A "map of maps" in C++ refers to a data structure where the value of each key in a map is another map, allowing for a hierarchical organization of key-value pairs.
#include <iostream>
#include <map>
#include <string>
int main() {
// Create a map of maps
std::map<std::string, std::map<std::string, int>> mapOfMaps;
// Adding elements
mapOfMaps["GroupA"]["Alice"] = 30;
mapOfMaps["GroupA"]["Bob"] = 25;
mapOfMaps["GroupB"]["Charlie"] = 28;
// Accessing an element
std::cout << "Alice's age in GroupA: " << mapOfMaps["GroupA"]["Alice"] << std::endl;
return 0;
}
Introduction to Maps in C++
In C++, a map is an associative container that stores elements in key-value pairs. Each key is unique and is mapped to a specific value, allowing for efficient retrieval and insertion of data. Maps are part of the Standard Template Library (STL) and provide a robust way to manage related data.
A map of maps is essentially a nested map structure where the value of an outer map is another map. This allows for the representation of complex relationships, enabling developers to create multi-dimensional data structures.
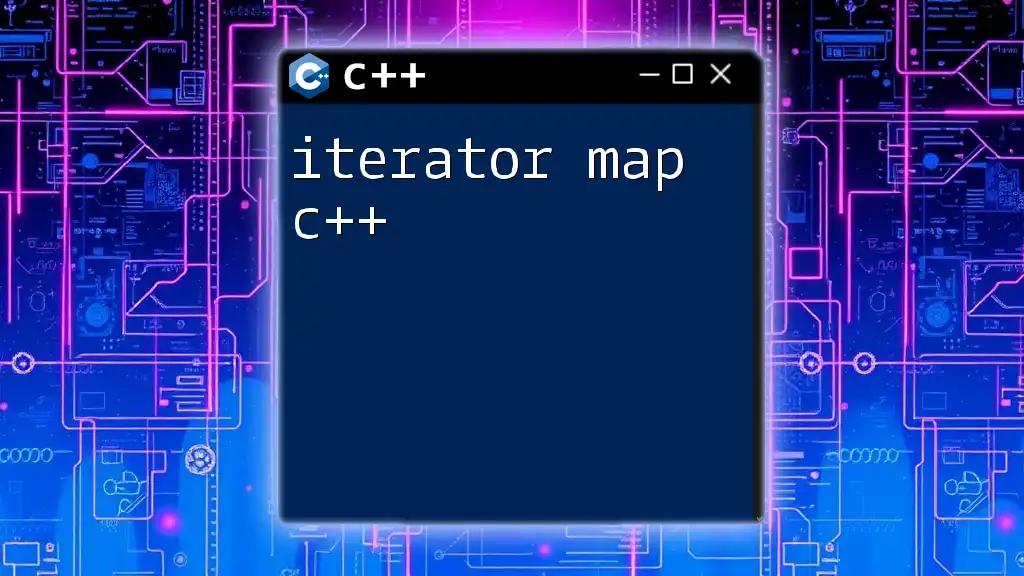
Understanding std::map
Overview of std::map in C++
The `std::map` class is designed for storing data in a sorted manner, automatically keeping keys in order. The general structure of a map consists of:
- Key: A unique identifier for the value.
- Value: The actual data associated with the key.
Using `std::map`, you can easily create associative arrays that allow for efficient data lookup, insertion, and deletion.
Basic operations on std::map
These operations are crucial when working with maps:
-
Insertion: You can add a new key-value pair using the `insert` method or the subscript operator (`[]`).
-
Accessing elements: Use the subscript operator or the `at` method to retrieve values associated with certain keys.
-
Deletion: You can remove elements using the `erase` method provided by the map.
Here’s a basic code snippet demonstrating these operations:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
// Insertion
myMap[1] = "One";
myMap.insert({2, "Two"});
// Accessing elements
std::cout << "Key 1: " << myMap[1] << std::endl;
// Deletion
myMap.erase(1);
return 0;
}
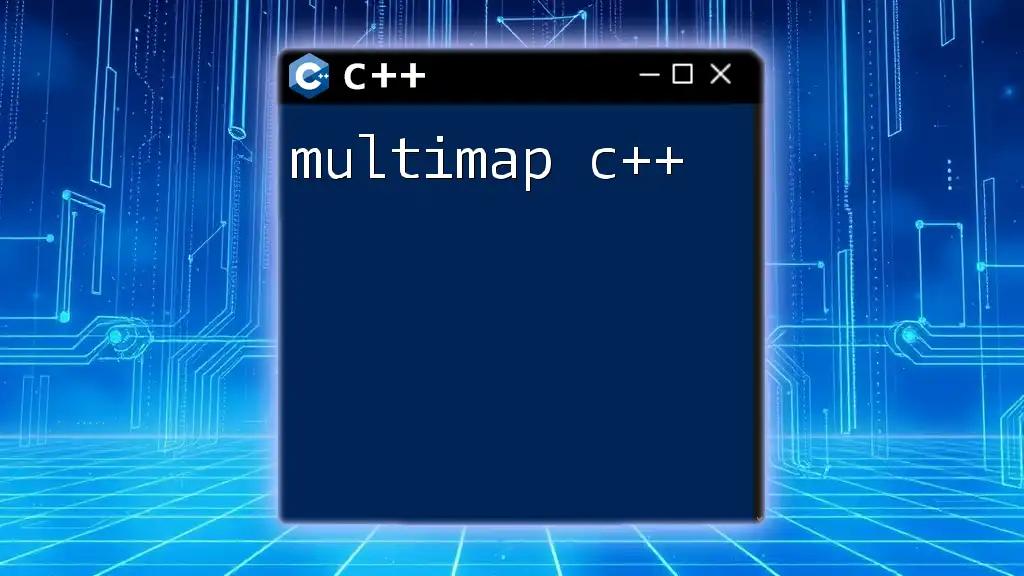
The Concept of a Map of Maps
Defining a map of maps
A map of maps in C++ is a data structure in which the value of each key in the outer map is another `std::map`. For instance, the syntax looks like this:
std::map<KeyType1, std::map<KeyType2, ValueType>>
In this setup, `KeyType1` serves as the key for the outer map, while `KeyType2` and `ValueType` are for the inner map.
Why use a map of maps?
A map of maps can be incredibly useful in various scenarios, particularly when representing hierarchical data or nested relationships. It allows you to maintain a complex data structure, such as:
- Student records: Where the outer map could represent different classes, and the inner maps could represent students and their grades.
- Configuration settings: Where the outer map represents categories of settings, and the inner maps hold specific settings and their values.
Compared to using a single map, a map of maps provides a more organized way to manage multi-layered data.
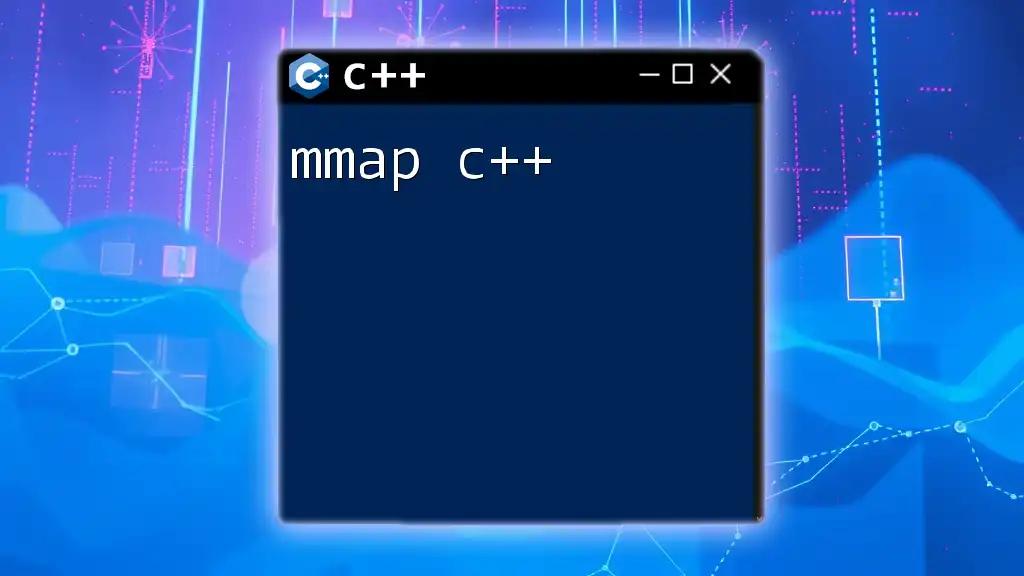
Creating a Map of Maps
Step-by-step guide to creating a map of maps
To create a map of maps, start by defining it according to the syntax discussed earlier. Here’s a demonstration:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, std::map<std::string, int>> mapOfMaps;
// Initializing a nested map
mapOfMaps["Math"]["Alice"] = 90;
mapOfMaps["Math"]["Bob"] = 85;
mapOfMaps["Science"]["Alice"] = 92;
return 0;
}
Populating the map of maps
Adding data to both the outer and inner maps is straightforward. Each key in the outer map will act as a unique identifier for the inner map it contains. The following example shows how to populate the structure:
mapOfMaps["English"]["Carol"] = 88;
mapOfMaps["English"]["Dave"] = 75;
In this case, we are updating our structure to include classes and students' scores.

Accessing and Manipulating Data in a Map of Maps
Accessing elements in a map of maps
To access elements in a map of maps, you must first reference the outer map, and then the inner map. Here’s how:
int aliceMathScore = mapOfMaps["Math"]["Alice"];
std::cout << "Alice's Math score: " << aliceMathScore << std::endl;
This code will retrieve Alice's score for Math.
Modifying values in the inner maps
You can modify existing entries easily. For example, if you want to update Carol's score in English:
mapOfMaps["English"]["Carol"] = 90; // Update Carol's score to 90
In addition to updates, you can remove entries within inner maps:
mapOfMaps["Science"].erase("Alice"); // Remove Alice from Science class
Iterating through a map of maps
To iterate through both outer and inner maps, nested loops are your best option. Here’s an example:
for (const auto& outer : mapOfMaps) {
std::cout << "Subject: " << outer.first << std::endl;
for (const auto& inner : outer.second) {
std::cout << " Student: " << inner.first << ", Score: " << inner.second << std::endl;
}
}
This effectively lists all subjects along with each student and their corresponding score.

Use Cases for Map of Maps
Exploring practical applications
Let’s consider two scenarios where a map of maps excels:
-
Example 1: Representing a database-like structure
For instance, if we wanted a database to track students and their grades by different subjects, a map of maps would be an excellent choice. Each subject could be a key, and each student with their score could be the value of an inner map.
-
Example 2: Storing configurations/settings
If you were designing an application with multiple modules, each needing its own configuration settings, a map of maps can neatly categorize all settings under respective modules. Each module can be a key, and each specific configuration a value in the inner map.
Advantages of using a map of maps for complex data structures
This data structure simplifies the handling of complex relationships, improves code readability, and allows for scalable data management. With a clear structure in place, developers can access and manipulate detailed information without excessive complexity.
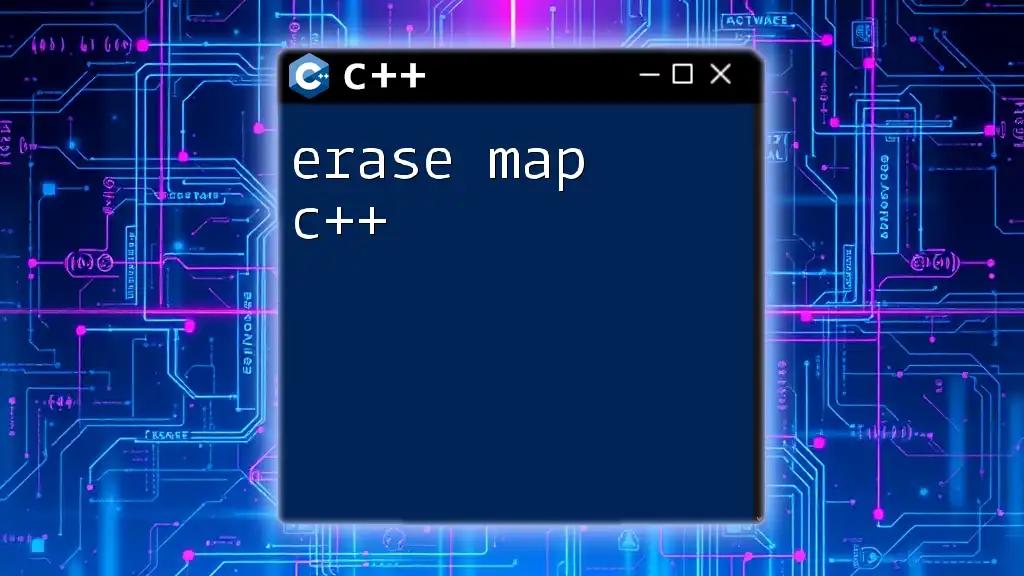
Common Pitfalls with Map of Maps
Performance considerations
When dealing with maps, especially nested maps, it’s important to understand the performance implications. While maps are efficient in accessing elements by key, excessive nesting can lead to increased complexity and possible latency in access times.
Avoiding misuse
Common mistakes include accessing keys that do not exist, leading to unexpected behaviors such as uninitialized values. Always ensure that a key exists before accessing it:
if (mapOfMaps.find("Science") != mapOfMaps.end()) {
// Safe to access science data
}
Being mindful of best practices will help maintain code clarity, making your work easier to manage and debug.
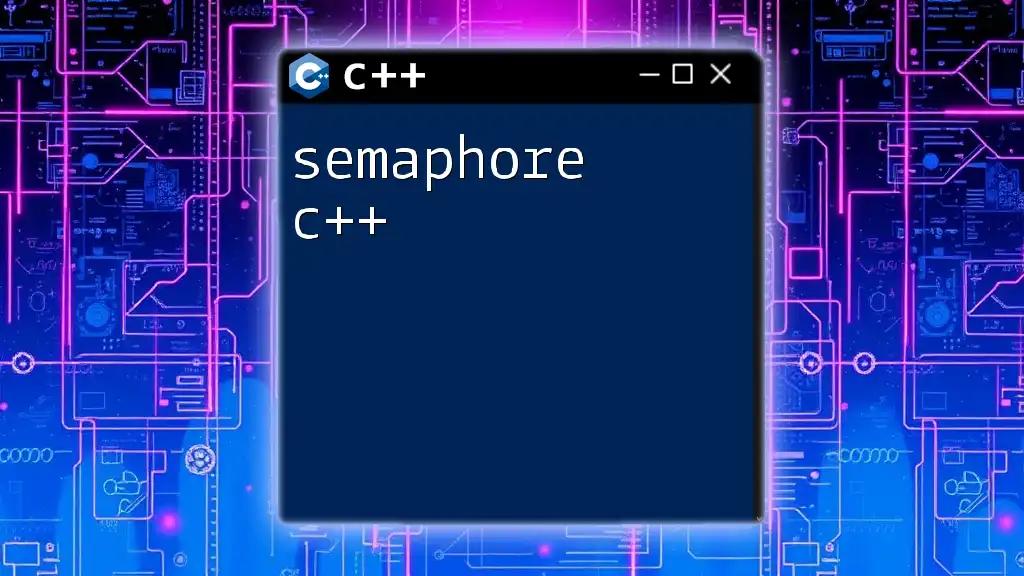
Conclusion
In summary, a map of maps in C++ provides an excellent way to manage complex, hierarchical data structures. By structuring data in this way, you can create organized representations of relationships, making it easier to develop robust applications. With practice and careful management, the potential for utilizing maps of maps is substantial.
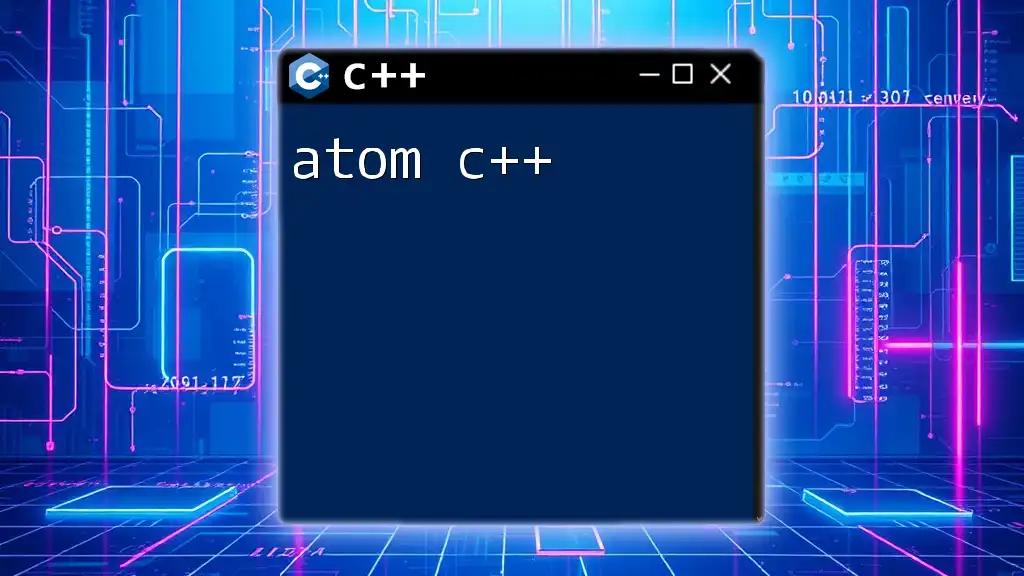
Additional Resources
For further learning, consider referring to the official C++ documentation, exploring recommended books, and engaging in community forums that focus on C++ programming. These resources can provide additional insights and foster better understanding of maps and their applications.