Function overriding in C++ allows a derived class to provide a specific implementation of a function that is already defined in its base class, enabling polymorphism.
#include <iostream>
using namespace std;
class Base {
public:
virtual void show() {
cout << "Base class show function." << endl;
}
};
class Derived : public Base {
public:
void show() override { // Function overriding
cout << "Derived class show function." << endl;
}
};
int main() {
Base* basePtr;
Derived derivedObj;
basePtr = &derivedObj;
basePtr->show(); // Calls Derived's show function
return 0;
}
Understanding the Basics of C++ Function Overriding
What is Function Overriding?
Function overriding in C++ refers to the ability of a derived class to provide a specific implementation of a method that is already defined in its base class. This concept is crucial in Object-Oriented Programming (OOP) as it allows the derived class to customize or extend the behavior of the base class method. It's important to note the distinction between function overriding and function overloading: the former involves redefining a function in a subclass while maintaining the same name and signature, while the latter refers to multiple functions with the same name but different parameters within the same scope.
Key Concepts of Function Overriding in C++
To understand function overriding in C++, it is essential to grasp the roles of virtual functions, base classes, and derived classes.
- Virtual Functions: These are members of the base class that are declared as virtual. They allow derived classes to override them. Virtual functions facilitate dynamic (or late) binding, crucial for implementing polymorphism in C++.
- Base Class vs Derived Class: The base class is the parent class where the original method is defined. The derived class is the child class that inherits from the base class and overrides the method.
- Polymorphism: This OOP concept allows objects of different classes to be treated as objects of a common base class. It can be achieved through function overriding, enabling methods to behave differently based on the object type.
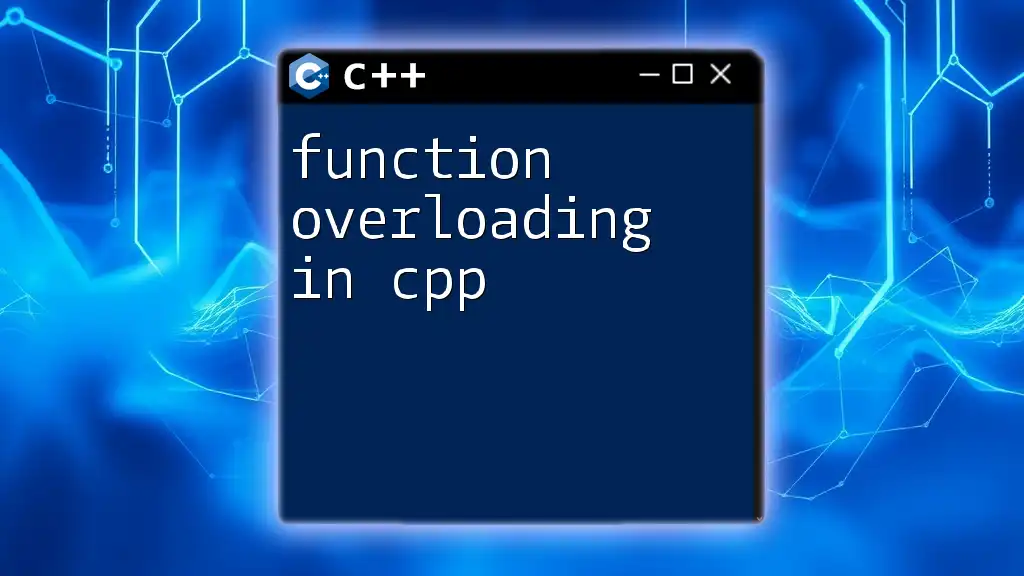
Syntax and Structure for Overriding in C++
Basic Syntax for Overriding Functions in C++
The syntax for function overriding in C++ involves declaring the method in the base class as a virtual function. When inheriting from this base class, the derived class uses the same name and signature but provides its own implementation.
In the base class:
class Base {
public:
virtual void display() { // Base class function
std::cout << "Display from Base Class" << std::endl;
}
};
In the derived class:
class Derived : public Base {
public:
void display() override { // Overriding function
std::cout << "Display from Derived Class" << std::endl;
}
};
Example of C++ Overriding Functions
Consider the following example that highlights the overriding functionality:
class Vehicle {
public:
virtual void start() {
std::cout << "Starting vehicle" << std::endl;
}
};
class Car : public Vehicle {
public:
void start() override {
std::cout << "Starting car" << std::endl;
}
};
In this case, when calling the `start()` method on a `Car` object, the derived class method is executed, demonstrating the overriding mechanism.
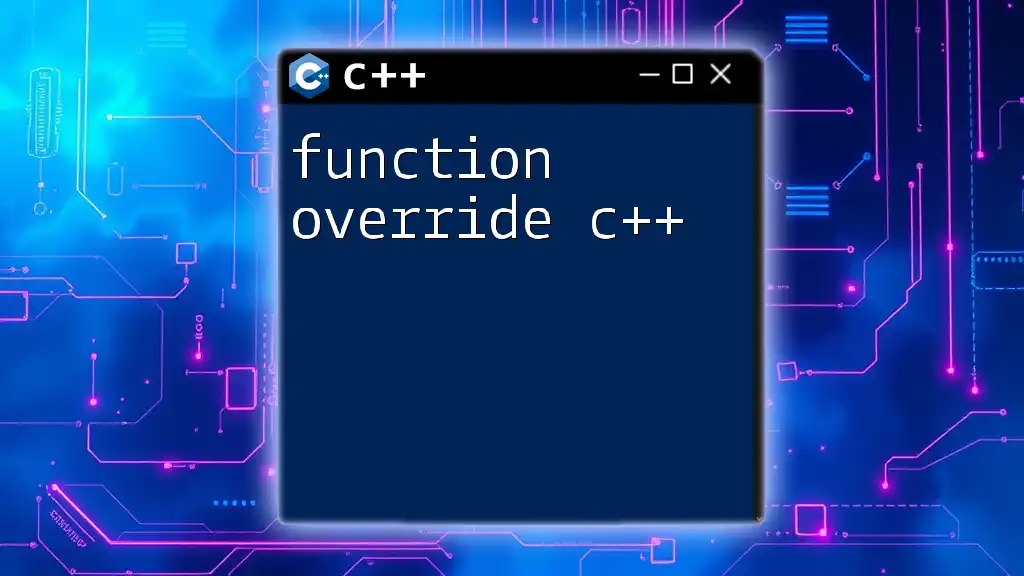
Rules and Best Practices for Function Overriding in C++
Key Rules for Method Overriding in C++
When working with function overriding in C++, certain rules must be adhered to ensure proper functionality:
- Access Specifiers: The overriding function in the derived class cannot have a more restrictive access level than that of the base class function.
- Function Signature: The overridden method in the derived class must match the name, return type, and parameters of the base class function.
- Using the `override` Keyword: This keyword is optional but highly recommended as it helps the compiler catch errors if the function does not actually override a base class function.
Best Practices for Function Overriding
- Always declare functions as virtual in the base class where inheritance is anticipated.
- Use the `override` specifier in the derived class for clarity and safety.
- Be mindful of performance implications, as excessive use of virtual functions can lead to slight overhead due to dynamic dispatch.
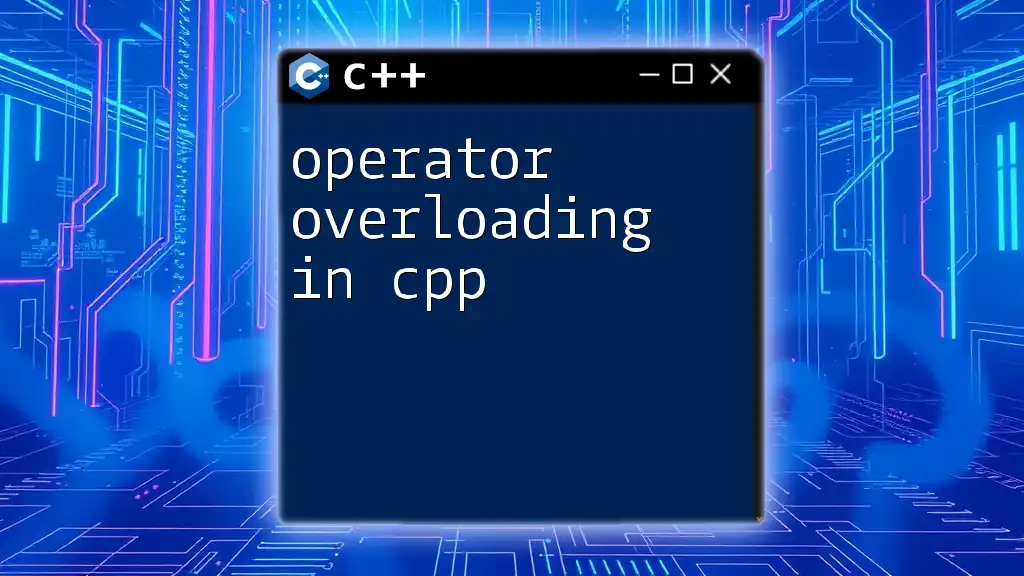
Examples Demonstrating Function Overriding in C++
Basic Example of Function Overriding
Here's a straightforward example that demonstrates how function overriding works:
class Animal {
public:
virtual void sound() {
std::cout << "Animal makes a sound" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Dog barks" << std::endl;
}
};
In this example, the `sound` method in the `Dog` class provides a specific implementation that overrides the generic `sound` method in the `Animal` class.
Advanced Example: Abstract Classes and Overriding
An abstract class in C++ is a class that contains at least one pure virtual function. Here's a practical example of how to utilize abstract classes in conjunction with function overriding:
class Shape {
public:
virtual void area() = 0; // Pure virtual function
};
class Triangle : public Shape {
public:
void area() override {
std::cout << "Calculating area of Triangle" << std::endl;
}
};
In this case, the `Shape` class defines a pure virtual function `area()` that must be overridden by any derived class, such as `Triangle`.
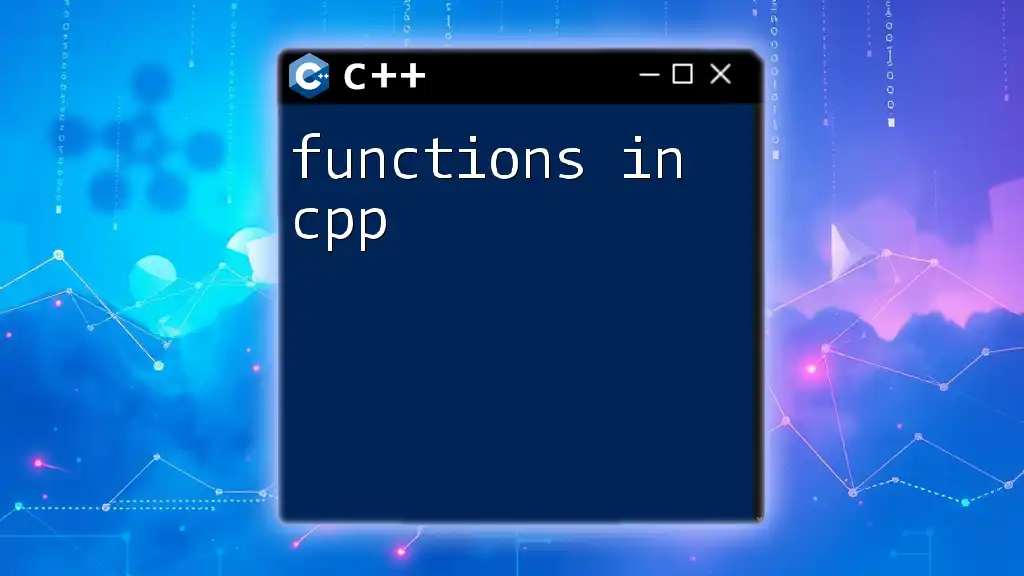
Common Errors and Troubleshooting in C++ Overriding
Common Mistakes in Function Overriding
Several potential pitfalls can arise while implementing function overriding:
- Failing to declare the base class method as virtual, leading to unexpected behaviors and not achieving polymorphism.
- Mismatching the function signature between the base and derived classes, resulting in the derived function being treated as a new function rather than an override.
- Misunderstanding access modifiers, such as trying to override a protected base method with a private derived method.
Troubleshooting Overriding Issues
To address issues related to function overriding:
- Utilize compiler warnings and errors to pinpoint where the overriding mechanism may have failed.
- Implement debugging techniques to inspect the behavior of derived class methods during runtime.
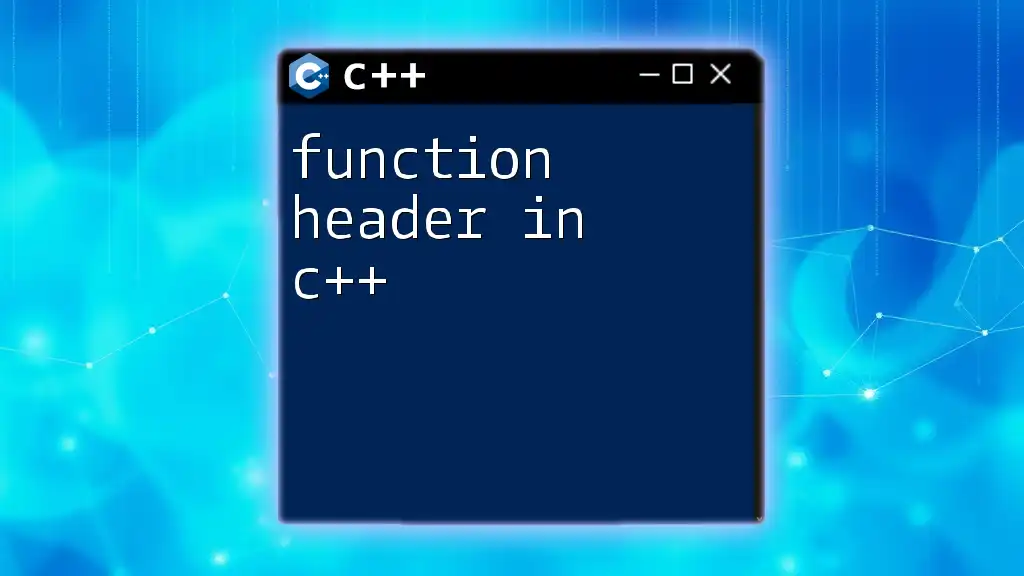
The Role of C++ Method Overriding in Real-World Applications
Practical Applications of Function Overriding
Function overriding is widely used in various domains:
- Game Development: In game engines, characters might have a base class called `Character` with methods like `move()` that can be overridden by specific character subclasses such as `Knight` or `Mage`.
- GUI Frameworks: Many GUI libraries use base classes for common controls where the rendered output may vary across different types of controls, necessitating overriding of methods like `draw()`.
Benefits of Utilizing Overriding Functions in C++
Implementing function overriding can lead to significant advantages:
- Code Reusability: Base class functionalities can be extended and reused in derived classes, fostering efficiency in coding practices.
- Enhanced Flexibility and Maintainability: Developers can easily adjust derived class behavior without modifying the overall structure, making it simpler to maintain and enhance applications.
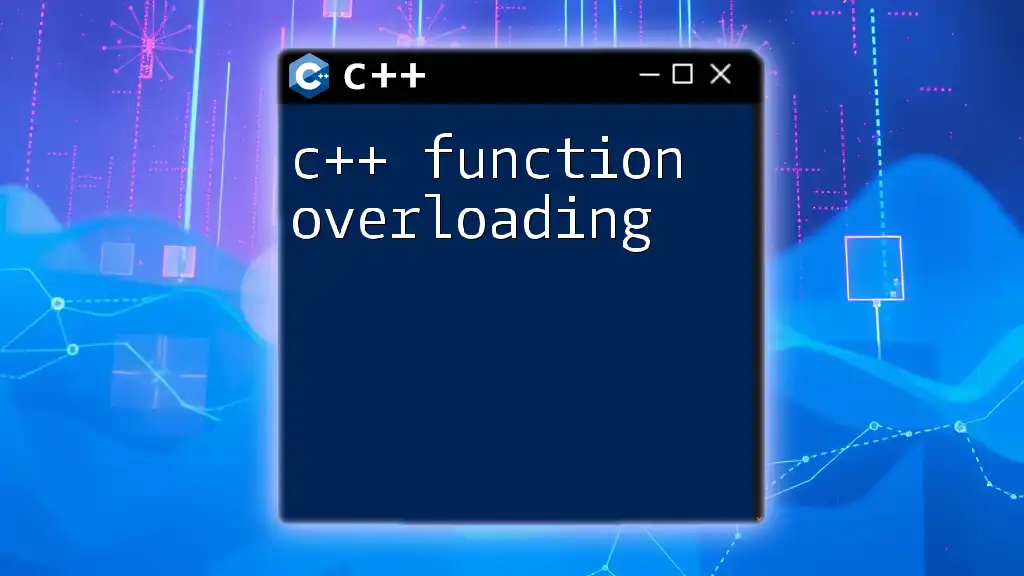
Conclusion
Function overriding in C++ is a powerful feature that enables derived classes to customize behaviors defined in base classes. Understanding its principles—including how to use virtual functions, adhere to best practices, and troubleshoot common issues—empowers developers to write more efficient and flexible code. Engaging actively with this concept in projects will elevate your programming skills and offer deeper insights into the world of Object-Oriented Programming.
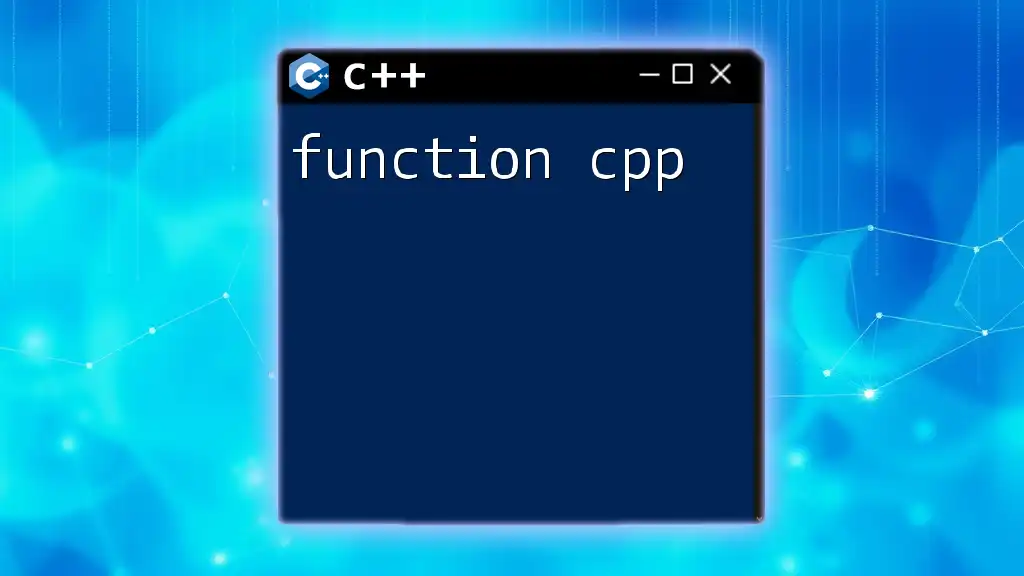
References and Further Reading
For further exploration of function overriding and its applications, consult recommended C++ programming books and online resources focusing on object-oriented design patterns, polymorphism, and inheritance practices.