In C++, a function is a reusable block of code that performs a specific task and can be defined using a return type, function name, and parameters.
Here’s a simple example of a function that adds two integers:
int add(int a, int b) {
return a + b;
}
What is a Function in C++?
A function in C++ is a block of code designed to perform a specific task. Functions allow programmers to break their code into smaller, manageable sections that can be reused throughout the program, improving code organization and readability. By utilizing functions, programmers enhance code reusability and can easily debug and maintain their code.
Characteristics of Functions
Functions have several defining characteristics:
- Modularity: Functions allow you to compartmentalize tasks, making code easier to read and maintain.
- Reusability: Once defined, functions can be reused in different parts of the program, reducing redundancy.
- Abstraction: Functions hide the complex logic from the user, offering a simpler interface.
- Scope: Variables defined within a function are typically local to that function, preventing conflicts with other parts of the code.
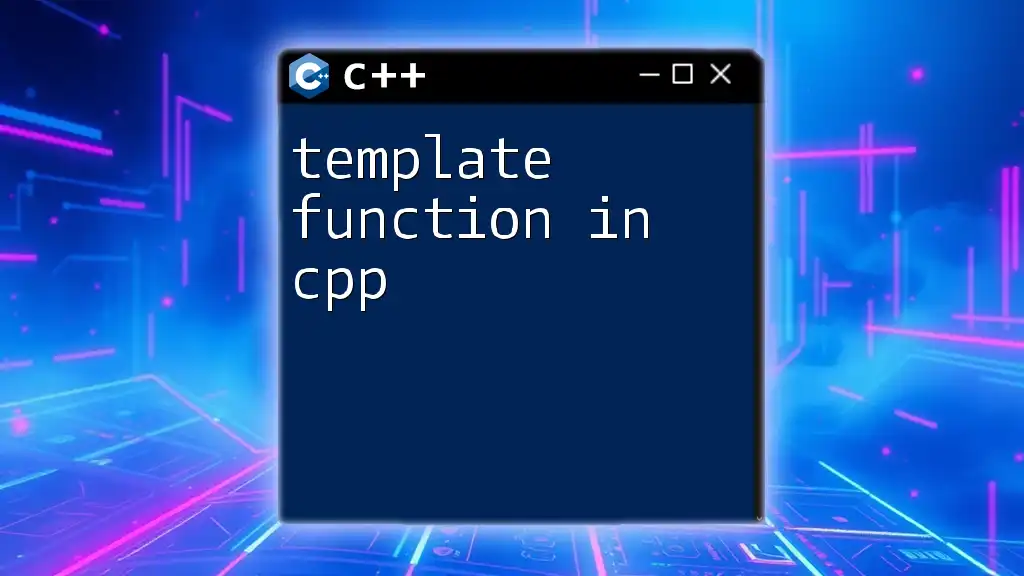
Structure of a Function
To write a function in C++, you need to adhere to a certain syntax which consists of several components.
Syntax of a Function
Here is the basic syntax for defining a function in C++:
return_type function_name(parameter_list) {
// function body
}
Components of the Syntax:
- Return Type: Specifies the type of value the function will return. Use `void` if no value is returned.
- Function Name: The identifier used to call the function. It should be descriptive of its functionality.
- Parameter List: A comma-separated list of input parameters the function takes. If no parameters, an empty set of parentheses is used.
- Function Body: The block of code that defines what the function does, enclosed in curly braces.
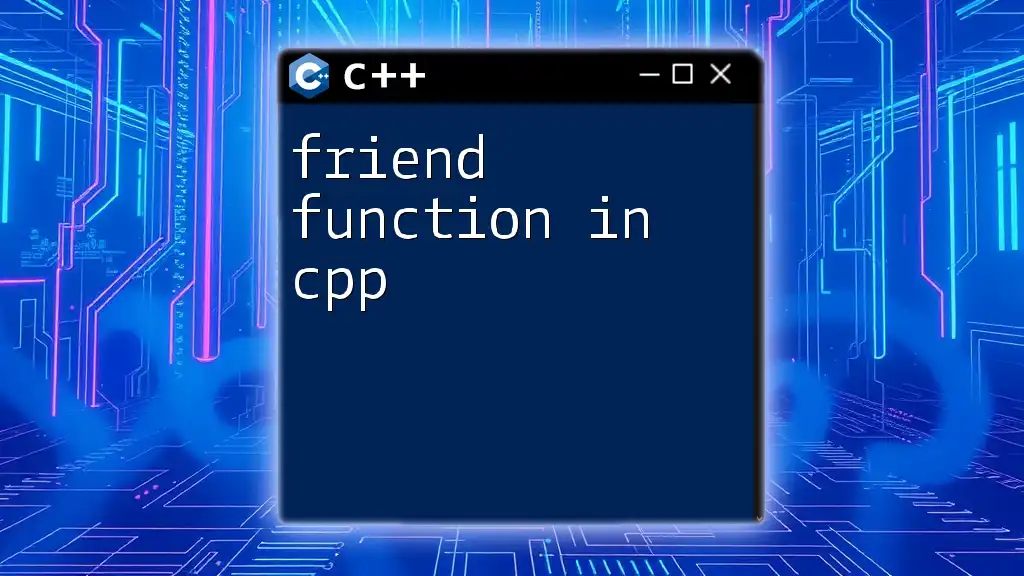
Types of Functions in C++
C++ supports several types of functions that serve different purposes.
Built-in Functions
C++ comes with a rich library of built-in functions, which are predefined functions that perform various tasks out of the box. For example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // 'cout' is a built-in function
return 0;
}
These functions are a part of the standard library and can be used directly in your code to perform various general operations.
User-defined Functions
User-defined functions are those that you create to perform specific tasks. They allow you to encapsulate code designed for a specific purpose, enhancing logic clarity and reusability across your application.
For example, a simple user-defined function to add two numbers could look like this:
int add(int a, int b) {
return a + b;
}
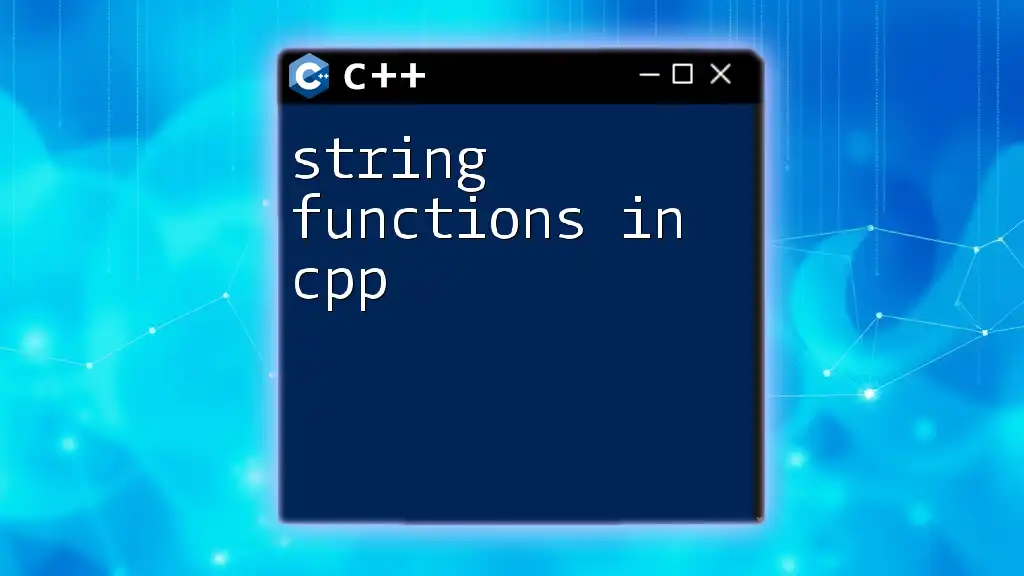
Function Declaration and Definition
In C++, functions can be declared before they are defined, which is known as a function declaration.
Function Declaration
A function declaration tells the compiler about the function’s name, return type, and parameters. It is typically placed before the main function or at the start of the code.
int add(int, int); // function declaration
Function Definition
The function definition provides the actual body of the function where the logic is implemented. It can be placed after the function's first call in the main program or anywhere if a prior declaration exists.
int add(int a, int b) {
return a + b; // function definition
}
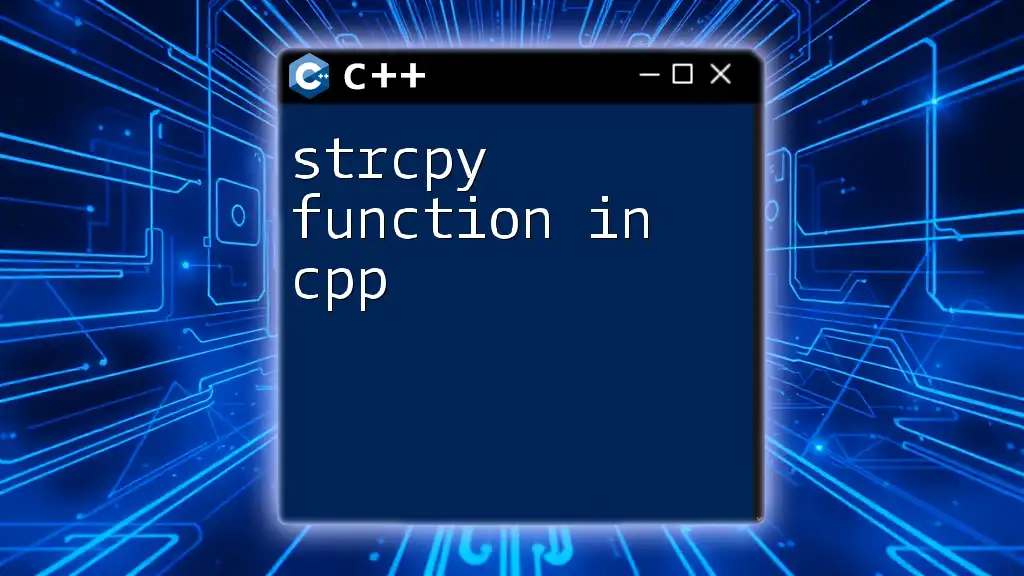
Function Parameters and Arguments
What are Parameters?
Parameters are placeholders in your function definition. They allow you to pass values into functions, which can then be utilized within their body. Understanding the difference between formal parameters (defined in the function definition) and actual parameters (the values you pass when calling the function) is crucial for effective function design.
Passing Arguments to Functions
When you call a function, you pass arguments that correspond to the parameters defined in that function.
Types of Argument Passing
-
Pass by Value: In this method, a copy of the actual parameter’s value is passed to the function. This means changes made to the parameter inside the function do not affect the original variable.
void increment(int value) { value++; } int main() { int num = 5; increment(num); cout << num; // Outputs: 5, as original variable 'num' is unaffected }
-
Pass by Reference: This method passes the address of the actual parameter. Any changes made to the parameter inside the function directly affect the original variable.
void increment(int &value) { value++; } int main() { int num = 5; increment(num); cout << num; // Outputs: 6, as original variable 'num' is modified }
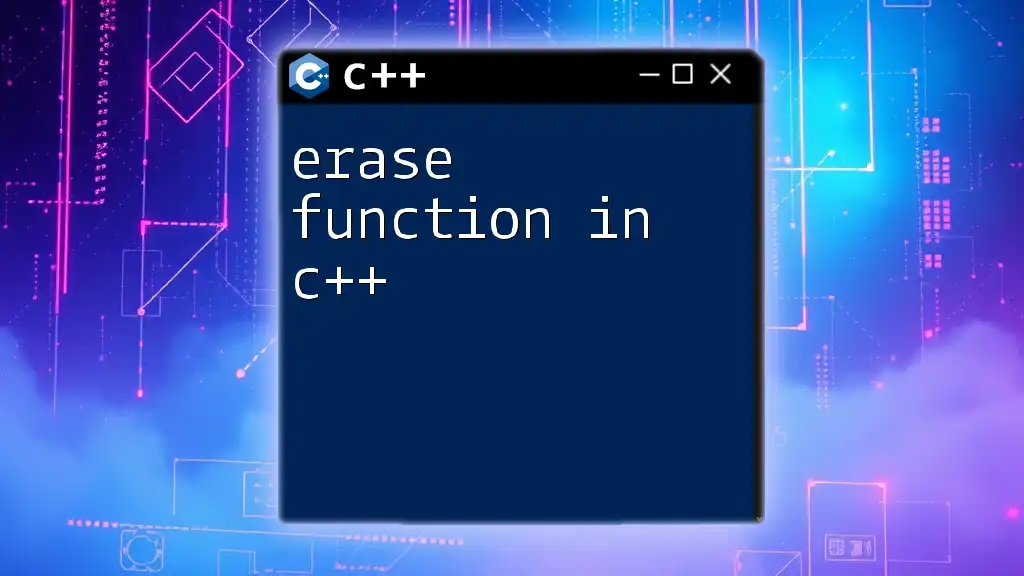
Return Values in Functions
Understanding Return Types
Functions can return values based on their return type. If a function is defined to return a value, it must contain a `return` statement that specifies the value to be returned.
Examples showcasing return values
A simple function to multiply two numbers can be written as follows:
int multiply(int a, int b) {
return a * b; // Returning the product
}
You can call this function from `main` and store its return value:
int main() {
int result = multiply(3, 4);
cout << "Result: " << result; // Outputs: Result: 12
}
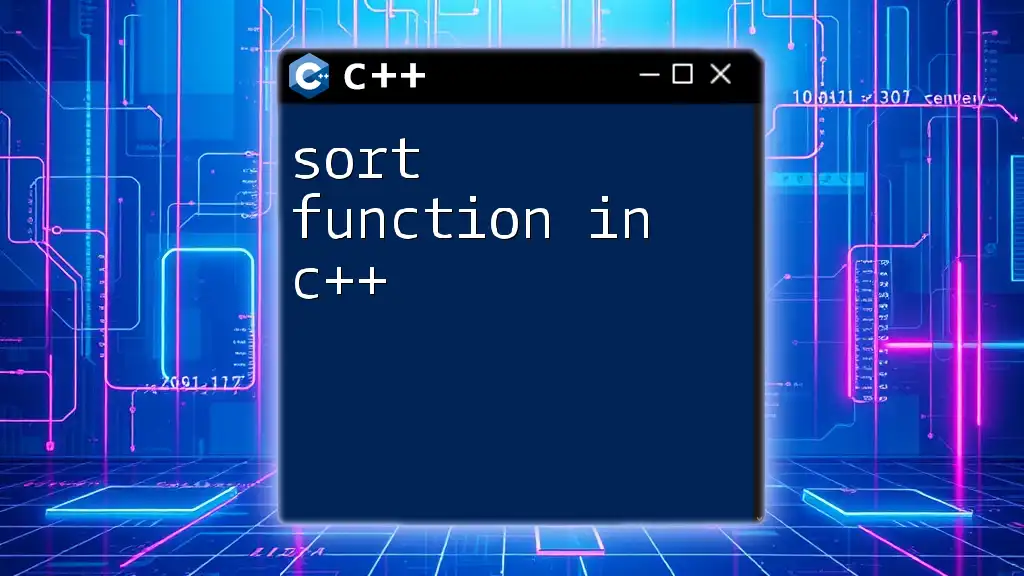
Scope and Lifetime of Functions
Function Scope
Scope refers to the visibility of variables within your program. In C++, variables declared in a function are local to that function. They cannot be accessed outside its body. Conversely, global variables can be accessed throughout the program.
Lifetime of a Function Variable
The lifetime of a variable indicates how long the variable exists in memory. Variables defined within a function are destroyed once the function exits. However, variables declared with the `static` keyword retain their values across function calls:
void counter() {
static int count = 0; // Static variable
count++;
cout << count << endl; // Always retains value across calls
}
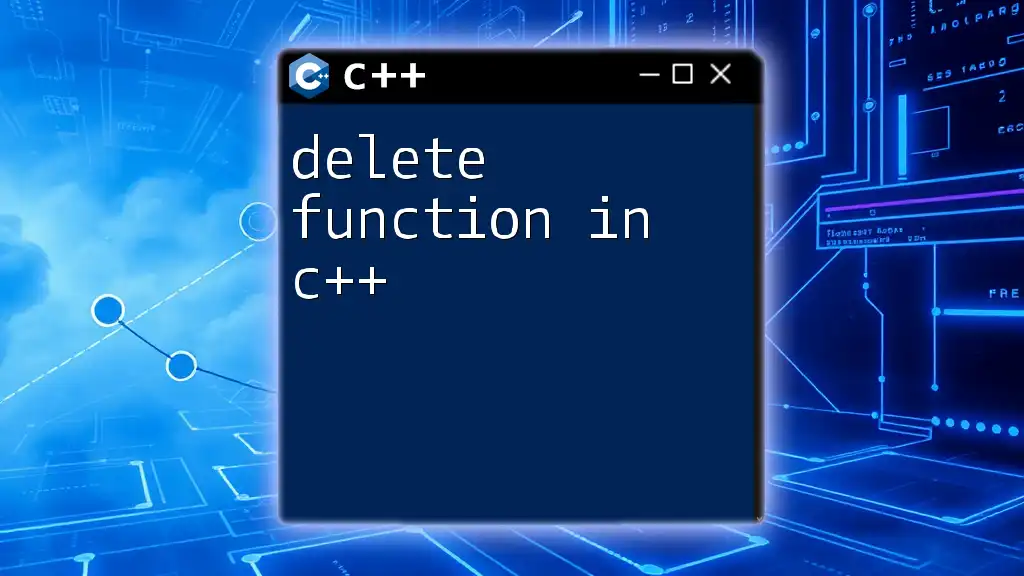
Function Overloading
What is Function Overloading?
Function overloading is a feature that allows multiple functions to have the same name but different parameters. This enables you to create multiple ways to execute similar tasks, making your code cleaner and more intuitive.
How to Create Overloaded Functions
You can define overloaded functions by changing the number or types of parameters. Here’s an example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
In this case, both functions share the name `add`, but they can be distinguished by their parameter types.
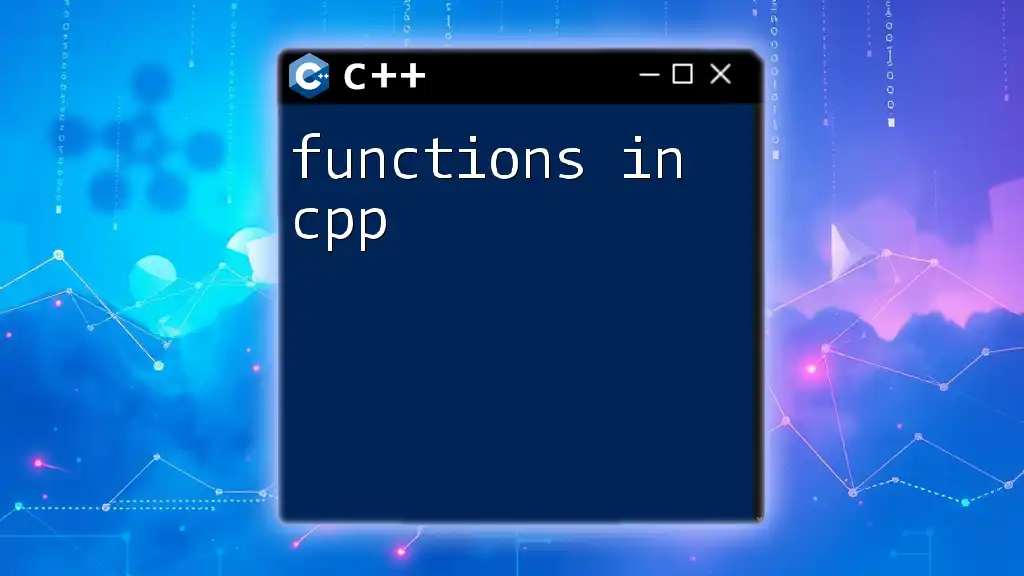
Recursion in C++
Introduction to Recursion
Recursion is a programming technique where a function calls itself in order to solve smaller instances of the same problem. This approach can simplify complex problems, but it's essential to have a base case to avoid infinite loops.
How to Write a Recursive Function
Here's an example of a recursive function that calculates the factorial of a number:
int factorial(int n) {
if (n == 0) return 1; // Base case
return n * factorial(n - 1); // Recursive call
}
You can call this function in the `main` program:
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "Factorial: " << factorial(num) << endl;
}
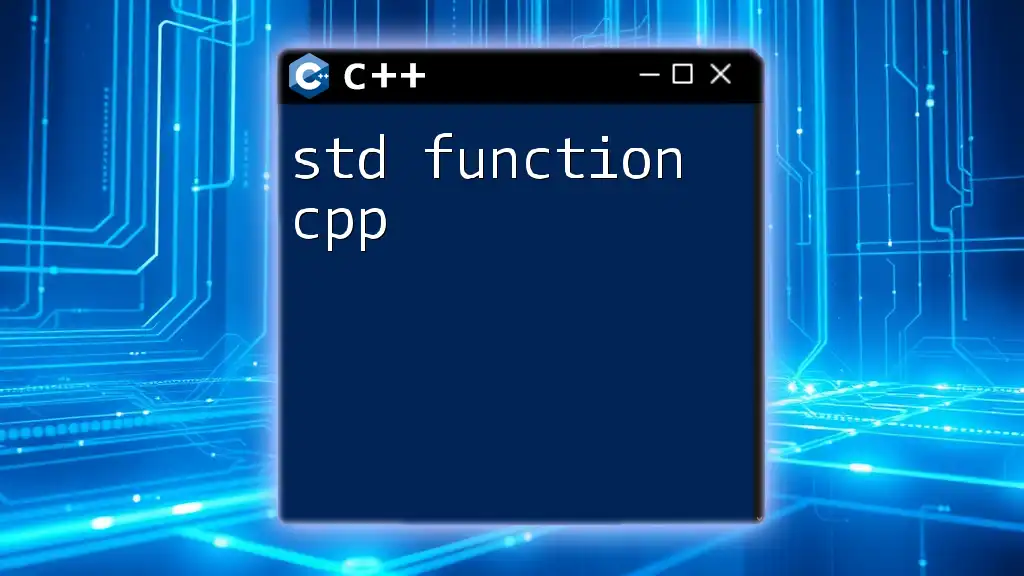
Conclusion
In this article, we've explored how to write functions in C++. We've gone through the definitions, structures, types, and intricacies of function usage, including advanced topics such as recursion and function overloading. Mastering these concepts is critical for effective programming in C++, as they contribute significantly to code organization, reusable design, and improved debugging. We encourage you to practice by creating your functions and experimenting with various parameters and return types to solidify your understanding.
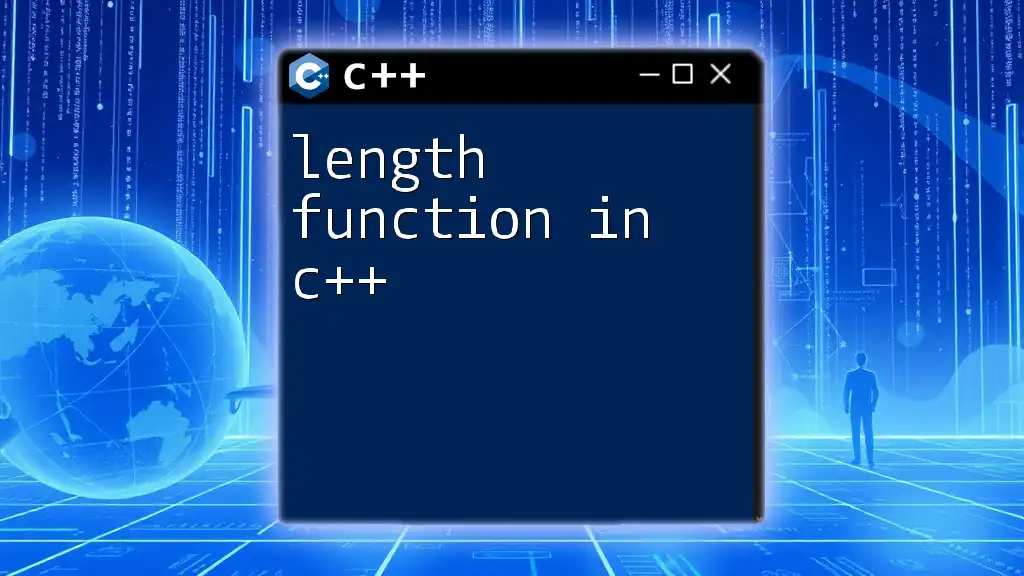
Additional Resources
For further learning, consider delving into recommended books and online tutorials that offer interactive experiences, coding challenges, and projects to help reinforce the concepts you've learned here. The world of C++ is vast, and with practice, you'll surely become proficient in harnessing the power of functions in your programming toolkit.