The `mod` function in C++ is used to obtain the remainder of the division of two integers, which can be implemented using the modulo operator `%`.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b; // result will be 1
std::cout << "The remainder of " << a << " divided by " << b << " is " << result << std::endl;
return 0;
}
Understanding the Mod Function
What is the Mod Function?
The mod function in C++, often represented by the operator `%`, is used to calculate the remainder of a division operation. When you divide two integers, the mod function returns the amount left over after the division is complete. For example, when you divide 10 by 3, you get 3 with a remainder of 1. Therefore, `10 % 3` equals 1. This operation is pivotal for various programming tasks and algorithms, especially those involving cycles, parity checks, and data structure manipulations.
Mathematical Background of Modulus
In mathematics, the modulus operation finds a prominent place in number theory. It is used to determine the remainder of a division operation. For instance, \( a \mod b \) returns the remainder when \( a \) is divided by \( b \). This concept applies widely in real-life scenarios, such as scheduling, cryptography, and computer graphics, where periodic or cyclic behaviors are crucial.
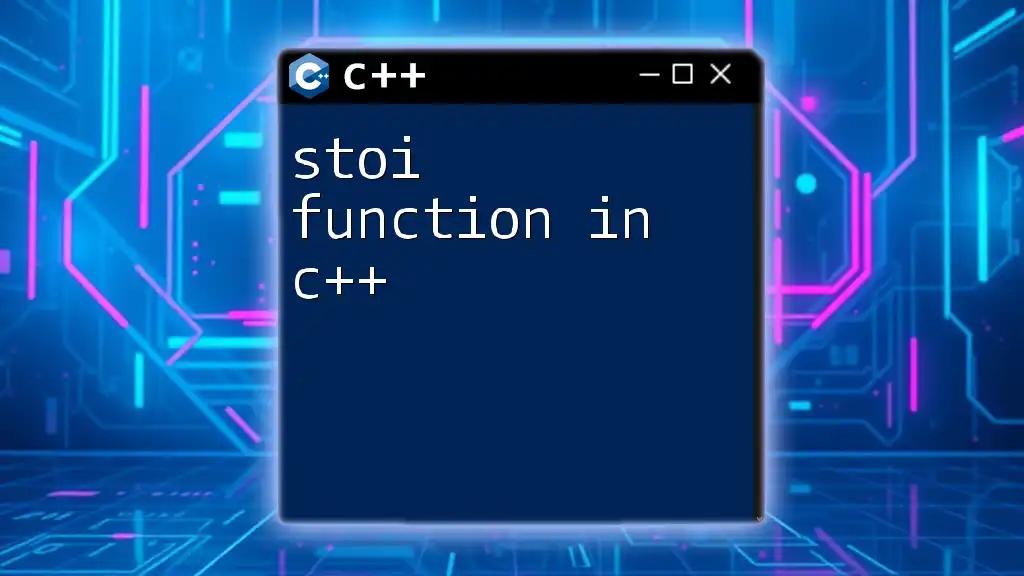
Using the Modulus Operator in C++
Introduction to the Modulus Operator
In C++, the modulus operator `%` serves as a straightforward and efficient way to find remainders. It is crucial for many applications, including but not limited to algorithm development and conditional checks.
Syntax of the Modulus Operator
Using the modulus operator requires a simple syntax structure where you place the two integers you want to divide on either side of the `%` symbol. For example:
int result = 10 % 3; // Result will be 1
In this example, `result` stores the value of the remainder when 10 is divided by 3.
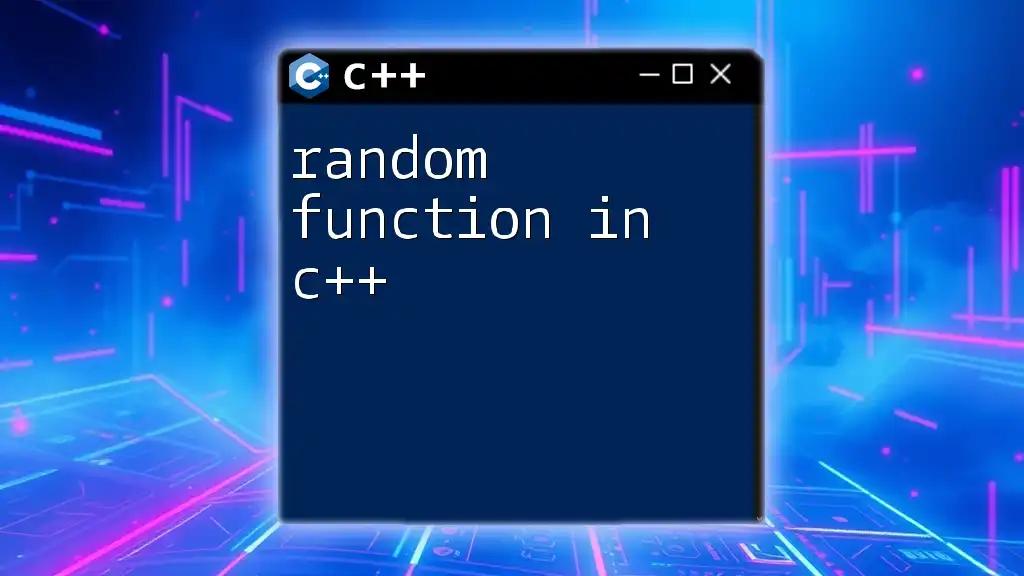
Practical Applications of Modulus in C++
Checking Even or Odd Numbers
One of the simplest yet practical uses of the mod function in C++ is to determine if a number is even or odd. By checking if a number gives a remainder of 0 when divided by 2, you can ascertain its parity.
int number = 4;
if (number % 2 == 0) {
std::cout << "Even";
} else {
std::cout << "Odd";
}
This snippet will output "Even" for the value of 4. If you input an odd number, it will print "Odd".
Circular Array Indexing
Another interesting application of the modulus operator is in circular array indexing. When you want to access elements in a cyclic manner, the modulus operator helps wrap around the indices. For instance:
int arr[] = {1, 2, 3, 4, 5};
int index = 7;
int circularIndex = index % 5; // Result will be 2
In this case, `circularIndex` will be 2, allowing you to access the 3rd element of the array.
Periodic Function Evaluation
The modulus operator is also handy for generating periodic sequences. For example, if you want to create a repeating pattern, you can leverage the modulus operation in a loop.
for (int i = 0; i < 10; ++i) {
std::cout << "Result: " << (i % 3) << std::endl; // Outputs 0, 1, 2, 0, 1, 2...
}
This code will print numbers in a repeating three-number cycle of 0, 1, 2.
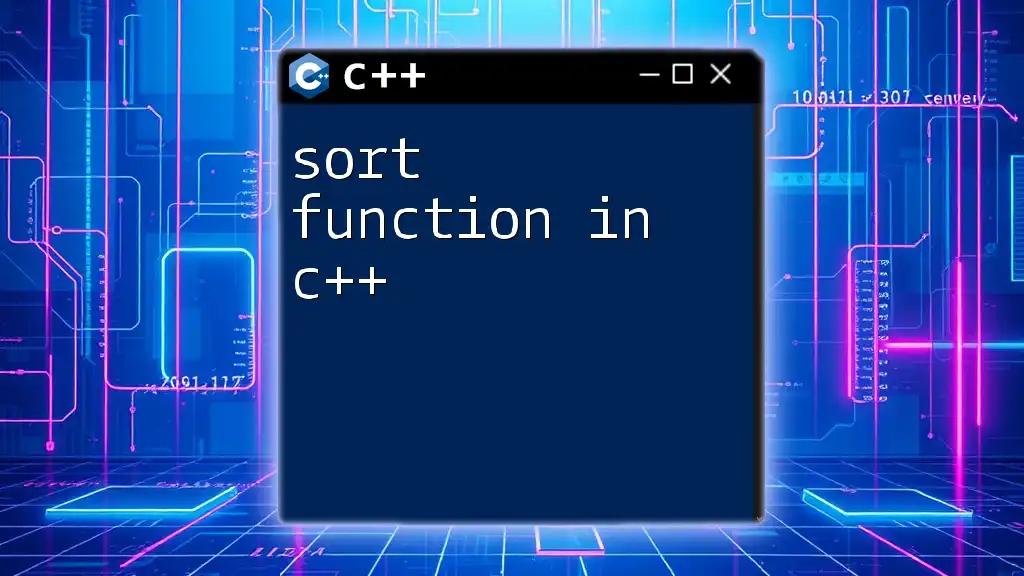
Common Pitfalls When Using Modulus
Negative Numbers and Modulus
One common mistake programmers encounter when using the modulus operator is the behavior of negative numbers. The result can be unintuitive since the sign of the result follows the numerator (the left operand):
std::cout << -10 % 3; // Outputs -1
std::cout << 10 % -3; // Outputs 1
std::cout << -10 % -3; // Outputs -1
Understanding this behavior is crucial when performing calculations with negative numbers, as it can lead to logic errors in programs.
Zero Division Error
Another pitfall involves dividing by zero, which is mathematically undefined. Applying the modulus operator with zero on the right will lead to runtime errors.
try {
int result = 10 % 0; // This will throw a runtime error
} catch (...) {
std::cout << "Cannot divide by zero!" << std::endl;
}
It's essential to check for zero before performing modulus operations to prevent crashes.
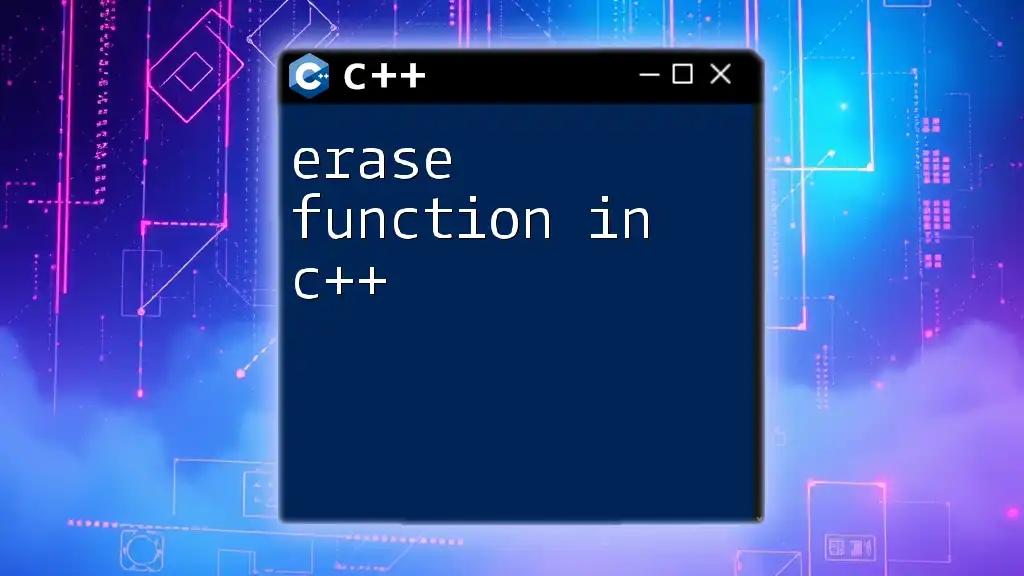
Performance Considerations
Efficiency of the Modulus Operator
In terms of efficiency, the mod function in C++ is generally fast. Most modern compilers optimize this operation well. However, in performance-sensitive applications, it's crucial to understand that using modulus can have varying complexities depending on context. For instance, the operation may be less efficient in tight loops if applied repeatedly without considering optimization strategies.

Conclusion
Recap of Key Points
The mod function in C++ is a powerful tool that provides a straightforward means of finding remainders in division operations. Its utility ranges from simple checks on number properties like parity to complex algorithm implementations involving cycles and periodic evaluations.
Encouragement for Experimentation
As you dive deeper into C++, don't hesitate to experiment with the modulus operator. Try out various implementations and scenarios; you'll find it a valuable addition to your programming toolbox.
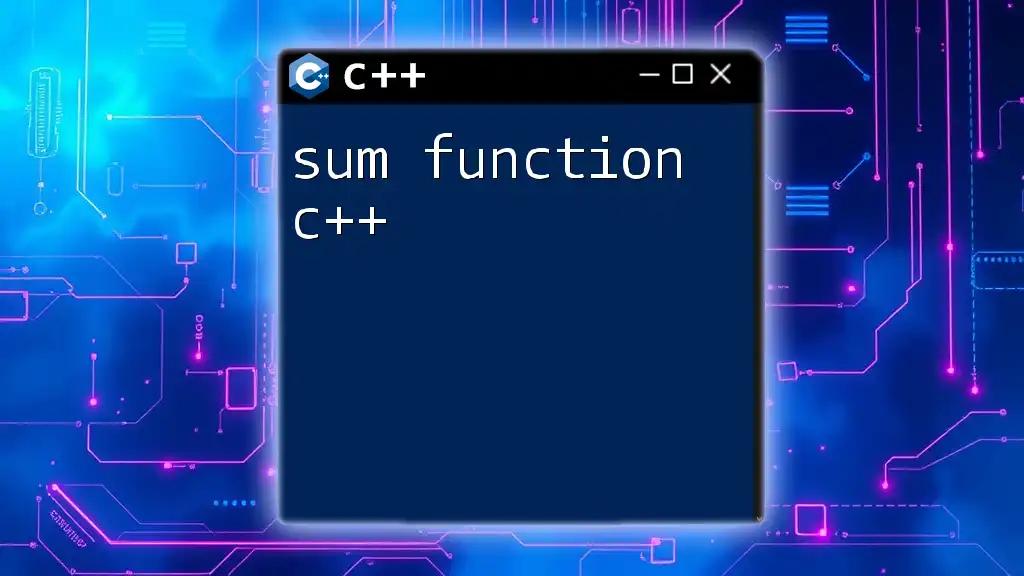
Additional Resources
Recommended Reading
For those looking to expand their knowledge further, consider reading books on C++ that cover data structures, algorithms, and number theory.
Community and Support
Joining online forums and communities can provide an excellent platform for asking questions, sharing knowledge, and discussing the intricacies of C++ programming, especially the use of the mod function. Engage with fellow programmers to enhance your learning experience!