The `erase` function in C++ is used to remove elements from a container, such as a vector or list, based on a specified position or range.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Erases the element at index 2 (which is 3)
for (int n : vec) {
std::cout << n << " "; // Output: 1 2 4 5
}
return 0;
}
Understanding the Basics of the Erase Function
The erase function in C++ is a powerful method used to remove elements from various standard library containers such as vectors, lists, and maps. Understanding how to utilize this function effectively is crucial for any C++ programmer, as it plays a significant role in memory management and manipulation of dynamic data structures.
What Does the Erase Function Do?
The erase function modifies the container by removing specified elements. This can be either by position, such as an iterator to an element, or by value, depending on the data structure used. This flexibility makes it an essential tool for maintaining and optimizing data collections during runtime. The ability to remove unwanted elements can enhance performance and ensure that your programs run smoothly.
Syntax of the Erase Function
The general form of the erase function is as follows:
container.erase(position);
or
container.erase(value);
Where `container` is the data structure you're working with, `position` refers to an iterator indicating which element to erase, and `value` is the element being removed. Depending on the type of container, the exact syntax and parameters may vary.
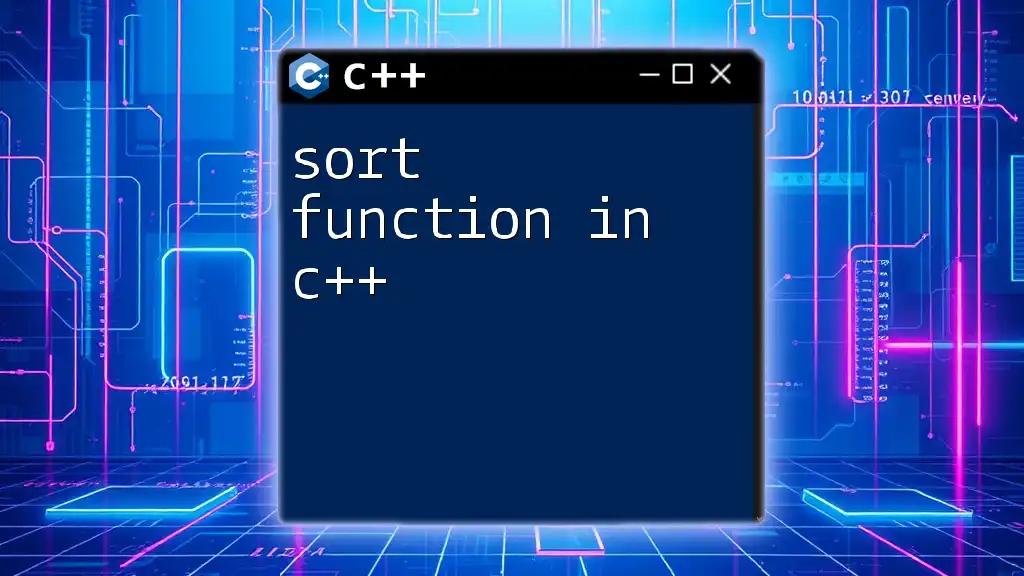
The Erase Function in Different Data Structures
Erase Function with Vectors
Vectors are dynamic arrays that can grow and shrink in size. The erase function is commonly used with vectors to remove elements.
Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.erase(numbers.begin() + 2); // Erasing the third element
// Output: 1, 2, 4, 5
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this example, we create a vector `numbers` containing five integers. The `erase` function is called to remove the third element (which is `3`). The output shows that the vector now contains `1, 2, 4, 5`, illustrating how the vector shrinks.
Erase Function with Lists
Lists in C++ are doubly-linked lists that allow for efficient insertions and deletions. The erase function works similarly but offers unique benefits due to the nature of linked lists.
Example:
#include <iostream>
#include <list>
int main() {
std::list<int> numbers = {1, 2, 3, 4, 5};
auto it = ++numbers.begin(); // Points to the second element
numbers.erase(it); // Erasing the second element
// Output: 1, 3, 4, 5
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this case, we erase the second element. By advancing the iterator, we remove the element containing `2`. The list’s integrity remains intact and continues to function correctly after the erasure.
Erase Function with Maps
Maps are collections of key-value pairs. The erase function in maps allows for the removal of pairs by key.
Example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> names = {{1, "Alice"}, {2, "Bob"}};
names.erase(2); // Erasing Bob
// Output: Remaining: 1 Alice
for (const auto& pair : names) {
std::cout << pair.first << " " << pair.second << "\n";
}
return 0;
}
In this snippet, we remove the entry with the key `2`. The output confirms that only Alice remains in the map, demonstrating how to manage key-value pairs effectively using the erase function.

Common Mistakes When Using the Erase Function
Incorrect Iterator Usage
One of the most frequent pitfalls when using the erase function is incorrect iterator management. Erasing an element invalidates the iterator pointing to it. Consequently, attempting to use the same iterator after an element has been erased leads to undefined behavior.
Example of a Common Mistake:
#include <iostream>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3, 4, 5};
auto it = v.begin();
v.erase(it++); // Incorrect approach as the iterator is invalid after erasure
// Further use of 'it' can cause issues
}
Attempting to Erase from an Empty Container
Another common mistake is attempting to use the erase function on an empty container. This action usually results in undefined behavior, as there are no elements to remove.
Example Demonstrating the Issue:
#include <iostream>
#include <vector>
int main() {
std::vector<int> emptyVec;
// The following line would cause undefined behavior
// emptyVec.erase(emptyVec.begin());
}
To avoid such issues, always ensure that the container has elements before invoking the erase function.
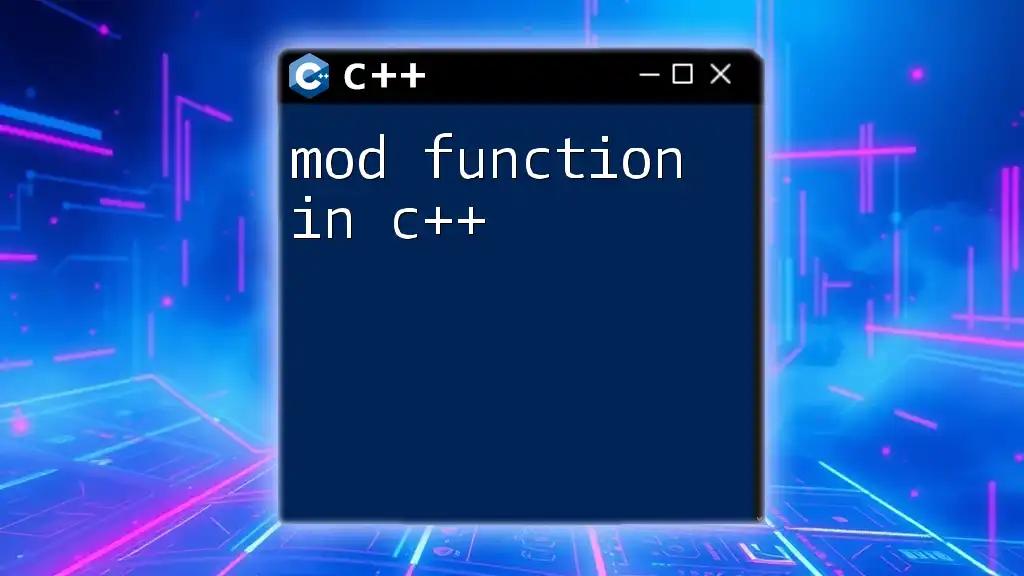
Best Practices for Using the Erase Function
Always Check Container Size Before Erasing
Before erasing an element, it's critical to check whether the container is empty. This small yet essential step prevents potential runtime errors.
Suggested Implementation:
if (!vec.empty()) {
vec.erase(vec.begin());
}
This code snippet ensures that we only attempt to erase an element when the vector is not empty, safeguarding against errors.
Efficient Erasure with Iterators
When erasing multiple occurrences of a particular value, utilizing iterators can enhance performance. The `remove` algorithm combined with `erase` helps to optimize the process.
Example of Using Iterators Correctly:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 2, 5};
int valueToRemove = 2;
auto it = std::remove(vec.begin(), vec.end(), valueToRemove);
vec.erase(it, vec.end()); // Efficiently removes all occurrences of 2
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
In this example, `remove` shifts all occurrences of the specified value to the end of the vector, and then `erase` ensures those occurrences are effectively removed. This method is cleaner and more efficient when dealing with larger datasets.
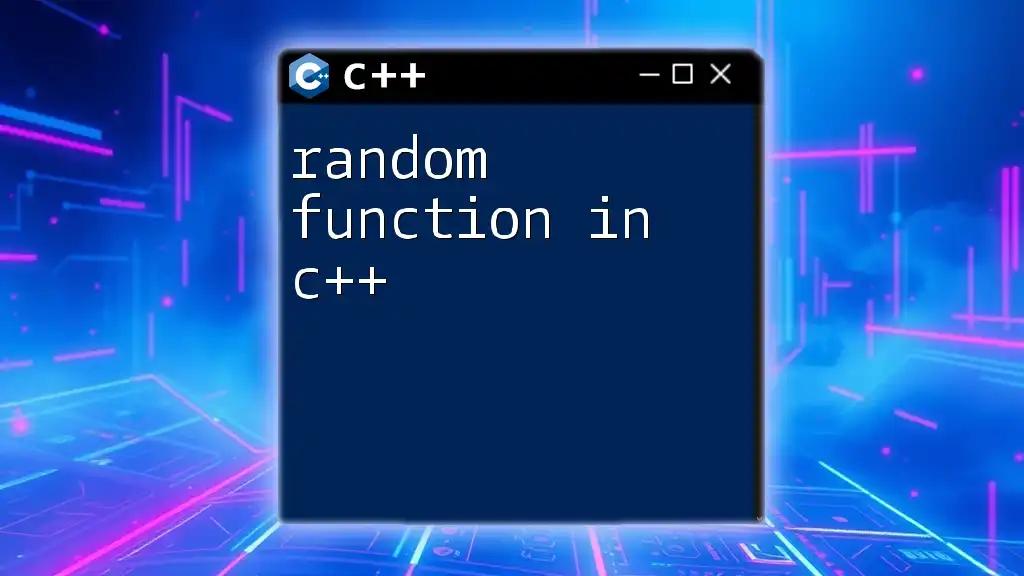
Conclusion
The erase function in C++ serves as an invaluable tool for managing data within containers. By mastering this function, programmers can optimize their applications, maintain data integrity, and enhance performance. With practical examples and adherence to best practices, you can navigate the complexities of data manipulation with confidence. Practicing these concepts and experimenting with different data structures will strengthen your understanding and expertise in C++.
As a final note, consider diving deeper into C++ resources or joining a community of C++ enthusiasts to continue honing your skills.