A function macro in C++ is a preprocessor directive that defines a reusable piece of code that can replace a function call with its defined content, allowing for inline code substitution.
Here's an example:
#define SQUARE(x) ((x) * (x))
#include <iostream>
int main() {
int num = 5;
std::cout << "The square of " << num << " is " << SQUARE(num) << std::endl;
return 0;
}
What are Function Macros?
Function macros in C++ are predefined commands that allow you to create reusable code snippets. They help enhance the efficiency of your code, making it easier to maintain and less prone to errors. Function macros are processed by the preprocessor before the compilation of your code, which allows for powerful text manipulation.
Difference Between Function Macros and Functions
While both function macros and functions can be used to encapsulate behavior, they differ significantly in operation:
- Performance: Macros are expanded inline, meaning that there is no overhead associated with function calls. This can lead to faster execution times but can also result in code bloat.
- Use Cases: Functions provide type safety and automatic parameter evaluation, making them preferable in most scenarios. Use function macros primarily for constants or simple repeated expressions where function calls are not appropriate.
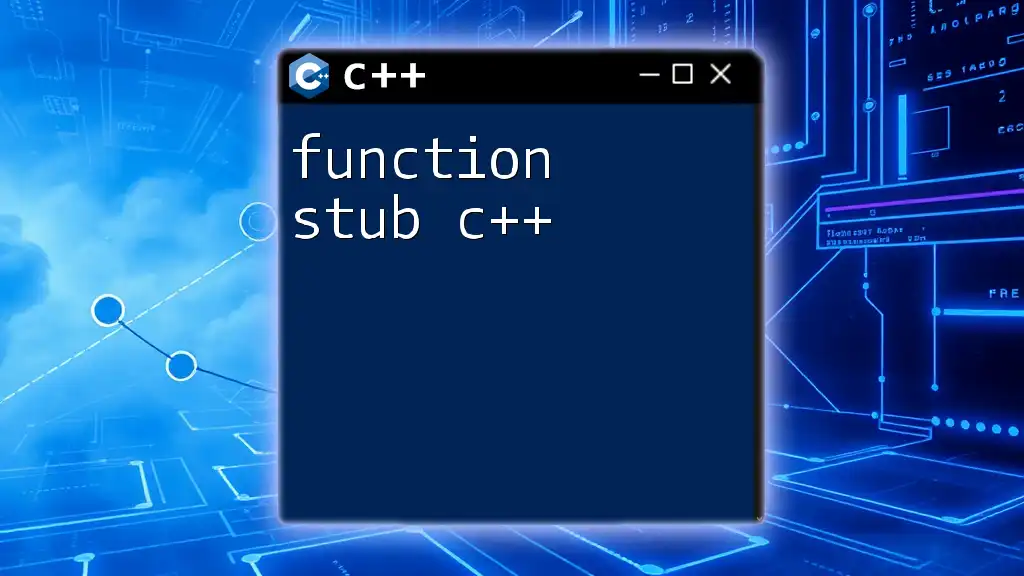
What is a Macro Definition in C++?
A macro definition in C++ defines a token or a sequence of tokens that will be replaced by a specific code segment before the actual compilation process begins. Macros offer a flexible way to insert code snippets without writing redundant code.
Standard Syntax for Macro Definition
The syntax for defining a macro is straightforward:
#define MACRO_NAME(value)
Example:
#define SQUARE(x) ((x) * (x))
This macro will replace any call to `SQUARE(x)` with `((x) * (x))`, allowing you to calculate the square of a number without having to write a separate function.
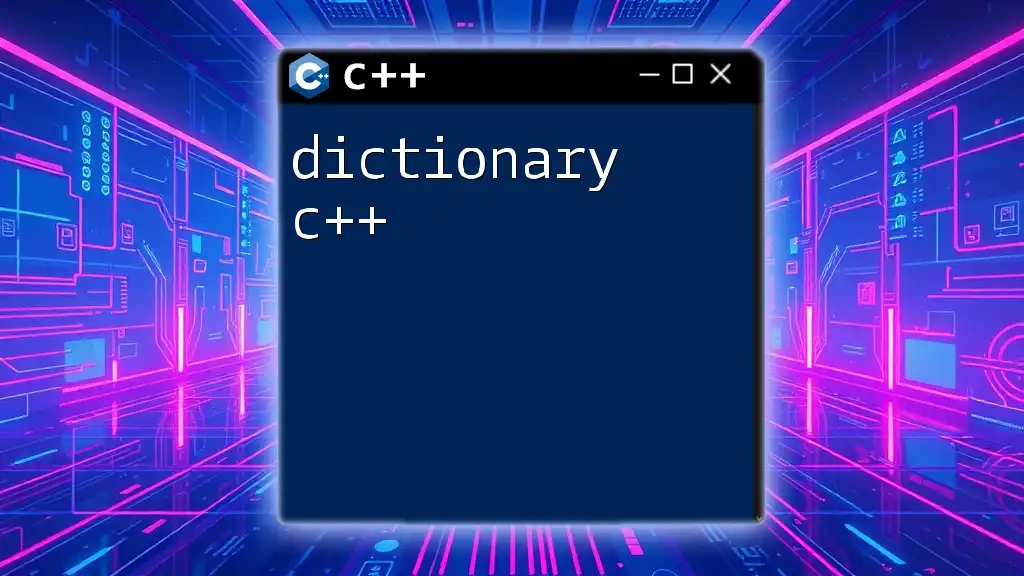
Creating Function Macros
Defining a Function Macro
Creating a function macro is as simple as defining a standard macro. Here’s a step-by-step guide to creating one:
- Use the `#define` preprocessor directive.
- Choose a unique name for your macro.
- Specify the parameters needed by your macro.
- Write the expression you want to return.
Example of a Function Macro
Consider the following example for calculating the maximum of two values:
#define MAX(a, b) ((a) > (b) ? (a) : (b))
In this case, anytime `MAX(a, b)` is invoked, it will be replaced by the conditional expression `((a) > (b) ? (a) : (b))`.
Explanation of the Example
This simple yet powerful macro compares two values and returns the larger one, leveraging the ternary operator. Note, however, that `a` and `b` are evaluated twice if they are complex expressions, leading to potential unwanted side effects.
Common Pitfalls with Function Macros
While function macros can be handy, they are fraught with potential issues:
-
Multiple Evaluations of Parameters: If a parameter is an expression, it can inadvertently be evaluated more than once. For example, if you define a macro as `#define DOUBLE(x) ((x) + (x))`, and you call `DOUBLE(i++)`, `i` will increment twice.
-
Lack of Type Checking: Macros do not perform type checking, which could lead to unexpected behavior if used with the wrong data types.
Example to Illustrate Each Pitfall
Consider the example of inappropriate use of macros:
#define INCREMENT(x) ((x) + 1)
int i = 5;
int result = INCREMENT(i++); // i is incremented twice!
In this case, `result` would not only be 6 but could lead to confusion as `i` will be 7 due to double evaluation.
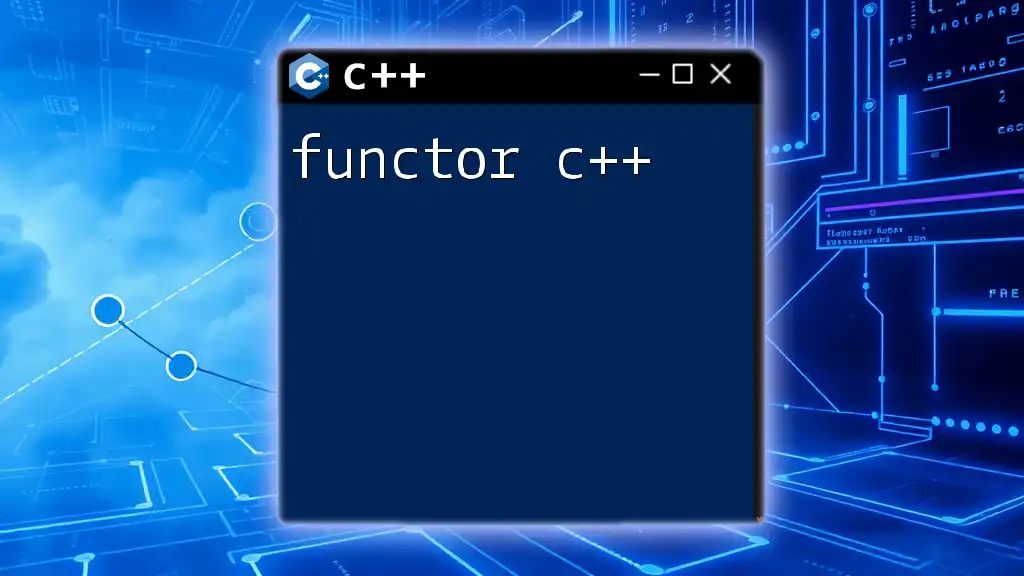
Best Practices for Using Function Macros
When to Use Function Macros
Function macros are most effective in the following situations:
- When you require simple repetitive expressions.
- When performance is critical and function call overhead is unacceptable.
Avoiding Unintended Side Effects
To avoid common problems with macros, consider the following strategies:
- Enclose Parameters in Parentheses: Always use parentheses around parameters and the entire macro body to avoid precedence issues.
Example:
#define SQUARE(x) ((x) * (x)) // Safer than #define SQUARE(x) x*x
Debugging Function Macros
If you encounter issues with macros, consider using `#undef` to remove the macro definition for a clean slate. This allows you to redefine the macro without losing focus on the resulting behavior.
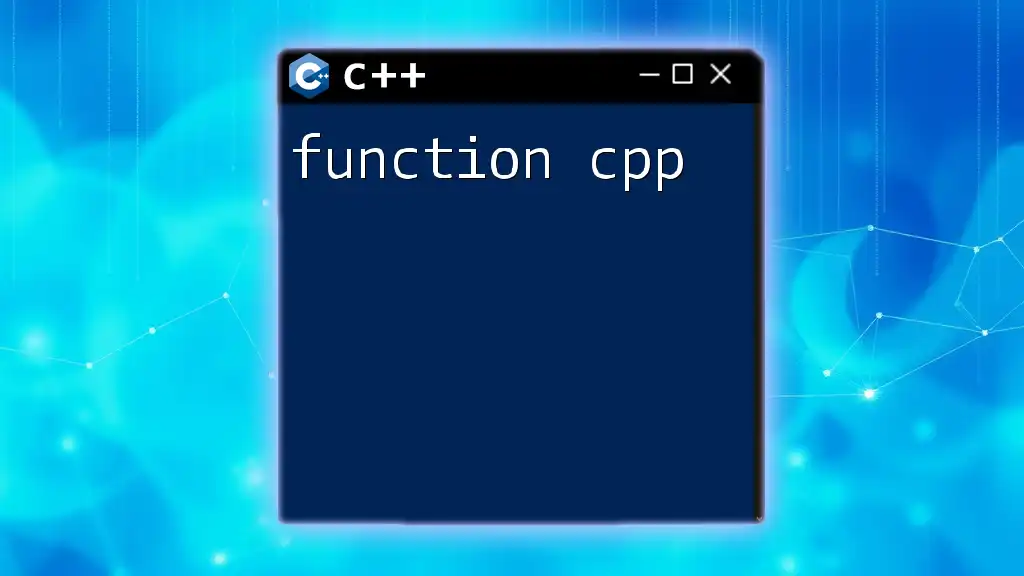
Advanced Concepts in Function Macros
Variadic Macros
Variadic macros allow you to accept a variable number of arguments, making your macros more flexible. The syntax for defining a variadic macro is as follows:
#define MACRO_NAME(...)
Example:
#define LOG(...) printf(__VA_ARGS__)
This macro can be used to log messages with varying numbers of parameters, providing a dynamic logging mechanism.
Macro Concatenation and Stringization
C++ macros also support advanced features like concatenation and stringization:
-
Concatenation: Use `##` to combine tokens.
#define CONCAT(a, b) a##b
-
Stringization: Use `#` to convert a token into a string.
#define TO_STRING(x) #x
These features make macros incredibly powerful, but they must be used judiciously to avoid confusion.
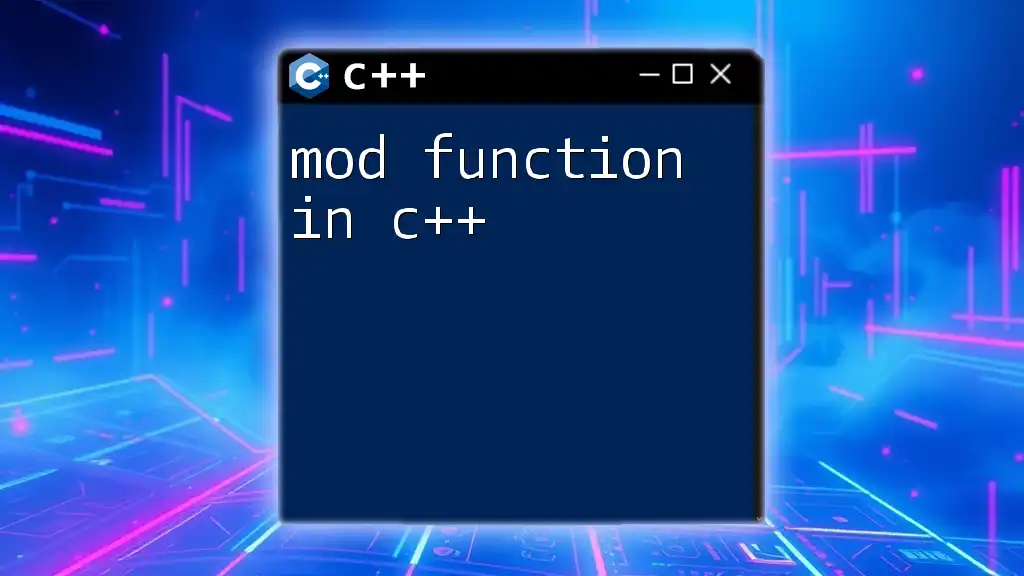
Comparison of Function Macros to Inline Functions
Definitions and Use Cases
Inline functions provide a safer and often more performant alternative to function macros by offering:
- Type Safety: Inline functions take advantage of C++'s type system to ensure parameters are of the expected types.
- No Multiple Evaluations: Inline functions evaluate expressions only once, preventing the unexpected behavior exhibited in macros.
Code Example Highlighting Differences
// Function Macro
#define CUBE(x) ((x) * (x) * (x))
// Inline Function
inline int cube(int x) { return x * x * x; }
The function `cube(int x)` is more robust than the macro `CUBE(x)`, providing better safety and maintainability.
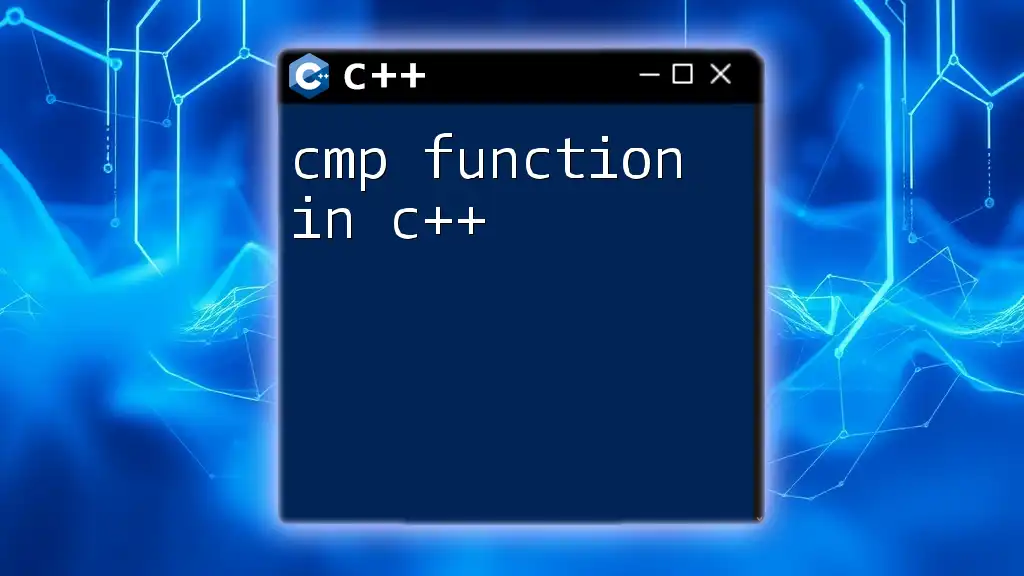
Real-World Applications of Function Macros
Common Use Cases of Function Macros in C++
Function macros are often seen in code for logging, debugging, and configuration.
For instance, a logging macro can simplify debugging by automatically adding file name and line number:
#define LOG(msg) printf("%s:%d: %s\n", __FILE__, __LINE__, msg)
Case Study or Example Project
Imagine a project that utilizes function macros across a large codebase to manage configuration settings. By defining macros for common settings and options, the code remains clean, and updates to these settings can be made in one centralized location.
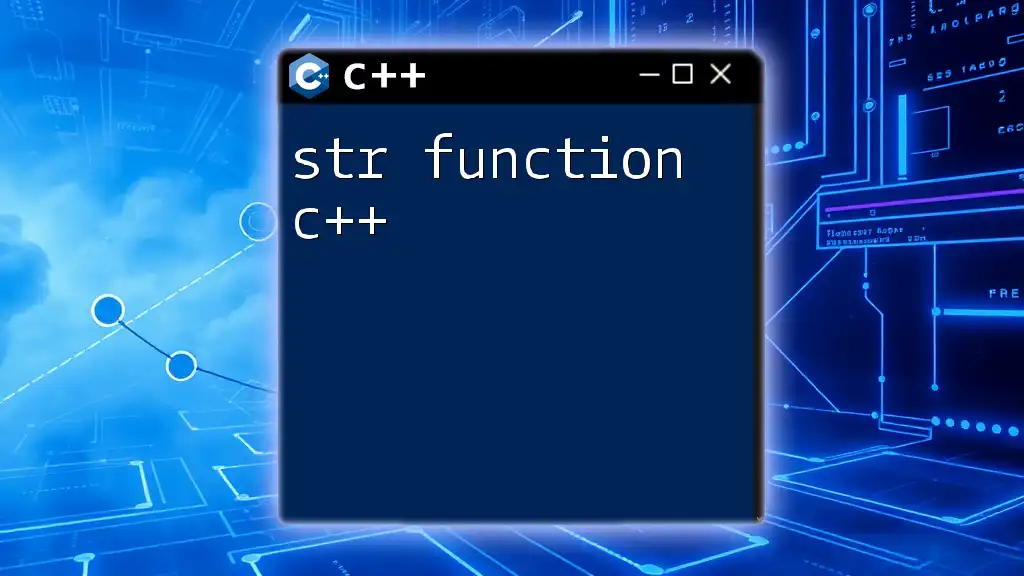
Conclusion
In conclusion, function macros in C++ are a powerful tool for any programmer when utilized correctly. Understanding their mechanisms, pitfalls, and best practices can greatly enhance your coding efficiency and effectiveness. However, it is essential to weigh the benefits against potential downsides and consider alternative solutions like inline functions when appropriate.
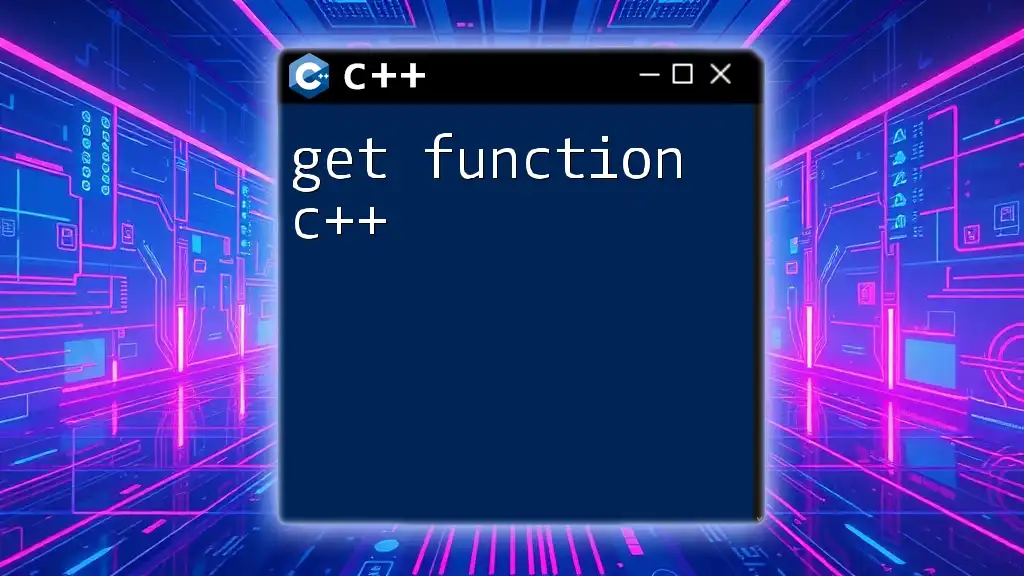
Additional Resources
For further learning, a wealth of resources is available:
- Books and Tutorials: Consider reading authoritative texts on C++ optimization and macros.
- Online Courses: Platforms like Coursera or Udacity offer courses focused on C++ programming.
- Community and Forums: Engage with communities on platforms like Stack Overflow or Reddit to ask questions and share insights with fellow developers.