Type conversion in C++ refers to the process of converting a variable from one data type to another, either implicitly or explicitly, to ensure compatibility during operations.
Here’s a code snippet demonstrating explicit type conversion:
#include <iostream>
using namespace std;
int main() {
double pi = 3.14;
int intPi = (int)pi; // Explicit type conversion from double to int
cout << "Integer value of pi: " << intPi << endl; // Outputs: Integer value of pi: 3
return 0;
}
Understanding Data Types in C++
In C++, understanding the fundamental data types is crucial before diving into type conversion. C++ supports both primitive and user-defined data types.
Primitive Data Types
C++ has several primitive data types that represent simple data. These include:
- Integral Types: Such as `int`, `char`, `short`, and `long`.
- Floating-Point Types: Such as `float` and `double`.
For example:
int age = 25; // integral type
float height = 5.9; // floating-point type
User-Defined Data Types
User-defined data types include structures, unions, and classes. These types allow the creation of complex data models. Understanding how they interplay with type conversion is critical, as converting between user-defined types can lead to different behaviors than with primitive types.
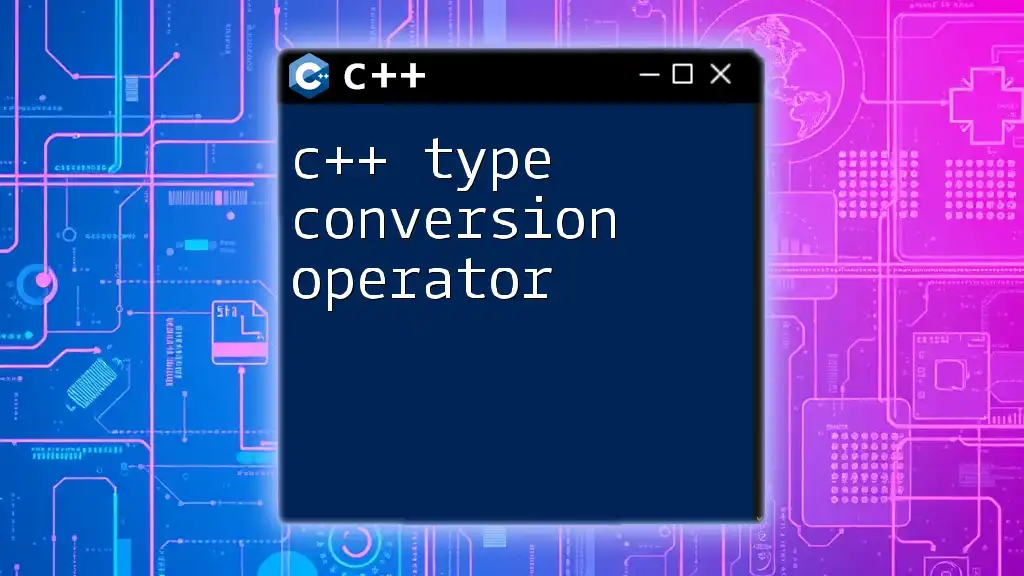
Types of Type Conversion
Type conversion in C++ falls into two main categories: Implicit Conversion and Explicit Conversion.
Implicit Type Conversion
Implicit type conversion, also known as automatic type conversion, occurs when the compiler automatically converts one data type to another without explicit guidance from the programmer. This usually happens in operations involving different data types.
For example:
int intVar = 10;
float floatVar = intVar; // Implicit conversion from int to float
In this scenario, `intVar` is converted to a `float` when assigned to `floatVar`. Such conversions are generally safe and do not lead to data loss.
Explicit Type Conversion (Casting)
Explicit type conversion, also known as casting, is when the programmer specifies the conversion. This method is often needed when there’s a risk of data loss or when dealing with pointers.
For instance:
double d = 9.7;
int i = (int)d; // C-style cast
Here, the double `d` is explicitly converted to an integer. Note that this truncates the decimal, highlighting the potential for data loss in explicit conversions.
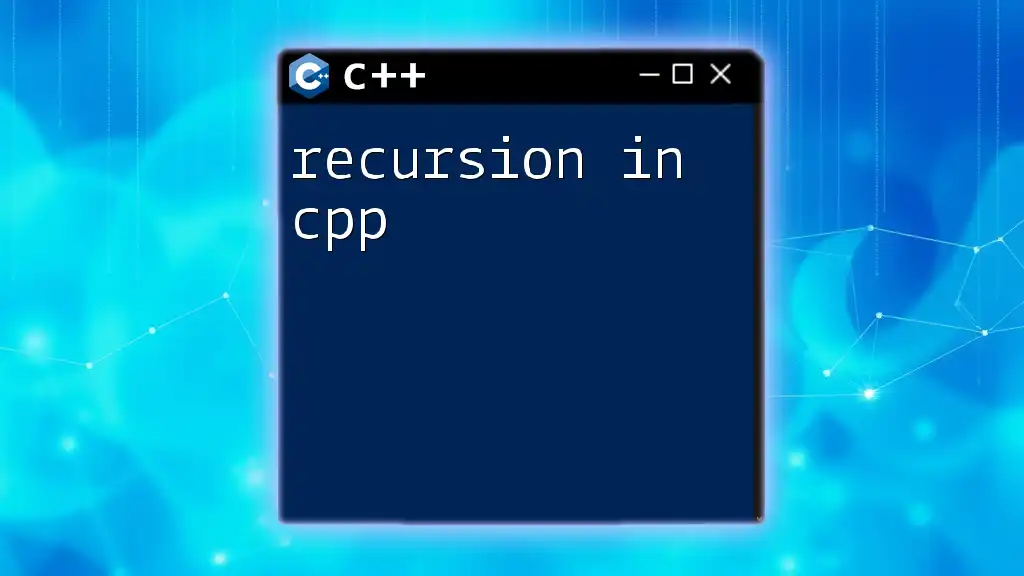
C++ Casting Operators
C++ provides four primary casting operators that offer more control over type conversion, which include `static_cast`, `dynamic_cast`, `const_cast`, and `reinterpret_cast`.
static_cast
`static_cast` is the most common casting operator, providing a way to safely convert between compatible types. It's useful for downcasting and upcasting in an inheritance chain.
Example:
class Base {};
class Derived : public Base {};
Derived d;
Base* b = static_cast<Base*>(&d); // Casting Derived to Base
In this example, the `Derived` type is safely converted to `Base` using `static_cast`.
dynamic_cast
`dynamic_cast` is specialized for use with polymorphic types (those that have at least one virtual function). It offers runtime checks to ensure that the cast is valid.
Example:
class Base {
public:
virtual ~Base() {} // Ensuring polymorphic behavior
};
class Derived : public Base {};
Base* b = new Derived();
Derived* d = dynamic_cast<Derived*>(b); // Safe downcast
If `b` does not point to a `Derived` object, `d` will be `nullptr`, ensuring safety in downcasting.
const_cast
`const_cast` is used to add or remove constness from variables. This is useful when dealing with APIs or functions that cannot be changed.
Example:
const int a = 10;
int* b = const_cast<int*>(&a); // Removing constness
This allows for modification, but it’s dangerous to change a variable that was originally defined as `const`.
reinterpret_cast
`reinterpret_cast` is the most dangerous cast, allowing for low-level reinterpreting of bit patterns. It does not perform any safety checks.
Example:
int* intPtr;
char* charPtr = reinterpret_cast<char*>(intPtr); // Reinterpreting pointer type
Use `reinterpret_cast` only when absolutely necessary, as it can lead to undefined behavior.
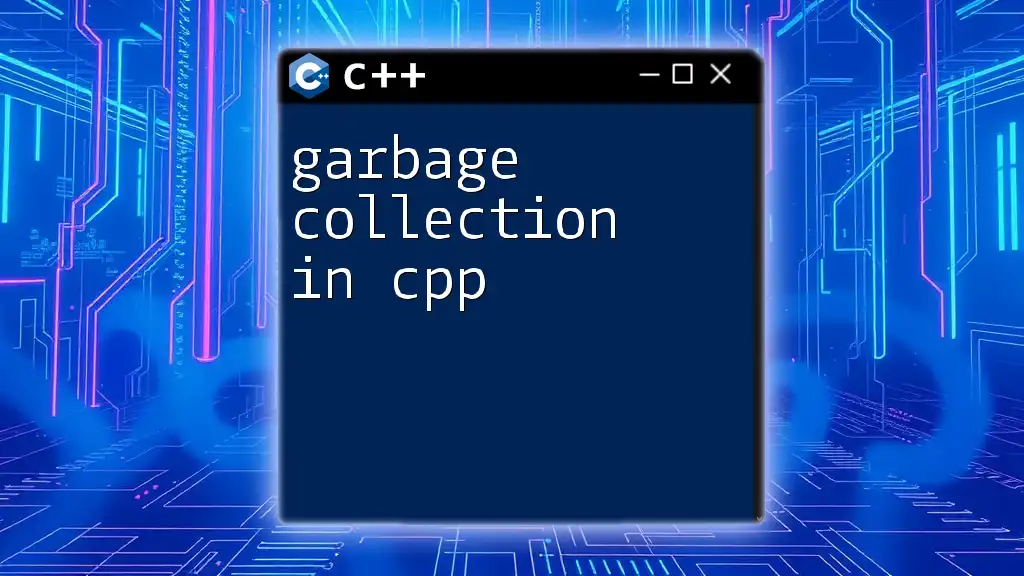
Best Practices for Type Conversion
When to Use Implicit vs. Explicit Conversions
Choose implicit conversions when you are confident that data loss will not occur. Use explicit conversions when there’s potential for loss of precision or when converting between incompatible types that the compiler cannot handle automatically.
Potential Pitfalls
Be mindful of the following common pitfalls in type conversion:
- Data Loss: When converting from a larger data type to a smaller type, data loss can occur.
- Example: Converting `double` to `int`.
- Invalid Memory Access: Using `reinterpret_cast` may lead to accessing invalid memory locations if used incorrectly.
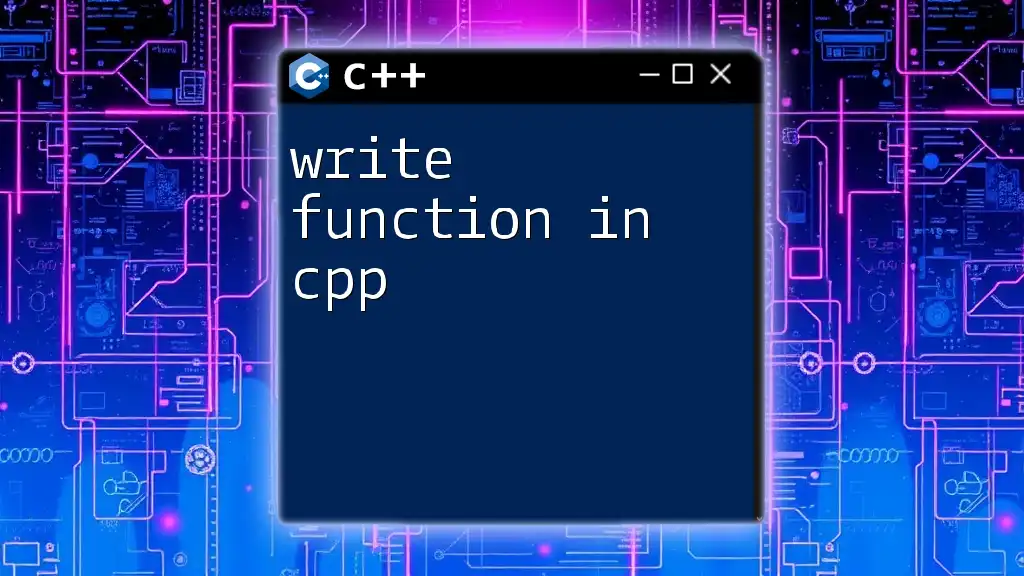
Conclusion
Understanding type conversion in C++ is essential for robust programming. Familiarity with implicit and explicit conversions, along with the casting operators, can significantly enhance your ability to manage data types effectively. Practice with these concepts through examples helps form a deeper understanding and prepares you for real-world programming challenges.
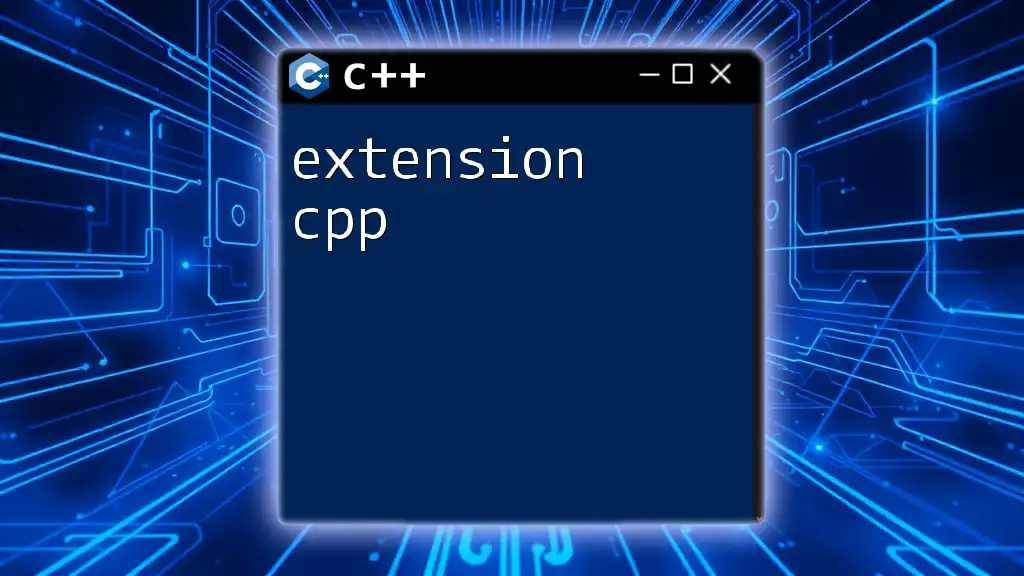
Additional Resources
To deepen your knowledge and experience with type conversion in C++, consider exploring books on C++ programming and online tutorials, as well as engaging with practical code examples on GitHub or personal coding blogs. These resources can provide valuable insights and hands-on experience in working with type conversions effectively.