if the result of `find` is equal to `last` can lead to unexpected behavior, such as dereferencing an invalid iterator.
- Ensuring that the type of the search value is consistent with the type of elements in the container is crucial. Mismatched types can lead to unintentional results or runtime errors.
Debugging Tips
Debugging is an essential skill when using the `find` function. If a value cannot be found:
- Verify the boundaries defined by `first` and `last`.
- Ensure the value's type matches the contained elements.
- Consider deploying logging or breakpoints to observe the flow of your search operation.
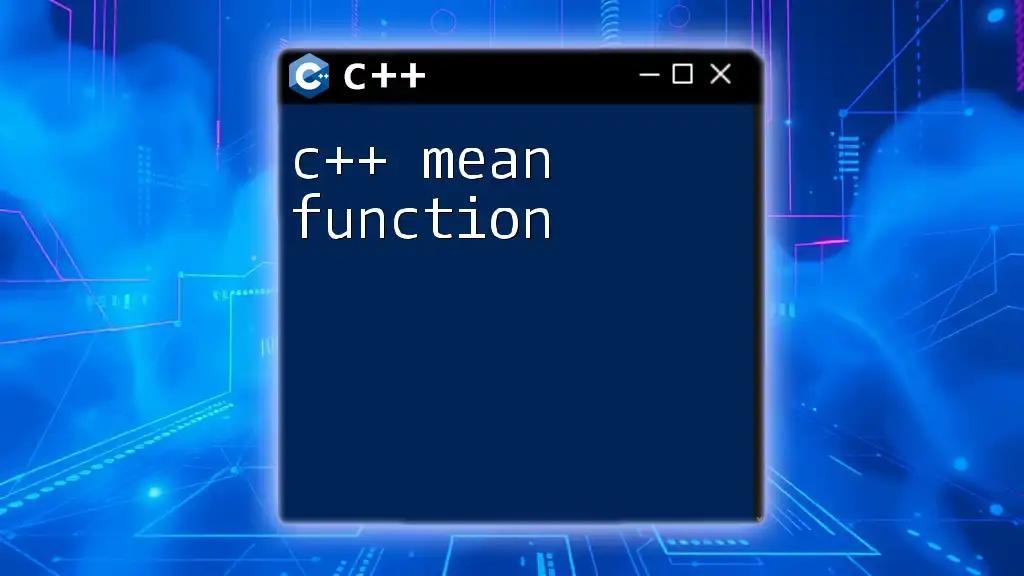
Conclusion
Understanding the C++ find function is essential for searching through various data structures effectively. By practicing its application within vectors, lists, and custom types, you'll increase your competency as a C++ developer. The `find` function is a vital tool in your programming toolkit, and as you explore its functionality, you’ll discover the power it brings to your applications.
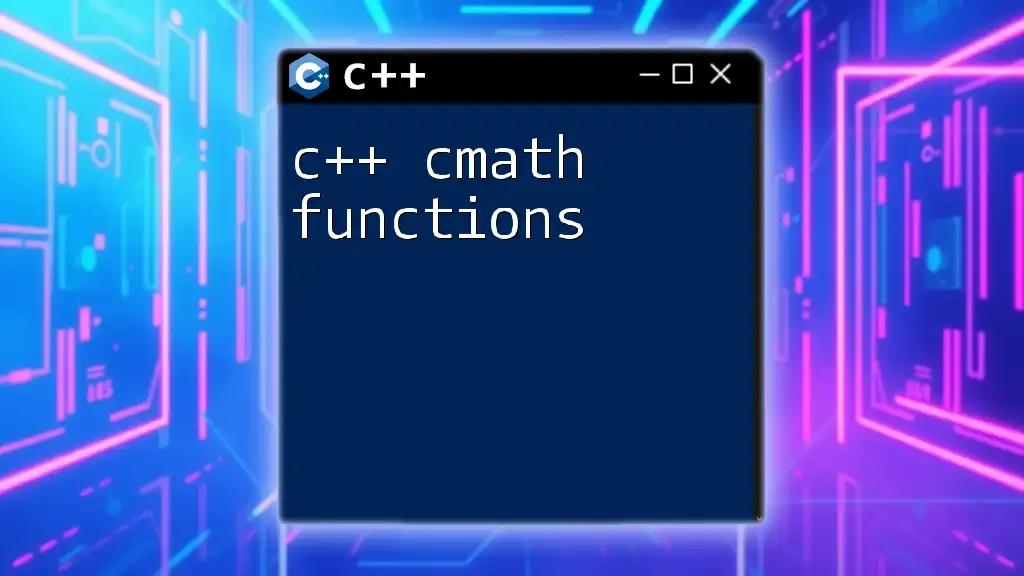
Further Reading
For those eager to deepen their knowledge, consider visiting official documentation or leveraging additional resources such as books, online tutorials, or community forums dedicated to C++ development.