Implicit conversions in C++ occur when the compiler automatically converts one data type to another without explicit instruction from the programmer, often enabled by defining conversion operators or constructors.
Here's a code snippet to illustrate this:
#include <iostream>
class MyClass {
public:
MyClass(int value) : value(value) {}
operator int() const { return value; } // Conversion operator
private:
int value;
};
int main() {
MyClass obj(42);
int num = obj; // Implicit conversion from MyClass to int
std::cout << "The number is: " << num << std::endl;
return 0;
}
The Concept of Implicit Conversion in C++
Understanding implicit conversions in C++ is essential for effective programming. Implicit conversion occurs when the C++ compiler automatically converts a value from one type to another without requiring explicit instructions from the programmer. This seamless system reduces the necessity for manual conversions, allowing for more intuitive and fluid code.
Definition of Implicit Conversion
In C++, implicit conversion is a type of conversion that happens automatically. When an expression requires a specific type, the compiler checks if the operands can be converted to that type without the programmer explicitly requesting it. This characteristic of C++ simplifies code and enhances readability, yet it demands that programmers recognize when these automatic transformations occur to avoid unexpected behaviors or errors.
How Implicit Conversion Works
Implicit conversions follow established rules defined by the C++ language, involving:
-
Standard Type Promotions: These are conversions that C++ applies to smaller types to ensure compatibility with larger ones. For example, an `int` can be promoted to `double` automatically.
-
User-Defined Conversions: By defining conversion operators within classes, programmers can specify how their custom types should convert implicitly to other data types.
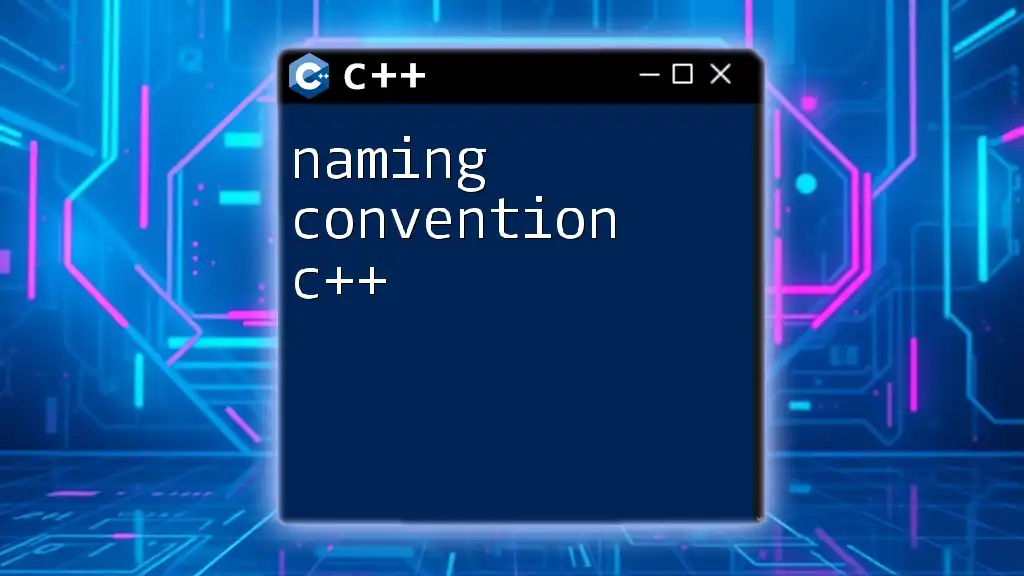
C++ Implicit Conversions: Built-in Types
Numeric Promotions
One of the most common forms of implicit conversions in C++ is numeric promotions, where smaller integral types are promoted to larger types. For instance:
int i = 10;
double d = i; // Implicit conversion: int to double
Here, the integer `i` is automatically converted to a double `d`. This process happens without any loss of information, as the integer is simply represented in a format that accommodates decimals.
Boolean Implicit Conversion
Boolean implicit conversion is another fascinating aspect. In C++, any non-zero integer value converts to `true`, while `0` converts to `false`. For example:
int x = 5;
bool b = x; // Implicit conversion: int to bool
Since `x` is non-zero, `b` now holds the value `true`. This behavior can be powerful but may also lead to misunderstanding if not correctly interpreted.
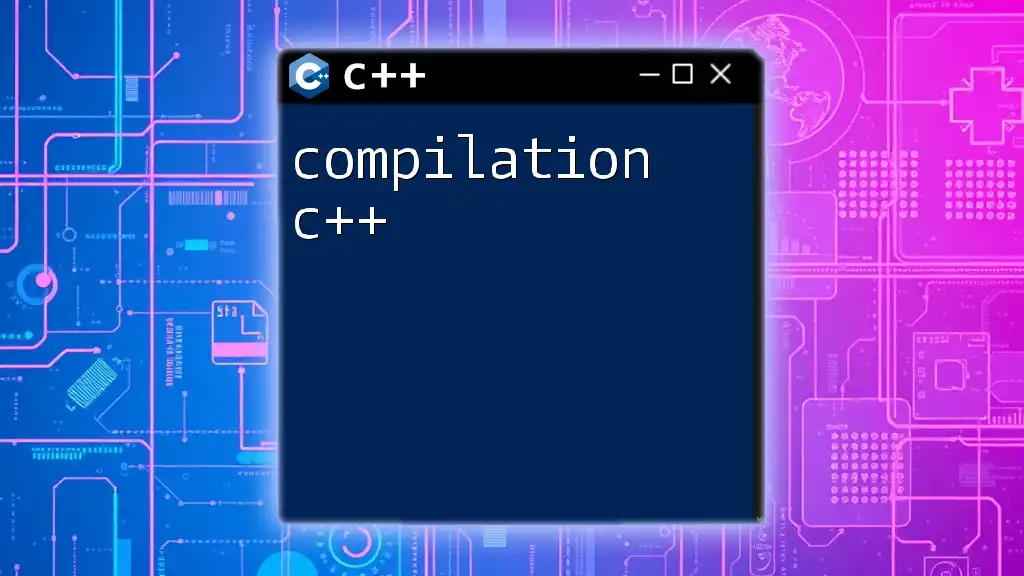
Implicit Conversions with User-Defined Types
Class Type Conversions
In addition to built-in types, C++ allows for user-defined implicit conversions through conversion operators. This feature allows class instances to convert to basic types. For example:
class Celsius {
public:
Celsius(float temp) : temperature(temp) {}
operator float() const { return temperature; } // Implicit conversion to float
private:
float temperature;
};
Celsius c(37.0);
float f = c; // Implicit conversion from Celsius to float
In this code, the `Celsius` class defines an operator that allows an instance of `Celsius` to be converted to a `float`. The line `float f = c;` demonstrates how you can assign a `Celsius` object to a `float` variable without needing to call a conversion function explicitly.
Implicit Conversion and Constructors
Constructors can also facilitate implicit conversions via conversion constructors. They allow objects to be created from single values, which can lead to more convenient coding patterns. Consider this example:
class Square {
public:
Square(double side) : area(side * side) {}
double area;
};
Square s = 5; // Implicit conversion using the constructor
Here, when `5` is assigned to `s`, the constructor `Square(double side)` takes over, creating a `Square` object from the integer `5`.
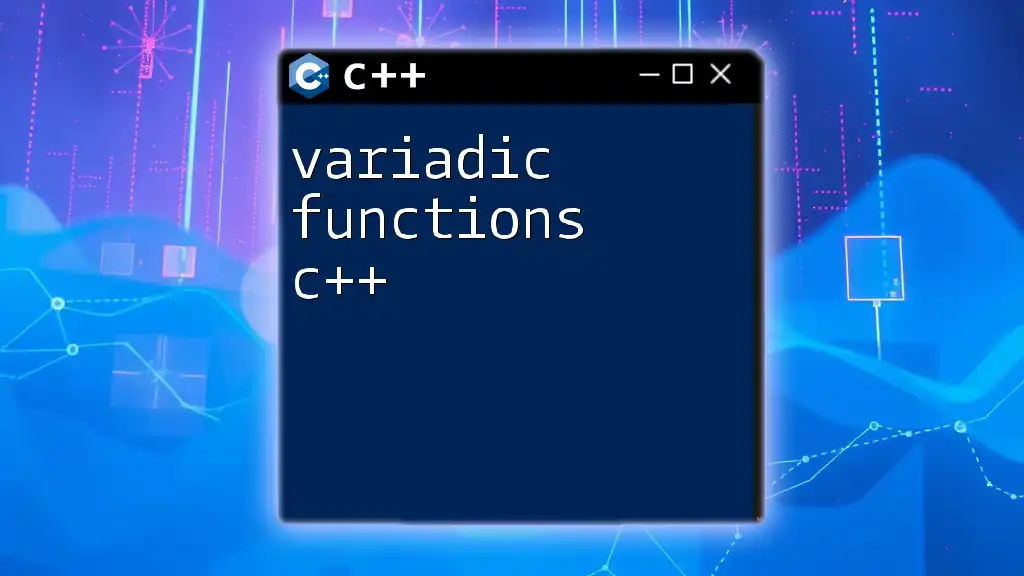
Potential Pitfalls of Implicit Conversions
Ambiguities and Confusions
Despite the advantages of implicit conversions, they can cause ambiguity and confusion. For instance:
int a = 5.5; // Might introduce subtle behaviors
In this case, assigning a `double` value to an `int` triggers an implicit conversion that truncates the decimal part, possibly leading to unintended consequences in calculations.
Loss of Information
Loss of information can occur during implicit conversions, particularly when converting types with different ranges. For example:
double d = 10.6;
int n = d; // Loss of fractional part
In this scenario, the value of `d` is truncated, resulting in a loss of the decimal. Such issues stress the importance of reviewing conversions carefully to ensure the desired data integrity.
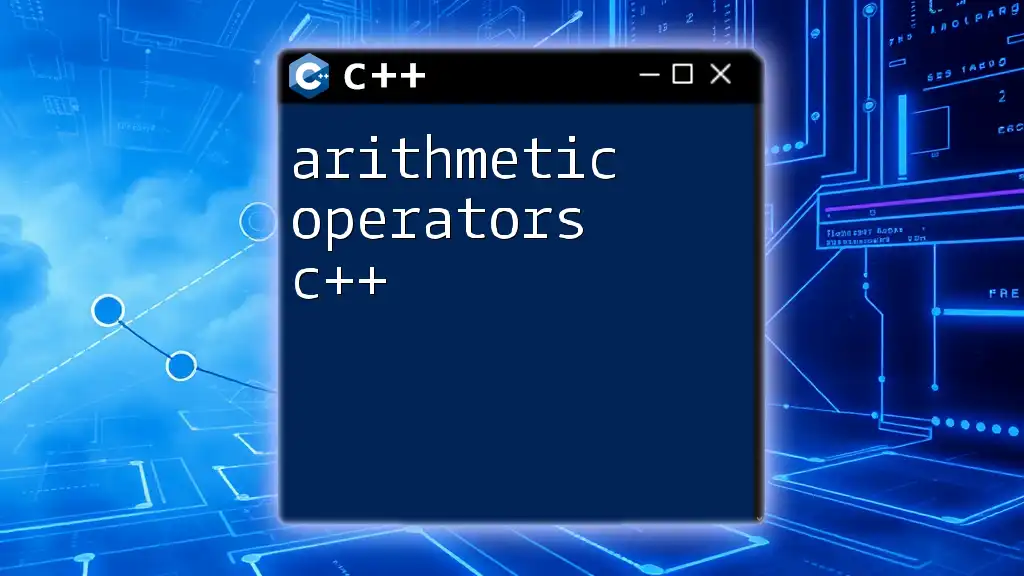
Best Practices for Using Implicit Conversions
When to Allow Implicit Conversions
Implicit conversions can enhance code clarity and maintainability when used judiciously. Allowing implicit conversions makes the code cleaner and more intuitive, especially when you want objects or data types to work together seamlessly.
When to Avoid Implicit Conversions
However, it’s essential to avoid implicit conversions in situations where they can lead to confusion, unexpected results, or significant loss of information. For instance, when dealing with critical computations that depend on precision, consider using explicit conversions to ensure clarity and correctness.
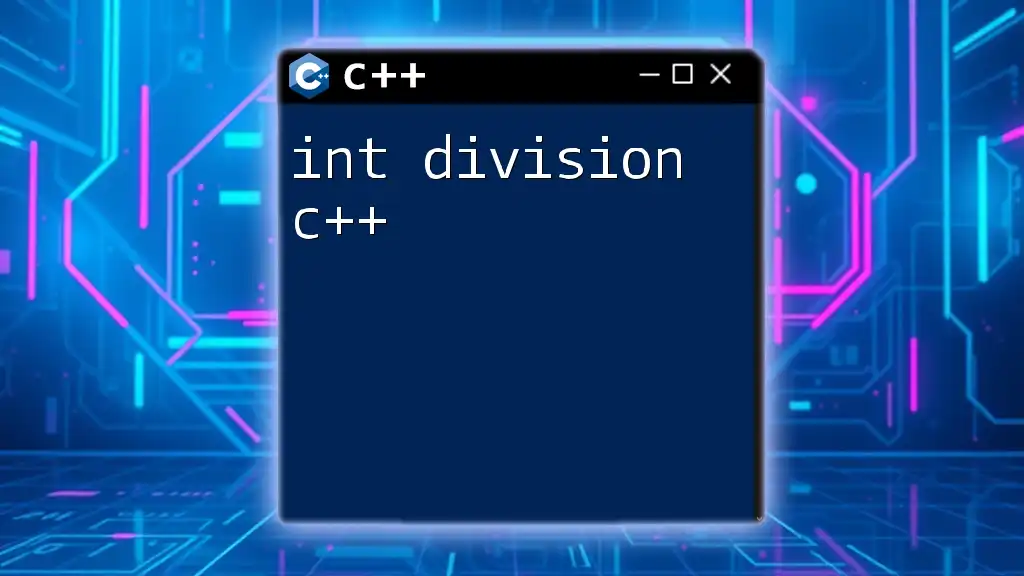
Conclusion
Understanding implicit conversions in C++ is critical for any programmer aiming to write efficient and error-free code. This guide highlights the mechanics behind these conversions, their benefits and pitfalls, and best practices for using them effectively. Arm yourself with this knowledge, and you can navigate C++ programming with confidence, ensuring that your code is both robust and intuitive.