The `sleep` function in C++ is used to pause the execution of a program for a specified duration, measured in seconds, providing a simple way to manage time delays in your applications.
Here's an example of how to use the `sleep` function:
#include <iostream>
#include <thread> // Include the thread header for sleep
#include <chrono> // Include the chrono header for duration
int main() {
std::cout << "Program will pause for 3 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(3)); // Pause for 3 seconds
std::cout << "Resuming after pause." << std::endl;
return 0;
}
Understanding Sleep Function in C++
The sleep function is a crucial tool in C++ that allows programmers to pause the execution of a program for a specified period. This can be particularly useful in scenarios that require timing control, such as managing the flow of a game loop, coordinating tasks in multithreaded applications, or simply spacing out operations to avoid overwhelming a system.
When discussing the sleep function in C++, two standard libraries typically come into play: `<chrono>` and `<thread>`.
Sleep Function Variants
std::this_thread::sleep_for
This variant suspends the execution of the current thread for the specified duration. The syntax is flexible, allowing you to specify time in various units (milliseconds, microseconds, or even seconds). Here's a common example:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Sleeping for 2 seconds..." << std::endl;
// Suspend the thread for 2 seconds
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Awake!" << std::endl;
return 0;
}
In this example, the program prints a message, sleeps for 2 seconds, and then prints another message indicating it has resumed. This function is highly useful for adding delays in execution, making it easier to control the flow of the application.
std::this_thread::sleep_until
In contrast to `sleep_for`, this function allows you to pause execution until a specific time point is reached. This can be vital for synchronizing various tasks.
#include <iostream>
#include <thread>
#include <chrono>
int main() {
auto wakeUpTime = std::chrono::steady_clock::now() + std::chrono::seconds(5);
std::cout << "Sleeping until a specific time..." << std::endl;
std::this_thread::sleep_until(wakeUpTime);
std::cout << "Awake again!" << std::endl;
return 0;
}
In this scenario, the program sleeps until a specified time (5 seconds later) instead of just sleeping for a given duration. This precision can be especially useful in real-time applications.
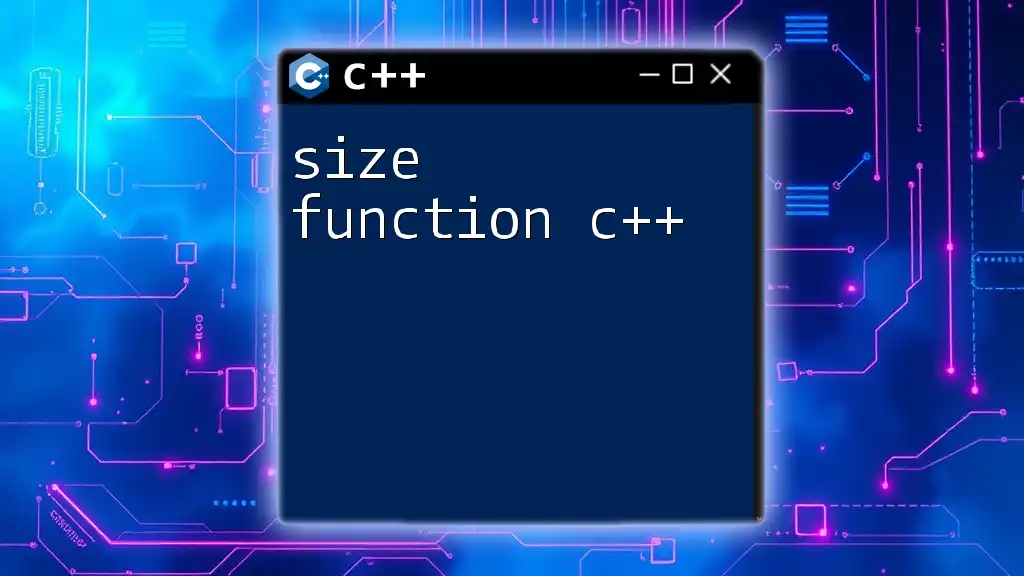
How to Use the Sleep Function
To begin using the sleep function in C++, you first need to set up your programming environment correctly. Make sure to include the necessary headers:
#include <iostream>
#include <thread>
#include <chrono>
Example Usage of Sleep Function
Here’s a simple example program that utilizes the sleep function c++ effectively.
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Starting countdown..." << std::endl;
for (int i = 3; i > 0; --i) {
std::cout << i << " seconds remaining." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
std::cout << "Go!" << std::endl;
return 0;
}
In this countdown example, each iteration of the loop prints the remaining seconds and then sleeps for 1 second, creating a simple yet effective countdown timer.
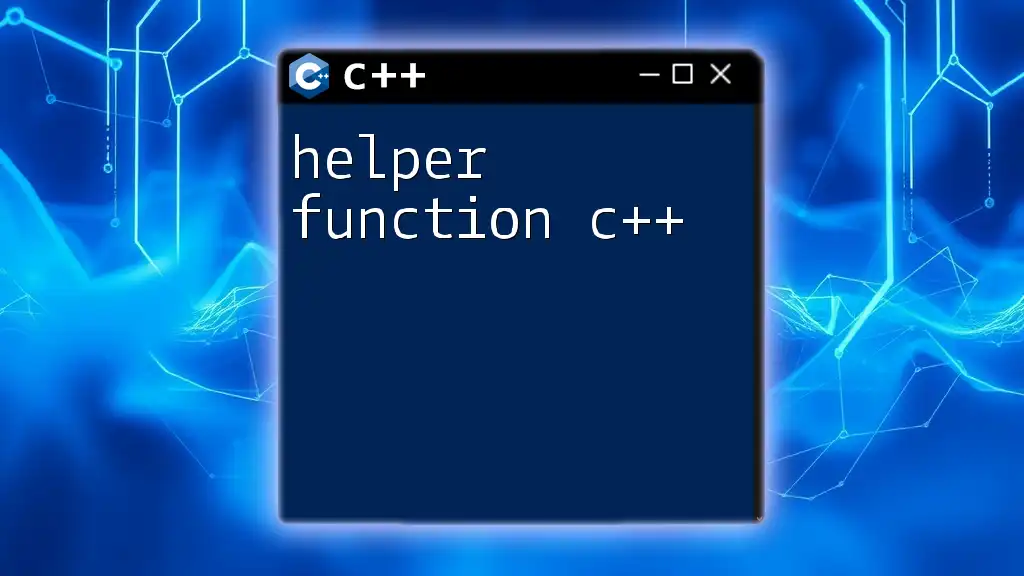
Practical Applications of the Sleep Function
The sleep function c++ is utilized in various scenarios, including:
Game Development
In game programming, controlling the frame rate is critical. The sleep function can be used to throttle the loop so that the game runs smoothly, without consuming unnecessary CPU resources. For example, adding a sleep during each loop iteration can help regulate the speed at which frames are rendered.
Multithreading
When dealing with multiple threads, using the sleep function allows a thread to yield execution, preventing resource contention. It’s essential in scenarios like waiting for shared resources or coordinating between producer and consumer threads.
Polling Mechanisms
In applications where certain conditions must be checked periodically without overwhelming the system, the sleep function can effectively space out these checks. This is particularly useful in network applications or callbacks involving sensor data.
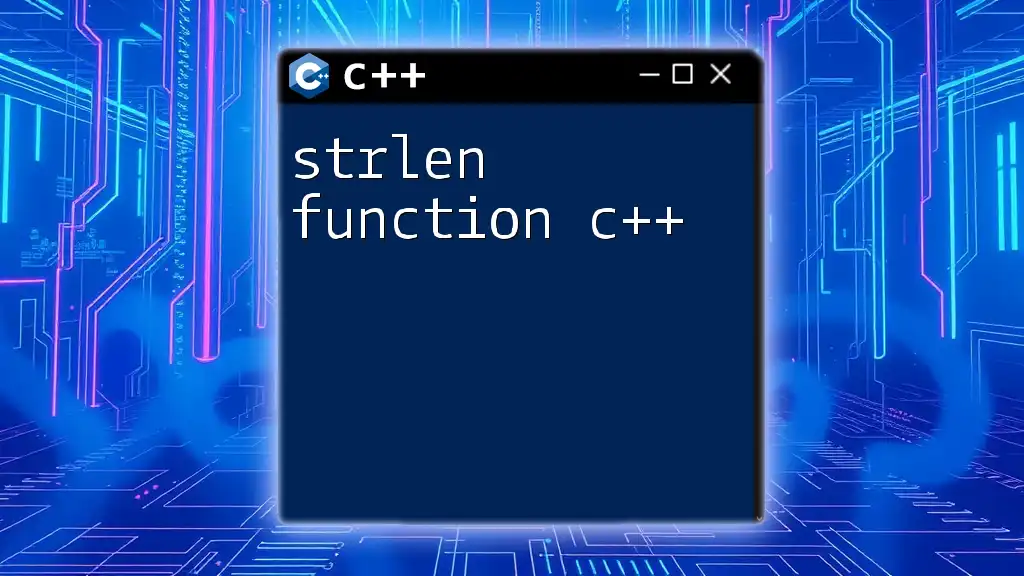
Potential Pitfalls and Best Practices
Using the sleep function is straightforward, but there are some best practices to keep in mind:
-
Impact on Performance: While the sleep function can help manage execution timing, overuse or inappropriate durations can degrade performance. It's essential to balance the need for pauses with system responsiveness.
-
Blocking Threads: Avoid blocking the main thread for long periods unless necessary; this can lead to a poor user experience in UI applications.
-
Alternatives: For more complex scenarios, consider using more sophisticated timing mechanisms, such as timers or condition variables.
Debugging Common Issues
When using the sleep function, you may encounter issues such as threads not waking up as expected. This can be caused by incorrect duration specifications or misunderstandings in thread synchronization. Always validate your time settings and ensure proper synchronization practices when dealing with multiple threads.
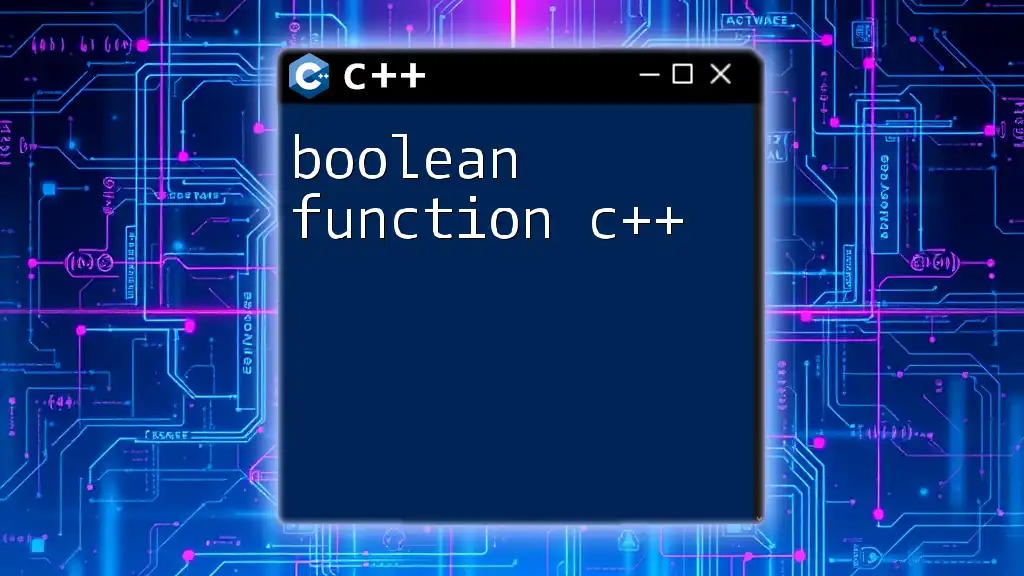
Conclusion
The sleep function in C++ is not only simple to use but also incredibly powerful when applied correctly. By adding timing control to your applications, you can manage execution flow effectively, enhancing performance and usability. As you experiment with sleep in your coding endeavors, you'll learn to appreciate its value and versatility in various programming contexts.
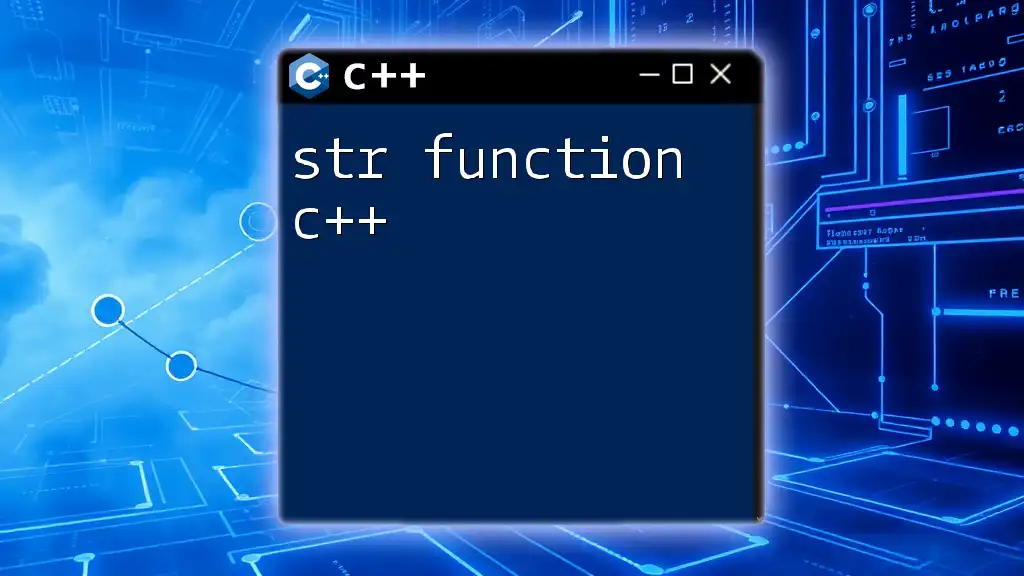
Additional Resources
For those looking to dive deeper, the official documentation for `<thread>` and `<chrono>` provides extensive information on the capabilities of these libraries. Consider exploring additional resources and tutorials on concurrency in C++ to further enhance your understanding.
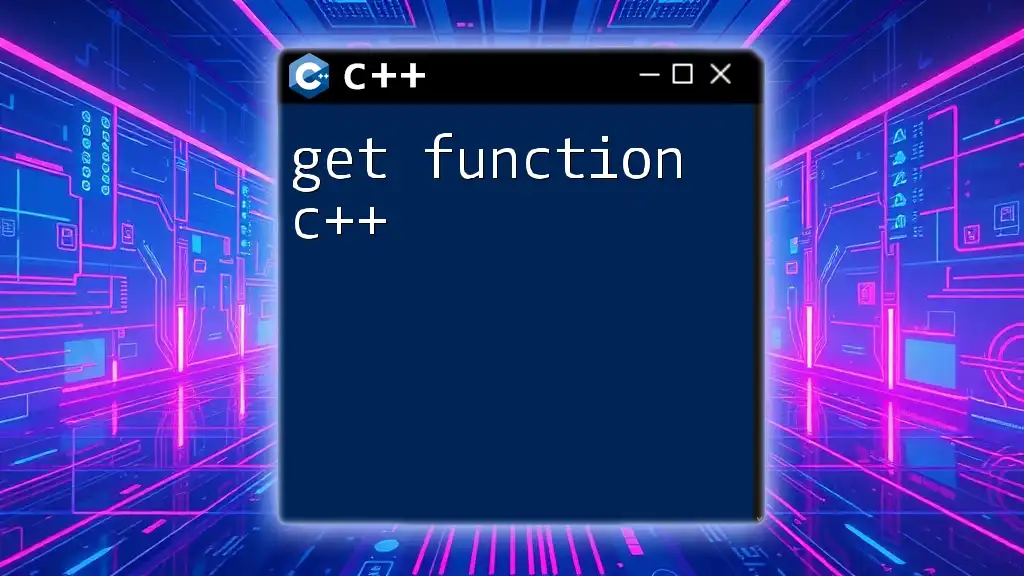
FAQs
How does sleep affect performance in C++ applications?
The sleep function can significantly impact performance if misused. While it can prevent resource congestion, excessive or unnecessarily long sleep calls can make an application unresponsive or slow.
Are there alternatives to using the sleep function in C++?
Yes, alternatives like timers, condition variables, or event-driven programming can be more efficient in specific scenarios, especially for maintaining responsiveness in applications.
Can I use sleep in a real-time application?
Using sleep in real-time applications requires careful consideration, as it can introduce latency. Alternative methods of synchronization or timing should be explored for such contexts.