Function overloading in C++ allows multiple functions to have the same name but differ in the type or number of their parameters, enabling more intuitive and flexible code.
Here’s a simple example of function overloading:
#include <iostream>
using namespace std;
class Print {
public:
void display(int i) {
cout << "Integer: " << i << endl;
}
void display(double d) {
cout << "Double: " << d << endl;
}
void display(string s) {
cout << "String: " << s << endl;
}
};
int main() {
Print obj;
obj.display(5);
obj.display(3.14);
obj.display("Hello, World!");
return 0;
}
Introduction to Function Overloading
Function overloading in C++ allows developers to define multiple functions with the same name but different parameter lists. This powerful feature enhances the readability and reusability of code, allowing programmers to use semantic naming while handling various data types and parameter numbers.

Understanding How Function Overloading Works
Definition of Function Overloading
Function overloading is an example of compile-time polymorphism, where the function to be executed is determined at compile time based on the function signature. A function's signature comprises its name and the number and type of its parameters. The return type alone does not form part of the function’s signature.
Rules for Function Overloading in C++
To successfully implement function overloading, specific rules must be followed:
- Different Parameter Types: Functions can be overloaded if they differ in the types of their parameters.
- Different Number of Parameters: Functions can also be overloaded if they have different numbers of parameters.
- Return Type Considerations: It’s important to note that overloads cannot be resolved solely by a different return type. The parameter list must differ.
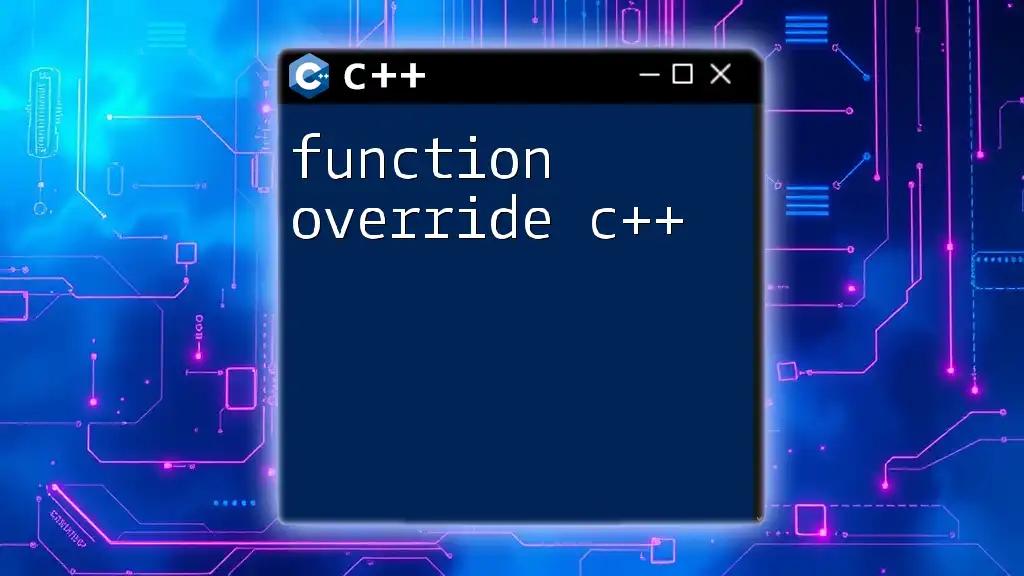
Benefits of Function Overloading
Function overloading provides several key benefits:
- Simplifying Code Maintenance: By using the same function name for similar operations, the maintenance of the code becomes easier and avoids redundancy.
- Improving Function Usability: Overloading allows functions to be more intuitive, letting users of the API understand behavior through consistent naming.
- Reducing the Need for Additional Functions: Without overloading, developers might create numerous differently named functions, making the codebase heavier and harder to navigate.
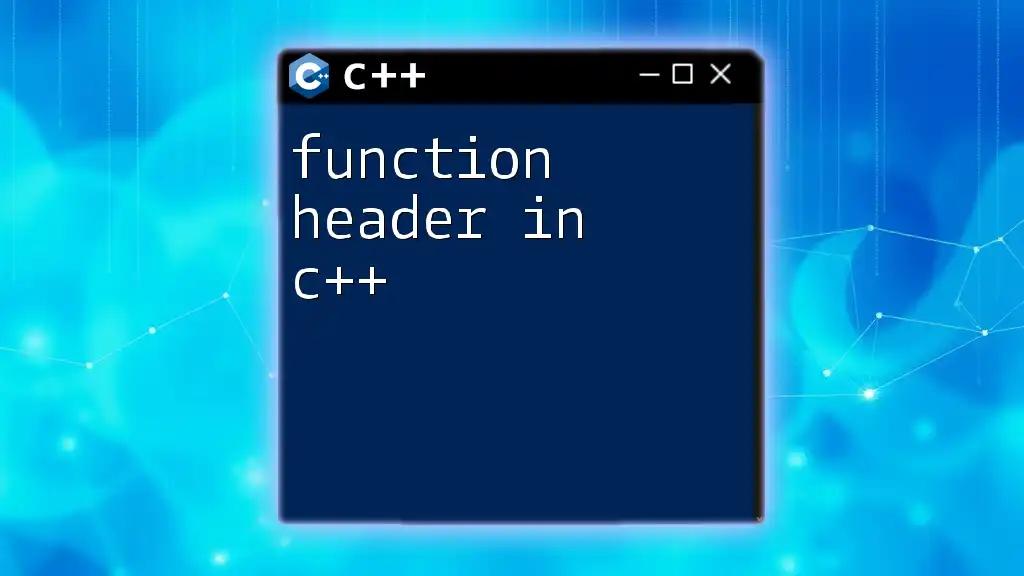
How to Implement Function Overloading in C++
Implementing function overloading in C++ is straightforward and typically involves creating multiple functions sharing the same name but differing in parameters.
The Syntax of Overloaded Functions
Below is a basic syntax example of function overloading:
void display(int num);
void display(double num);
In this example, two `display` functions are defined: one that accepts an integer and another that accepts a double. The compiler can distinguish between them based on the argument passed.

Practical Examples of Function Overloading
Example 1: Overloading with Different Data Types
One common use case for function overloading involves handling different data types:
void printValue(int i) {
cout << "Integer: " << i << endl;
}
void printValue(double d) {
cout << "Double: " << d << endl;
}
In this example, the `printValue` function is defined to accept either an integer or a double. When called, the appropriate function is invoked based on the argument type, demonstrating the flexibility of function overloading in C++.
Example 2: Overloading with Different Number of Parameters
Overloading can also be achieved by varying the number of parameters in the functions:
int add(int a, int b) {
return a + b;
}
int add(int a, int b, int c) {
return a + b + c;
}
In this snippet, two `add` functions are created, one that takes two parameters and another that takes three. This allows users to call `add` with either two or three integers while keeping the code organized and user-friendly.
Example 3: Handling Default Arguments
Function overloading can take advantage of default parameters for additional flexibility:
void volume(int r, int h, double pi = 3.14) {
return pi * r * r * h;
}
In this example, the `volume` function calculates the volume of a cylinder, utilizing a default argument for `pi`. Users can call the function with just the radius and height if they wish, or explicitly pass a different value for `pi`.

Common Mistakes in Function Overloading
When utilizing function overloading, developers must be cautious about common pitfalls:
Ambiguity in Overloading
Whenever an overloaded function is called, the compiler resolves which function to call based on the parameters. If it cannot determine the correct function because of ambiguous parameters, it will result in a compilation error. Always ensure that overloaded functions have distinct signatures.
Overloading with Different Return Types
It’s a common misconception that functions can be overloaded based solely on return type. This is not possible. For example, the following scenarios do not constitute valid overloads:
int func();
double func();
The above might confuse the compiler since the parameter lists are identical. Ensure that overloads differ in parameters.
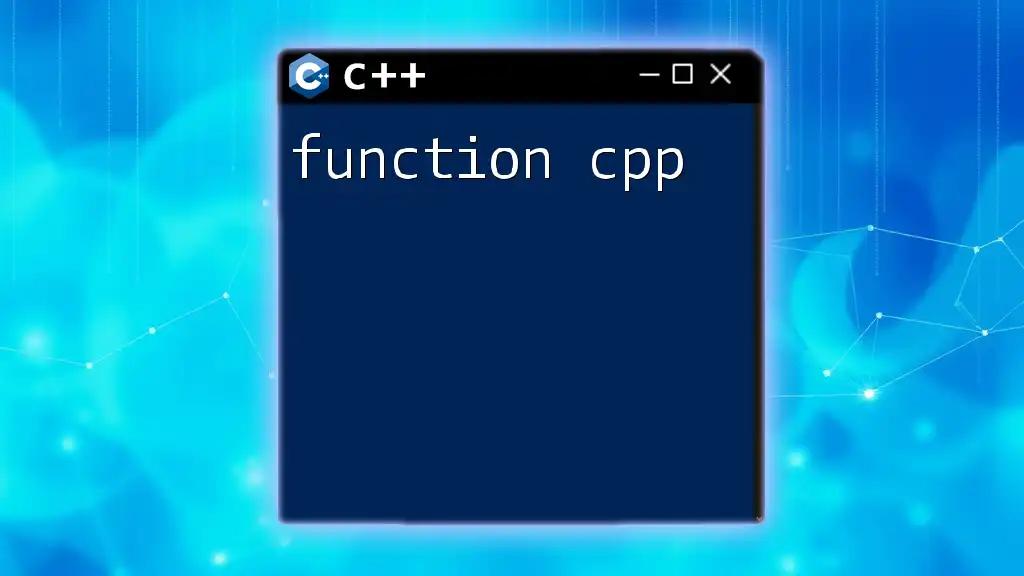
Best Practices for Using Function Overloading in C++
To maximize the benefits of function overloading, consider the following best practices:
-
When to Use Function Overloading: Opt for overloading in scenarios where logically related functionalities can be grouped under one name. This promotes clarity while offering versatility.
-
Design Principles for Effective Overloading: Keep overloads intuitive; functions with similar behaviors should be grouped, and the intent should be clear through the name and parameter types.
-
Ensuring Clarity and Maintainability: Document your overloaded functions sufficiently. This assists others (and yourself in the future) in understanding the differences in behavior among overloaded versions.
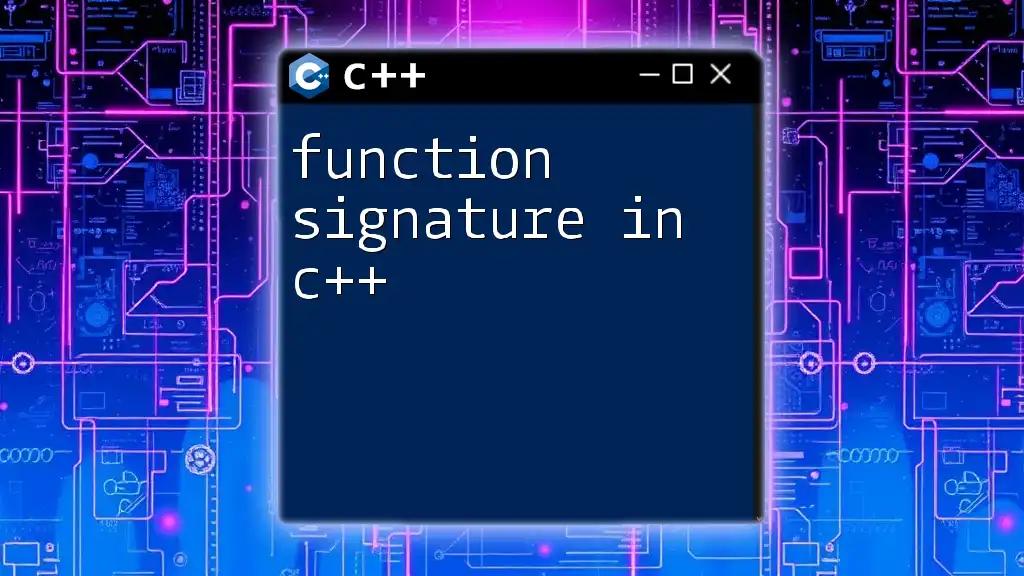
Conclusion
Function overloading in C++ is a critical feature that significantly enhances code efficiency and usability. By being able to define functions that differ in parameters while sharing the same name, developers can write more intuitive and organized code. To master this concept, practice implementing various examples and recognize the potential pitfalls to avoid. Overloading can simplify both code and its maintenance, allowing developers to remain focused on solving problems rather than getting bogged down by complicated function naming schemes.

Additional Resources
To further deepen your understanding, consult recommended books and tutorials on C++ overloading methods or explore online resources that provide exercises and examples. Engaging with community discussions can also offer insights and different perspectives on best practices in C++.