In C++, the `std::map` container is used to store key-value pairs, and you can check if a specific key exists using the `find()` method or the `count()` method.
Here's a code snippet demonstrating how to check if a map contains a specific key:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
int keyToFind = 2;
if (myMap.find(keyToFind) != myMap.end()) {
std::cout << "Key " << keyToFind << " exists in the map." << std::endl;
} else {
std::cout << "Key " << keyToFind << " does not exist in the map." << std::endl;
}
return 0;
}
Understanding C++ Maps
What is a Map in C++?
A map in C++ is an associative container that stores elements in key-value pairs. Each key in a map is unique, and it is used to access the corresponding value. This structure allows for efficient lookups, insertions, and deletions.
The syntax to declare a map is as follows:
#include <map>
std::map<KeyType, ValueType> myMap;
This versatile structure provides the ability to perform searches at logarithmic complexity, making it considerably more efficient than linear searches, such as those found in arrays or lists.
Importance of Maps in C++
Maps are integral to many programming solutions. They enable developers to:
- Store Relationships: Key-value pairs elegantly represent relationships between data.
- Efficient Retrieval: C++ maps offer efficient retrieval times, which are especially beneficial in large datasets.
- Dynamic Growth: Maps can grow and shrink dynamically, depending on the operations performed.
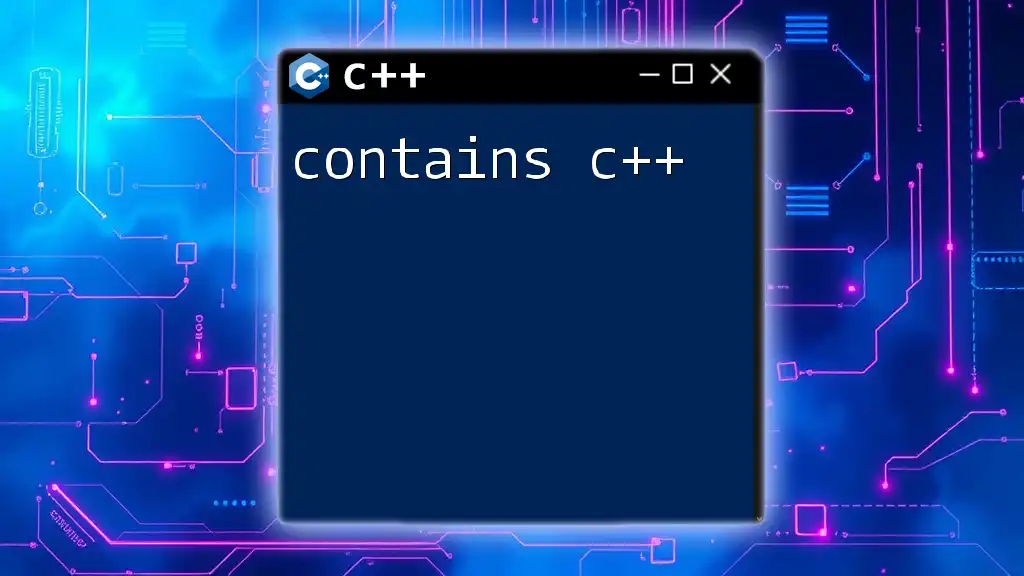
How to Check if a Map Contains a Key
Using the `find()` Method
One of the most common and efficient ways to check if a map contains a specific key is to use the `find()` method. This method returns an iterator to the element if it is found, or `end()` if it is not.
Here's how it works:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Key found: " << it->second << std::endl;
} else {
std::cout << "Key not found!" << std::endl;
}
return 0;
}
In this example, we attempt to find the key `2`. The `find()` function checks if the key is present and returns an appropriate iterator. If the key is found, it accesses the corresponding value through the iterator.
Using the `count()` Method
Another method to check for the existence of a key is to use the `count()` method. This method returns the number of elements with the specified key, which will either be `0` (if the key does not exist) or `1` (if it does).
Consider the following example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
if (myMap.count(3) > 0) {
std::cout << "Key found: " << myMap[3] << std::endl;
} else {
std::cout << "Key not found!" << std::endl;
}
return 0;
}
By checking `myMap.count(3)`, we determine the presence of the key `3`. This method is straightforward and efficient, making it a reliable option for checking the existence of keys.
Using the `operator[]` for Key Lookup
While it may be tempting to use `operator[]` for checking key existence, it’s important to understand its behavior. The `operator[]` will create an entry in the map if the key does not exist, which may lead to unintended side effects.
Here is a demonstration:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
std::string value = myMap[1]; // Will return existing value
std::cout << "Value: " << value << std::endl;
// Caution: This will insert a new key-value pair if key '4' doesn't exist
value = myMap[4]; // This will create myMap[4] with a default value
std::cout << "Value for non-existent key: " << value << std::endl;
return 0;
}
Using `operator[]` to search can lead to unintentionally modifying the map. Therefore, it should be used with caution and is generally not recommended for checking if a key exists.
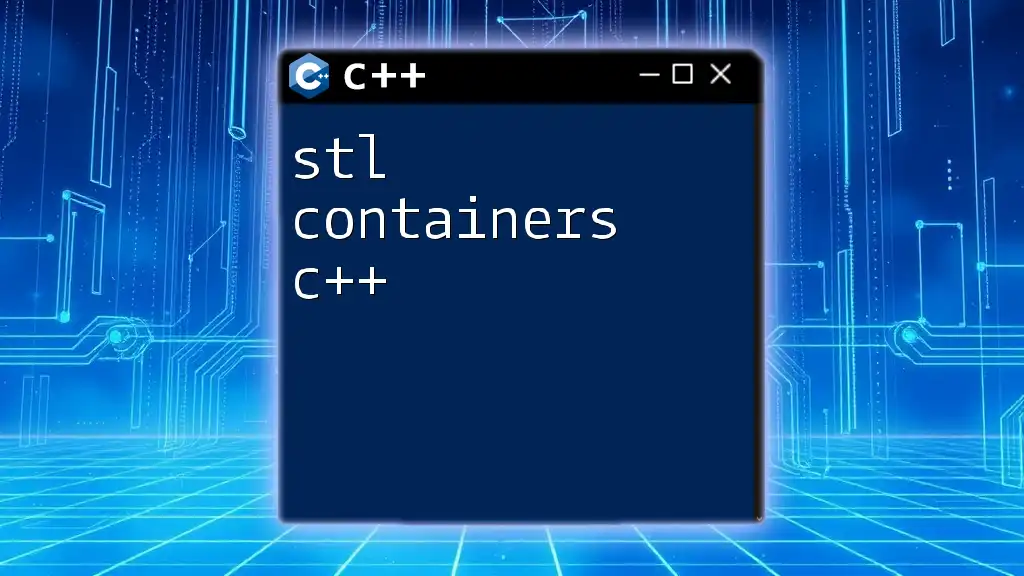
Performance Considerations
Time Complexity of Key Checks
Different methods of checking for key existence in a map come with varying time complexities:
- `find()`: O(log n) due to the underlying balanced binary tree implementation.
- `count()`: Also O(log n) for the same reason.
- `operator[]`: O(log n) for lookup, but O(log n) + possible O(1) for insertion if the key does not exist. This is why using `operator[]` just for checking is discouraged.
Space Complexity
Maps in C++ can involve various memory allocations depending on key/value pair insertions. Understanding memory usage is crucial, especially in resource-constrained environments. Maps usually have overhead due to their dynamic nature, which can lead to higher memory consumption compared to static arrays.
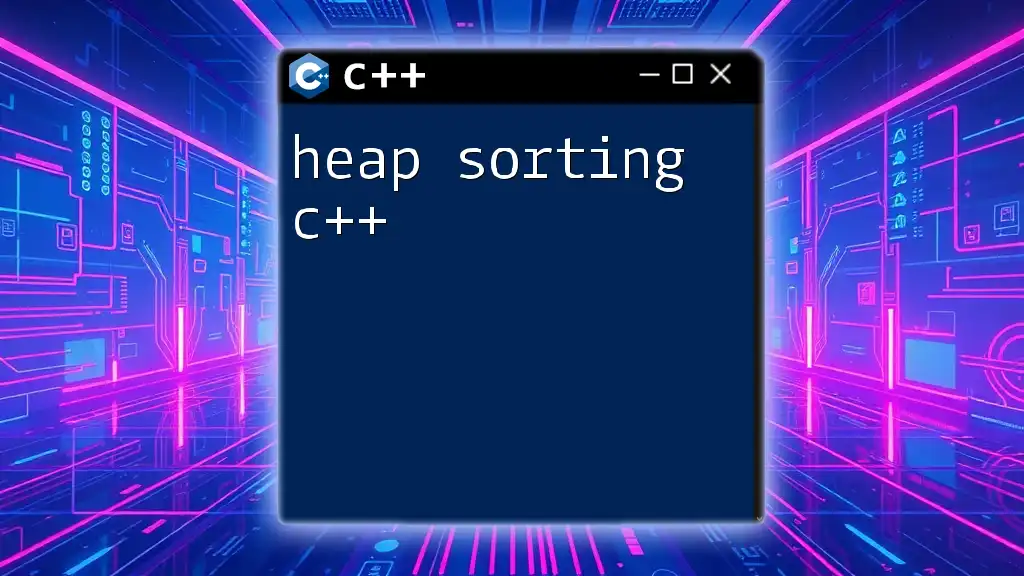
Best Practices for Using Maps
Choosing the Right Key Type
When selecting a key type, consider:
- Uniqueness: Choose a type that naturally fits the use case of unique keys.
- Performance: Prefer built-in types (like `int`, `string`) for better performance in hashing and comparison.
- Complex Types: If using complex types as keys, ensure to provide proper comparison operators to avoid undefined behavior.
Avoiding Common Pitfalls
Common mistakes developers might make with maps include:
- Using `operator[]` for existence checks, leading to accidental insertions.
- Ignoring the efficiency of accessing the elements by choosing inappropriate key types.
- Not considering the performance trade-offs when choosing the appropriate method for checking key existence.
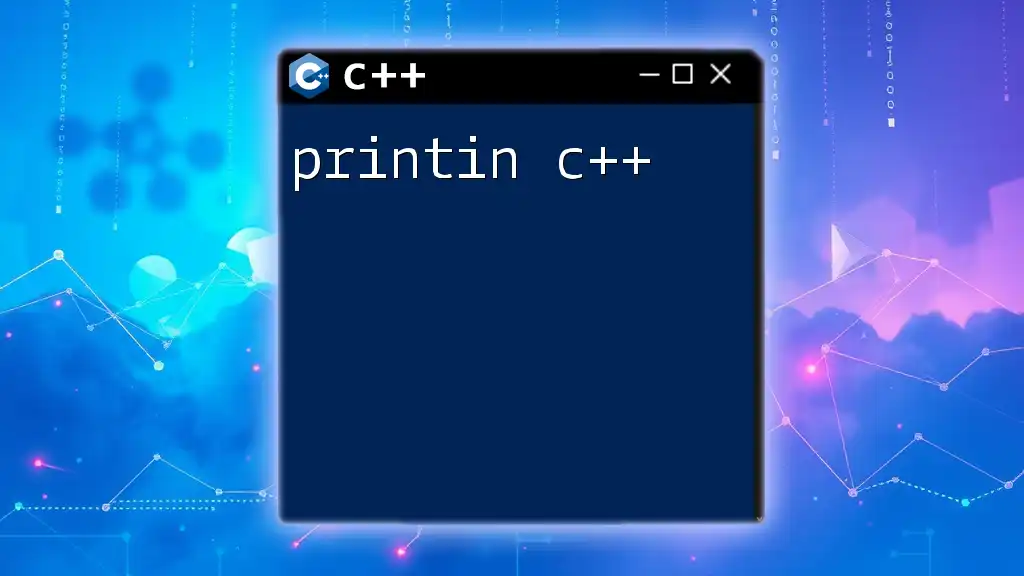
Conclusion
In summary, understanding how to check if a map contains a key is critical when working with C++. By knowing how and when to use the `find()`, `count()`, and `operator[]` methods, you can write more efficient and clean code. Remember to think about performance and best practices when utilizing maps in your applications.
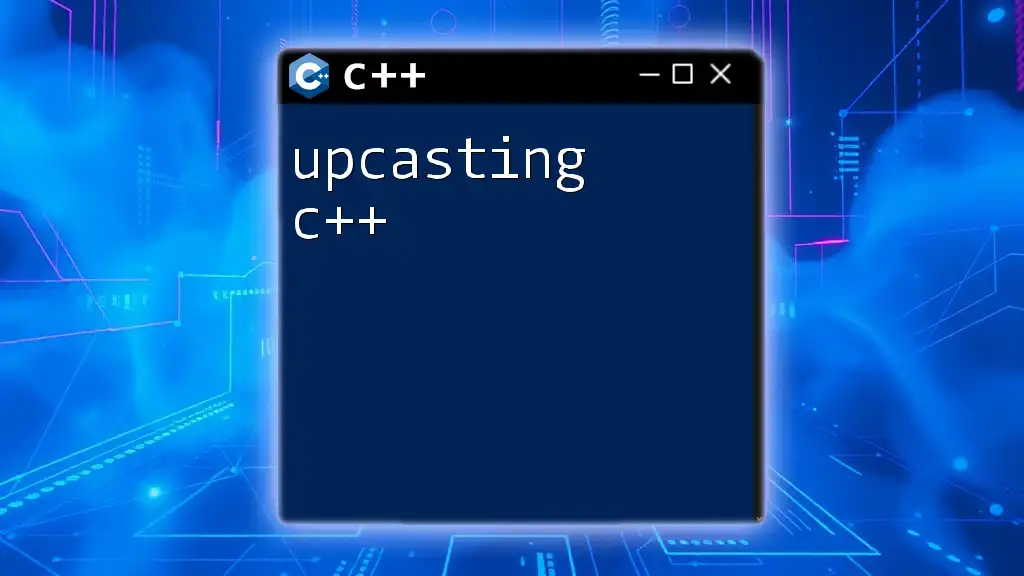
Additional Resources
For further exploration, consider reviewing the official C++ documentation on maps. Engaging with coding platforms like LeetCode or HackerRank can also provide practical experience with maps and their functionalities.
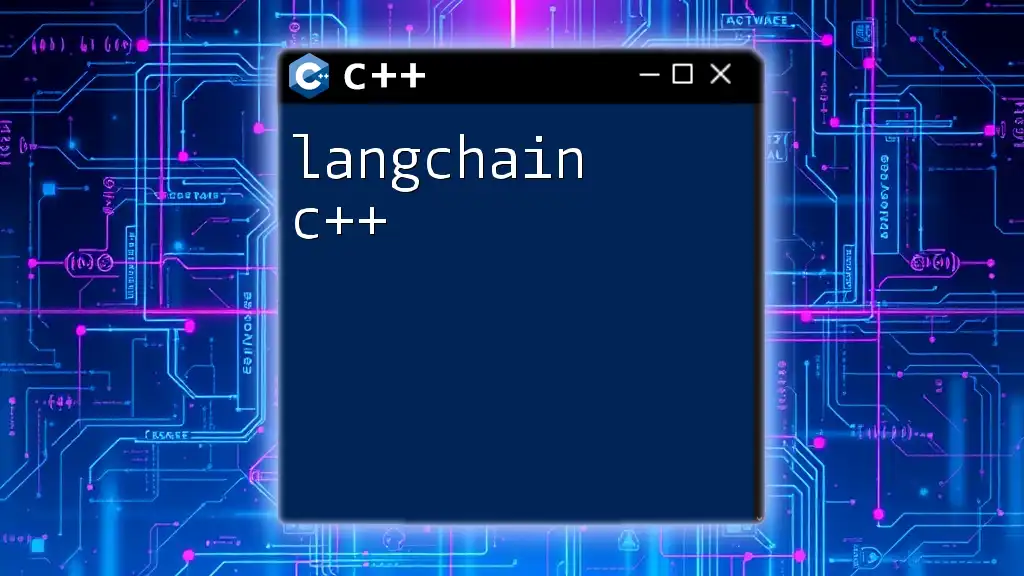
Frequently Asked Questions
Some common questions related to checking if a map contains a key in C++ include:
- What happens if I use `operator[]` on a non-existent key?
- Is there a performance difference between `find()` and `count()`?
- When should I prefer `unordered_map` over `map`?
This comprehensive overview of "map contains C++" will equip you with the necessary knowledge to effectively utilize maps in your programming tasks!