A function stub in C++ is a placeholder function that has the same signature as the intended function but is implemented with minimal code, often used during the early stages of development for testing purposes.
void exampleFunction(int a) {
// Function stub: implementation to be added later
}
What is a Function Stub?
Definition of Function Stub
A function stub in C++ is a placeholder for a function that has not been implemented yet. It provides a way to declare a function's existence without providing its complete definition. This approach allows developers to outline the structure of their programs without needing to write extensive code immediately.
Purpose of Function Stubs
Function stubs serve several purposes in software development:
- They enable high-level design of a program. By outlining function stubs, developers can visualize how various components will interact.
- Stubs act as temporary replacements for functions that are yet to be coded, allowing teams to proceed with development without getting bogged down.
- In unit testing, stubs can emulate the behavior of functions that are still in development, facilitating early validation of other components in the application.
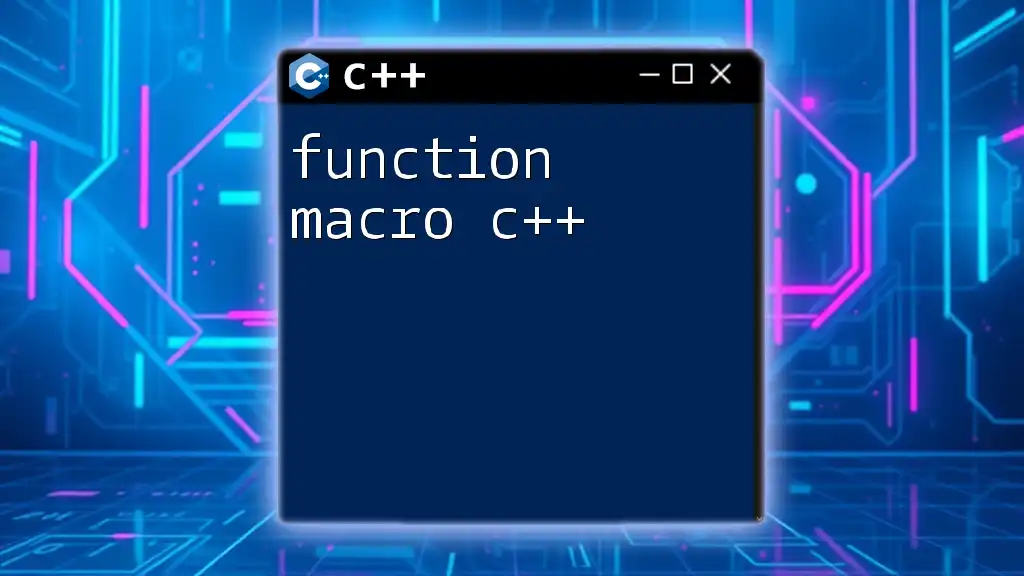
Creating a Function Stub in C++
Basic Syntax of a Function Stub
The syntax for defining a function stub is straightforward. A stub consists of the function signature—this includes the function name and its parameter types, but it lacks the function body.
For example:
void myFunction();
In this snippet, `myFunction` is declared but not implemented. The function stub specifies that `myFunction` will take no arguments and return no value.
Implementing Function Stubs with Return Types
Function stubs can also specify return types. For instance, consider a function designed to add two integers:
int add(int a, int b);
Here, `add` takes two integer parameters and is expected to return an integer, but no implementation details are provided. This allows other code to compile and run without error, even if `add` isn't fully defined yet.
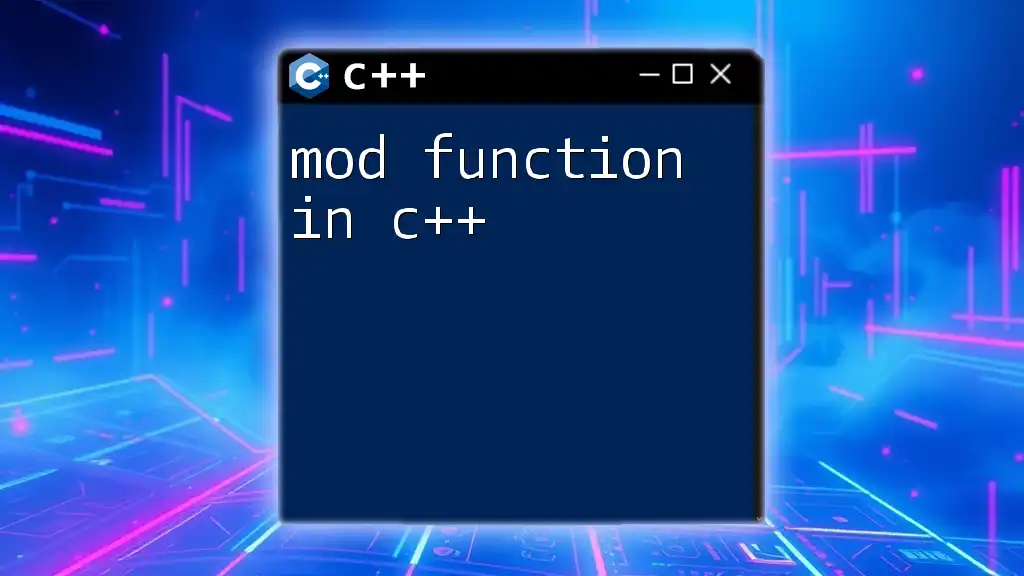
Advantages of Using Function Stubs
Simplifying the Development Process
Function stubs streamline the development process by allowing developers to concentrate on high-level logic before delving into low-level details. Developers can draft the overall flow of their programs and address the logic behind the scenes without needing to flesh out every function immediately. This method fosters collaboration; multiple team members can work on different modules simultaneously without waiting for one another to finish their code.
Facilitating Unit Testing
One of the most significant benefits of function stubs is their role in unit testing. When a function is stubbed, developers can create tests that ensure other modules behave as expected, even when some functions are still being developed. For instance, if a function `calculateSum(int a, int b)` is stubbed, developers can still test the modules dependent on `calculateSum` to ensure they correctly call and handle the result from the stubbed version.
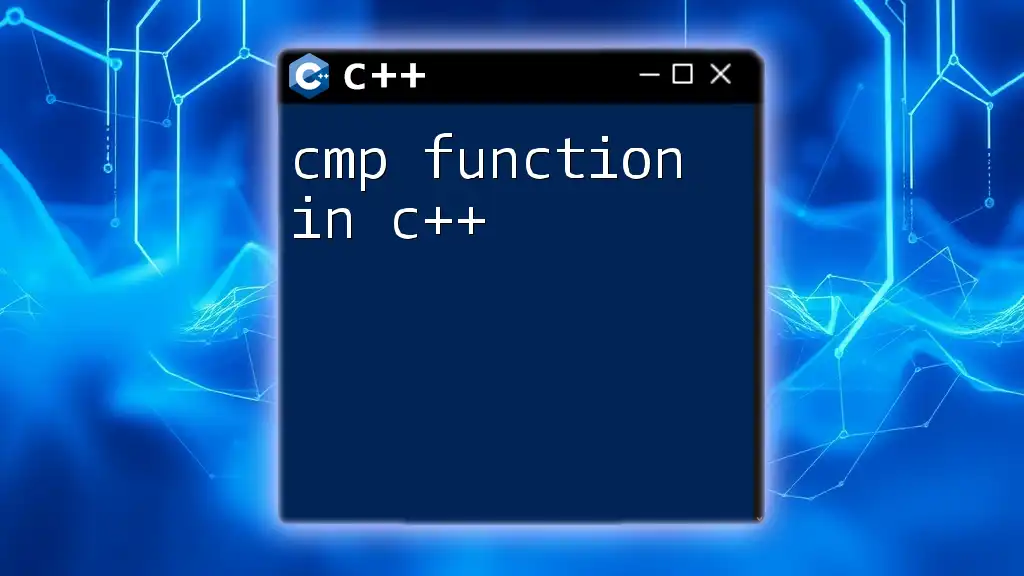
Real-World Examples
Example 1: Stubbing a Function for Testing
Let’s consider a scenario in which we want to test a function that calculates the sum of two integers using a stub.
int calculateSum(int a, int b) {
return a + b; // Stubbed function
}
void testCalculateSum() {
assert(calculateSum(2, 3) == 5);
}
In this example, `calculateSum` is a simple stub that provides the expected behavior, allowing us to run tests on its usage without waiting for the complete logic to be implemented.
Example 2: Using Stubs in Larger Projects
Function stubs are particularly valuable in larger projects where multiple functions interact. For instance, consider the following example where we define stubs for future implementation:
void processInput(int value); // Stub for future implementation
void validateData(int data) {
// Validation logic
}
Here, `processInput` is declared but not yet defined. This allows the `validateData` function to be implemented without needing `processInput` to be fully formed, enabling parallel development efforts.
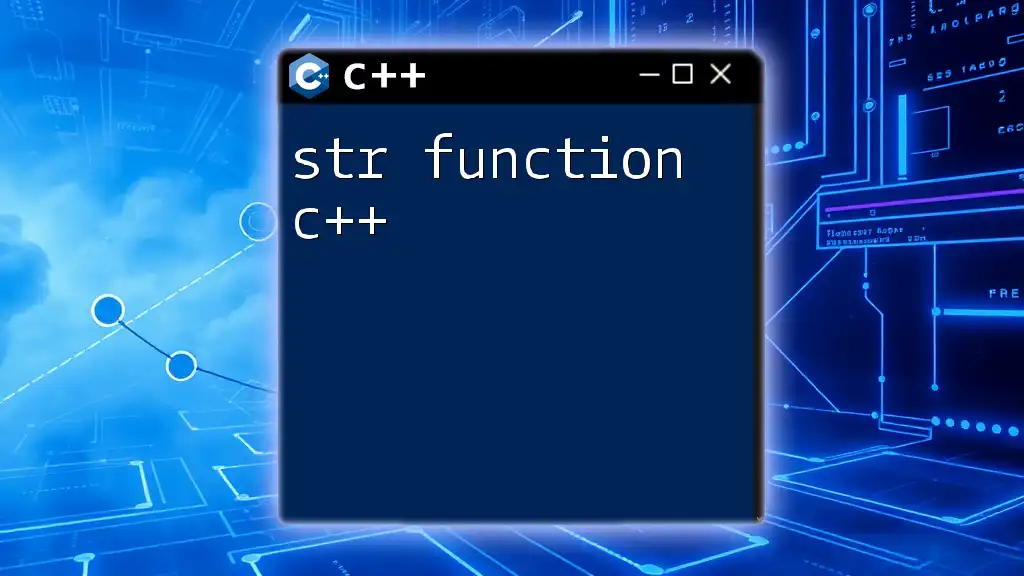
Best Practices for Using Function Stubs
Keeping Stubs Simple
When creating function stubs, it is crucial to maintain simplicity. Avoid incorporating complex logic or additional features into a stub, as its purpose is merely to serve as a placeholder. A clear and concise stub aids in understanding without introducing distractions.
Regularly Updating Stubs
As development progresses, stubs should be replaced with the actual implementations in a timely manner. Failing to do so may lead to technical debt, where stale or incomplete code accumulates over time, making the codebase harder to maintain or understand.
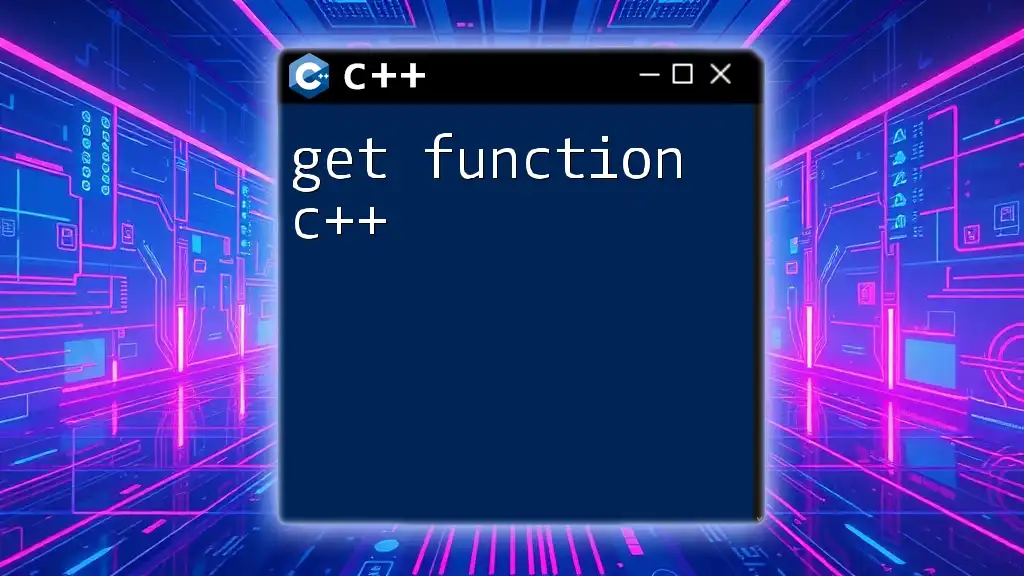
Common Pitfalls to Avoid
Overusing Function Stubs
While function stubs are beneficial, they should not be overused. Relying too heavily on stubs can lead to a situation where the actual code becomes neglected. This can cause confusion and make it difficult to understand how the final code behaves, especially for new team members.
Misunderstanding the Purpose of Stubs
It’s essential to clarify that stubs are intended as temporary placeholders. They should not be mistaken for mocks or fakes, which simulate specific behaviors of other functions or classes intended for testing. Stubs should generally return benign or default responses rather than mimic complex logic.
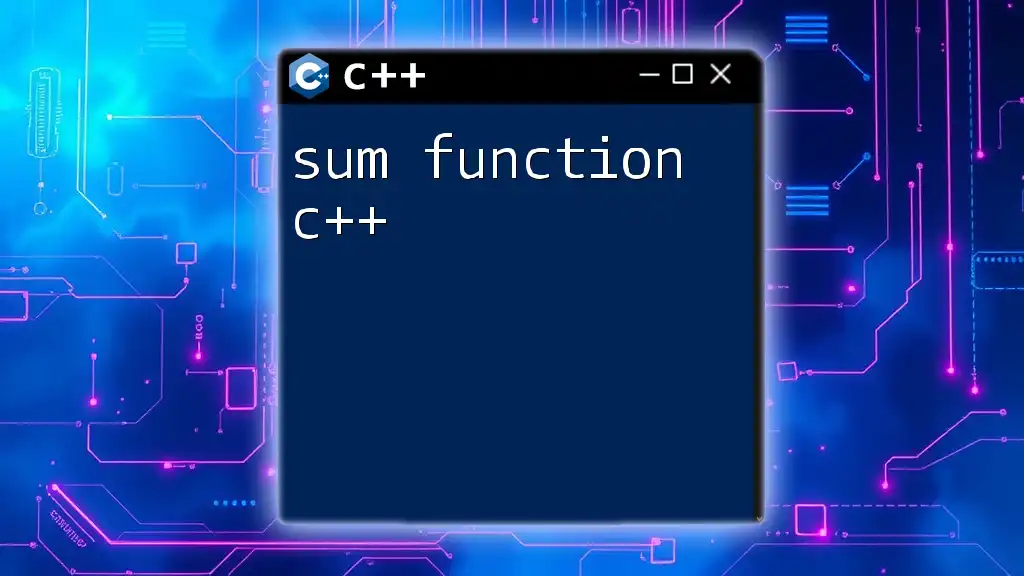
Conclusion
Understanding and effectively using function stubs in C++ is crucial for software development and testing. They enable developers to outline and test their applications without needing complete implementations. By creating stubs, programmers can facilitate collaboration, ensure code quality through unit tests, and maintain a clear focus on the application's architecture. As you dive into your own projects, practice creating function stubs to better structure your code and enhance your development process.
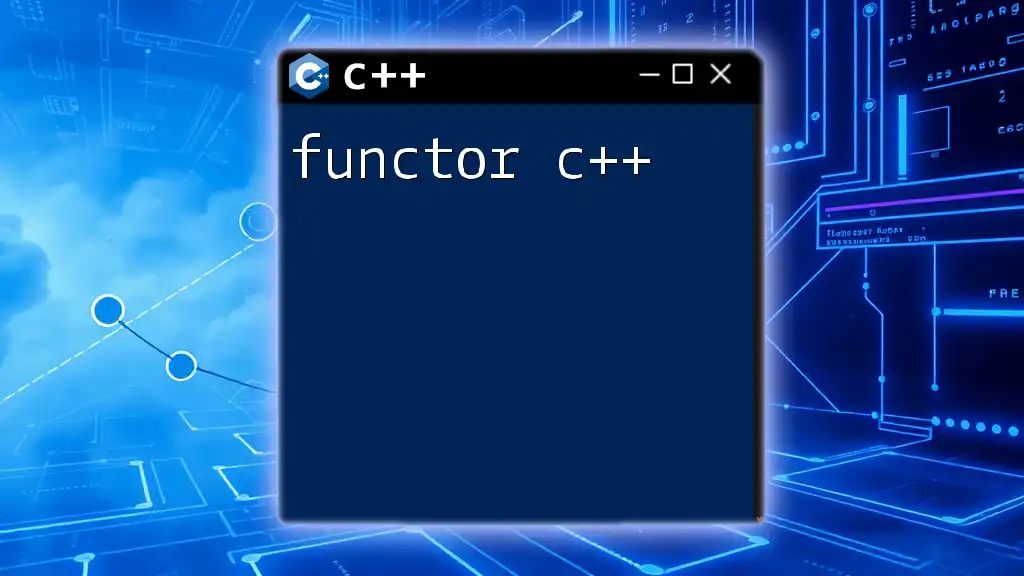
Additional Resources
For further development and testing in C++, consider exploring tutorials on software architecture, Agile methodologies, and advanced unit testing techniques to deepen your understanding and application of programming best practices.