A function prototype in C++ is a declaration of a function that specifies its name, return type, and parameters, but does not include the function body, allowing the compiler to understand the function's interface before it's defined.
int add(int a, int b); // Function prototype
What is a Function Prototype in C++
A function prototype is a declaration of a function that specifies the function's name, return type, and parameters, but does not provide the body or implementation of the function. It serves as a promise to the compiler that the function will be defined later in the program, allowing other functions to call it before its actual definition appears in the code. Understanding what a function prototype is crucial for effective programming in C++.

Purpose of Function Prototypes
Code Organization
Function prototypes aid in organizing code by allowing you to separate the declarations of functions from their definitions. This separation is particularly important in larger programs where function definitions might be lengthy or potentially complex. By declaring a function prototype at the beginning of your code, you can maintain a clear and understandable structure.
Compilation Understanding
Function prototypes inform the compiler about the function’s signature, enabling proper compilation of the code. When the main function or any other function calls a user-defined function, the prototype ensures that the compiler understands what to expect regarding the return type and parameters, even if the actual function implementation is located later in the code.

Syntax of Function Prototypes
The general format of a function prototype in C++ is as follows:
return_type function_name(parameter_type1 parameter_name1, parameter_type2 parameter_name2);
Breaking this down:
- Return Type: Specifies the type of value the function will return (e.g., `int`, `float`, `void`).
- Function Name: The name of the function which should be descriptive of its action, following standard naming conventions.
- Parameters: Lists the types and names of each parameter the function will accept.
Example of Function Prototype
Consider the function prototype for an addition function:
int add(int a, int b);
In this example, `int` is the return type, `add` is the function name, and it takes two integer parameters `a` and `b`. This prototype informs the compiler of what to expect when the `add` function is called elsewhere in the code.

Importance of Function Prototypes
Enhancing Readability
Function prototypes significantly enhance the readability and maintainability of code. By having all the function prototypes declared at the beginning, developers can quickly glance at the available functions, their purposes, and their inputs without wading through lengthy implementation details.
Supporting Forward Declarations
Forward declarations — which allow functions to be called before they are defined — are enabled by function prototypes. This is especially crucial in cases where a function needs to call another function that is defined later in the code.
Example of Using Function Prototypes
Here's an example to illustrate the concept:
#include <iostream>
void greet(); // Function prototype
int main() {
greet(); // Calling the function
return 0;
}
void greet() { // Function definition
std::cout << "Hello, welcome to C++ programming!" << std::endl;
}
In this code, the `greet` function is called in `main`, despite its definition appearing afterward. The prototype `void greet();` allows the compiler to recognize and correctly process this function call.
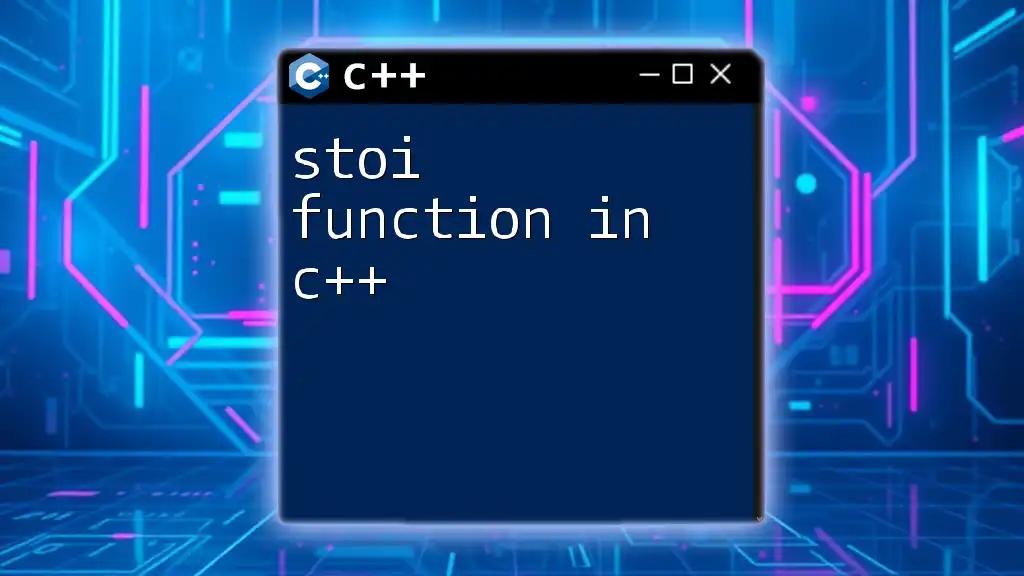
Calling Functions with Prototypes
How to Declare and Define Functions
When a function is declared with a prototype, it is essential that its definition matches the prototype exactly. Any mismatch can lead to compilation errors or unexpected behavior.
Example of Declaration and Definition
Here’s another illustrative example:
float calculateArea(float radius); // Prototype
float calculateArea(float radius) { // Definition
return 3.14 * radius * radius;
}
In this code, the prototype and definition match perfectly, ensuring that calls to `calculateArea` work correctly throughout the program.
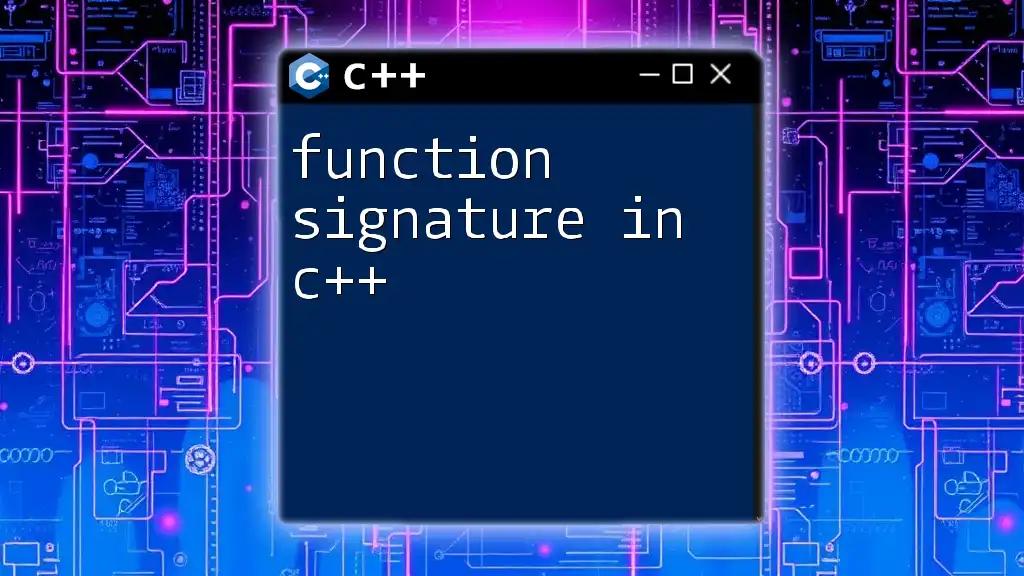
Common Mistakes with Function Prototypes
Forgetting to Use Prototypes
One common pitfall is forgetting to use function prototypes altogether. Omitting them can lead to compilation errors, especially if the function calls precede their definitions. The compiler will not recognize the function's existence, causing it to generate an error.
Mismatched Function Signatures
Another mistake occurs when there is a mismatch between the prototype and the function definition. For example:
int multiply(int a, int b); // Prototype
float multiply(int a, int b) { // Mismatched definition
return a * b;
}
In the above code, the return type of the prototype is `int`, but the definition incorrectly states `float`. This mismatch will lead to a compilation error.
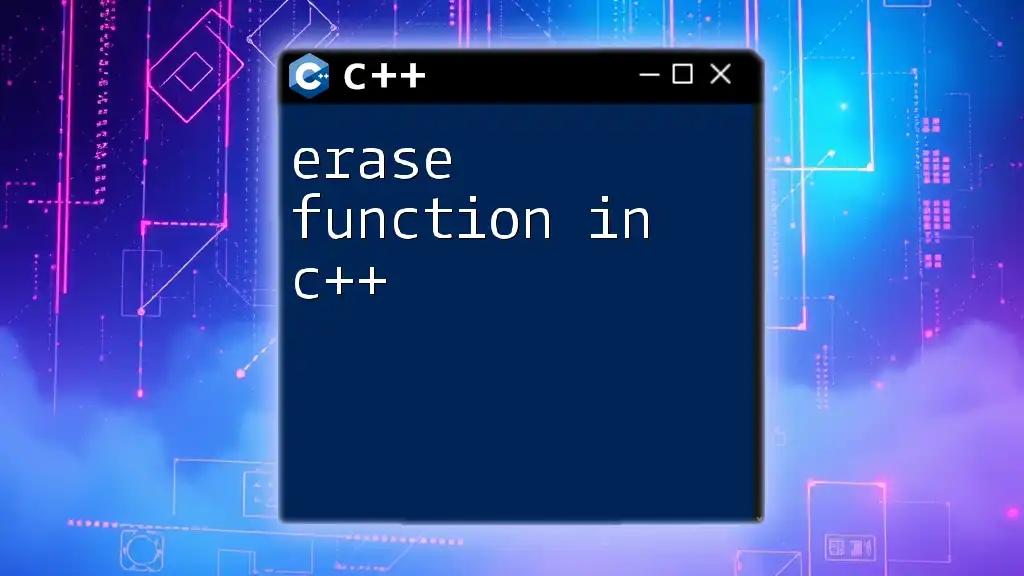
Additional Considerations
Function Overloading
Function overloading allows multiple functions to have the same name, provided their parameter lists are different. In such a scenario, prototypes are essential to ensure that the compiler understands which version of the function is being called based on arguments passed.
Default Arguments
Function prototypes can also handle default arguments, allowing programmers to specify default values for parameters that may not always be required. For example:
void printMessage(std::string message = "Hello, World!"); // Prototype with a default argument
In this case, if the `printMessage` function is called without any arguments, it will default to displaying "Hello, World!".
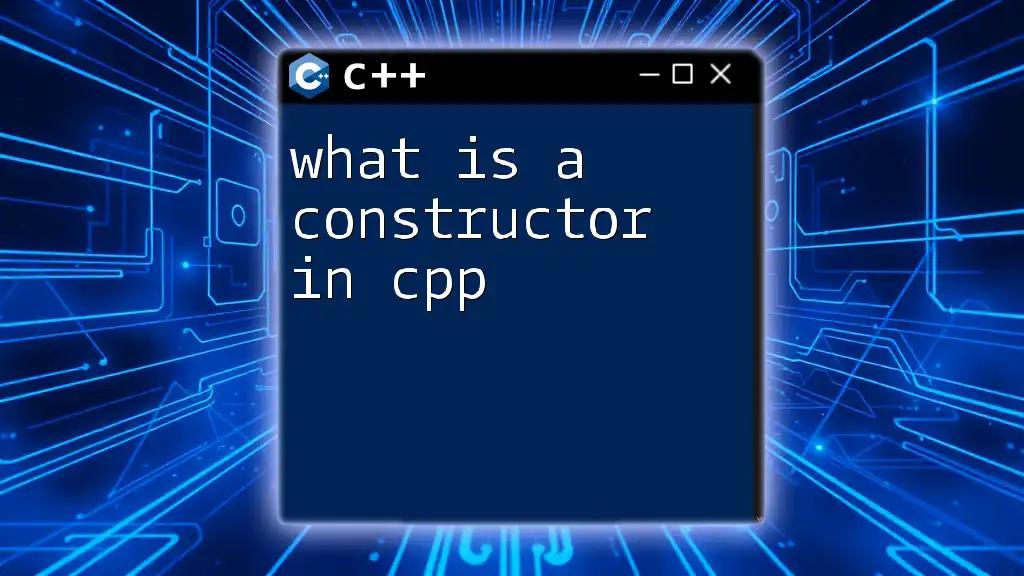
Conclusion
In summary, understanding what a function prototype in C++ is and how to use it effectively is integral to writing clear, organized, and efficient C++ programs. Function prototypes allow code to be structured logically, enhance readability, and simplify the compilation process. By employing function prototypes, you can improve the maintainability of your codebase while avoiding common errors associated with function definitions and calls.
Engage with the challenge of mastering function prototypes in C++, and explore how they can bolster your coding practices. Be sure to practice creating and using function prototypes as you advance your skills in C++.