A getter function in C++ is a member function that provides access to the private data members of a class, allowing retrieval of their values without direct access.
Here’s a simple code snippet demonstrating a getter function:
#include <iostream>
using namespace std;
class MyClass {
private:
int value;
public:
MyClass(int v) : value(v) {}
// Getter function
int getValue() const {
return value;
}
};
int main() {
MyClass obj(42);
cout << "Value: " << obj.getValue() << endl; // Output: Value: 42
return 0;
}
What are Getter Functions?
Getter functions, often referred to simply as "getters," are crucial components in the design of classes in C++. They are used to access the values of private class members. By using getter functions, developers adhere to the principle of encapsulation, which safeguards object data and ensures that it cannot be altered directly from outside the class.
The Role of Getters in Object-Oriented Programming
In object-oriented programming (OOP), encapsulation is fundamental. By restricting direct access to some of an object’s components, we maintain control over how the data is accessed and modified. This promotes higher integrity and reduces the chance of inadvertent data corruption. Getter functions serve as the controlled points of access, allowing code to retrieve values safely while keeping the rest of the class’s implementation hidden.
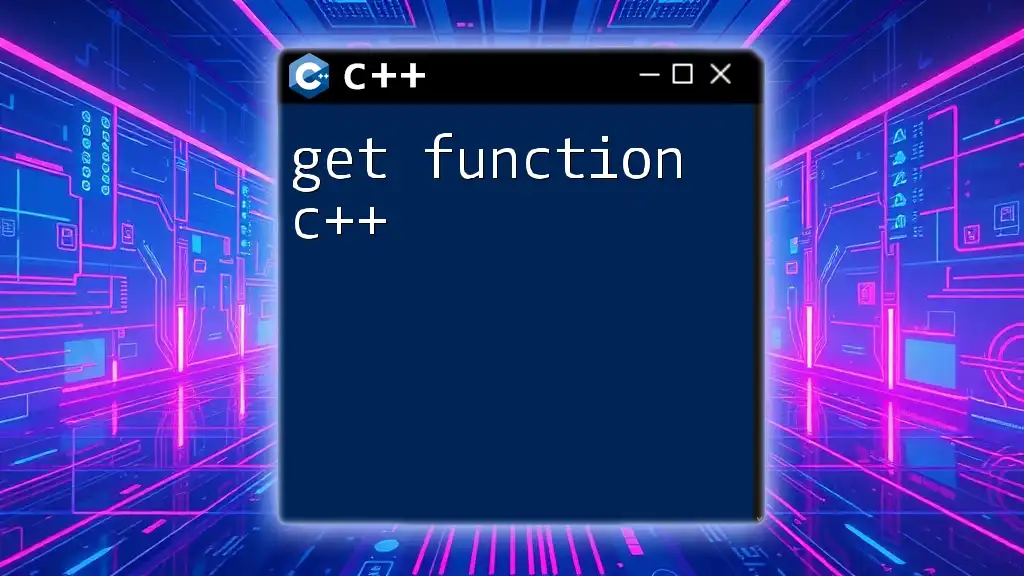
Understanding Getter Functions in C++
What is a Getter Function?
A getter function is a public member function of a class that allows external code to obtain the value of a private member variable. By providing a public interface, these functions ensure that any external access to internal class data adheres to the rules defined within the class.
Basic Syntax of Getter Functions
The syntax for implementing a getter function follows a straightforward format. It typically includes the return type, the name (often prefixed with "get" followed by the member variable name), and an optional `const` qualifier to indicate that the function does not modify the object’s state. Here’s a basic example:
class Example {
private:
int value;
public:
int getValue() const {
return value;
}
};
In this example, the getter function `getValue` retrieves the private member `value`. The use of `const` signifies that the method does not alter the state of the object.
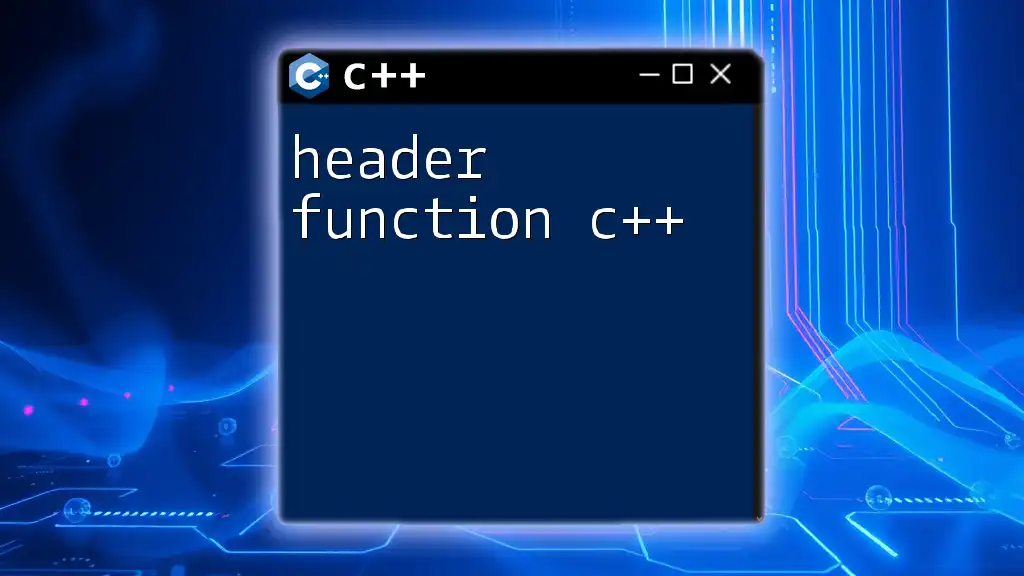
Why Use Getter Functions?
Advantages of Getters
Getter functions provide several benefits:
- Encapsulation and Data Protection: They restrict direct access to member variables, which enhances data integrity.
- Flexibility: Changes to internal data structures can be made without altering external code that relies on these getters.
- Read-Only Access: Getters can provide a way to expose data while preventing changes to it.
Common Use Cases
Getters are especially valuable in scenarios such as:
- Retrieving user information (e.g., usernames or email addresses).
- Accessing configuration settings (e.g., application settings stored in a class).
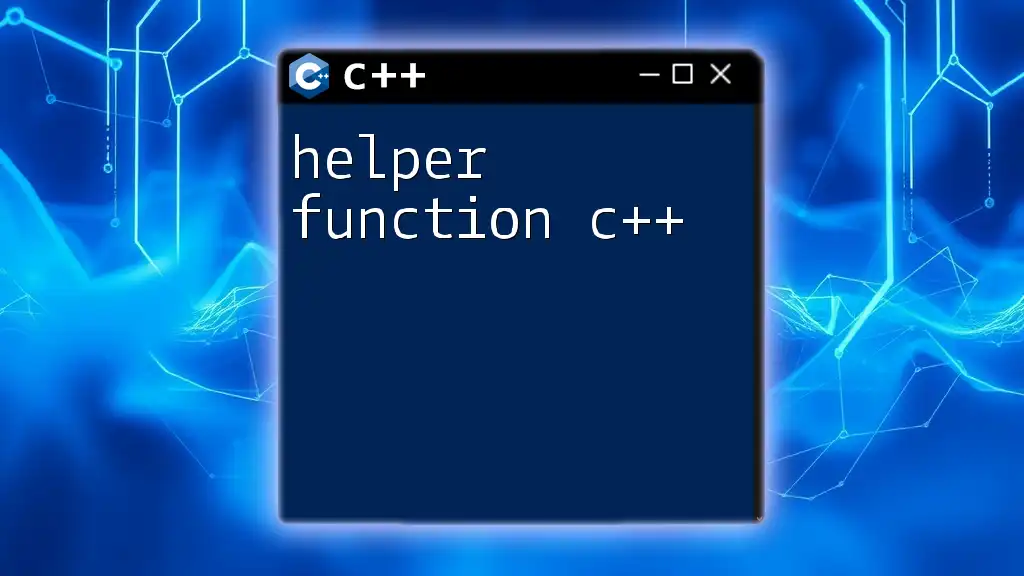
Implementing Getter and Setter Functions in C++
What are Setter Functions?
Setter functions, or "setters," complement getters by allowing controlled modification of private member variables. While getters retrieve data, setters enable clients to modify it under specified rules.
Full Example of Getters and Setters in C++
Here’s a full example of how to implement both getter and setter functions within a C++ class. The class `User` will contain private members and respective getter and setter functions:
class User {
private:
std::string name;
int age;
public:
// Getter for name
std::string getName() const {
return name;
}
// Setter for name
void setName(const std::string& newName) {
name = newName;
}
// Getter for age
int getAge() const {
return age;
}
// Setter for age
void setAge(int newAge) {
if (newAge >= 0) {
age = newAge;
}
}
};
In this `User` class, `getName()` and `getAge()` retrieve private member variables, while `setName` and `setAge` allow controlled updates. The setter for age includes input validation to ensure that age cannot be set to a negative value.
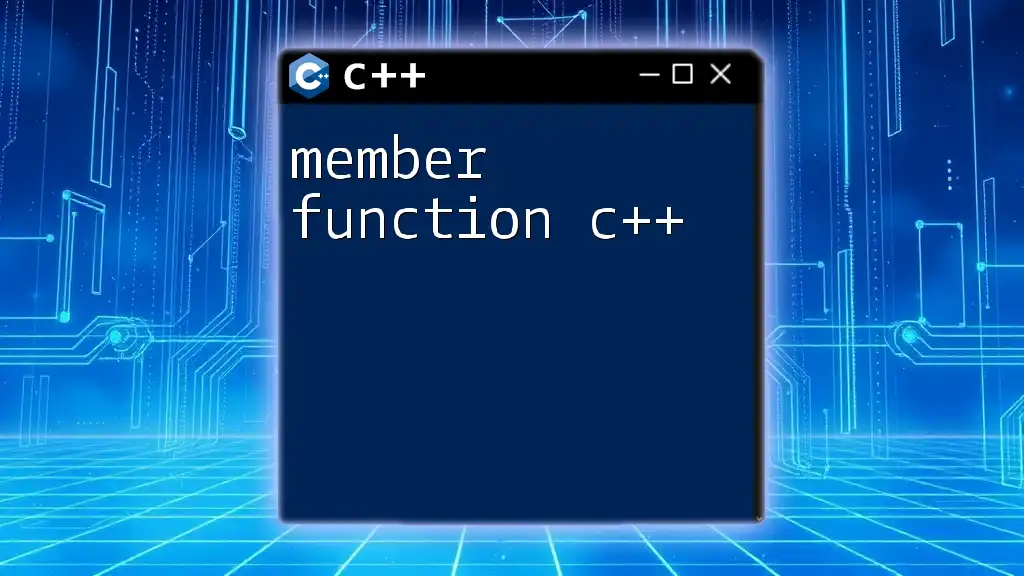
Best Practices for Implementing Getters and Setters in C++
Naming Conventions
When creating getter and setter functions, adopt a consistent naming pattern. Generally, use the prefix "get" for getters followed by the member variable name (e.g., `getName`) and "set" for setters followed by the variable name (e.g., `setName`). This clarity helps maintain readability.
When to Use Const Qualifier
Using the `const` keyword is a best practice for getter functions, as it formally indicates that the function will not modify the object's state. This can prevent unintended side effects and assists consumers of the class to understand the function’s behavior.
Avoiding Unnecessary Complexity
Keep getter functions straightforward and focused solely on retrieving data. Overly complex getter implementations can confuse users and violate the principle of simplicity.
Access Control
Ensure the appropriate use of encapsulation by declaring member variables as private or protected while providing public access through getters and setters. This design protects internal implementation while still allowing necessary interaction from the class's users.
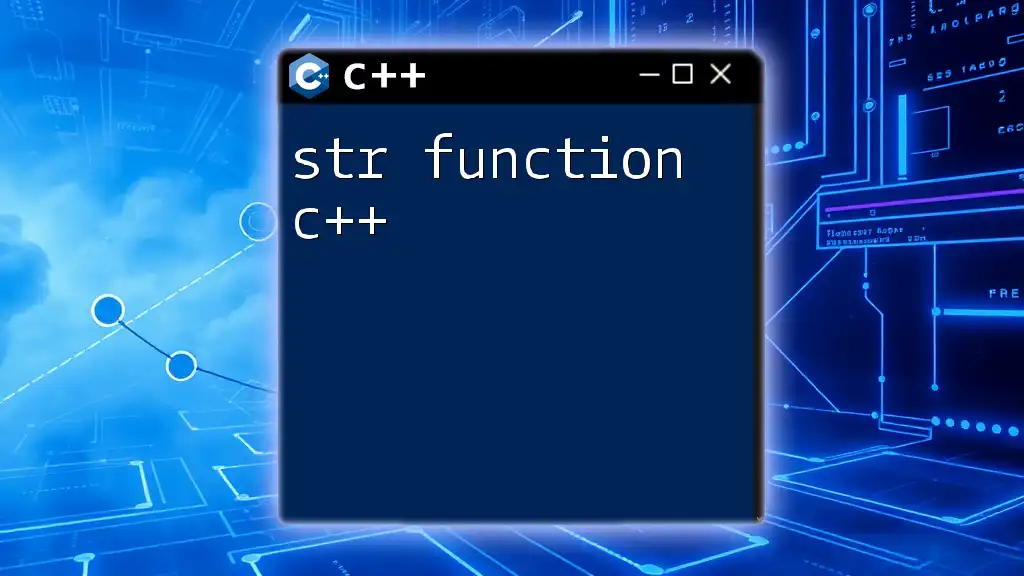
Common Mistakes to Avoid
Overusing Getters and Setters
While getters and setters are useful, relying on them excessively can expose too much of a class's internal data structure, leading to violations of encapsulation. Use them judiciously to maintain control over how data is accessed and manipulated.
Neglecting Input Validation in Setters
Always validate inputs in setter functions. Failing to do so can result in inconsistent state and corrupted data. For instance, the setter for age must ensure that it only accepts non-negative integers, as demonstrated in the earlier example.
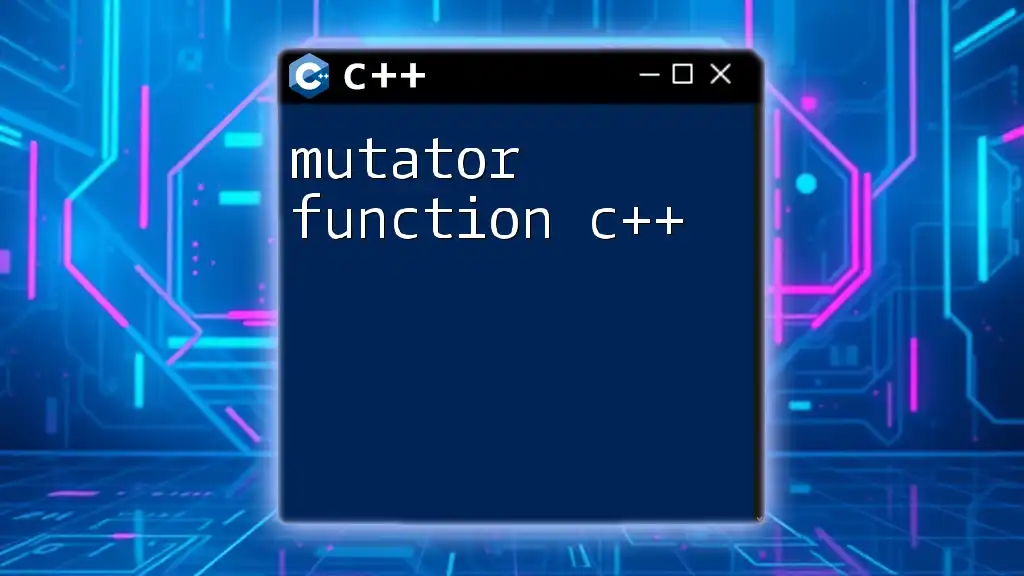
Conclusion
In C++, getter functions play a pivotal role in maintaining the integrity and encapsulation of class data. By utilizing getters and setters effectively, you can achieve greater flexibility and security in your object-oriented programming.
Encourage yourself to practice implementing getters and setters in various scenarios, as doing so will deepen your understanding and proficiency with object-oriented principles in C++.
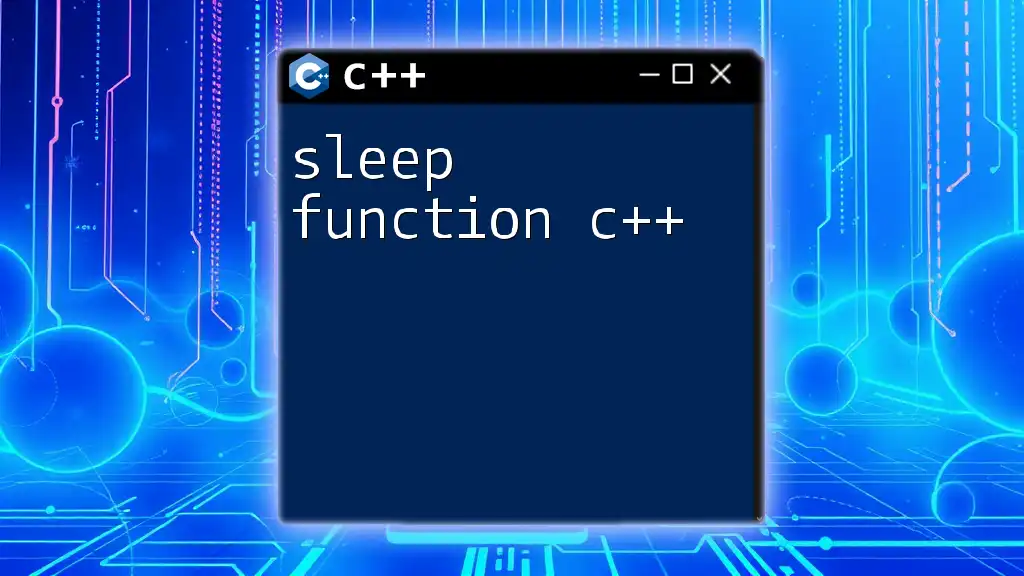
Resources and Further Reading
Books
Consider reading "Effective C++" by Scott Meyers and "C++ Primer" by Stanley B. Lippman for a comprehensive dive into encapsulation and object-oriented design principles.
Online Resources
Explore C++ reference websites and tutorials that focus on best practices for getters and setters in C++, helping you further refine your coding techniques.
Forums and Community
Engage with online C++ communities, such as Stack Overflow and C++ subreddits, where you can ask questions, share insights, and learn from the experiences of seasoned developers.