A C++ function prototype is a declaration of a function that specifies its name, return type, and parameters, enabling the compiler to recognize the function before its actual definition.
int add(int a, int b); // Function prototype for adding two integers
What is a Function Prototype in C++?
A C++ function prototype serves as a declaration of a function that specifies its return type, name, and parameter types. It tells the compiler about the function's existence before its actual implementation appears in the code. This is particularly useful in complex programs where functions might be defined after their points of invocation.
Understanding the purpose of function prototypes is crucial in C++. They allow for type checking during calls, enhancing the robustness of your code by preventing mismatch errors.
Benefits of Using Function Prototypes
- Code Organization: Function prototypes help organize code by allowing function definitions to appear after their usage, improving readability.
- Type Safety: They provide compile-time checking of parameter and return types, catching errors before runtime.
- Modularity: By clearly defining interfaces, they foster a modular approach where functions can be easily reused and tested independently.
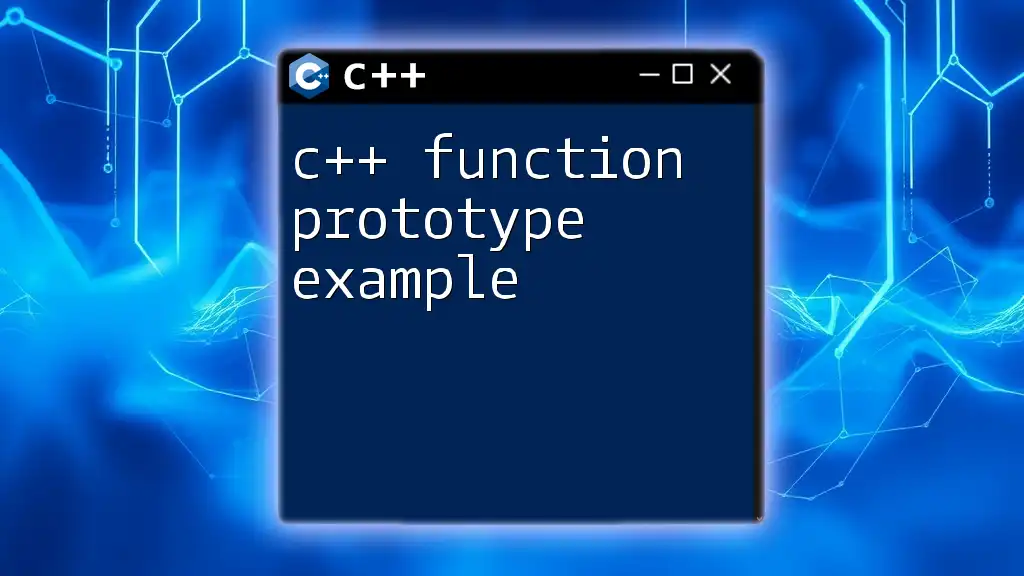
Structure of a Function Prototype
Key Components of Function Prototypes
Every function prototype comprises three main components: return type, function name, and parameter list.
-
Return Type: This indicates what data type the function will return. Common return types include `int`, `float`, `double`, and `void`.
-
Function Name: It’s essential to follow established naming conventions for clarity and to avoid conflicts. Names should be descriptive of the function’s purpose.
-
Parameter List: This includes the data types of the parameters the function accepts, stated in parentheses. Each parameter should have its type specified.
Example:
int add(int a, int b);
In this prototype, `int` is the return type, `add` is the function name, and it takes two integer parameters.
Syntax of Function Prototype in C++
The general syntax of a function prototype in C++ is as follows:
return_type function_name(parameter_type1 parameter1, parameter_type2 parameter2);
Each component contributes to the clear delineation of how the function operates, ensuring that when the function is called, it is in alignment with how it has been declared.
Common errors often stem from forgetting to mention parameter types or mismatching parameter lists between the prototype and the definition.
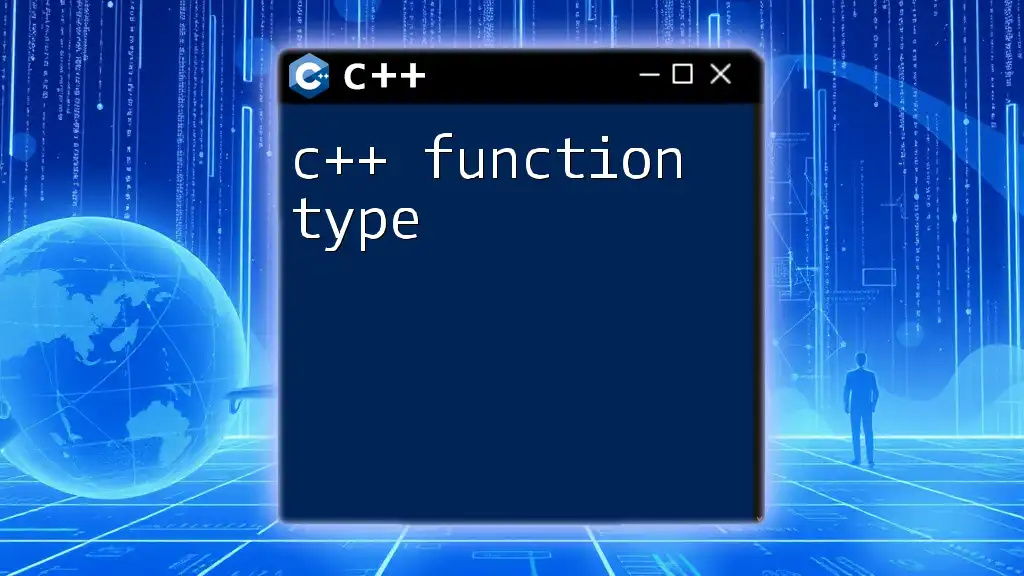
Using Function Prototypes in C++
Why Use Function Prototypes?
Employing function prototypes enhances the organization of your code and aids greatly in debugging. Unlike languages where functions must be defined before they are called, C++ allows for flexibility by letting you declare a prototype before the `main()` function.
How to Implement Function Prototypes
- Declare the Prototype: Before your `main()` function, declare the prototype you intend to use.
- Define the Function Later: After the `main()`, you can flesh out what the function does.
- Call the Function: In the `main()` function or other functions, you can invoke the operation defined by your prototype.
Example of Function Prototype Declaration and Implementation:
// Function prototype
void displayHello();
// Function definition
void displayHello() {
std::cout << "Hello, World!" << std::endl;
}
Calling Functions with Prototypes
Call the function just as you would with any defined function. The prototype ensures that the compiler knows about the function’s existence and checks types accordingly.
Example:
int main() {
displayHello();
return 0;
}
The separation of declaration and definition provides flexibility while maintaining clarity.
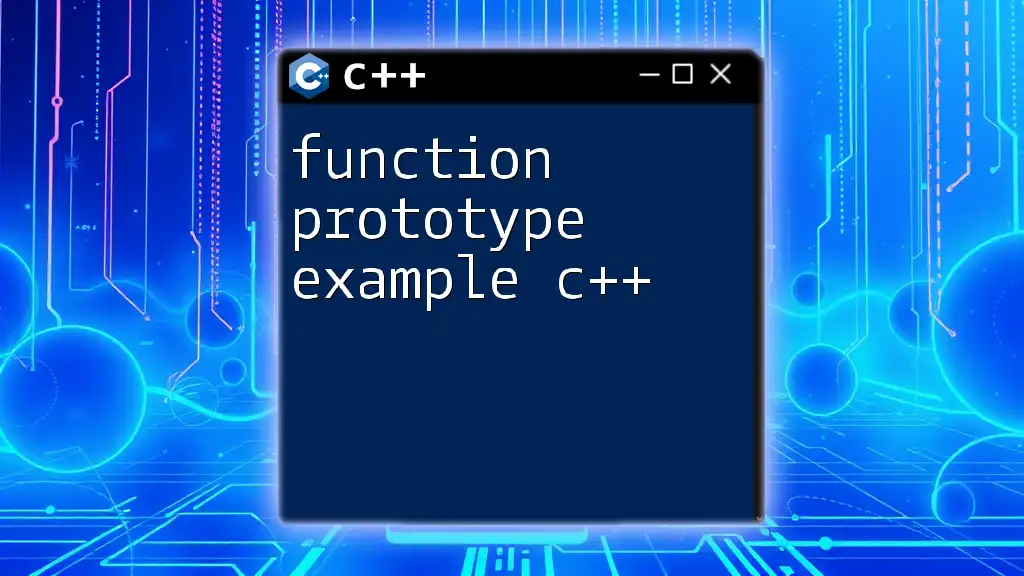
Function Prototypes in Action
A Comprehensive Example
Let’s consider a practical example that demonstrates the utility of function prototypes in a complete program.
#include <iostream>
// Function prototype
int multiply(int x, int y);
// Function definition
int multiply(int x, int y) {
return x * y;
}
int main() {
int result = multiply(5, 10);
std::cout << "The result is: " << result << std::endl;
return 0;
}
This program starts with the function prototype for `multiply`, followed by its definition and invocation in `main()`. Notice how the prototype allows us to call `multiply` before its actual implementation.
Common Mistakes with Function Prototypes
Mistakes can occur when defining a function prototype. For example, forgetting to specify a parameter type can lead to compilation errors.
Common Mistake Example:
// Incorrect prototype (missing parameter type)
void calculate(x, int y); // Error: x is a missing type
Ensure that every parameter has an explicitly stated type to avoid ambiguity.
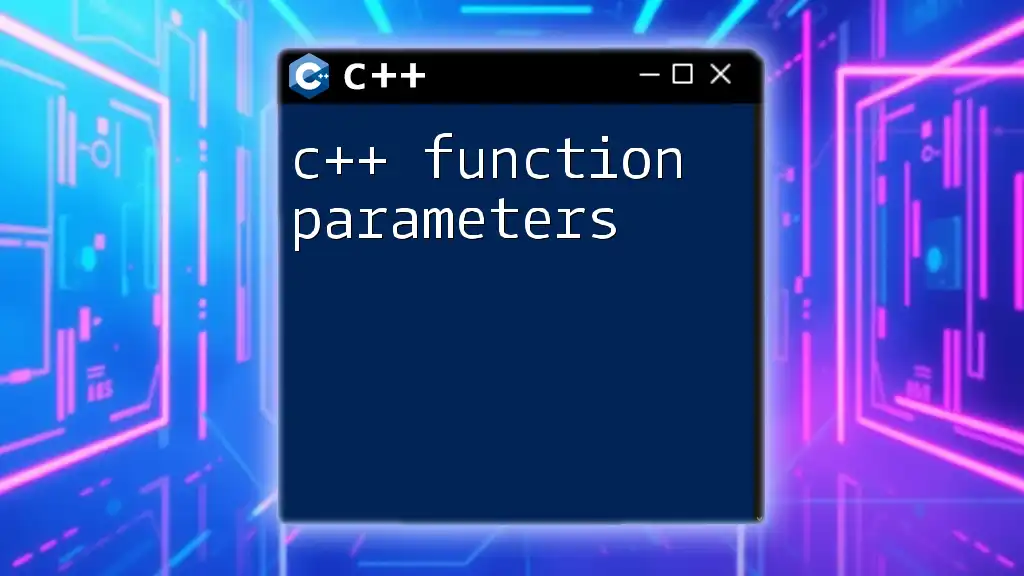
Advanced Topics in Function Prototyping
Overloading Functions with Prototypes
C++ allows for function overloading, where multiple functions can share the same name but have different parameter lists. In this case, you must define a prototype for each variant.
Example of Overloaded Function Prototypes:
int add(int a, int b);
double add(double a, double b);
Each `add` function behaves similarly but operates on different data types. The compiler distinguishes them based on their prototypes.
Function Templates as Prototypes
Function templates offer yet another layer of versatility. They allow you to write a single function prototype that can work with various data types.
Example:
template <typename T>
T add(T a, T b);
Using `T` as a placeholder allows you to create a function that can accept any data type.
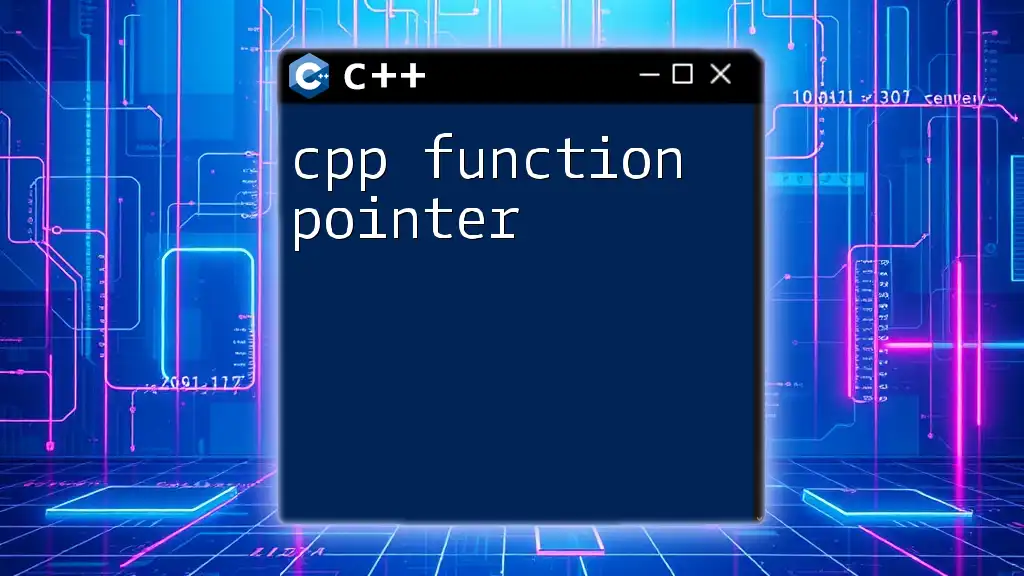
Prototyping in C++ Best Practices
Tips for Effective Function Prototypes
- Maintain clear naming conventions to enhance code readability and avoid conflicts.
- Ensure that function prototypes are synchronized with function definitions to prevent mismatch errors.
- Use comments to provide context for complex functions, simplifying future edits.
Frequently Asked Questions
-
What is the difference between function declaration and function prototype? A function declaration specifies the function’s name and parameters but may lack a complete prototype's format, while a prototype gives a complete overview inclusive of return type.
-
Can function prototypes be used with classes? Yes, function prototypes apply to member functions defined in classes.
-
How do function prototypes interact with namespaces? Function prototypes can exist within namespaces to organize code better. Ensure to use the proper namespace prefix while calling them.
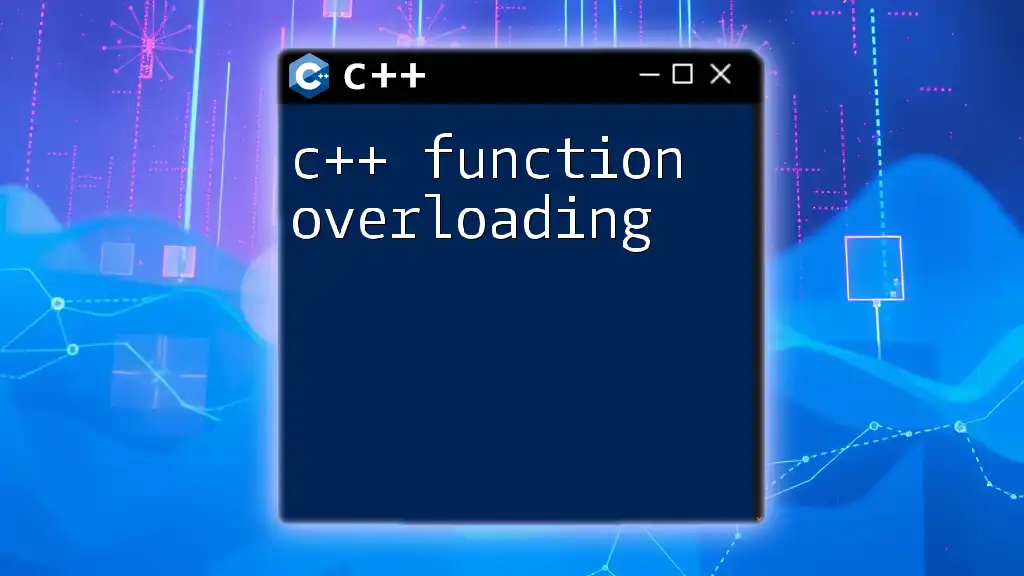
Conclusion
Understanding C++ function prototypes not only adds to your programming toolkit but enhances your coding proficiency overall. They streamline function declarations, enable type safety, and promote better organization in your code.
As you delve deeper into C++, take the time to practice creating and utilizing function prototypes in your projects. Share your experiences, questions, or examples in the comments below!