In C++, a function is called by using its name followed by parentheses, and you can pass arguments inside the parentheses if needed.
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet(); // Calling the function
return 0;
}
What is a Function in C++?
Definition of a Function
A function in C++ is a block of code designed to perform a particular task. It's a reusable component that helps in organizing code, making it easier to read and maintain. Functions can take input, process it, and return an output. They form the building blocks of C++ programs, fostering modularity and reusability.
Types of Functions
Functions in C++ can be categorized into two main types:
-
Built-in Functions: These are provided by the language itself, such as `cout` for output and `cin` for input. They are readily available for use without needing to define them.
-
User-defined Functions: These are functions created by the programmer. They allow for customized functionality tailored to specific needs and encourage a clearer structure in code.
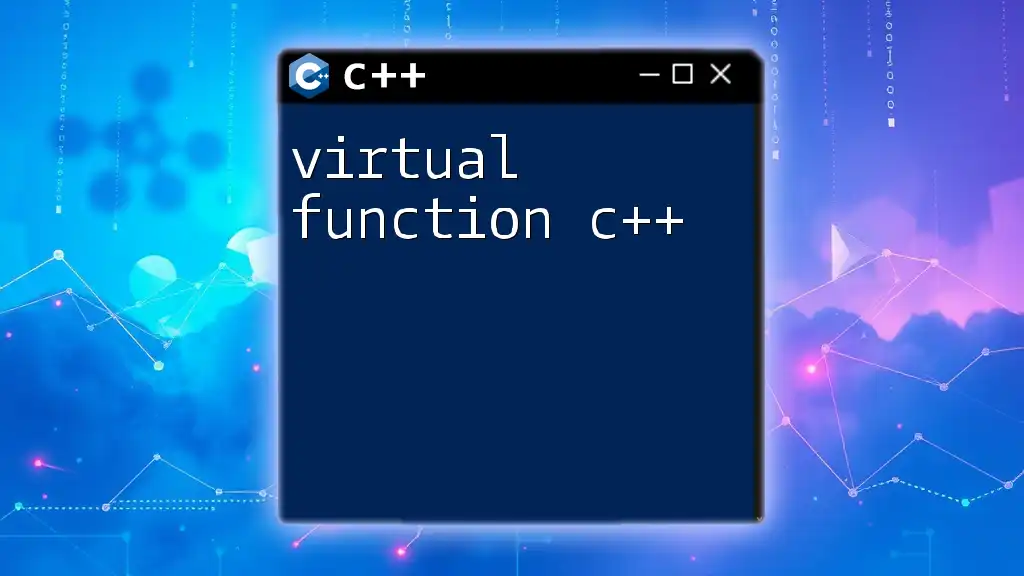
Understanding Function Syntax in C++
Function Declaration
A function declaration, also known as a function prototype, introduces the function to the compiler. It informs the compiler about the function's name, return type, and the types of parameters it accepts.
Syntax Example
return_type function_name(parameter_list);
This declaration is essential for the compiler to recognize the function before its actual definition.
Function Definition
The actual implementation of a function is known as a function definition. It comprises the function’s name, the parameter list, and the body of the function, where the processing occurs.
Syntax Example
return_type function_name(parameter_list) {
// function body
}
This is where the function's specific operations take place, making it crucial for functionality.
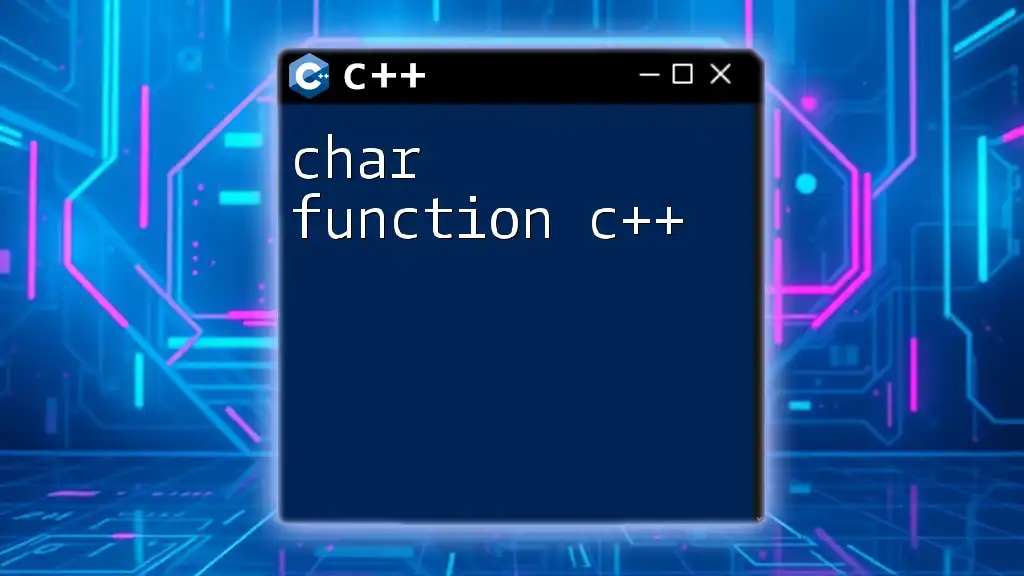
How to Call a Function in C++
Calling a Function
To call a function in C++, you use the function's name followed by parentheses, which can optionally include arguments that match the parameters defined in the function.
Syntax for Calling a Function
The basic syntax for calling a function is:
function_name(arguments);
This tells the program to execute the function with the provided arguments.
Example of Calling a Function
Consider the following C++ code:
#include <iostream>
using namespace std;
void greet() {
cout << "Hello, World!" << endl;
}
int main() {
greet(); // Calling the greet function
return 0;
}
In this example, when `greet()` is called in the `main()` function, it executes the code within the `greet()` function, displaying "Hello, World!" to the output.
Explanation of the Example
In the above code, the function `greet()` does not take any parameters nor return any value. By simply calling `greet();`, we invoke its behavior, demonstrating one of the simplest ways to call a function in C++.
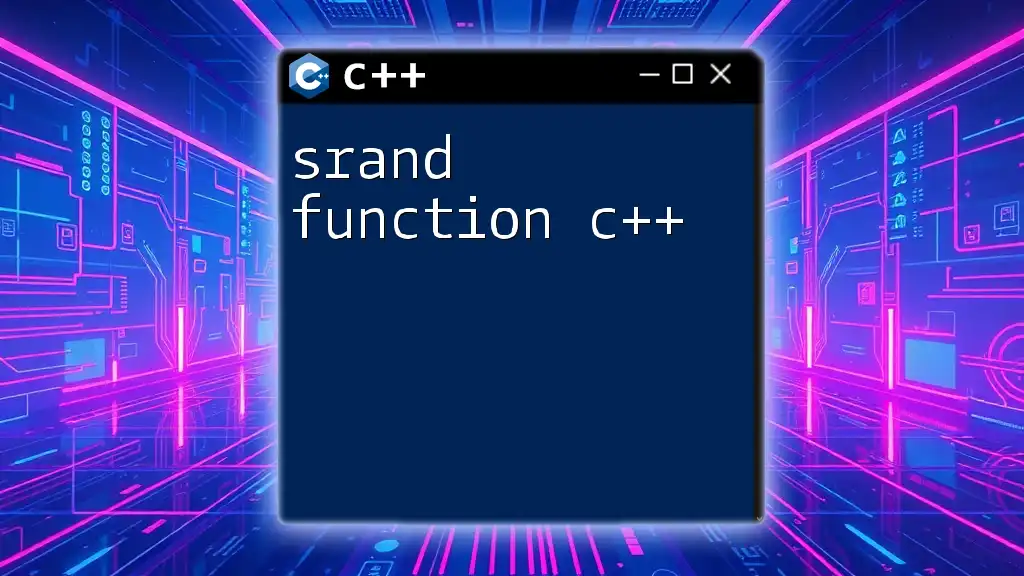
Types of Function Calls in C++
Call by Value
Call by Value is a method where the value of an argument is passed to the function. In this case, a copy of the value is made, so changes made to the parameter inside the function do not affect the argument.
Example
void increment(int num) {
num++;
cout << "Inside increment: " << num << endl;
}
int main() {
int value = 5;
increment(value);
cout << "Outside increment: " << value << endl; // value remains 5
return 0;
}
Here, despite modifying `num` within the `increment` function, the value of `value` outside remains unchanged.
Call by Reference
Call by Reference, on the other hand, transmits a reference (or address) of the variable to the function. Consequently, changes to the parameter affect the original argument, as both share the same memory location.
Example
void increment(int &num) {
num++;
cout << "Inside increment: " << num << endl;
}
int main() {
int value = 5;
increment(value);
cout << "Outside increment: " << value << endl; // value is now 6
return 0;
}
In this case, the output demonstrates that the `value` variable has changed after the function call, illustrating the power of call by reference.
Differences between Call by Value and Call by Reference
Understanding the differences between these two methods is vital. Call by Value creates copies of the arguments, protecting the original data, whereas Call by Reference allows for direct manipulation, which can be both powerful and risky depending on the context.
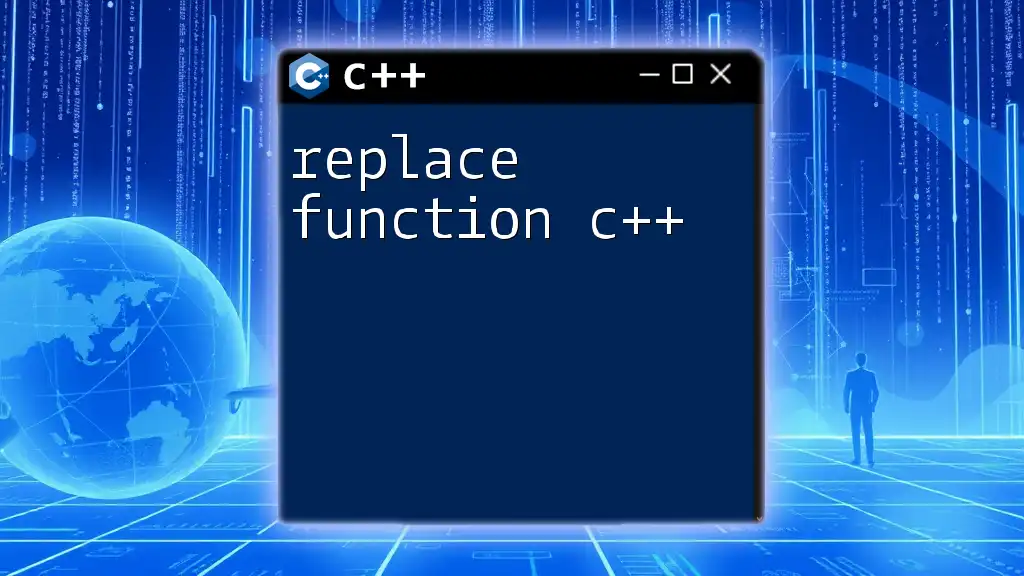
Passing Arguments to Functions
Default Arguments
C++ allows the specification of default arguments for function parameters, making arguments optional. If a caller does not provide an argument, the default value is used.
Example
void display(int a, int b = 10) {
cout << "a: " << a << ", b: " << b << endl;
}
int main() {
display(5); // Outputs: a: 5, b: 10
display(5, 15); // Outputs: a: 5, b: 15
return 0;
}
This feature enhances flexibility and simplifies function calling.
Overloaded Functions
Overloaded functions allow the same function name to be used for different types or numbers of parameters. This promotes code reuse and enhances clarity.
Example
void show(int a) {
cout << "Integer: " << a << endl;
}
void show(double a) {
cout << "Double: " << a << endl;
}
int main() {
show(5); // Calls the integer version
show(5.5); // Calls the double version
return 0;
}
Overloading helps keep the naming consistent while providing different functionalities based on parameter types.
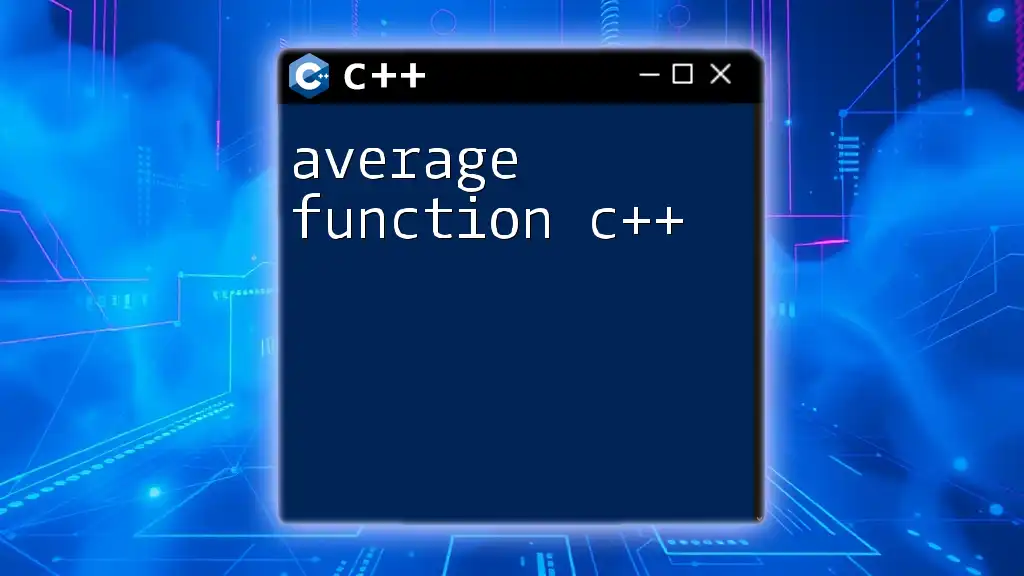
Best Practices for Calling Functions in C++
Keep Functions Short and Concise
It's essential to keep functions short and ready for quick comprehension. A function should ideally perform a single task, making debugging easier and enhancing readability.
Name Functions Appropriately
Functions should have descriptive names that convey their purpose. Naming conventions play a crucial role in code maintainability. For example, a function named `calculateArea()` clearly indicates its function.
Use Function Prototypes
In larger programs, declaring function prototypes improves organization. It helps define functions before their use and allows for better separation of function implementation and function calling.
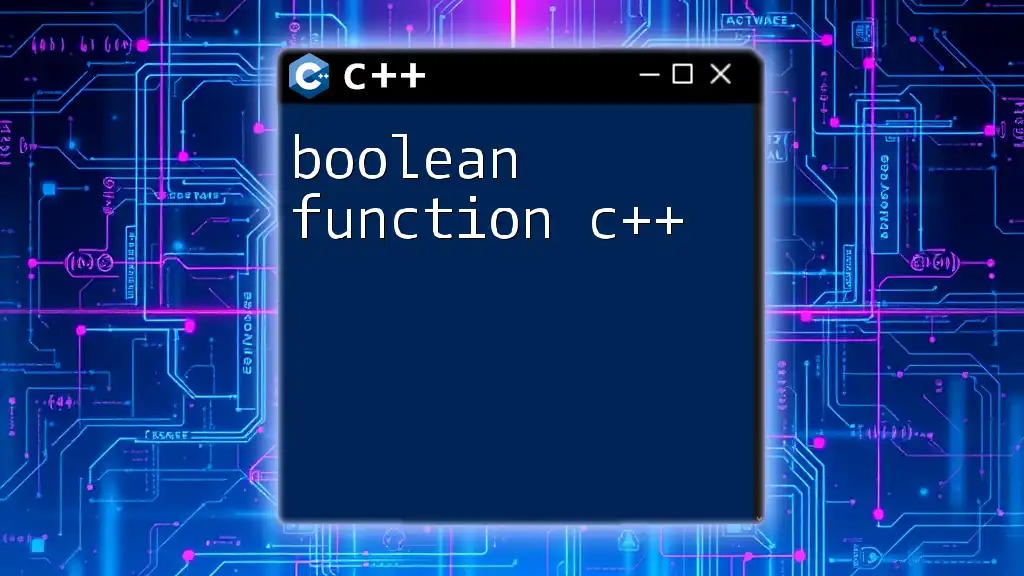
Troubleshooting Common Issues
Common Errors When Calling Functions
While working with functions, programmers often encounter mistakes like syntax errors, mismatched parameter types, or forgetting to include necessary headers. Awareness of these common pitfalls can significantly streamline the coding process.
Error Handling in Functions
Incorporating error handling in your functions provides greater robustness. Using `try-catch` blocks and validating inputs before processing can prevent runtime exceptions and ensure that your functions run smoothly.
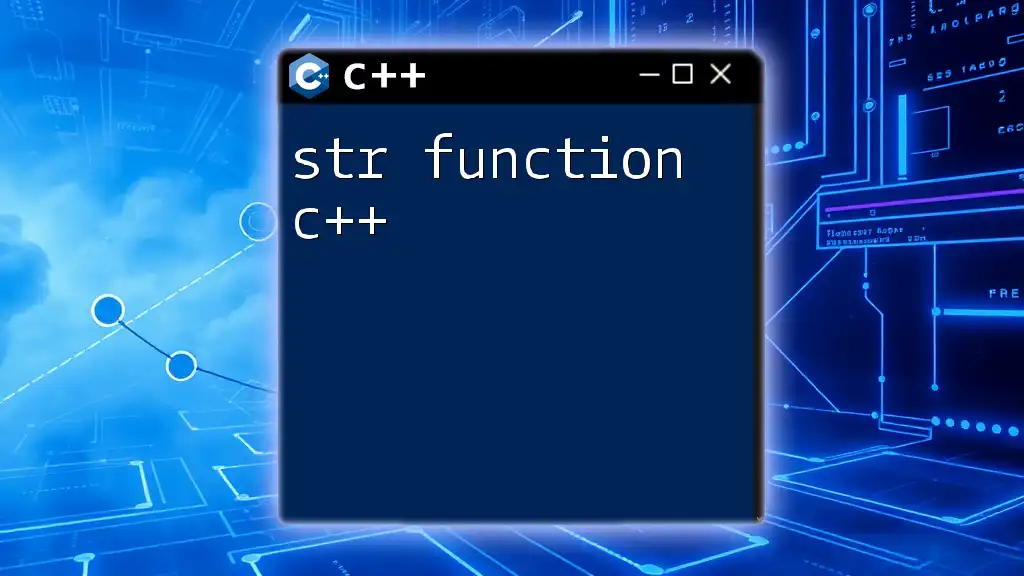
Conclusion
Understanding how to effectively call a function in C++ is fundamental for any programmer. The concept enhances code modularity, allowing for reusability and clarity. As you practice constructing and calling your own functions, applying the principles of call by value and call by reference will further enhance your programming skills. Embrace the power of functions and explore the possibilities they offer in C++.
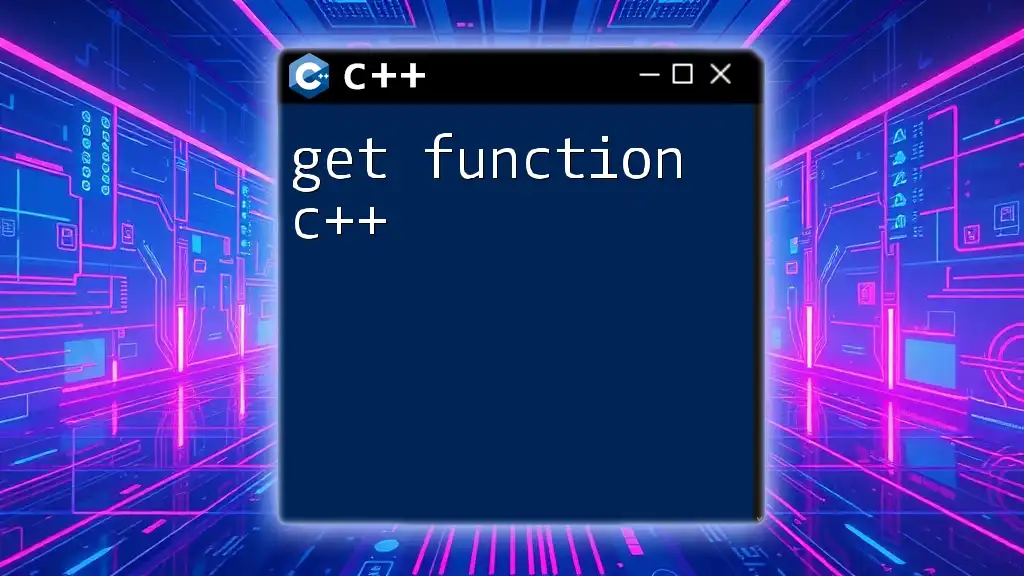
Call-to-Action
We encourage you to share your experiences with function calling in C++. If you have any questions, do not hesitate to ask! Explore our additional resources or courses to further elevate your C++ skills.