Function prototyping in C++ is the declaration of a function's name, return type, and parameters before its actual implementation, allowing the compiler to understand how to handle calls to the function.
Here’s a simple example:
int add(int a, int b); // Function prototype
int main() {
int result = add(5, 3); // Function call
return 0;
}
int add(int a, int b) { // Function definition
return a + b;
}
What is Function Prototype in C++?
A function prototype in C++ is a declaration of a function that informs the compiler about the function's name, return type, and parameters. The function prototype allows the compiler to know what to expect before the function is actually defined, serving as a promise that the function will be defined later in the code.
Purpose and Benefits of Using Function Prototypes
Function prototypes provide several key advantages:
-
Clarity: They help make your code more readable and understandable at a glance. With prototypes in place, other developers (or you, in the future) can quickly grasp how functions interact without digging into their implementations.
-
Code Organization: By separating function declarations from definitions, you can maintain a cleaner, more organized codebase. This is especially helpful in larger programs where functions may be defined far from where they are used.
-
Early Error Detection: If you make a mistake in how you've defined a function after its prototype, the compiler can catch these issues early, preventing runtime errors.
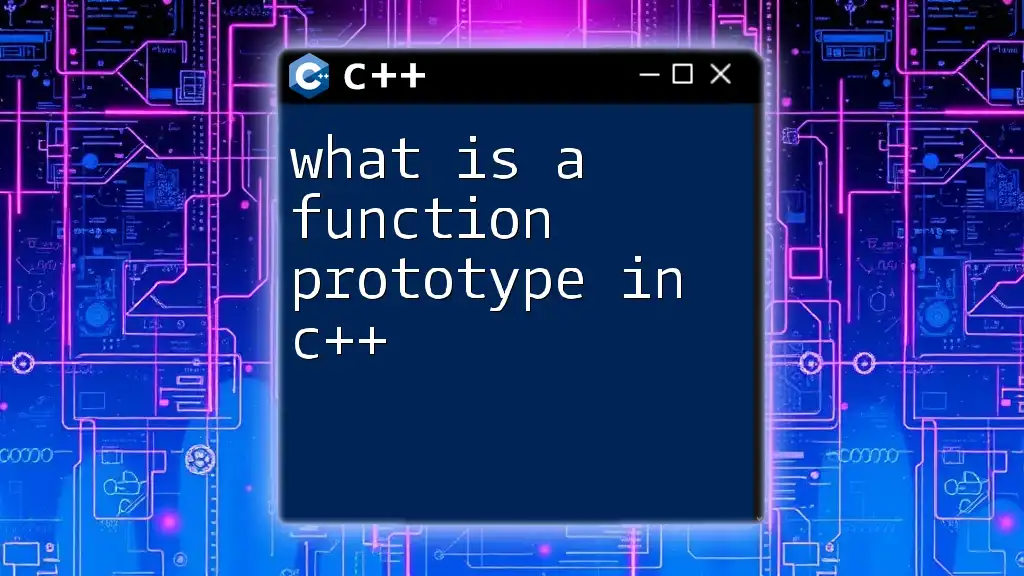
Syntax of a Function Prototype
The general format for a function prototype in C++ is as follows:
return_type function_name(parameter_type1 parameter_name1, parameter_type2 parameter_name2, ...);
Each part serves a specific purpose:
-
Return Type: Indicates the type of data that the function will return after processing. For example, `int` signifies that the function will return an integer.
-
Function Name: A descriptive label that identifies the function. This should be meaningful to convey what the function does.
-
Parameters: Listed within parentheses, these specify the types and names of the inputs the function will accept. Each parameter is defined in the format `parameter_type parameter_name`.
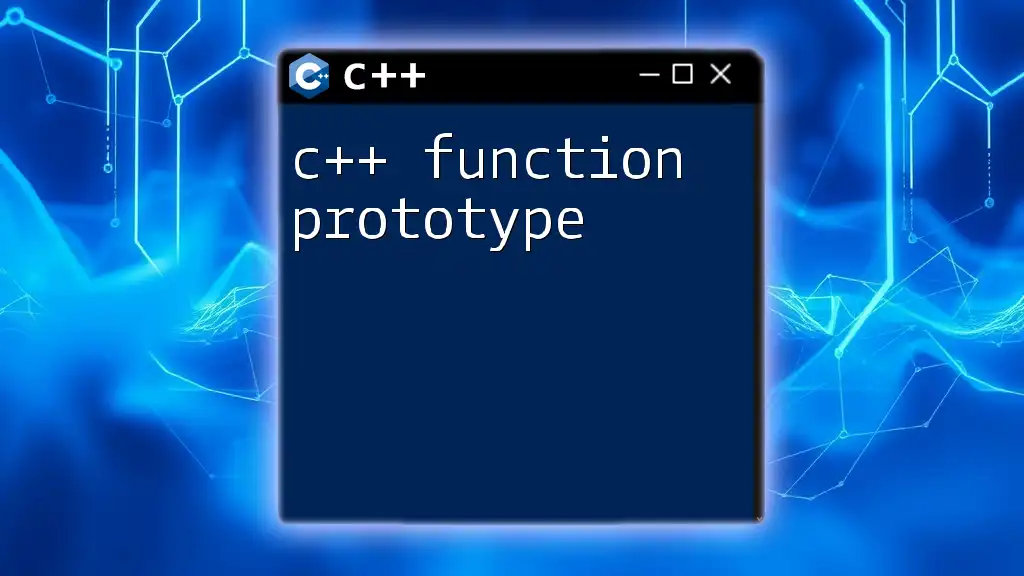
Why Use Function Prototypes?
The importance of function prototypes extends beyond mere syntax. They enhance code quality in several ways:
-
Improved Readability: Prototypes allow developers to read and comprehend the function’s intended behavior without getting lost in implementation details.
-
Facilitates Function Declaration Before Usage: Especially in larger codebases, it is not always practical to define functions in the order they are used. Prototypes enable proper compilation even when a function is called before its definition appears in the code.
-
Support for Recursive Functions: If a function calls itself (as in recursion), you must provide a prototype declaration before its first call; otherwise, the compiler will raise an error.
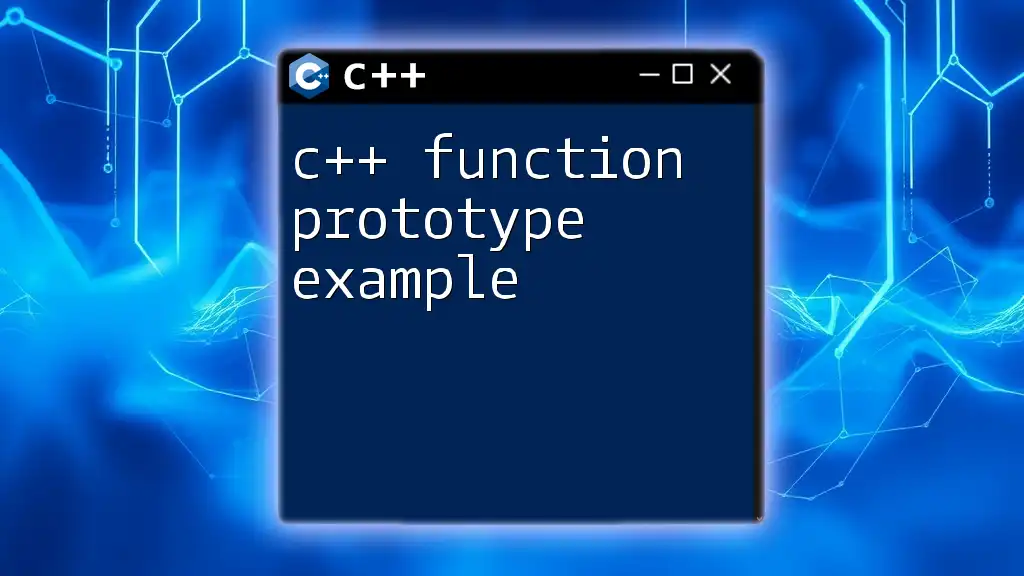
Example of a Function Prototype
Here's a straightforward example illustrating function prototypes:
int sum(int a, int b);
In this case, the prototype declares a function named `sum` that takes two integer parameters (`a` and `b`) and returns an `int`.
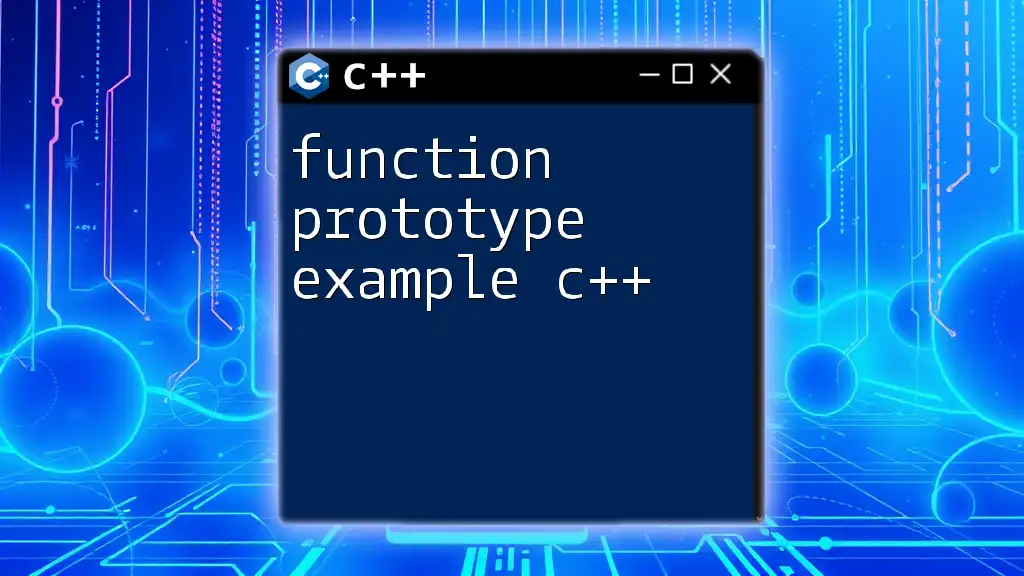
Using Function Prototypes in Your Code
Example of Implementing a Function Prototype
To understand how function prototypes work in practice, consider the following complete program:
#include <iostream>
// Function prototype
int sum(int, int);
int main() {
int result = sum(5, 10);
std::cout << "Sum: " << result << std::endl;
return 0;
}
// Function definition
int sum(int a, int b) {
return a + b;
}
In this program, the `sum` function prototype is declared before the `main` function. This informs the compiler that a function called `sum` exists and will be defined later on. Inside `main`, we call `sum(5, 10)`, which ultimately returns `15`. The definition of `sum` follows after, showcasing how the prototype and definition are integrated.
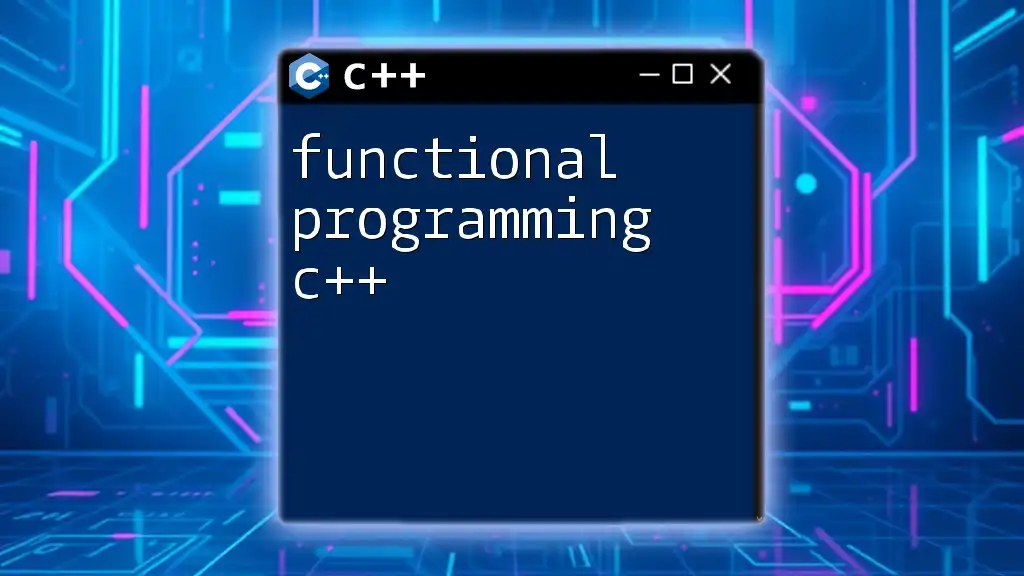
Common Mistakes with Function Prototypes
New C++ developers often encounter common pitfalls with function prototypes, such as:
-
Mismatched Return Types: If the return type specified in the prototype does not match the actual return type in the definition, it will lead to compilation errors and unexpected behavior.
-
Parameter Mismatch: Errors may arise if the number or types of parameters in the function call do not align with those declared in the prototype. This may result in incorrect computations or crashes.
To avoid these issues, maintain consistency and verify the details of each declaration and definition.
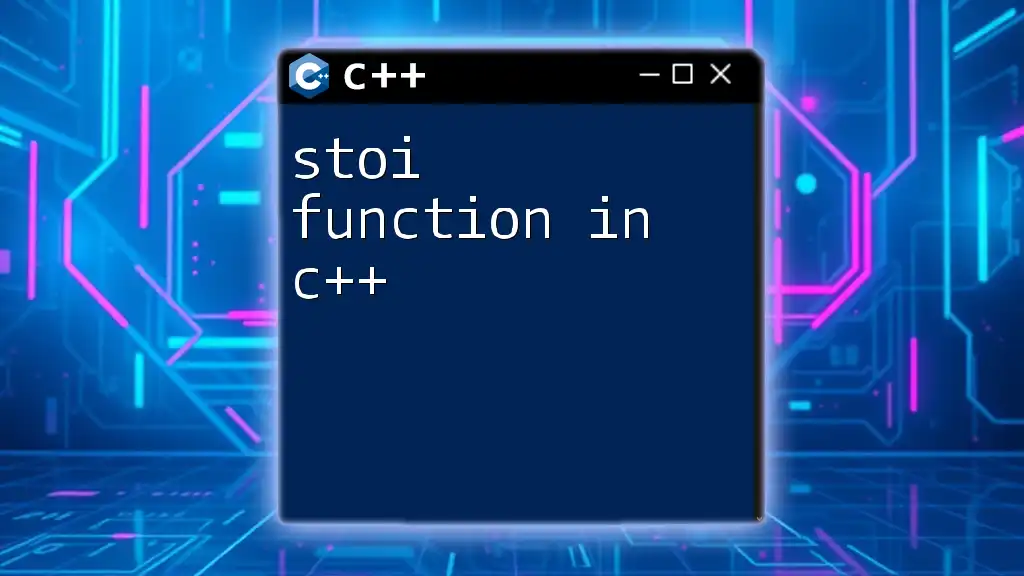
Function Prototypes vs Function Definitions
Understanding the difference between function prototypes and function definitions is crucial for writing effective C++ code.
-
A function prototype merely announces the function to the compiler. It specifies the return type, name, and parameters but does not include the body or details of the implementation.
-
A function definition, on the other hand, includes the complete implementation or body of the function, detailing what the function does.
Here’s an example to differentiate them:
// Function prototype
void displayMessage();
// Function definition
void displayMessage() {
std::cout << "Hello, World!" << std::endl;
}
In the above code, `displayMessage` is declared first with a prototype, then defined with a complete implementation that prints a message to the console.
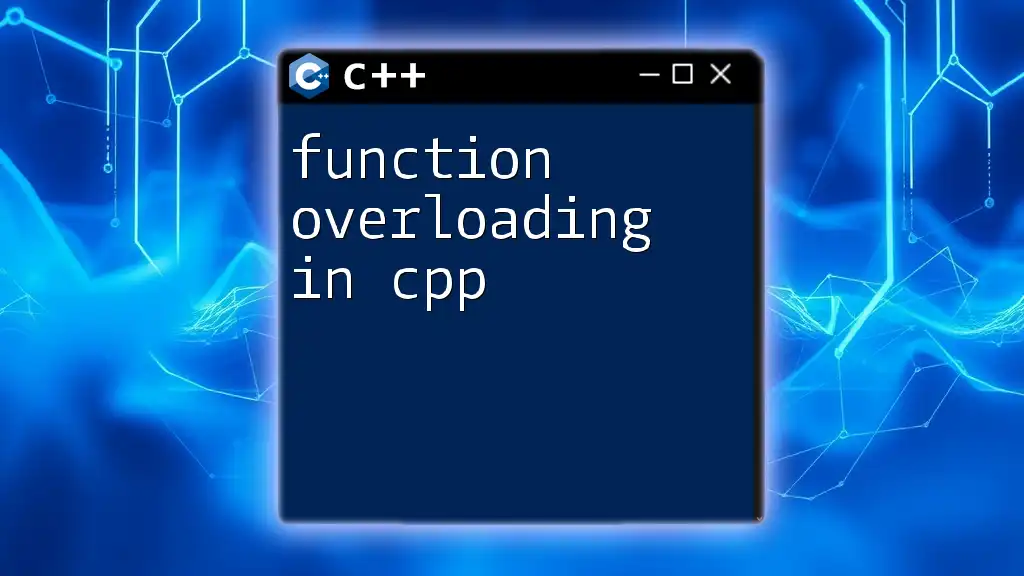
Best Practices for Function Prototyping
To maximize the benefits of function prototypes, consider these best practices:
-
Keep Prototypes at the Top of Your Files: Placing prototypes at the beginning of your source files allows for easy reading and access, ensuring that the function's interface is clear before implementation.
-
Use Clear and Descriptive Names: Function names should intuitively describe their purpose, improving clarity for anyone reading the code.
-
Maintain Consistency in Naming Conventions: Use consistent naming conventions (like camelCase or snake_case) throughout your codebase. This helps in identifying functions and parameters easily.
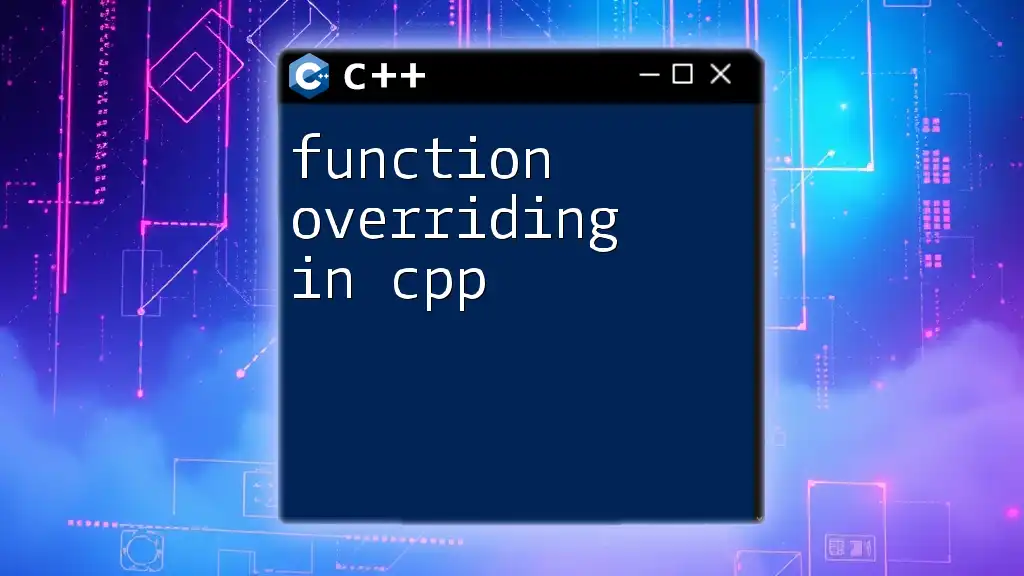
Conclusion
Function prototyping plays a pivotal role in C++ programming, offering clarity, organization, and early error detection. Understanding the syntax, benefits, and best practices of function prototypes will enhance your coding capabilities and lead to more effective software development. By utilizing function prototypes, you not only improve your own coding practices but also facilitate better collaboration and communication within your team.