A "Tour of C++" provides a quick overview of fundamental C++ concepts, showcasing key syntax and commands to help beginners get started efficiently.
Here's a simple example illustrating basic C++ syntax for defining a function that adds two numbers:
#include <iostream>
// Function to add two numbers
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 3);
std::cout << "The sum is: " << result << std::endl;
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is a powerful, high-performance programming language that supports both procedural and object-oriented programming paradigms. It is widely used in systems programming, game development, and applications requiring high performance. The language's foundation lies in C, allowing for direct manipulation of memory and excellent control over system resources.
Key Features of C++
C++ boasts numerous features that make it a favored choice among developers. Notable highlights include:
-
Standard Template Library (STL): A collection of pre-written classes and functions, STL provides a rich set of data structures and algorithms. It streamlines programming by allowing developers to use ready-made components instead of coding from scratch.
-
Memory Management: C++ gives programmers fine-grained control over memory allocation and deallocation, which is crucial for performance in applications where efficiency is paramount.
-
Portability and Performance: C++ code can be executed on various platforms with minimal modifications, making it a versatile choice for multiple environments.
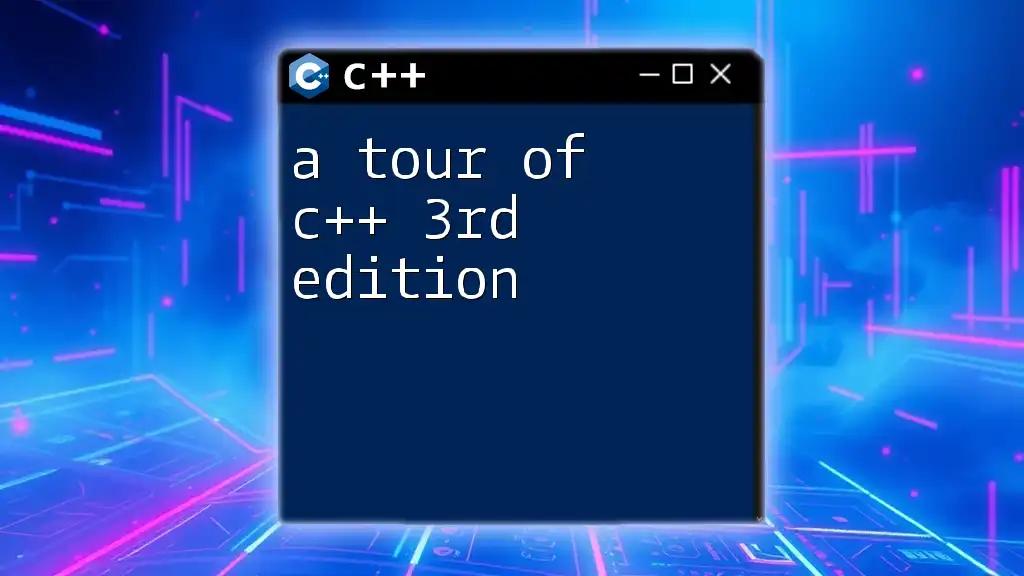
Setting Up Your C++ Environment
Choosing the Right IDE
Selecting an Integrated Development Environment (IDE) can significantly enhance the coding experience. Some popular options include:
- Visual Studio: Known for its robust features and user-friendly interface, Visual Studio is excellent for Windows developers.
- Code::Blocks: A versatile, open-source IDE suitable for beginners and experienced programmers alike.
- Eclipse: Ideal for C++ development with its powerful plugins and extensive community support.
Compiling and Running C++ Code
To run C++ code, you’ll need a compiler like GCC or Clang. Compiling transforms C++ source code into executable code.
A basic compile and run through the command line looks like this:
g++ your_program.cpp -o your_program
./your_program
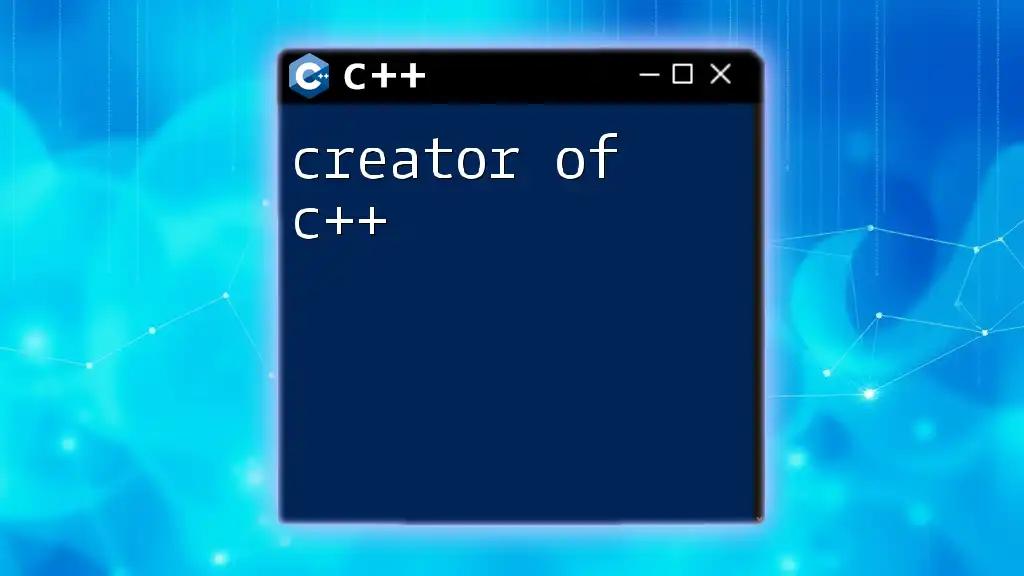
Core C++ Syntax and Structure
Basic Structure of a C++ Program
Every C++ program starts with the inclusion of essential headers and the definition of the main function. Below is a simple program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` imports the standard input-output stream library.
- `int main()` defines the main function, where program execution begins.
- `std::cout` outputs text to the console, and `std::endl` adds a new line. The `return 0;` statement indicates successful execution.
Data Types and Variables
In C++, data types are essential for defining the nature of data stored in variables. The most common data types include:
- int: Represents integer values.
- float: Represents floating-point numbers.
- char: Represents single characters.
- bool: Represents Boolean values (true or false).
Variable declaration and initialization are straightforward:
int age = 25;
float salary = 50000.50;
char initial = 'D';
bool isEmployed = true;
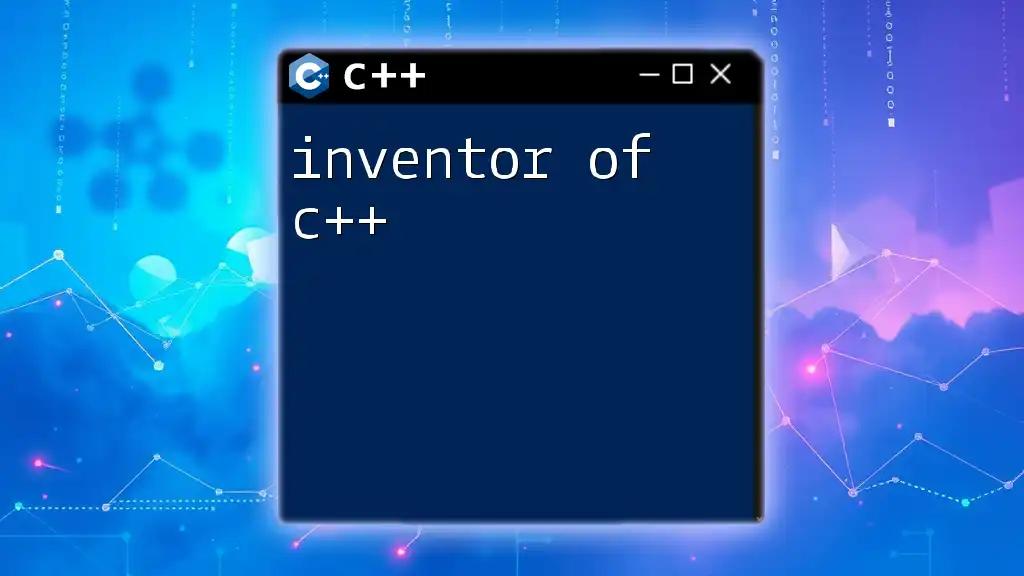
Control Structures
Conditional Statements
C++ employs conditional statements like `if`, `else`, and `switch` to control the flow of the program. This enables decision-making in your code. An example of an `if` statement follows:
int x = 10;
if (x > 5) {
std::cout << "x is greater than 5" << std::endl;
}
When `x` is greater than 5, the program outputs the message to the console.
Loops
Loops enable repetitive execution of code blocks. C++ provides several loop types, including `for`, `while`, and `do-while`. Here’s an example of a `for` loop iterating through an array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
This loop will print each element of the array to the console.
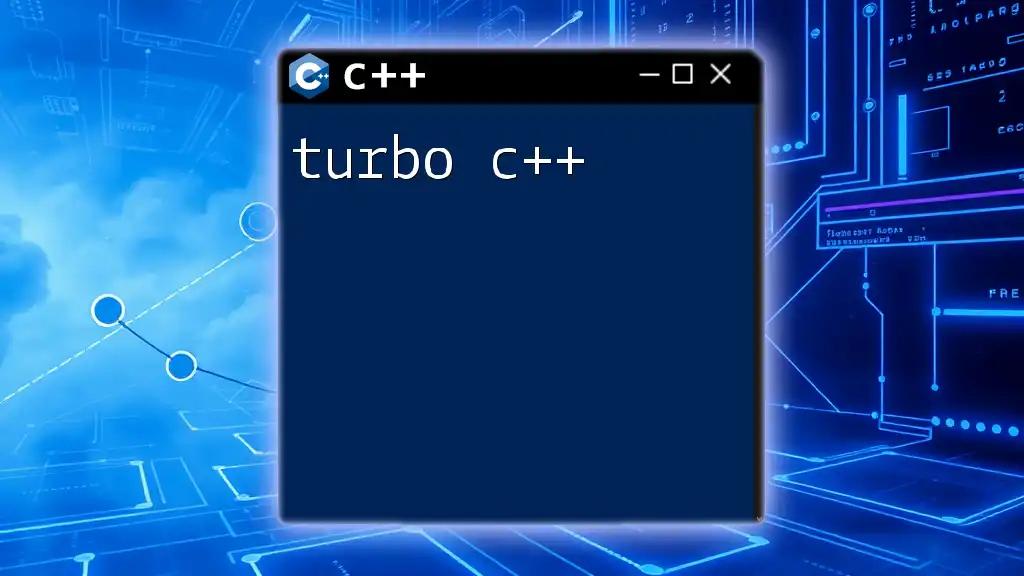
Functions
Defining Functions
Functions in C++ promote code reuse and modular programming. A function is defined with a return type, name, and parameters. Here's a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
This function can be called within the `main` function to get the sum of two numbers.
Function Overloading
C++ supports function overloading, allowing multiple functions to share the same name as long as they differ in parameters. An example is shown below:
int multiply(int a, int b) {
return a * b;
}
double multiply(double a, double b) {
return a * b;
}
Both functions `multiply` perform different tasks based on input parameters.
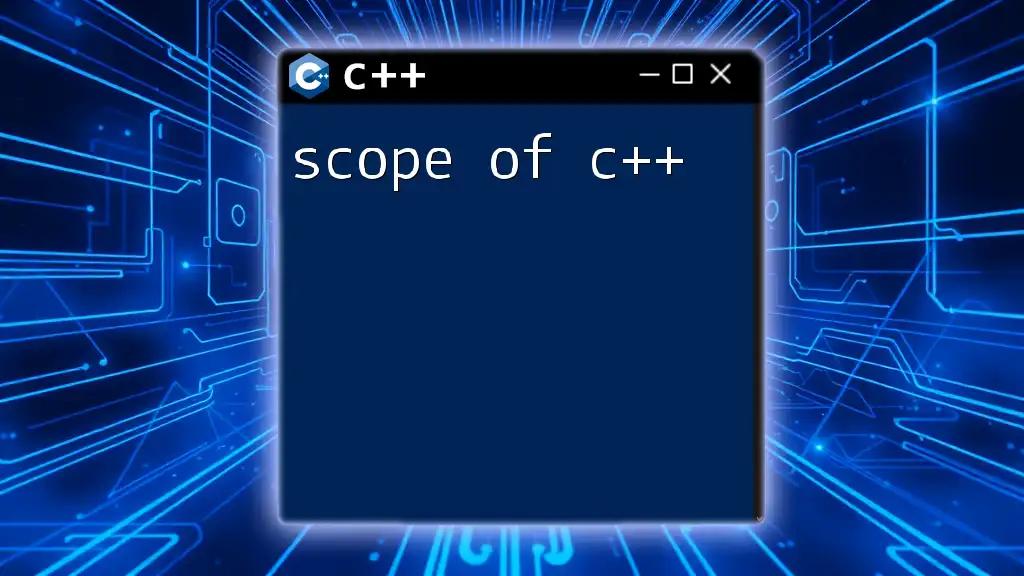
Object-Oriented Programming in C++
Classes and Objects
Central to C++ is the concept of object-oriented programming (OOP). A class defines a blueprint for objects, encapsulating data and functions. Here’s an example of a simple class called `Dog`:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Dog myDog;
myDog.bark();
This creates an object `myDog` and invokes the `bark` method.
Inheritance and Polymorphism
C++ supports inheritance, enabling classes to acquire properties and methods from other classes. This reduces code duplication and enhances reuse.
Polymorphism allows methods to do different things based on the object that it is operating on, fostering code flexibility. For example, a base class can have a method that is overridden by a derived class, enabling runtime decisions based on object types.
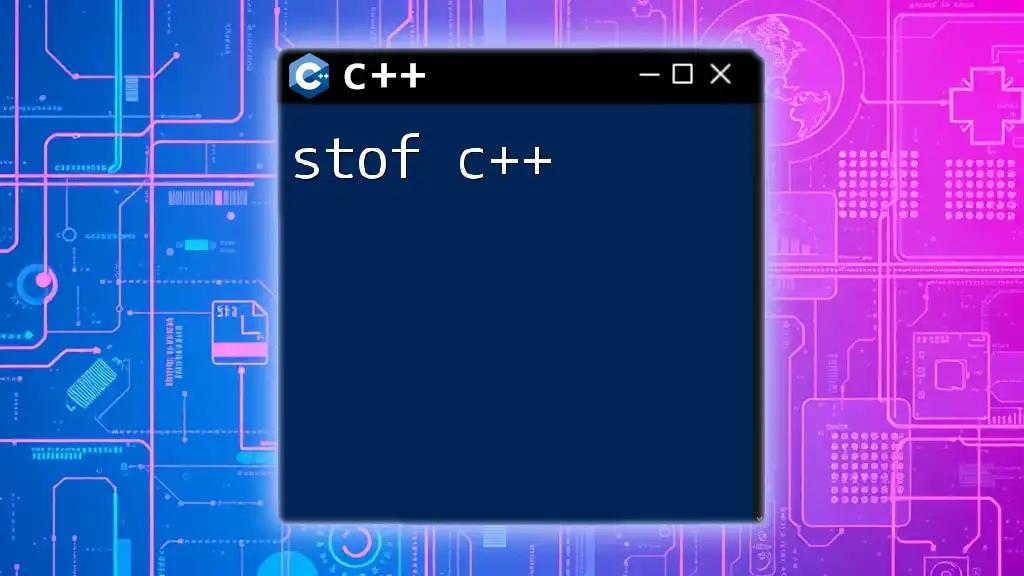
Memory Management
Dynamic Memory Allocation
C++ allows programmers to allocate memory dynamically using the `new` keyword. This offers the flexibility to create variables at runtime:
int* ptr = new int;
*ptr = 10;
std::cout << *ptr << std::endl;
delete ptr;
In this snippet, `new int` allocates memory for an integer, and `delete ptr` deallocates it, emphasizing the importance of managing memory responsibly to avoid leaks.
Smart Pointers
Smart pointers (e.g., `unique_ptr`, `shared_ptr`) automate memory management, providing automatic deletion of unused objects. This minimizes human error and is a best practice for professionals writing C++.
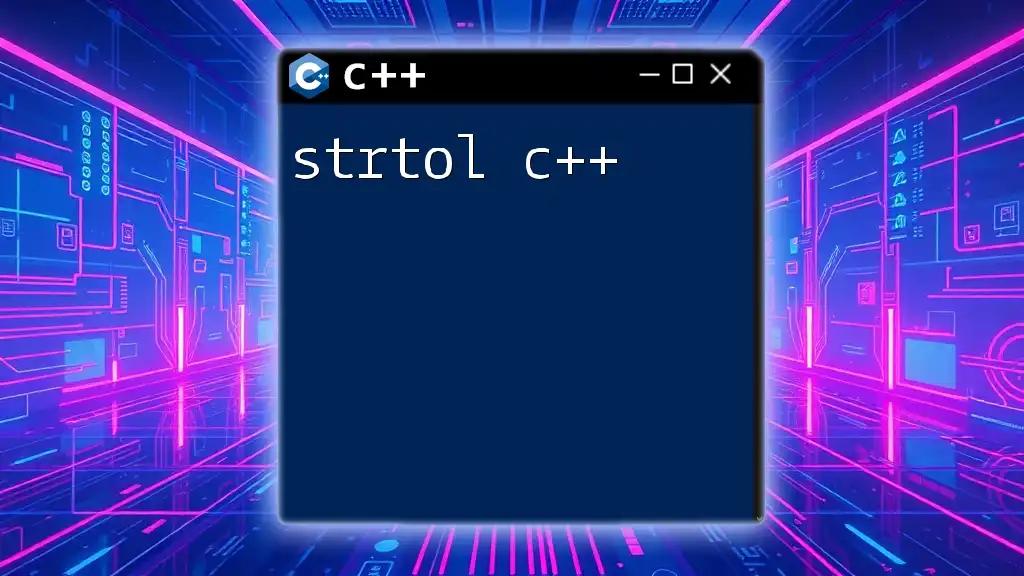
Standard Template Library (STL)
Introduction to STL
The Standard Template Library (STL) is an integral part of C++. It provides data structures, algorithms, and iterators that enhance productivity and efficiency in programming.
Commonly Used Containers
STL contains several data structures, including:
- Vectors: Dynamic arrays that can resize automatically.
- Lists: Doubly linked lists that allow fast insertions and deletions.
- Sets: Collections of unique elements, automatically sorted.
- Maps: Key-value pairs that offer fast lookup.
Here’s an example of using a vector in C++:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
This code demonstrates how to initialize a vector and iterate over its elements.
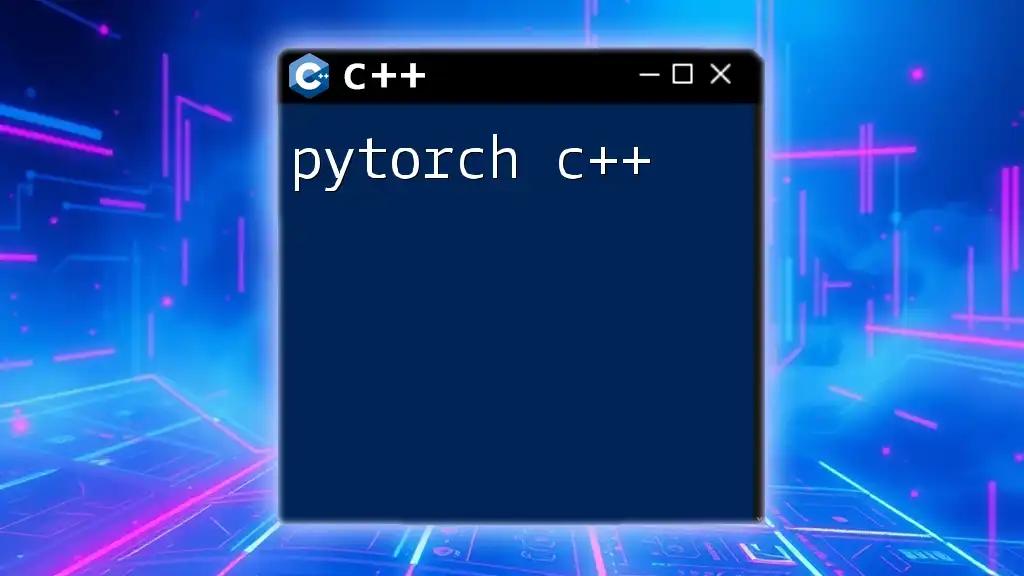
Advanced C++ Concepts
Templates
Templates are a powerful feature in C++, allowing functions and classes to operate with generic types. This increases code reusability and type safety. Below is an example of a template function that adds two values of any type:
template <typename T>
T add(T a, T b) {
return a + b;
}
Exception Handling
C++ has built-in mechanisms for error handling through exceptions. The `try`, `catch`, and `throw` keywords manage runtime errors gracefully, maintaining program stability. An example is as follows:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
In this code, an error is thrown and caught, demonstrating effective error management.
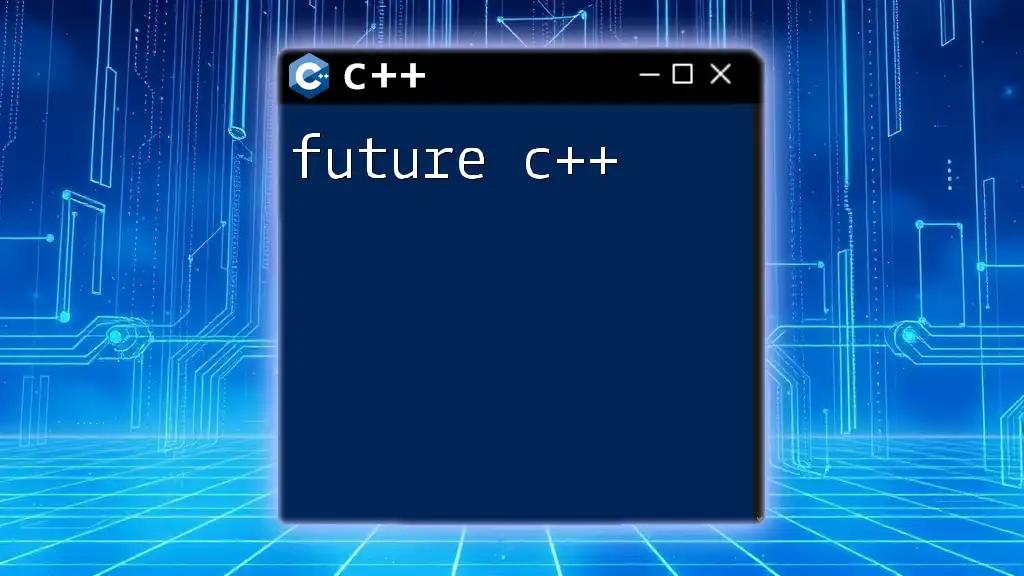
Conclusion
In this tour of C++, we explored the vast landscape of this versatile language, covering foundational concepts, syntax, advanced features, and best practices. As you delve deeper into C++, practice coding regularly and explore more complex projects to solidify your understanding. Continuous learning through online resources, books, and tutorials is key. Embrace the journey of mastering C++, and you will discover the powerful impact of this language on your programming career.