C++ was created by Bjarne Stroustrup in 1979 as an enhancement to the C programming language, introducing object-oriented features.
Here's a simple code snippet demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Evolution of C++
Birth of C
The story of C++ begins with the creation of the C language by Dennis Ritchie at Bell Labs in the early 1970s. C revolutionized programming by providing a powerful yet simple syntax, enabling programmers to write efficient system software. Its influence is still felt today, as many modern programming languages borrow extensively from C's structure and concepts.
C was designed with a focus on performance and flexibility, which made it an ideal choice for system-level programming. However, it lacked support for object-oriented programming (OOP), which is essential for managing complex software projects. This gap in functionality laid the groundwork for Bjarne Stroustrup's groundbreaking work.
Bjarne Stroustrup: The Visionary Behind C++
Bjarne Stroustrup, born in 1950 in Aarhus, Denmark, would become the creator of C++. His journey began with a strong foundation in mathematics and computer science, leading him to pursue a Ph.D. in computer science at the University of Cambridge. Stroustrup's experiences at Bell Labs inspired him to enhance C by integrating OOP principles, aiming to create a language that combined the efficiency of C with the flexibility of OOP.
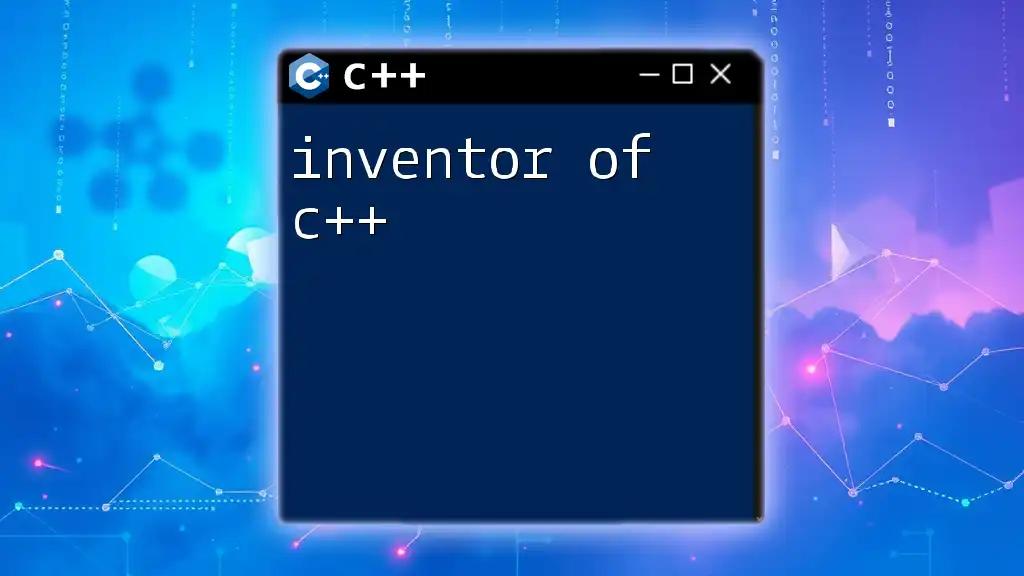
The Genesis of C++
What Inspired C++?
In the late 1970s, Stroustrup recognized the growing complexity of software systems. High-level languages like Simula 67 showcased OOP benefits but lacked C's performance. He sought to develop a language that combined the powerful capabilities of C with OOP features, ultimately leading to the creation of C++. This new language allowed for better organization of code and facilitated development on a larger scale.
Timeline of Development
Key milestones in the development of C++ include:
- 1980: Initial implementation of C++ began, introducing key features such as classes and basic inheritance.
- 1985: The first book on C++ was published, further solidifying the language's place in the programming world.
- 1998: The first official standard of C++ was released (C++98), establishing a foundation for future versions and ensuring global compatibility.
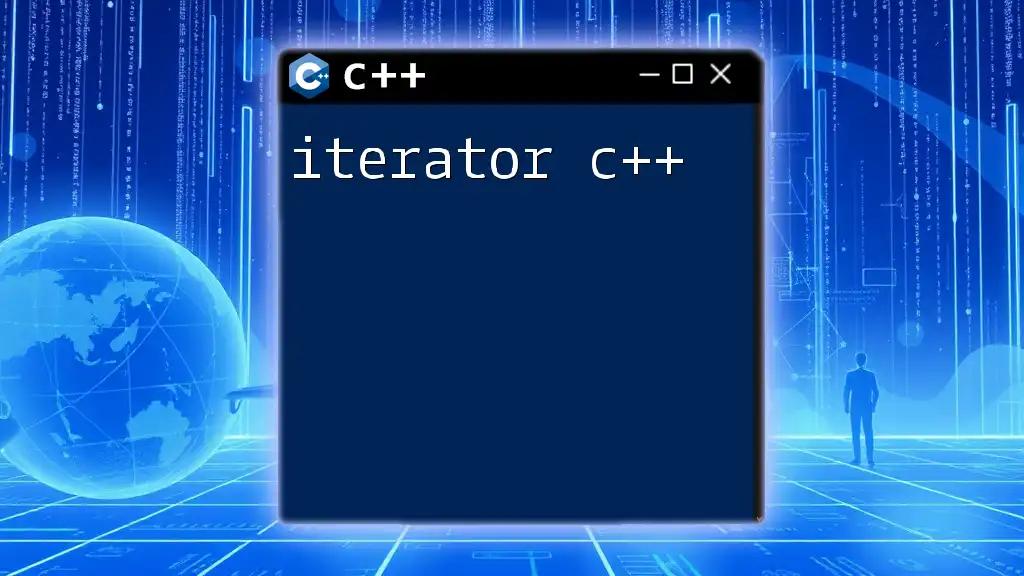
Core Principles of C++
Object-Oriented Programming (OOP) Concepts
C++ is built on the foundational principles of OOP, which include:
Encapsulation: This principle involves bundling data and methods that operate on that data within a single unit, usually known as a class. This helps to restrict direct access to some of an object's components.
class Rectangle {
private:
int width, height;
public:
void setDimensions(int w, int h) {
width = w;
height = h;
}
int area() {
return width * height;
}
};
Inheritance: Inheritance allows a new class to inherit properties and behaviors from an existing class, promoting code reusability.
class Shape {
public:
void setColor(string color) {
// set the color for the shape
}
};
class Circle : public Shape {
public:
void setRadius(int radius) {
// set radius
}
};
Polymorphism: This allows methods to do different things based on the object performing the action. C++ supports two types of polymorphism: compile-time (function overloading) and run-time (virtual functions).
class Animal {
public:
virtual void sound() {
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark" << endl;
}
};
Generic Programming
C++ also embraces the concept of generic programming, which enables developers to write flexible and reusable code through templates. By allowing functions and classes to operate on any data type, templates promote type safety and reduce redundancy.
template <typename T>
T add(T a, T b) {
return a + b;
}
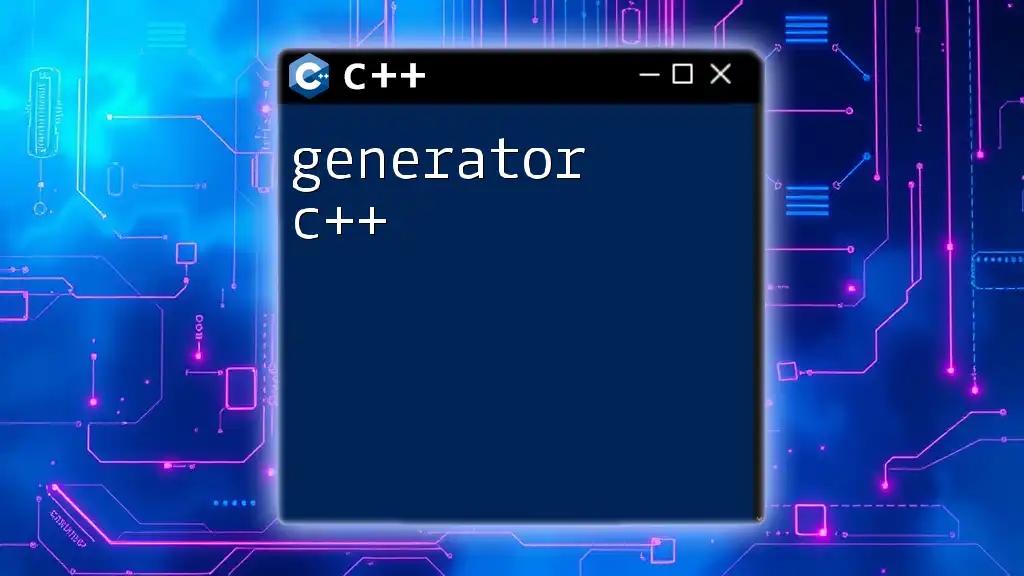
Impact of C++
C++ in the Software Industry
C++ has become an integral part of various industries, owing to its performance and versatility. Its applications range from system software and game development to financial systems and real-time simulations. Notable examples of software and games developed using C++ include:
- Operating Systems: Windows, Linux
- Game Engines: Unreal Engine, Unity
- Web Browsers: Google Chrome, Mozilla Firefox
- Finance Applications: Bloomberg Terminal, high-frequency trading platforms
C++ and Modern Programming
The evolution of C++ has kept it relevant in modern programming practices. Subsequent standards have introduced features that further extend C++'s capabilities and align it with the needs of contemporary developers. Each new version has brought enhancements, such as:
- C++11: Added auto keyword, range-based for loops, and smart pointers.
- C++14: Introduced variable templates and generic lambdas.
- C++17: Added std::optional, std::variant, and improved STL functionality.
- C++20: Introduced concepts and coroutines, further enhancing the language.

Bjarne Stroustrup's Philosophy
Design Goals of C++
Stroustrup's design philosophy aimed to create a language that was not only practical but also aligned with the complexities of modern software engineering. A few key goals included:
- Efficiency: C++ was designed to be as efficient as C, minimizing overhead for critical systems.
- Flexibility: The language allows developers to use different programming styles, from procedural to object-oriented and functional programming.
- Performance: Stroustrup emphasized that C++ should allow developers to write code that is close to hardware for high-performance applications.
Quotes from Bjarne Stroustrup
Stroustrup’s vision can be encapsulated in some of his well-known quotes:
- "The most important thing is not to stop questioning."
- "A programming language is software for building software."
These quotes reflect his belief in constant improvement and innovation within the programming community.
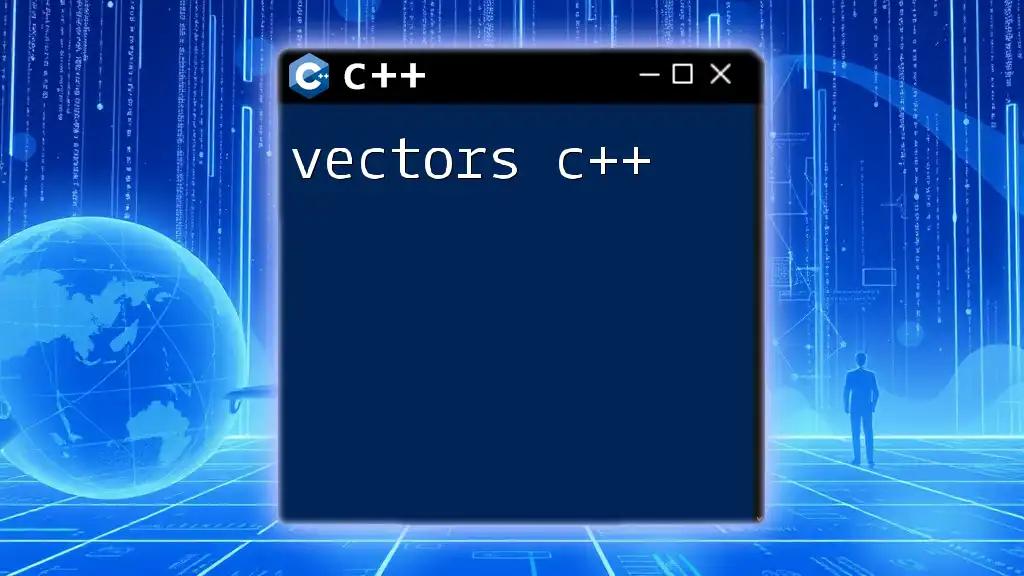
C++ Community and Contribution
Influence of Bjarne Stroustrup on the Developer Community
Bjarne Stroustrup has been an active participant in the programming community, leveraging conferences and educational initiatives to share his insights. His ongoing contributions include writing books, participating in forums, and speaking at international conferences, inspiring both new and experienced developers.
Ongoing Influence on C++ Standards
Stroustrup has continually influenced the direction of C++ through active participation in standards committees. His insights help ensure that C++ evolves in a way that meets the future needs of programmers while maintaining its core principles.

Conclusion
Bjarne Stroustrup, the creator of C++, has not only developed a powerful programming language but has also shaped the landscape of software development itself. His contributions are felt worldwide in diverse applications, from gaming to finance. The language he created continues to evolve, ensuring relevance in modern computing environments.

Call to Action
If you're keen to dive deeper into the world of C++, consider engaging with our community or enrolling in our C++ programming tutorials. Join us on our journey to unlock the full potential of C++ commands and techniques.

Additional Resources
For those looking to expand their knowledge further, we recommend the following:
Books and Articles by Bjarne Stroustrup
- The C++ Programming Language (3rd Edition)
- Programming: Principles and Practice Using C++
Online Communities and Forums
- Stack Overflow
- C++ Reddit Community
- C++ Programming Language Discussion Groups
With that, you are well-equipped to understand the history and contributions of the creator of C++.