The `count` function in C++ is used to determine the number of occurrences of a specific element in a container, such as a vector or a list.
Here's a simple example using `std::count` from the `<algorithm>` header to count the occurrences of the number 5 in a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 5, 3, 5, 4, 5};
int count_of_five = std::count(vec.begin(), vec.end(), 5);
std::cout << "Number of occurrences of 5: " << count_of_five << std::endl;
return 0;
}
Understanding the Basics of Counting Elements
What is Counting in C++?
Counting in C++ refers to the process of tallying the occurrences of a specific element within a collection, such as an array or a vector. It is fundamental in various programming tasks, especially in data analysis, statistics, and algorithmic implementations where insight into frequency distribution is needed.
The Standard Library and Counting
C++ offers a robust Standard Library filled with functions that streamline the counting process. Utilizing standard functions, like the count function in C++, promotes more efficient and readable code. The count function is located in the `<algorithm>` header, eliminating the need for manual counting routines.
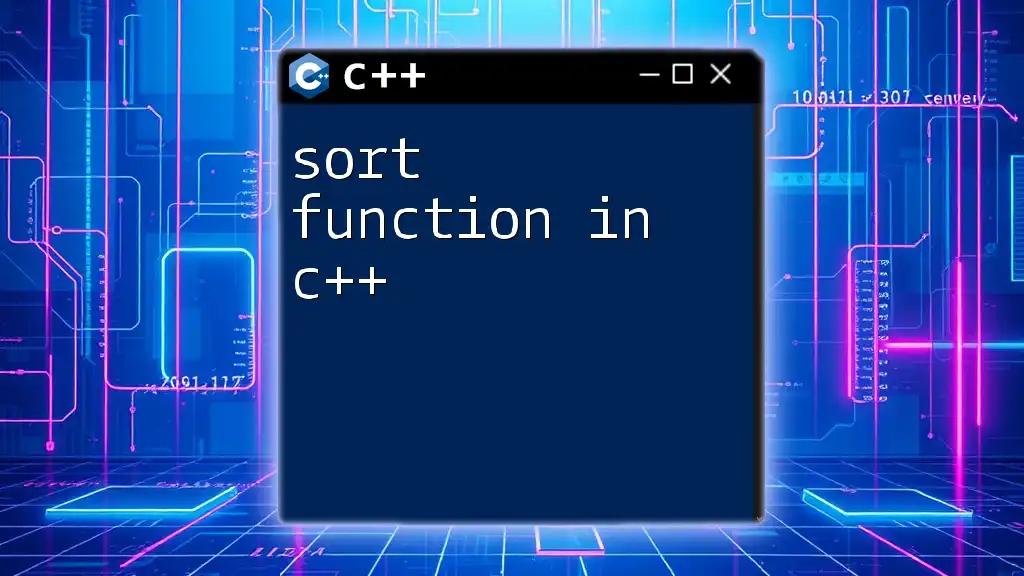
The Count Function in C++
Definition and Syntax of the Count Function
The count function provides a way to quickly determine how many times a specified value occurs within a range defined by iterators. The syntax for the count function is as follows:
template<class ForwardIt, class T>
constexpr typename std::iterator_traits<ForwardIt>::difference_type
count(ForwardIt first, ForwardIt last, const T& value);
This flexibility allows you to count occurrences across various types of iterators, making it a versatile choice for many scenarios.
Parameters Explained
- `first`: This is the start iterator indicating where the counting begins.
- `last`: The end iterator that specifies where the counting stops (exclusive).
- `value`: This is the value you wish to count across the specified range.
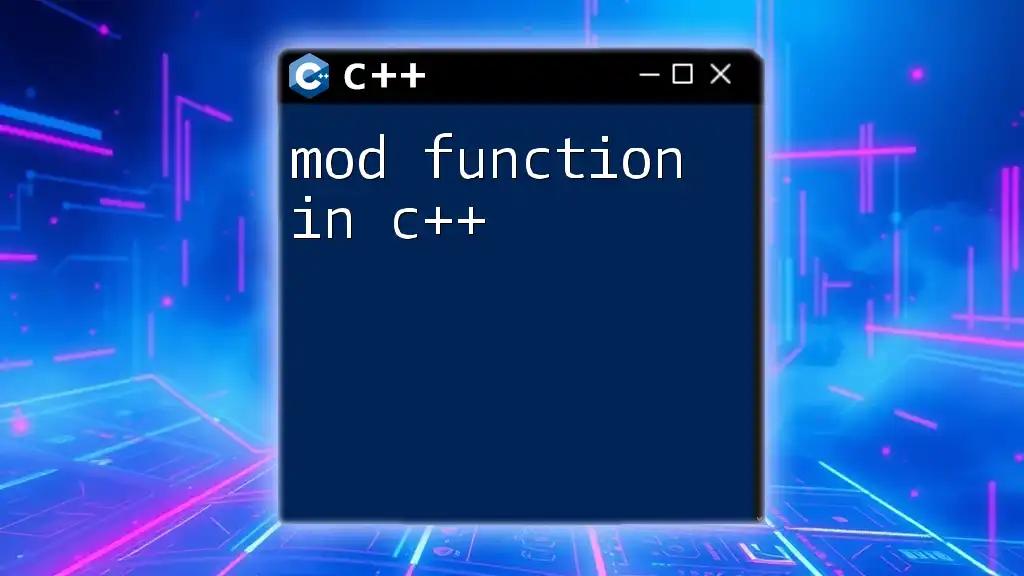
Practical Examples of Counting Elements
Basic Example of Count Function
To understand the use of the count function in C++, let's consider the following example that counts the occurrences of the number '1' in a vector of integers:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 1, 4, 1, 5};
int countOfOnes = std::count(numbers.begin(), numbers.end(), 1);
std::cout << "Count of 1s: " << countOfOnes << std::endl;
return 0;
}
In this code snippet, we include the necessary headers and initialize a vector named `numbers`. The count function is called with `numbers.begin()` and `numbers.end()` as iterators along with the value '1'. The output of this program will be Count of 1s: 3, reflecting that the number '1' appears three times in the vector.
Advanced Example: Counting Characters in a String
Count function can also be applied to strings. Here is an example that counts how many times the character 'l' appears in the specified string:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string text = "Hello World!";
int countOfL = std::count(text.begin(), text.end(), 'l');
std::cout << "Count of 'l': " << countOfL << std::endl;
return 0;
}
In this example, we utilize the string `text` to call the count function. The output will be Count of 'l': 3, which is the number of times 'l' appears in "Hello World!".
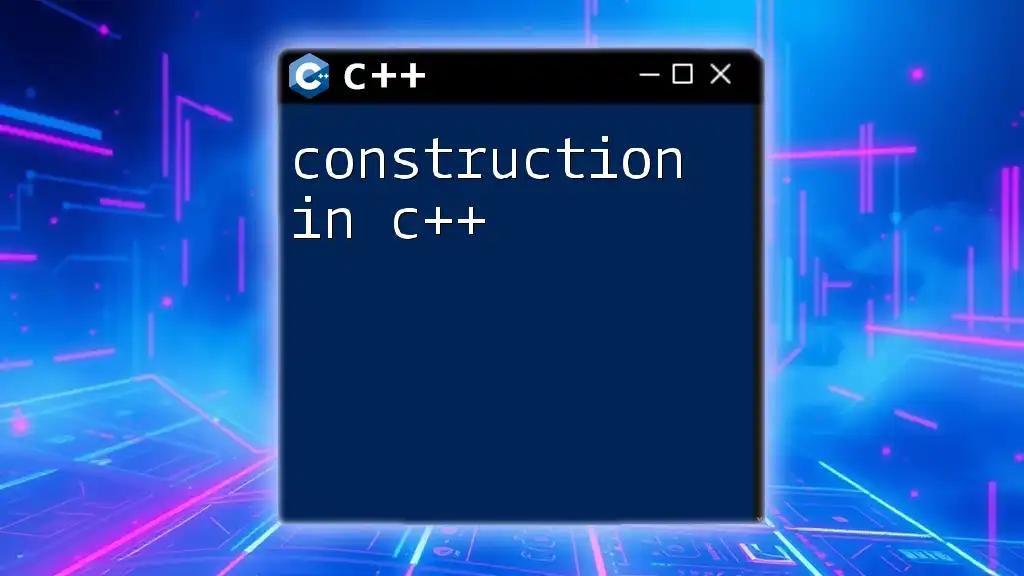
Comparing Count with Other Counting Methods
Manual Counting: Pros and Cons
While the count function effectively simplifies the counting process, it's also helpful to know how to manually count elements. Here is a simple method for doing so:
int manualCount(const std::vector<int>& vec, int value) {
int count = 0;
for (int num : vec) {
if (num == value) {
++count;
}
}
return count;
}
Pros and Cons of Manual Counting:
- Pros: More control over counting logic, can incorporate conditions, and works with complex data structures.
- Cons: More verbose, prone to errors, and less efficient than built-in functions.
Performance Comparison
When comparing `std::count` with manual counting, it's essential to acknowledge that `std::count` is optimized for performance by the C++ library. For large datasets, using the count function is generally preferable due to its efficiency. In scenarios where quick iterations or dynamic conditions are implemented, one might favor manual counting. Choose the method that best fits your specific use case.
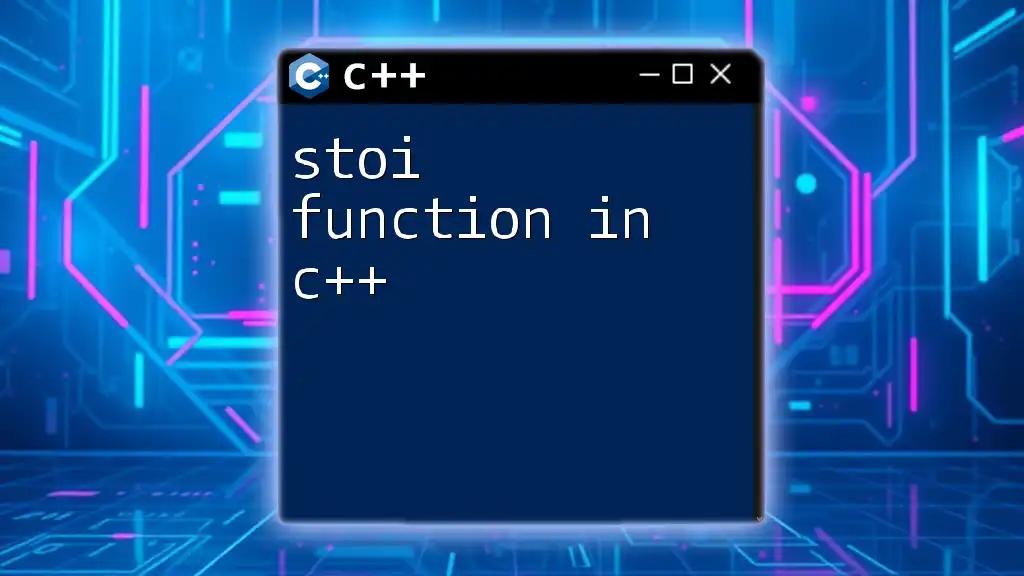
Common Mistakes and Troubleshooting
Misusing the Count Function
When utilizing the count function, several common pitfalls can arise, including:
- Incorrect iterator usage: Ensure that the iterators point to the correct range within the collection.
- Counting incompatible data types: Type mismatches can lead to errors, especially when counting objects or custom types.
To avoid these mistakes, always verify your data types and ensure that the iterators encompass the area you intend to analyze.
Debugging Count Issues
If you encounter issues while using the count function, here are a few strategies to consider:
- Review iterator boundaries: Make sure that you are not exceeding the bounds of your data container.
- Check return values: Always validate the results returned by the count function to catch any discrepancies promptly.
- Analyze error messages: Understanding common error messages can shed light on what the issue may be.
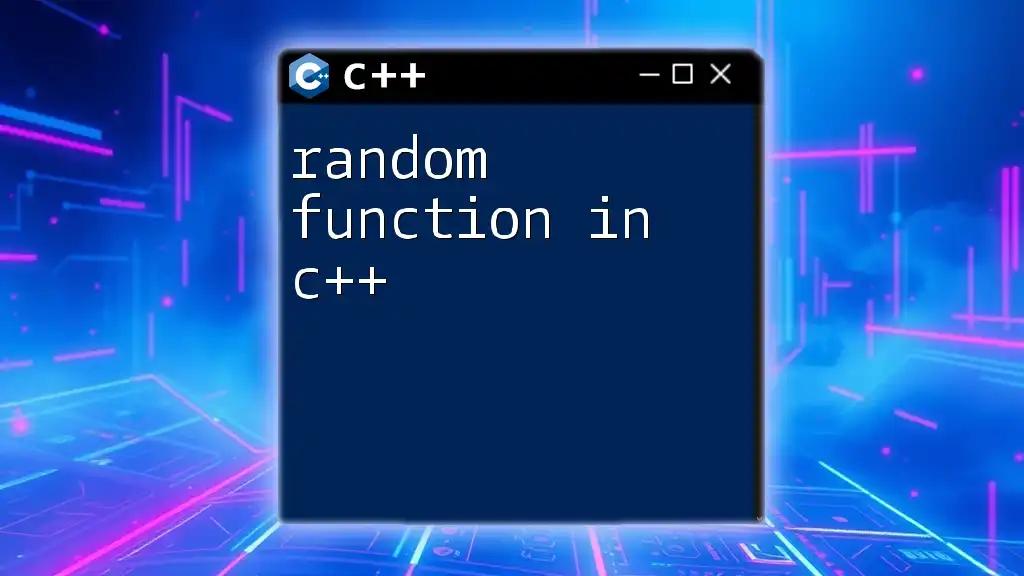
Conclusion
The count function in C++ plays an integral role in simplifying the process of counting occurrences within collections. It streamlines your code and enhances readability, making it a go-to choice for many developers. Practice with various examples to internalize the function's mechanics. Don't hesitate to experiment and create your implementations of the count function to strengthen your understanding!

Additional Resources
For further reading on counting elements and other features of the C++ Standard Library, consult additional tutorials and documentation dedicated to mastering algorithms in C++. Such resources can deepen your grasp of this powerful programming language.
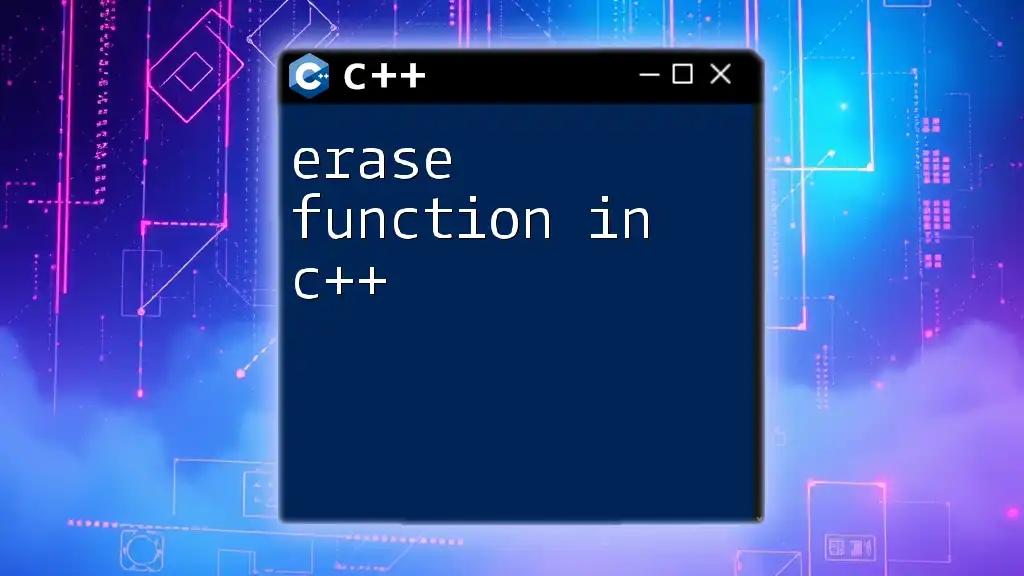
FAQs About the Count Function in C++
What is the difference between `std::count` and `std::count_if`?
`std::count_if` allows counting based on a predicate condition, making it more flexible than `std::count`, which only checks for a specific value. This capability is useful when you want to count items fulfilling a specific criterion rather than matching a single value.
Can `std::count` handle non-trivial data types?
Yes, the count function can count occurrences of non-trivial data types as long as the type supports equality comparison. Ensure that the type has the appropriate operators defined.
How can I count elements based on a condition?
You can utilize `std::count_if` combined with a lambda function or a predicate to count the elements fulfilling a particular condition. This provides greater functionality when dealing with complex conditions or types.
By seeking to master the count function in C++, you are taking essential steps toward writing more efficient and effective code!