In C++, the `clear()` method is used to remove all elements from a map, effectively resetting it to an empty state.
Here's an example of how to use it:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "Apple";
myMap[2] = "Banana";
std::cout << "Map size before clear: " << myMap.size() << std::endl;
myMap.clear(); // Clears all elements from the map
std::cout << "Map size after clear: " << myMap.size() << std::endl;
return 0;
}
Understanding Maps in C++
What is a Map?
A map in C++ is an associative container that stores elements in key-value pairs. It is defined in the C++ Standard Template Library (STL) and is implemented as a binary search tree. Some essential characteristics of maps include:
- Unique keys: Each key in a map must be unique, which ensures that each value is associated with a distinct key.
- Sorted order: Maps maintain their keys in a sorted order, allowing for efficient searching, insertion, and deletion operations.
- Dynamic sizing: Maps can dynamically grow and shrink in size as elements are added or removed, providing flexibility in data management.
Comparing maps to other STL containers like vectors and lists, maps are particularly efficient for operations requiring quick retrieval based on keys, while vectors and lists are more suitable for ordered data and index-based access.
When to Use Maps
Maps are particularly beneficial in scenarios where you need to associate values with unique identifiers. Examples of such use cases include:
- Counting occurrences of items (e.g., counting the frequency of words in a text).
- Creating index-based data structures (e.g., implementing a phone book).
- Associating user data with identifiers (e.g., mapping user IDs to user profiles).
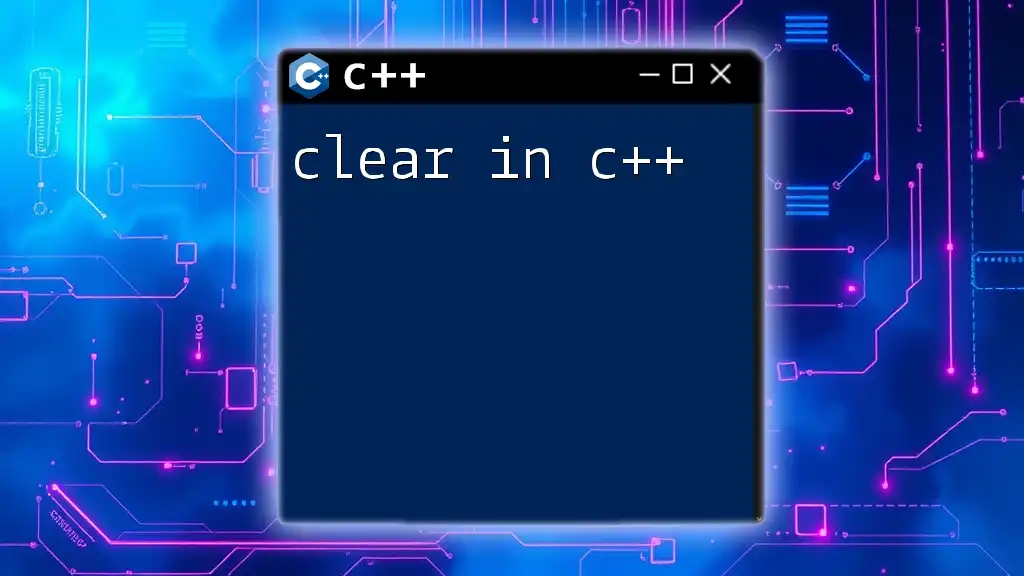
The `clear()` Function in C++
What Does `clear()` Do?
The `clear()` function is a member function of the map class that removes all elements from the map. When `clear()` is called, the map becomes empty, but the map itself is not destroyed. This function is crucial for effectively managing the contents of maps without needing to deallocate the map structure.
Syntax of `clear()`
The syntax for using the `clear()` function is straightforward. Here's how it looks:
std::map<KeyType, ValueType> myMap;
myMap.clear();
In this syntax, replace `KeyType` and `ValueType` with the appropriate data types for your use case.
Return Value of `clear()`
The `clear()` function does not return any value; its purpose is solely to empty the map. This means that developers do not receive any feedback regarding the operation's success or failure, which is typical for operations that modify container states in C++.
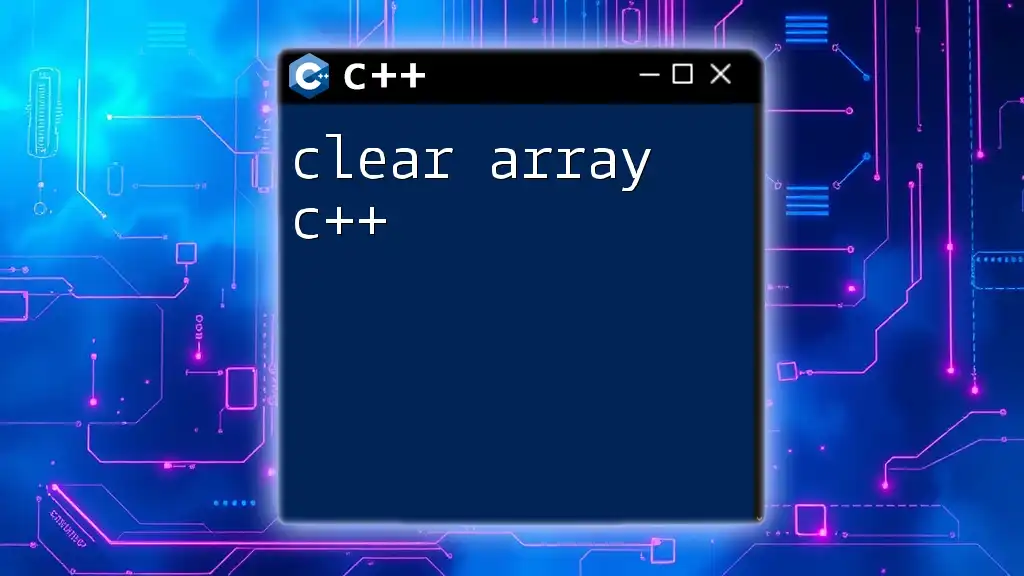
Practical Use Cases for `clear()`
Clearing Maps of Different Data Types
Consider a common situation where you have a map with integer keys and string values. Here's an example of initializing a map, adding data to it, and then clearing it:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "One";
myMap[2] = "Two";
std::cout << "Map size before clear: " << myMap.size() << std::endl;
myMap.clear(); // Now myMap is empty
std::cout << "Map size after clear: " << myMap.size() << std::endl;
return 0;
}
In this example, the output will show that the size of `myMap` is 2 before the `clear()` call and 0 afterward, demonstrating the effectiveness of the `clear()` function.
Clearing Maps in Real-world Applications
In practical applications, the `clear()` function is crucial. For instance, in a game inventory system, you might have a map that keeps track of items. When a player restarts the game or clears their inventory, you can easily remove all the items by calling `clear()`.
std::map<int, Item> inventory;
inventory[1] = Item("Sword");
inventory[2] = Item("Shield");
// Clear inventory when player restarts
inventory.clear();
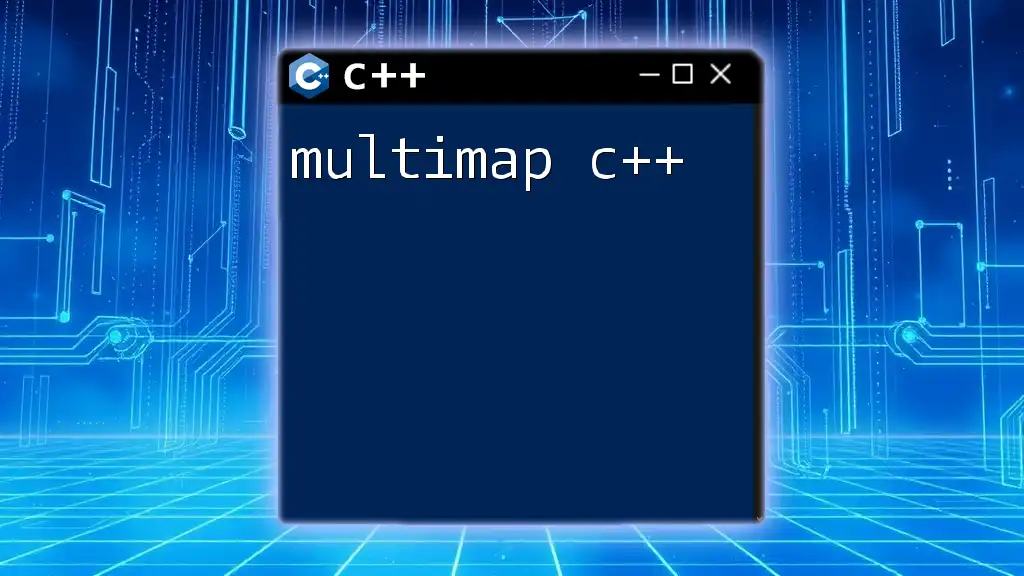
Performance Considerations
Time Complexity of `clear()`
The time complexity of the `clear()` function is O(n), where n is the number of elements in the map. This complexity arises because `clear()` needs to traverse and deallocate every element in the map to free up resources. Despite being linear, `clear()` is typically optimized for performance in practice.
Memory Usage After Clearing
After calling `clear()`, the map remains in existence, but all the elements are removed, and the memory used for these elements is returned to the system. This means that while the map is empty, it still retains its capacity for new elements, which can improve performance if new entries are added shortly after.
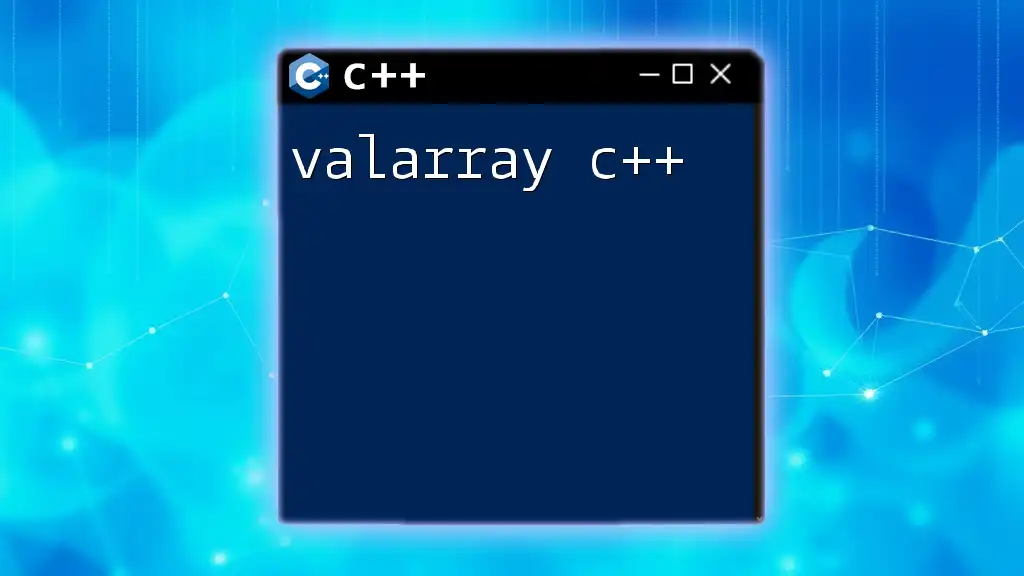
Alternative Approaches to Resetting Maps
Using the Assignment Operator
Another way to clear and reinitialize a map is by using the assignment operator, which allows you to replace the current map with a new one. For example:
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}};
myMap = std::map<int, std::string>(); // Reset using a new map
This method has the advantage of immediately resetting the map's capacity and can lead to more predictable memory use patterns. However, it generates a temporary object, which may have performance implications in memory-constrained environments.
Using `erase()` vs. `clear()`
Though `clear()` is the simplest way to remove all elements, you can also achieve a similar outcome using the `erase()` method. For instance, using `erase()` like this:
myMap.erase(myMap.begin(), myMap.end()); // Similar effect as clear()
This approach, while functionally equivalent, is less concise than `clear()` and is primarily used when a subset of elements needs to be removed.
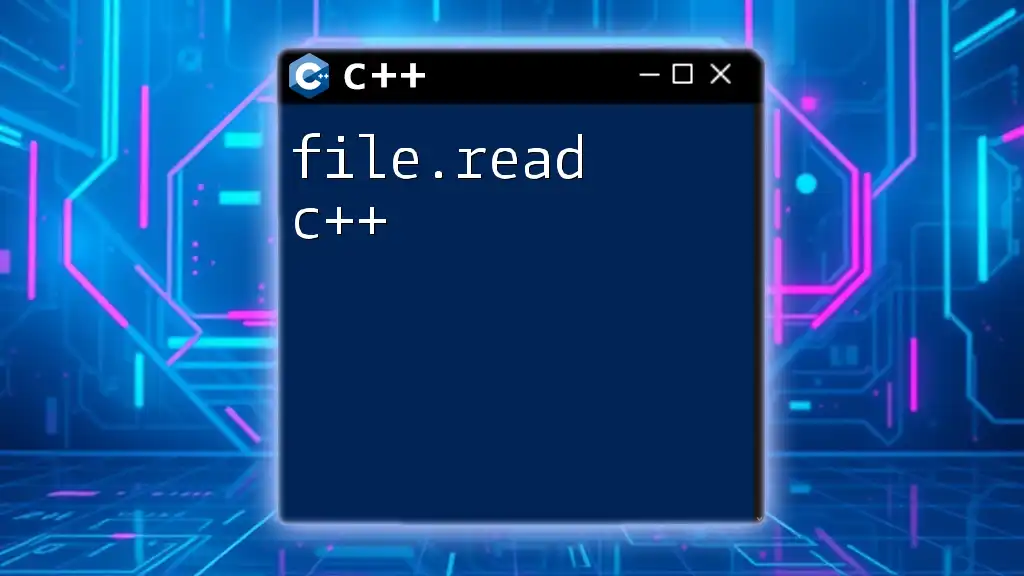
Conclusion
The `clear map c++` function is a fundamental tool for managing the contents of maps, providing a straightforward method to empty them without needing to recreate the entire map structure. Understanding how and when to use `clear()` in C++ maps allows developers to maintain efficient memory usage and performance in their applications. As you advance in your C++ journey, experimenting with `clear()` in various scenarios will deepen your comprehension of maps and enhance your programming skills. Always remember to share your experiences or challenges with map operations in the comments section below!
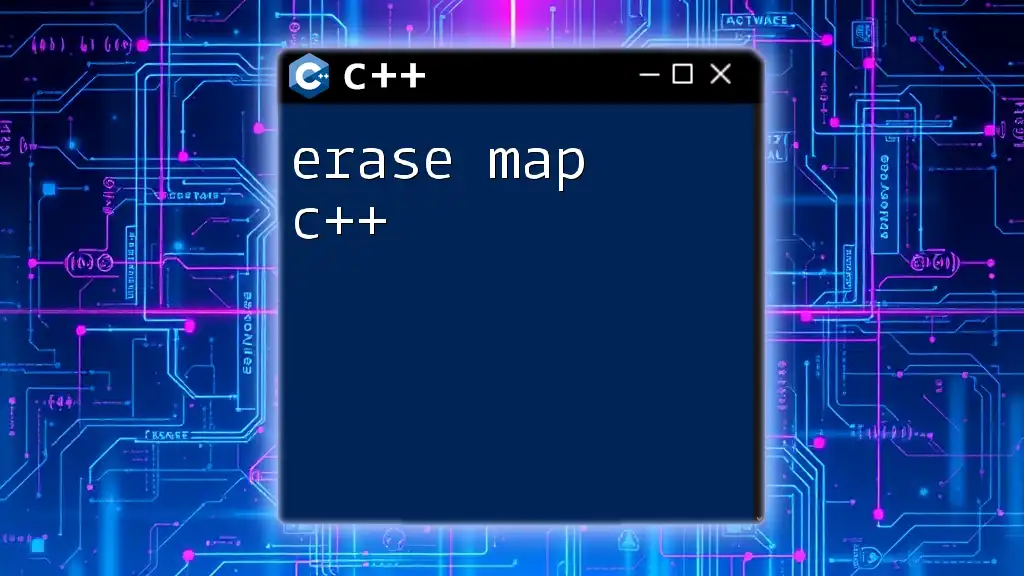
Additional Resources
For further reading, consider the official C++ documentation on maps and the `clear()` function. Additionally, there are numerous books and online courses available that delve deeper into STL containers and C++ programming fundamentals. If you have questions or need assistance, feel free to reach out for more learning resources!