In C++, function parameters are variables listed as part of a function's definition that allow you to pass values into the function when it is called.
#include <iostream>
void greet(const std::string &name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
greet("Alice");
return 0;
}
Understanding Function Parameters
Function parameters are integral components of functions in C++. They allow functions to operate on different inputs, making them versatile and reusable. In C++, multiple types of function parameters can be defined, each with its own purpose and behavior.
Definition of Function Parameters
A function parameter is a variable listed within parentheses in a function's definition. They serve as placeholders for the actual values (or arguments) that can be passed to the function when it is called. It's important to understand that while parameters are specified when a function is created, arguments are the actual values supplied to those parameters when the function is invoked.
Types of Function Parameters
C++ supports several types of function parameters, each with specific use cases that can affect performance, memory usage, and functionality.
Value Parameters
Value parameters are the most straightforward type. When a function is defined with value parameters, a copy of the argument is made, enabling the function to work with that copy. Modifying the parameter within the function does not affect the original argument outside of it.
Here’s a simple example of value parameters:
#include <iostream>
void add(int a, int b) {
a += b; // modifies the copy of a
std::cout << "Inside function: " << a << std::endl;
}
int main() {
int x = 5, y = 10;
add(x, y);
std::cout << "Outside function: " << x << std::endl; // x remains unchanged
return 0;
}
In this example, the output will show that the value of `x` remains unchanged after calling the `add` function, demonstrating that the value was passed by copy.
Reference Parameters
Reference parameters allow functions to operate directly on the original argument rather than a copy. This means that if the parameter is modified within the function, the original argument is also affected. To declare a reference parameter, use an ampersand (`&`) before the parameter name.
Here’s an example:
#include <iostream>
void add(int &a, int &b) {
a += b; // modifies the original a
}
int main() {
int x = 5, y = 10;
add(x, y);
std::cout << "After function: " << x << std::endl; // x is now modified
return 0;
}
In this instance, `x` will be updated to `15` after the function call, demonstrating the impact of reference parameters.
Pointer Parameters
Pointer parameters enable a function to directly access and modify the value stored at a particular memory address. When a pointer is used as a parameter, the function can change data, making it a powerful but complex option.
See this code example:
#include <iostream>
void increment(int *ptr) {
(*ptr)++; // increments the value at the address
}
int main() {
int x = 5;
increment(&x);
std::cout << "After function: " << x << std::endl; // x is now 6
return 0;
}
Using pointer parameters can be particularly useful for handling dynamically allocated memory or when you need to return multiple values from a function.
Default Parameters
Default parameters in C++ allow a function to be called with fewer arguments than it is defined to accept. This is useful for providing flexibility in how functions can be utilized. When a default parameter is specified in the function declaration, if no argument is passed for that parameter, the default value is utilized.
Example:
#include <iostream>
void greet(std::string name = "Guest") {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
greet(); // Outputs: Hello, Guest!
greet("Alice"); // Outputs: Hello, Alice!
return 0;
}
In this example, the function `greet` has a default parameter, resulting in different behaviors based on the presence of an argument.
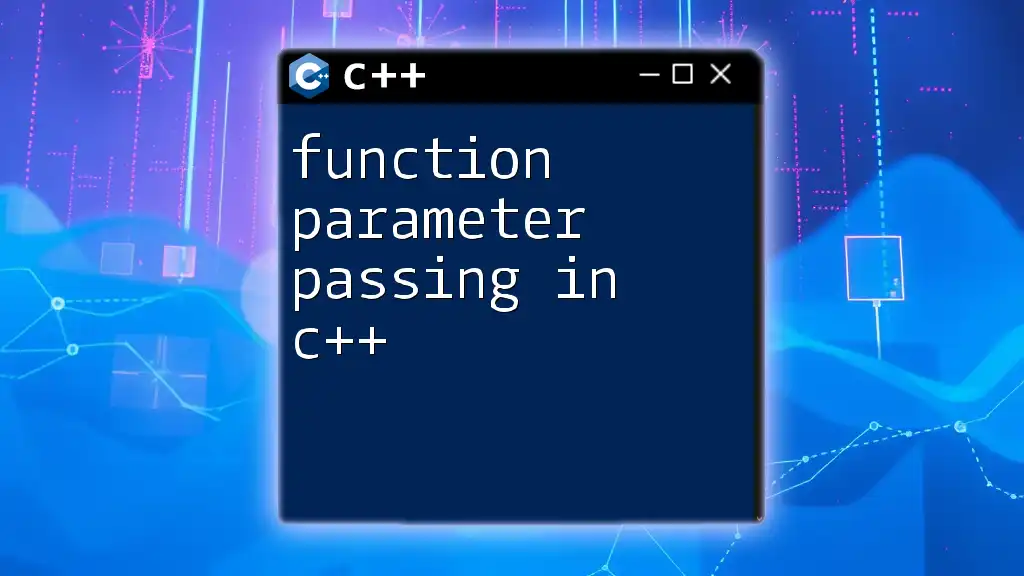
Function Parameter Variants
Function Overloading
Function overloading allows multiple functions to have the same name but different parameter lists (in terms of type or number) within the same scope. This capability is particularly useful for providing various ways to perform similar tasks without cluttering your code with different function names.
Example of function overloading:
#include <iostream>
void print(int i) {
std::cout << "Integer: " << i << std::endl;
}
void print(double d) {
std::cout << "Double: " << d << std::endl;
}
int main() {
print(5); // Calls the first function
print(3.14); // Calls the second function
return 0;
}
Template Parameters
C++ templates allow functions to operate with any data type. This flexibility means you can write generic functions that work with various types without rewriting the same logic for each type.
Here’s an example of a function with template parameters:
#include <iostream>
template <typename T>
void show(T val) {
std::cout << "Value: " << val << std::endl;
}
int main() {
show(42); // Works with int
show(3.14); // Works with double
show("Hello"); // Works with const char*
return 0;
}
Templates promote code reusability and type safety, making them a powerful tool in C++ programming.
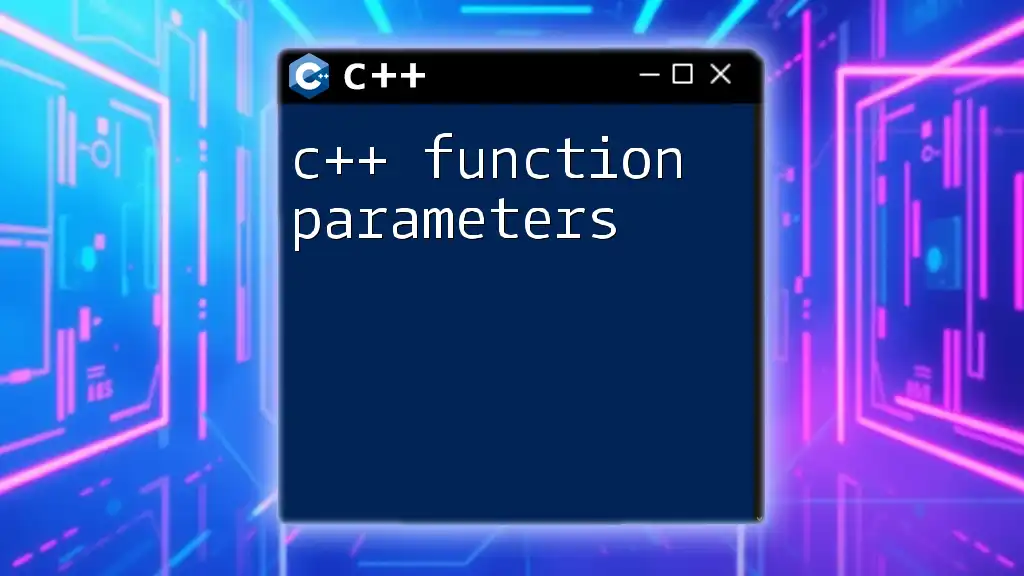
Best Practices for Using Function Parameters
Consistency and Readability
Consistency in naming conventions for parameters enhances code readability. Using meaningful names provides context for each parameter’s purpose, helping others (or your future self) understand the code more quickly.
Avoiding Common Pitfalls
Common mistakes with function parameters include unintended side effects and ignoring const-correctness. Always ensure that functions do not modify data unintentionally unless that is the desired behavior.
Parameter Passing Strategies
Choosing between value and reference or pointer parameters often hinges on performance and the function's requirements. Generally, larger data types (like structs or classes) are better passed by reference to avoid copying overhead, while primitive types can generally be passed by value efficiently.
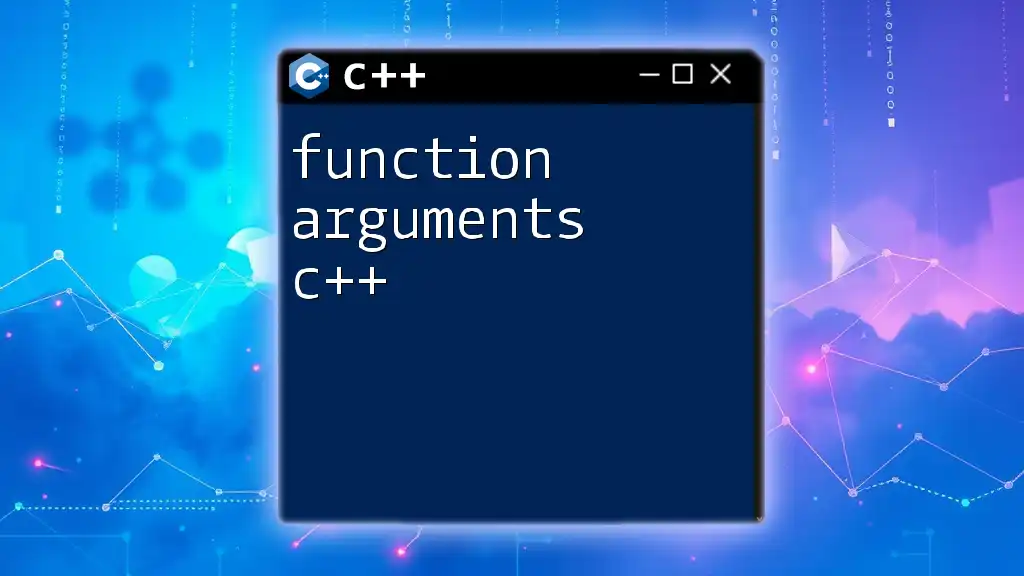
Conclusion
Understanding function parameters in C++ is essential for writing robust, flexible, and efficient code. By leveraging different types of parameters, such as value, reference, and pointer parameters, as well as utilizing advanced features like default parameters and function overloading, programmers can create cleaner, more maintainable code.
In your journey through C++, continue to practice by implementing these concepts in your functions, as hands-on experience is the best way to cement your understanding.