The `cmp` function in C++ is typically used to compare two sequences or strings and determine their lexicographical order; however, since there is no built-in `cmp` function in C++, you can achieve similar functionality using the `std::lexicographical_compare` or by implementing your custom comparison function.
Here’s an example using `std::lexicographical_compare`:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str1 = "apple";
std::string str2 = "banana";
if (std::lexicographical_compare(str1.begin(), str1.end(), str2.begin(), str2.end())) {
std::cout << str1 << " is less than " << str2 << std::endl;
} else {
std::cout << str1 << " is not less than " << str2 << std::endl;
}
return 0;
}
What is the `cmp` Function?
The `cmp` function serves as a comparison function, vital for determining the order of elements in various data structures and algorithms. Rooted in the C++ standard libraries, it allows developers to evaluate relationships between values, primarily for sorting and searching purposes.
Purpose of the `cmp` Function
The primary utility of the `cmp` function lies in its ability to facilitate operations that require comparisons. Whether you're sorting arrays, searching through data structures, or implementing custom functionality within algorithms, leveraging a `cmp` function can significantly streamline operations and enhance readability in your code.
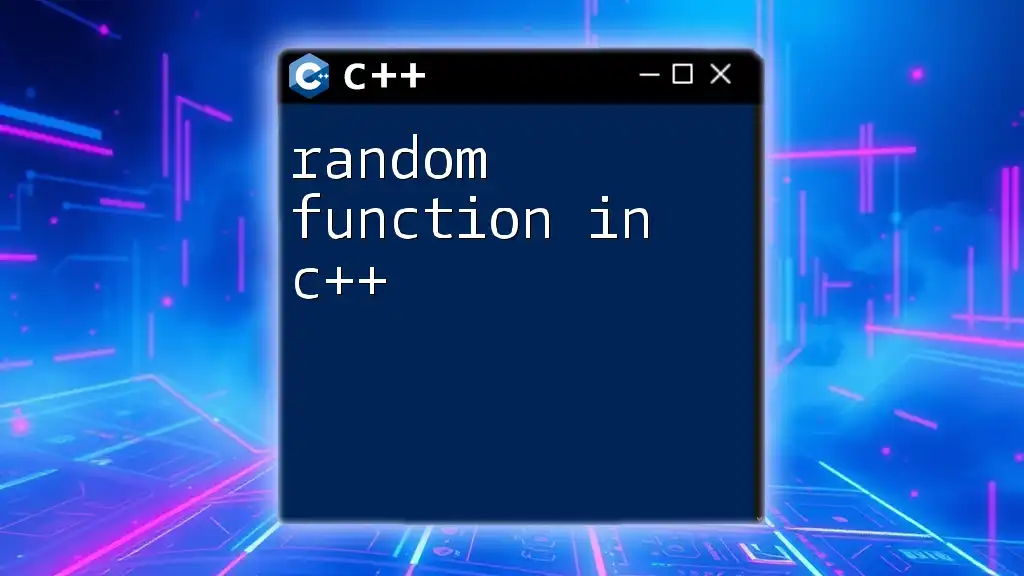
Syntax of the `cmp` Function
To understand the `cmp` function better, let’s explore its basic syntax:
int cmp(T a, T b);
In this syntax:
- `T` represents the data type being compared, which can be any fundamental data type or a user-defined type.
- `a` and `b` are the two elements being compared.
Parameter Details
The parameters passed to the `cmp` function generally include:
- `a`: The first element to compare.
- `b`: The second element to compare.
Return Values
The return values of the `cmp` function are crucial for understanding the comparison results:
- A value greater than zero indicates that `a` is greater than `b`.
- A value less than zero signifies that `a` is less than `b`.
- A return value of zero shows that `a` is equivalent to `b`.
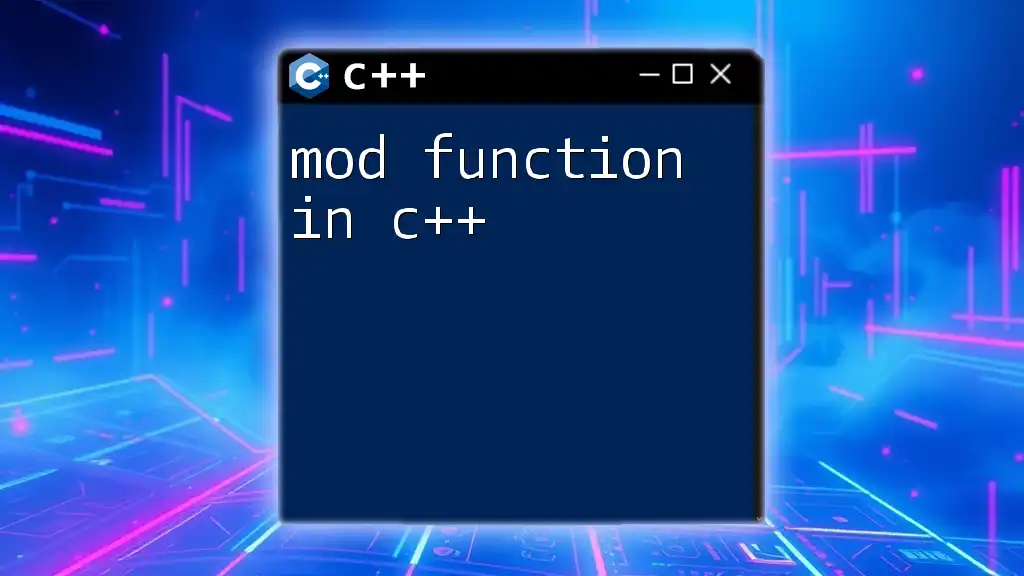
Usage of the `cmp` Function in C++
Custom Comparison
Creating a custom `cmp` function allows you to dictate how objects of your defined types are compared. For example:
struct Person {
std::string name;
int age;
};
bool cmp(const Person& p1, const Person& p2) {
return p1.age < p2.age; // Comparison based on age
}
In this snippet, we've defined a struct `Person` and a comparison function `cmp` that sorts `Person` objects by age.
Integration with STL
The `cmp` function is heavily utilized in conjunction with the C++ Standard Template Library (STL). For instance, when sorting a collection with `std::sort`, you can pass in the `cmp` function:
std::vector<Person> people = { ... }; // populate vector
std::sort(people.begin(), people.end(), cmp);
This code snippet sorts the vector of `Person` objects in ascending order based on age, utilizing the previously defined `cmp` function.
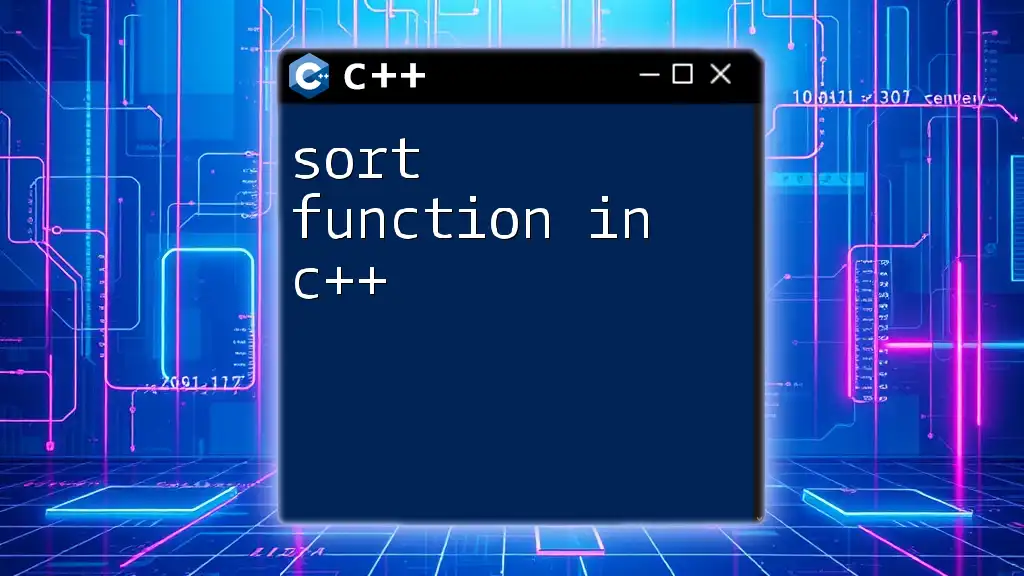
Common Applications of the `cmp` Function
Sorting Collections
One of the most prevalent uses of the `cmp` function is for sorting. For example, consider a simple comparison for sorting integers:
bool cmp(int a, int b) {
return a < b; // Ascending order
}
You can employ this function with sorting algorithms for lists or arrays, providing a clean and efficient mechanism to sort data.
Binary Search
The `cmp` function is not only pivotal for sorting but also plays a key role in implementing search algorithms. When performing a binary search, the `cmp` function helps in determining which half of the array to continue searching through.
Custom Data Structure Operations
Beyond sorting and searching, the `cmp` function is integral when working with custom data structures. For instance, when implementing a priority queue or a heap, the `cmp` function defines the order of elements based on their priority.
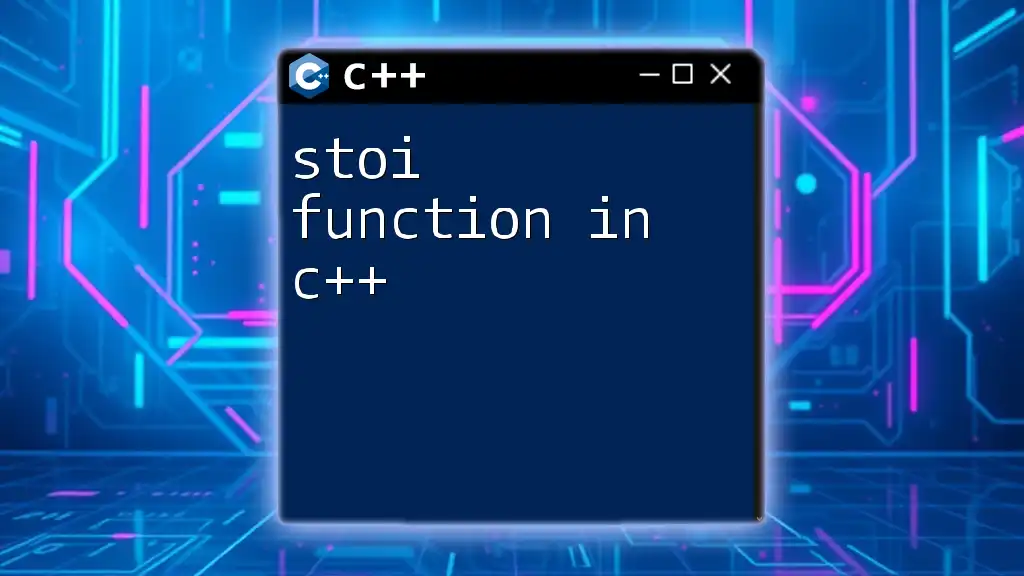
Comparison of `cmp` with Other Comparison Techniques
Lambda Functions vs. `cmp`
While traditional `cmp` functions are widely used, lambda functions offer a modern and succinct alternative. You can define comparison behavior inline, enhancing code clarity:
std::sort(people.begin(), people.end(), [](const Person& p1, const Person& p2) {
return p1.name < p2.name; // Comparison based on name
});
Both approaches have their merits. Lambda functions enhance readability for simple comparisons, while the `cmp` function is often preferred for complex, reusable logic.
Function Objects
An alternative to using `cmp` is to define function objects, which encapsulate the comparison logic within a class that overloads the `operator()`. This can also be beneficial for maintaining code structure.
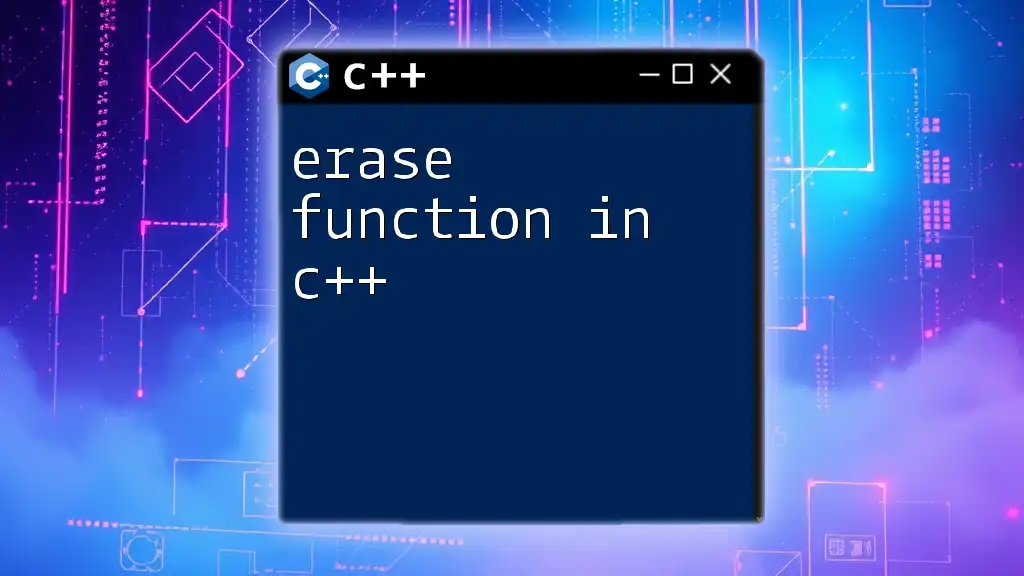
Best Practices for Implementing the `cmp` Function
Consistency in Comparison
To avoid ambiguous results, ensure that your `cmp` function is consistent. For instance, if two elements are considered equal, the function should return zero consistently for both combinations of the elements.
Efficiency Considerations
When designing your `cmp` function, strive for efficiency. If your comparison involves complex calculations or multiple comparisons, it might slow down your algorithms. Optimize the logic to maintain performance.
Readability and Maintainability
The clarity of your code is paramount. Implement the `cmp` function in a way that is straightforward to read and understand. Well-named parameters and concise logic enhance the maintainability of your code.
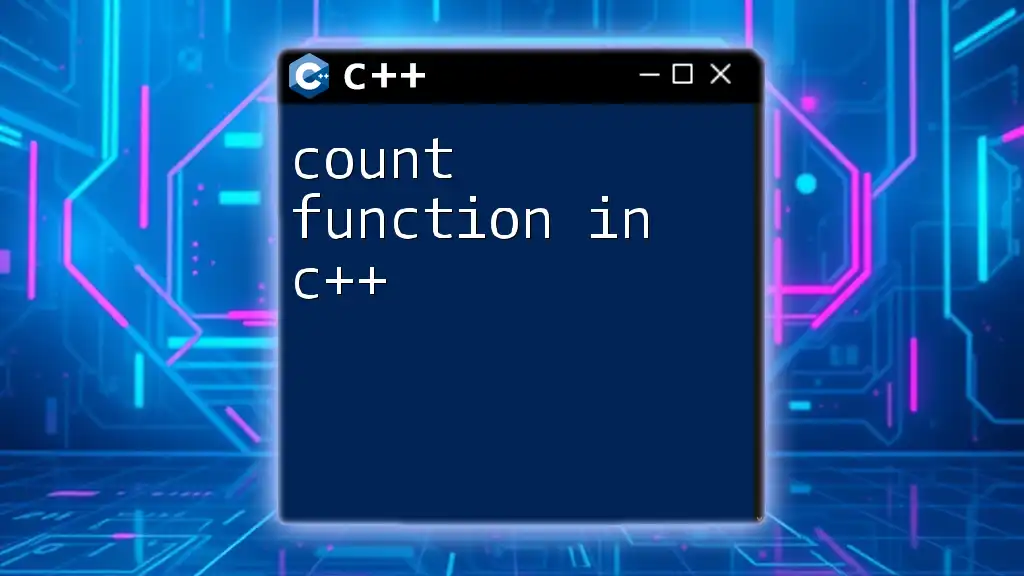
Common Pitfalls and Troubleshooting
Common Mistakes
Many developers overlook edge cases in their `cmp` functions, leading to unexpected behaviors. Always consider scenarios such as equal values, null pointers, or boundary conditions.
Debugging Tips
When experiencing issues with your `cmp` function, use print statements or logging extensively to understand the control flow. This can help reveal the cause of discrepancies in expected comparison results.
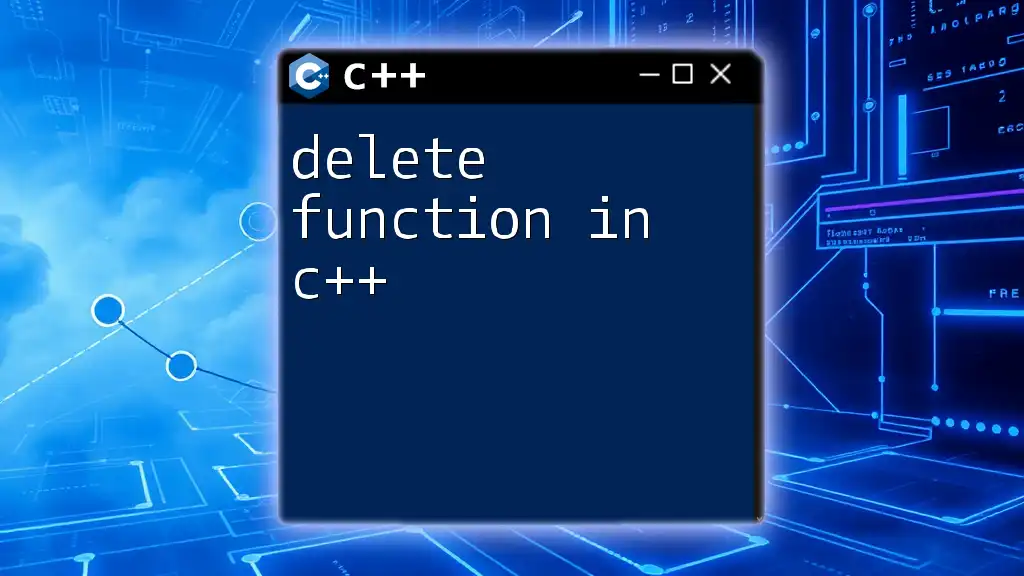
Conclusion
The `cmp function in C++` is an indispensable tool for developers, significantly enhancing the ability to perform sorting, searching, and structuring data comparisons. By understanding its syntax, implementing effective comparisons, and recognizing its applications, you will be well on your way to mastering its use in your C++ projects. Transitioning from traditional comparison methods to the innovative use of lambdas or function objects can further elevate your programming skills.
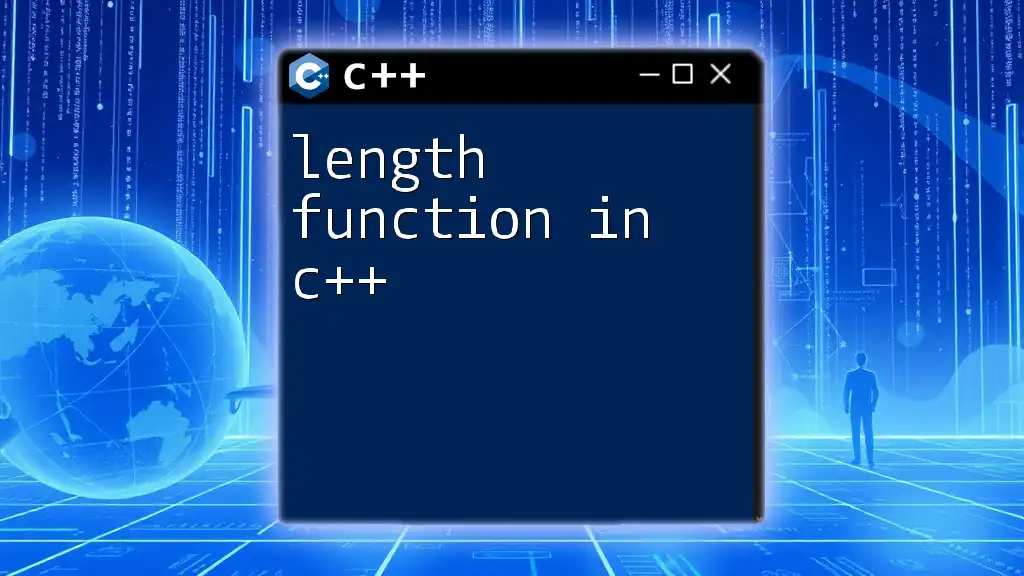
Additional Resources
Consider exploring books on C++ programming to deepen your understanding of concepts like comparison functions. Online courses can also provide a structured approach to learning these pivotal aspects of C++. Don’t forget to check C++ documentation for a detailed overview of functions and STL capabilities.