A template function in C++ allows you to create a generic function that can operate with any data type, promoting code reusability and type safety.
Here’s a simple example of a template function that swaps two values:
#include <iostream>
using namespace std;
template <typename T>
void swap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
swap(x, y);
cout << "x: " << x << ", y: " << y << endl; // Output: x: 10, y: 5
return 0;
}
Introduction to Template Functions in C++
Template functions in C++ represent a powerful feature designed to enable developers to write generic and reusable code. By allowing a function to operate on different data types without redundancy, template functions increase code reusability, ensure type safety, and often yield improved performance.
Definition of Template Functions
A template function is essentially a blueprint for creating functions that can be applied to any data type. Instead of writing multiple versions of a function, one for each data type, a single template function can adapt to various types when called.
Purpose and Benefits of Using Template Functions
- Code Reusability: Write once, use multiple times. This minimizes duplication and enhances maintainability.
- Type Safety: Template functions respect C++’s strong typing, helping to catch errors during compile time rather than runtime.
- Performance Advantages: Templates can lead to better performance due to optimizations by the compiler.

Understanding the Syntax of Template Functions
Basic Syntax of a Template Function
The syntax of a template function may appear slightly complex at first, but it is straightforward once broken down:
template <typename T>
T functionName(T parameter) {
// function body
}
- keyword `template`: Indicates that the following code is a template definition.
- `<typename T>`: Introduces a template parameter (`T` in this case), which can represent any data type.
- `T functionName(T parameter)`: The function itself can accept parameters and return types of the unspecified type `T`.
Template Functions with Multiple Parameters
Template functions can also be defined for multiple types, increasing their versatility. Here’s an example:
template <typename T, typename U>
auto add(T a, U b) -> decltype(a + b) {
return a + b;
}
In this example, `add` can take two parameters of different types (`T` and `U`), and the return type is automatically deduced using `decltype(a + b)`. This is particularly valuable for operations on different numeric types.
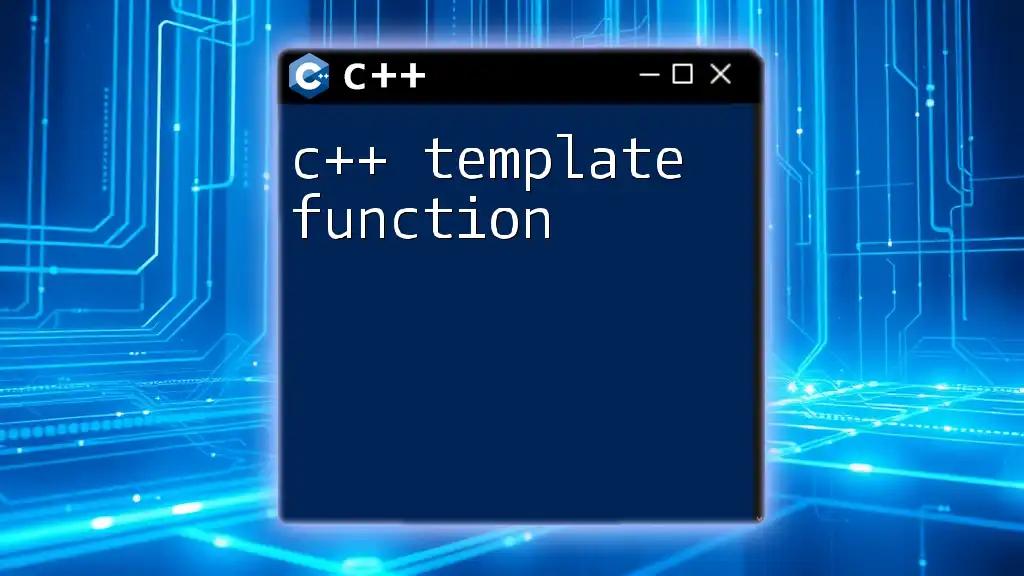
How Template Functions Work
Compilation Process of Template Functions
When you define a template function, the compiler does not generate code until it is instantiated with specific data types. This means that when you call a template function with a certain type, like `int` or `float`, the compiler creates a fresh instance of that function tailored to the specified type.
Example of Template Function Instantiation
For instance, calling the `add` function with two integers:
int result = add(5, 10);
The compiler generates a version of `add` specifically for integers and keeps it for future use.
Template Specialization
Template specialization allows different implementations of a template function for specific types.
What is Template Specialization?
This can be accomplished in two ways: full specialization (defined specifically for one type) and partial specialization (defined for a subset of types, such as all pointers or all classes).
Example of Template Specialization
Here’s a simple example of full specialization:
template <>
void display<double>(double value) {
std::cout << "Specialized double value: " << value << std::endl;
}
In this snippet, a specialized version of `display` exists just for `double` values, providing specific functionality when this data type is used.
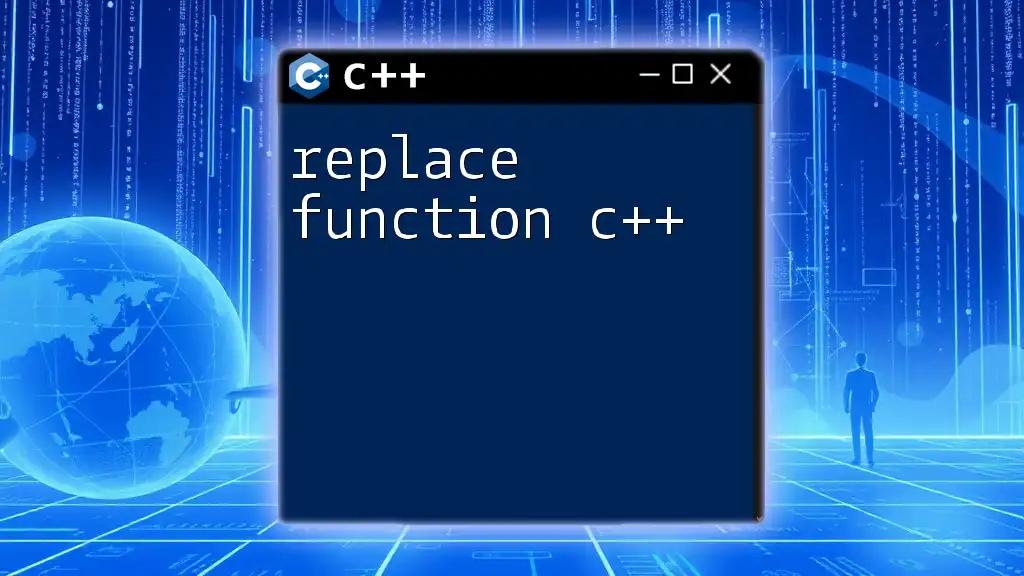
Use Cases for Template Functions
Common Use Cases
Template functions are commonly utilized in various scenarios:
- Creating Generic Data Structures: Allowing a data structure to manage different types, such as linked lists or stacks that can store any data type.
- Algorithms: Many algorithms in C++, like sorting or searching, can be written generically to work with any comparable data types.
Real-World Example: Sorting Function
Here's a generic bubble sort implementation:
template <typename T>
void bubbleSort(T arr[], int n) {
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
std::swap(arr[j], arr[j+1]);
}
This function sorts an array of any comparable type, demonstrating the flexibility of template functions.
Advantages in Libraries and Frameworks
The Standard Template Library (STL) in C++ heavily relies on template functions. Collections like vectors, lists, and maps benefit from templates by supporting diverse data types.
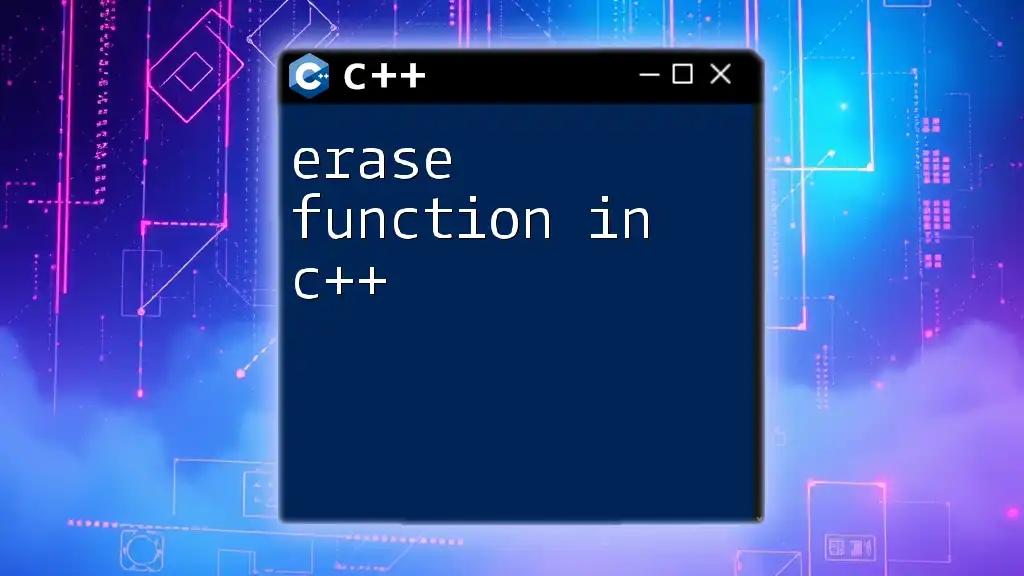
Advanced Template Function Concepts
Variadic Templates in C++
Variadic templates enable templates to accept an arbitrary number of template parameters. This feature provides immense flexibility for functions that need to operate over an unknown number of inputs.
What are Variadic Templates?
These templates utilize an ellipsis `...` to accept any number of arguments.
Example of Variadic Templates
Here’s a simple example of a function that can print different datatypes:
template<typename... Args>
void print(Args... args) {
(std::cout << ... << args) << std::endl;
}
This leverages a fold expression to print all arguments passed to the function.
Type Traits and SFINAE (Substitution Failure Is Not An Error)
Type traits allow developers to enable or disable template instantiations based on types.
Using Type Traits to Enhance Template Functions
Using type traits can create more robust and type-checked templates.
Example of SFINAE Implementation
template<typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
process(T value) {
// Function specifically for integral types
}
The `process` function will only compile for integral types, avoiding errors during instantiation for non-integer types.

Best Practices for Using Template Functions
Naming Conventions and Documentation
When working with template functions, clear naming is essential. Functions should communicate their purpose transparently, and documentation should clearly explain template parameters.
Avoiding Common Pitfalls
While template functions are powerful, they can lead to certain pitfalls:
- Overuse: Excessive templating can result in code bloat, where compilation time and binary size become an issue.
- Compiler Errors: Template errors are often less clear than non-template errors. Using clear and concise code structures can mitigate confusion and improve maintainability.

Conclusion
Template functions in C++ provide a robust way to write generic, type-safe, and reusable code. By understanding their syntax, functionality, and best practices, you can leverage this powerful feature effectively in your projects. Practice writing and using template functions to discover their full potential and appreciate their vast possibilities for creating efficient and maintainable code.

Additional Resources
For further exploration of template functions, consider looking into books and online courses focused on advanced C++ concepts. Engaging with community forums can also provide insight into real-world applications and resolve specific queries you may have. Check official C++ documentation for deeper understanding and updated practices.