In C++, an iterator for a map allows you to traverse the key-value pairs in the map container efficiently.
Here's a simple example of using an iterator with a `std::map`:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {
{1, "one"},
{2, "two"},
{3, "three"}
};
for (std::map<int, std::string>::iterator it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
Understanding C++ Maps
What is a Map in C++?
A map in C++ is a type of associative container that stores elements in key-value pairs. Each element, known as a pair, consists of a unique key and a corresponding value. The underlying data structure is usually implemented as a balanced binary tree, allowing for efficient insertion, deletion, and search operations.
Maps are widely used due to their fast look-up times, which are typically logarithmic with respect to the number of entries. This makes them far more efficient than linear structures like arrays when it comes to searching for elements by key.
Why Use Maps in C++?
Using maps offers several advantages over traditional data structures:
- Key-based Access: Maps allow you to access values using unique keys, making data retrieval intuitive.
- Automatic Sorting: By default, maps are sorted by their keys. This allows for ordered traversal of the elements.
- Efficient Operations: With logarithmic complexity for insertions and lookups, maps are ideal for managing large datasets where quick access is crucial.
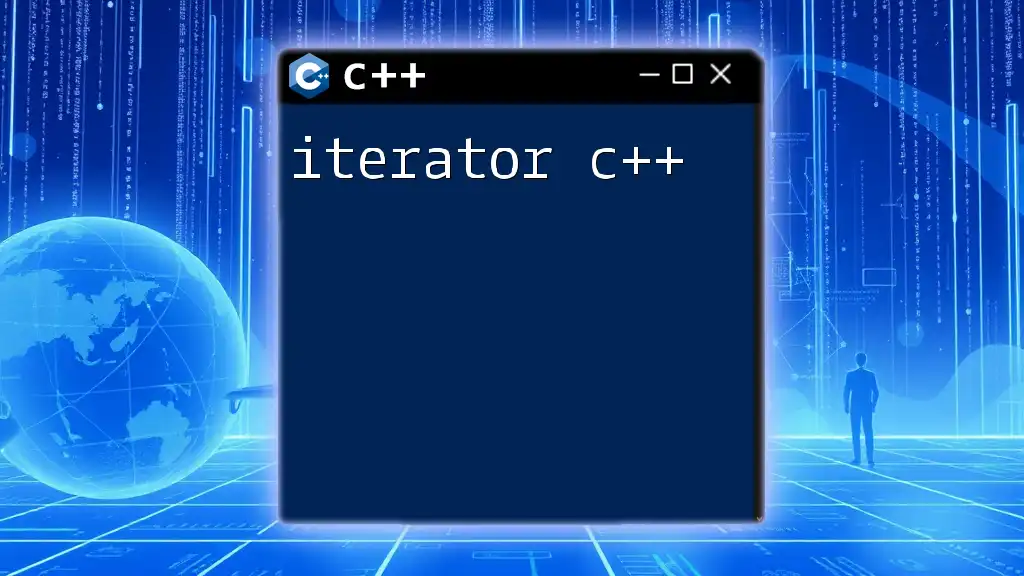
Introduction to Iterators
What is an Iterator?
An iterator is an object that enables you to traverse a container, such as a map, without exposing the underlying structure. Iterators are essential in C++ as they provide a uniform way to access the elements of various STL containers.
Types of Iterators in C++
There are several types of iterators available in C++:
- Input Iterators: Allow reading elements from a container.
- Output Iterators: Permit writing elements to a container.
- Forward Iterators: Can read and write elements but can only move forward.
- Bidirectional Iterators: Allow moving both forward and backward.
- Random Access Iterators: Support all operations of bidirectional iterators, along with jump-to operations and arithmetic.
Understanding these types will help you choose the right iterator for your specific needs.
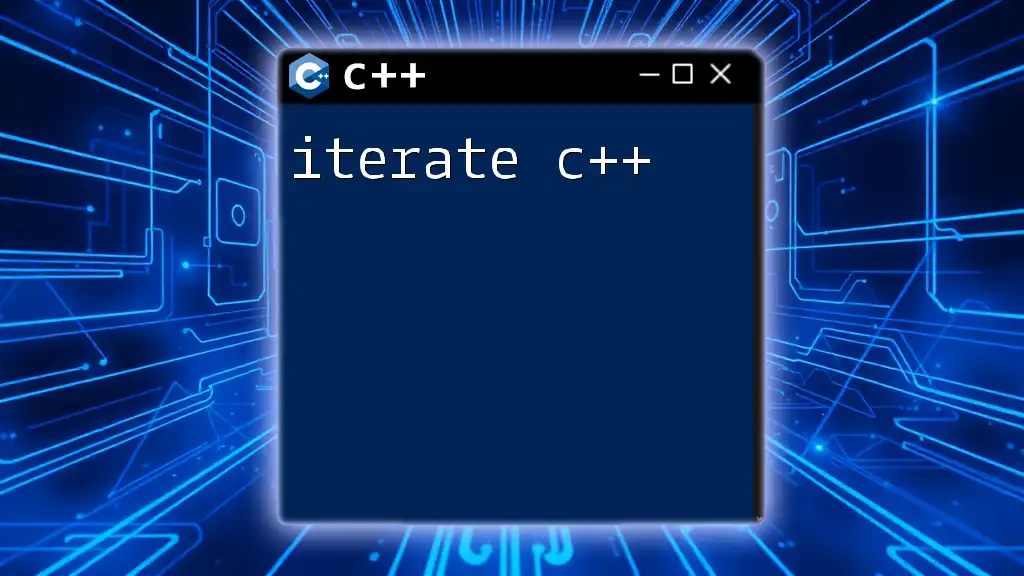
Map Iteration in C++
What is Map Iteration?
Map iteration is the process of traversing through the elements of a map to access its key-value pairs. This is crucial in scenarios where you need to read, modify, or display the entries stored in the map.
How to Iterate Over a Map
Using Basic Iterators
You can use the `map::iterator` and `map::const_iterator` to iterate through a map. The key here is to understand the syntax and the methods available for iterators.
Here's a simple example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
for (std::map<int, std::string>::iterator it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
In this example, we declare a map and iterate through its elements using a basic iterator. The `begin()` method returns an iterator pointing to the first element, and `end()` returns an iterator pointing past the last element. By incrementing the iterator `it`, we can traverse through the map.
Using Range-Based For Loops
C++11 introduced the range-based for loop, which simplifies the iteration process:
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This method enhances readability and is less error-prone, as you do not have to manage the iterator explicitly. The `auto` keyword automatically infers the type of the elements, making the code succinct.
Using C++11 Features for Map Iteration
Auto Keyword
The `auto` keyword simplifies the code further. By stating that you want to use `auto` in the loop, the compiler will determine the type for you, which can be especially useful when dealing with complex types:
for (auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This example is functionally identical to the previous one but eliminates the need to specify the type explicitly.
Lambda Expressions
Lambda expressions provide a powerful way to create inline functions, making it easy to perform operations on map entries:
std::for_each(myMap.begin(), myMap.end(), [](const std::pair<int, std::string>& pair) {
std::cout << pair.first << ": " << pair.second << std::endl;
});
This code utilizes the `std::for_each` algorithm along with a lambda function to iterate over `myMap`. This approach can enhance code readability by encapsulating logic directly in the iteration statement.
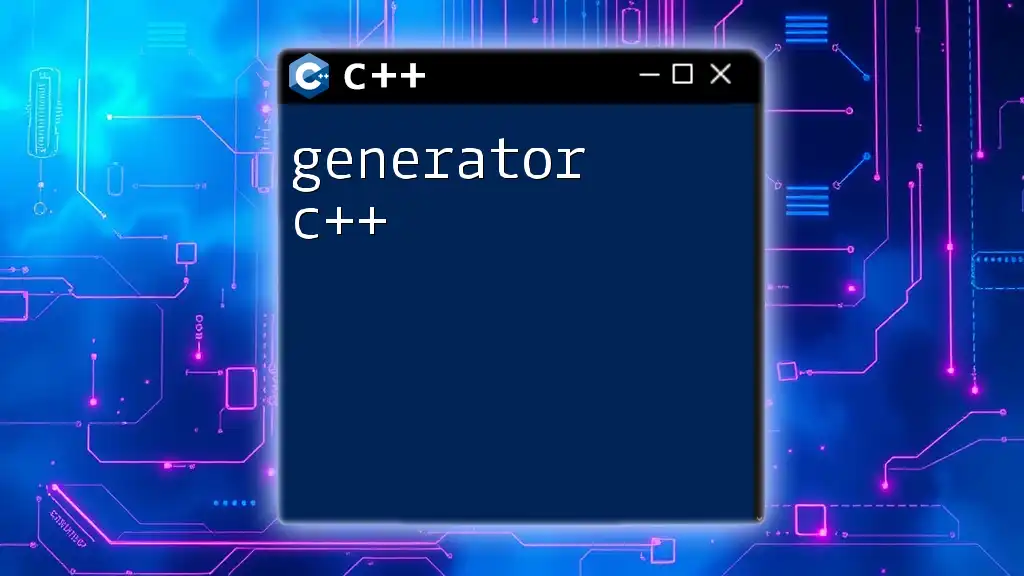
Conclusion
Summary of Key Points
In summary, understanding the use of the iterator map in C++ is essential for any C++ programmer. By leveraging the strengths of maps and iterators, you can create efficient and readable code. We explored various methods for iterating over maps, including basic iterators, range-based for loops, and advanced C++11 features like `auto` and lambda expressions.
Next Steps
Continue practicing map iteration and experiment with different projects. Further topics such as advanced STL features and custom iterators await, offering deeper insights into the powerful capabilities of C++.
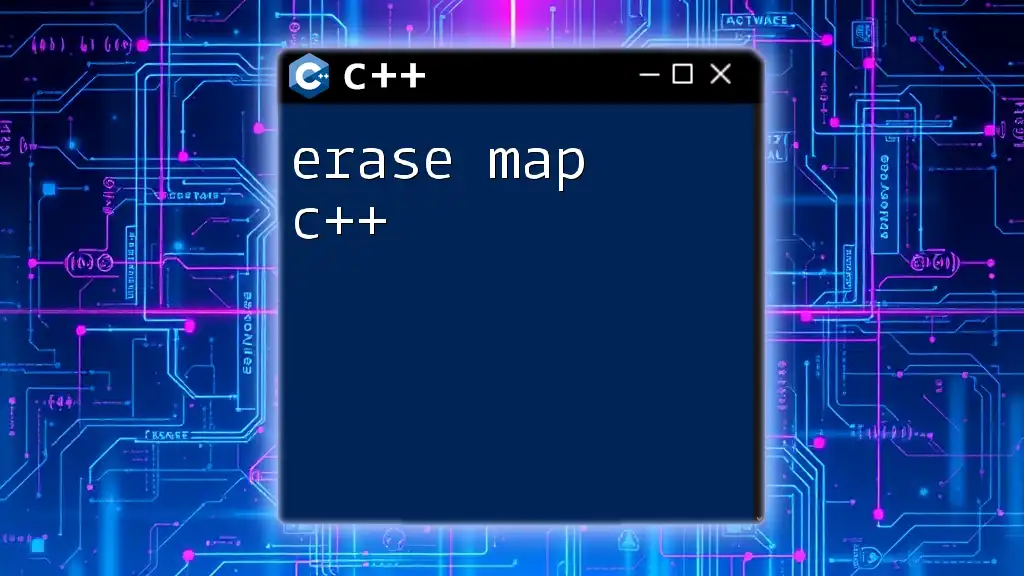
Call to Action
Join Us
If you found this article helpful, consider joining our community for more tutorials focused on C++ commands. Sign up for our newsletters or enroll in our courses to take your C++ skill set to the next level!
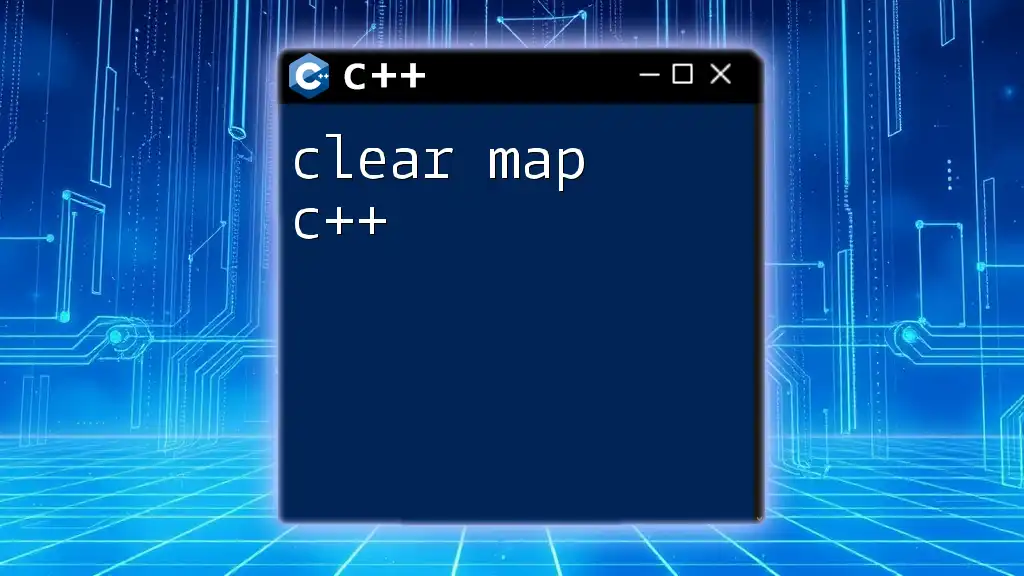
Additional Resources
For more detailed information, refer to the [official C++ documentation](https://en.cppreference.com/w/cpp/container/map) on maps and iterators. Explore suggested books and websites dedicated to expanding your C++ knowledge and skill set.
FAQs
- What should I do if an iterator becomes invalid? Ensure that you do not modify the container (e.g., insert/delete elements) while iterating through it with the current iterator.
- Can I iterate through a map in reverse order? Yes, you can use reverse iterators provided by C++ which can be accessed using `rbegin()` and `rend()` functions.